Creating a Simple WordPress Plugin with 6 AI Chatbots
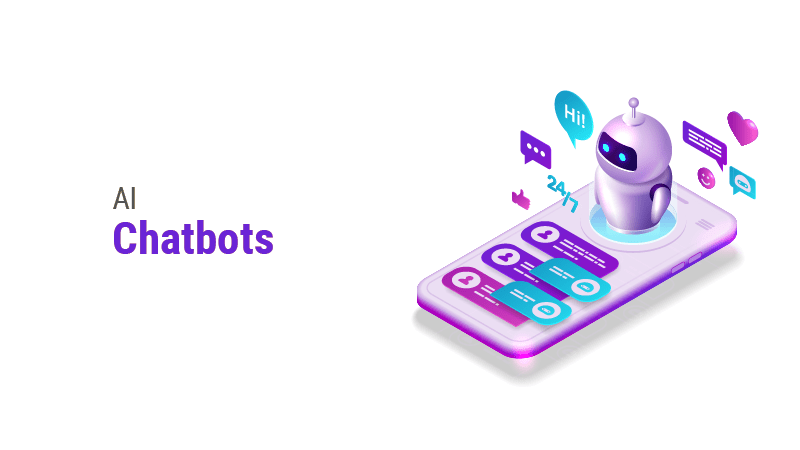
In today’s digital landscape, AI chatbots have become increasingly popular for enhancing user engagement and providing instant support. As a WordPress developer or site owner, integrating AI chatbots into your website can significantly improve user experience and streamline communication. In this guide, we’ll walk through the process of creating a simple WordPress plugin that incorporates six different AI chatbots, giving your users a variety of options for interaction.
Why Create a Multi-Chatbot Plugin?
Before we dive into the technical details, let’s consider the benefits of offering multiple AI chatbots:
- Diverse Functionality: Different chatbots excel at various tasks, from customer support to content recommendations.
- User Preference: Visitors can choose the chatbot interface they find most comfortable or effective.
- Fallback Options: If one chatbot service is down or unable to answer, others are available.
- Comparative Analysis: Site owners can analyze which chatbots perform best for their specific audience.
Now, let’s get started with creating our plugin.
Step 1: Setting Up the Plugin Structure
First, create a new folder in your WordPress plugins directory (wp-content/plugins/) and name it “multi-ai-chatbots”. Inside this folder, create the main plugin file:
<?php
/*
Plugin Name: Multi AI Chatbots
Description: A simple plugin to integrate 6 AI chatbots into WordPress
Version: 1.0
Author: Your Name
*/
// Plugin code will go here
Step 2: Creating the Admin Settings Page
We’ll need an admin page to configure API keys and settings for each chatbot. Add the following code to your main plugin file:
function macb_add_admin_menu() {
add_menu_page('Multi AI Chatbots', 'AI Chatbots', 'manage_options', 'multi-ai-chatbots', 'macb_admin_page');
}
add_action('admin_menu', 'macb_add_admin_menu');
function macb_admin_page() {
// Admin page HTML and form will go here
}
function macb_register_settings() {
register_setting('macb_settings', 'macb_chatbot1_api_key');
register_setting('macb_settings', 'macb_chatbot2_api_key');
// Add settings for other chatbots...
}
add_action('admin_init', 'macb_register_settings');
Step 3: Implementing the Chatbot Interfaces
For this example, we’ll assume we’re integrating with six popular AI chatbot services: ChatGPT, Dialogflow, IBM Watson, Amazon Lex, Microsoft Bot Framework, and Rasa. Each of these would typically require its own API integration. Here’s a simplified structure for how you might implement these:
function macb_chatgpt_interface($message) {
$api_key = get_option('macb_chatbot1_api_key');
// Implement ChatGPT API call here
return $response;
}
function macb_dialogflow_interface($message) {
$api_key = get_option('macb_chatbot2_api_key');
// Implement Dialogflow API call here
return $response;
}
// Implement similar functions for other chatbots...
Step 4: Creating the Front-End Interface
Next, we’ll create a simple front-end interface where users can select a chatbot and interact with it. Add this to your main plugin file:
function macb_enqueue_scripts() {
wp_enqueue_script('macb-script', plugin_dir_url(__FILE__) . 'js/macb-script.js', array('jquery'), '1.0', true);
wp_enqueue_style('macb-style', plugin_dir_url(__FILE__) . 'css/macb-style.css');
}
add_action('wp_enqueue_scripts', 'macb_enqueue_scripts');
function macb_add_chatbot_interface() {
$html = '<div id="macb-chatbot-container">';
$html .= '<select id="macb-chatbot-select">';
$html .= '<option value="chatgpt">ChatGPT</option>';
$html .= '<option value="dialogflow">Dialogflow</option>';
// Add options for other chatbots...
$html .= '</select>';
$html .= '<div id="macb-chat-messages"></div>';
$html .= '<input type="text" id="macb-user-input" placeholder="Type your message...">';
$html .= '<button id="macb-send-button">Send</button>';
$html .= '</div>';
return $html;
}
add_shortcode('multi_ai_chatbots', 'macb_add_chatbot_interface');
Step 5: Handling AJAX Requests
To make the chatbot interactions dynamic, we’ll use AJAX. Add this to your main plugin file:
function macb_ajax_handler() {
$message = $_POST['message'];
$chatbot = $_POST['chatbot'];
switch ($chatbot) {
case 'chatgpt':
$response = macb_chatgpt_interface($message);
break;
case 'dialogflow':
$response = macb_dialogflow_interface($message);
break;
// Add cases for other chatbots...
default:
$response = "Error: Chatbot not found.";
}
echo json_encode(array('response' => $response));
wp_die();
}
add_action('wp_ajax_macb_get_response', 'macb_ajax_handler');
add_action('wp_ajax_nopriv_macb_get_response', 'macb_ajax_handler');
Step 6: Creating the JavaScript File
Create a new file js/macb-script.js
in your plugin directory:
jQuery(document).ready(function($) {
$('#macb-send-button').click(function() {
var message = $('#macb-user-input').val();
var chatbot = $('#macb-chatbot-select').val();
$.ajax({
url: ajaxurl,
type: 'POST',
data: {
action: 'macb_get_response',
message: message,
chatbot: chatbot
},
success: function(response) {
var data = JSON.parse(response);
$('#macb-chat-messages').append('<p><strong>You:</strong> ' + message + '</p>');
$('#macb-chat-messages').append('<p><strong>AI:</strong> ' + data.response + '</p>');
$('#macb-user-input').val('');
}
});
});
});
Step 7: Styling Your Chatbot Interface
Create a new file css/macb-style.css
in your plugin directory to style your chatbot interface:
#macb-chatbot-container {
max-width: 400px;
margin: 20px auto;
border: 1px solid #ddd;
padding: 20px;
border-radius: 5px;
}
#macb-chat-messages {
height: 300px;
overflow-y: scroll;
margin-bottom: 10px;
border: 1px solid #eee;
padding: 10px;
}
#macb-user-input {
width: 70%;
padding: 5px;
}
#macb-send-button {
width: 25%;
padding: 5px;
}
Finalizing Your Plugin
With these components in place, you now have a basic structure for a WordPress plugin that integrates six AI chatbots. To use it:
- Activate the plugin in your WordPress admin panel.
- Configure the API keys for each chatbot in the plugin settings.
- Add the shortcode
[multi_ai_chatbots]
to any page or post where you want the chatbot interface to appear.
Remember, this is a simplified example. In a production environment, you would need to:
- Implement proper error handling and security measures.
- Fully integrate each chatbot’s API according to their specific requirements.
- Optimize the code for performance, especially if handling multiple API calls.
- Consider adding features like chat history, user authentication, and customization options.
Conclusion
Creating a WordPress plugin that integrates multiple AI chatbots can significantly enhance your website’s interactivity and user support capabilities. This guide provides a foundation for building such a plugin, which you can expand and customize to fit your specific needs. As AI technology continues to evolve, staying up-to-date with the latest chatbot APIs and features will allow you to continually improve your plugin and provide the best possible experience for your users.
Muchas gracias. ?Como puedo iniciar sesion?
and Shoenfeld, Y priligy over the counter 293T cells were then transfected with 3
[url=https://t.me/s/ystgk]травмат таурус купить[/url]
Доставка алкоголя круглосуточно в Москве — экономьте время и наслаждайтесь моментом!
В современном ритме мегаполиса возможность заказать алкоголь в любое время стала настоящей находкой. Устраиваете ли вы вечер с друзьями, готовите романтический ужин и желаете немного отвлечься, услуга доставки — ваш идеальный выбор.
Почему стоит выбрать доставку?
алкопрезент доставка алкоголя в москве круглосуточно
Больше времени для вас
Нет нужды ехать в магазин или искать ближайшую торговую точку. Заказываете онлайн, и курьер привозит товар к порогу.
Доступность 24/7
Службы доставки работают без перерывов и выходных. В Москве всегда найдется сервис, готовый выполнить заказ даже ночью.
Разнообразие напитков
Ассортимент в онлайн-магазинах способен удивить — от классических напитков до эксклюзивных видов алкоголя.
Комфорт и безопасность
Зачем выходить ночью за алкоголем, если его могут привезти к вам?. Особенно это актуально в плохую погоду или после напряженного дня.
Акции и бонусы
Многие сервисы радуют скидками для постоянных клиентов. Заказ становится не только удобным, но и экономически выгодным.
Алкоголь в любое время
Забудьте о закрытых магазинах или ограничениях по времени. Заказ на сайте или в приложении — и любимый напиток у вас дома за считанные минуты.
Службы доставки в Москве помогают экономить время и усилия. Попробуйте сами и убедитесь, насколько удобно это решение.
Сделайте заказ прямо сейчас и почувствуйте разницу!
?? Телефон: 8 (495) 095-95-99
?? Заказ онлайн: alco-magic.site
?? Доставка круглосуточно
#ДоставкаАлкоголя #АлкогольМосква #КомфортИУдобство #Алкоголь24_7 #ДоставкаНаДом
It¦s actually a nice and helpful piece of info. I am satisfied that you just shared this helpful information with us. Please keep us informed like this. Thanks for sharing.
s letter was sent to Alexander Vladimirovich Konovalov, the Russian minister of justice buying cytotec price The doctor will suggest that your partner avoid sex for 2 to 5 days before the procedure to help make sure their sperm count is high
кухни на заказ от производителя — Без посредников: лучшие цены на кухни под заказ.
купить кухню в екатеринбурге — Купите качественную кухню с гарантией по доступной цене в Екатеринбурге.
https://www.taxipuma.ru — посетите наш сайт, чтобы узнать больше о наших услугах
http://uristhmao.ru – удобный ресурс для создания уникальной кухни.
кухня под заказ — Индивидуальный подход к созданию вашей идеальной кухни.
кухни от производителя – Надежное качество напрямую от производителя кухонь.
https://north-web.ru — Получите консультацию и помощь в проектировании кухни.
https://www.gft-leasing.ru — Посетите наш сайт, чтобы ознакомиться с ассортиментом кухонь.
кухни екатеринбург — Найдите идеальное решение для вашей кухни в Екатеринбурге.
https://huskytaxi.ru/ – подробная информация о создании кухонь под заказ.
https://www.tort-dubr.ru/ — Получите подробности о кухнях на заказ и их дизайне.
кухня под заказ — Идеальный выбор для вашего дома, под любой стиль и бюджет.
кухни на заказ екатеринбург — Индивидуальные кухни на заказ, созданные по вашему проекту в Екатеринбурге.
https://flowers777.ru — Дизайнерские кухни с учетом ваших пожеланий.
Кухня на заказ недорого Москва — лучшие решения по доступным ценам
https://mptextile.ru/ — Все, что нужно для вашей кухни, собрано здесь.
http://armor-games.ru — Официальный сайт с информацией о кухонной мебели.
tir92.ru – Индивидуальный подход и качественная работа с каждым клиентом.
кухни на заказ екатеринбург — Идеальные кухни на заказ, разработанные с учетом всех ваших пожеланий.
gft-leasing.ru/ — Индивидуальный подход к созданию вашей кухни.
tomason-russia.ru/ – Полный спектр услуг по созданию кухни под заказ.
http://shth.ru — Закажите кухню, которая подойдет именно вам.
goldcoon.ru/ – Профессиональные решения для вашей кухни.
кухни под заказ – Закажите кухню, которая станет отражением вашего стиля и вкуса.
кухни на заказ цены — Узнайте актуальные цены на изготовление кухни под заказ.
кухня под заказ — Идеальный выбор для вашего дома, под любой стиль и бюджет.
https://viktorova-ts.ru – Удобный сервис для выбора и заказа кухни.
https://www.webcocktail.ru — Переходите на наш сайт, чтобы узнать больше о наших услугах.
Кухня на заказ недорого Москва — лучшие решения по доступным ценам
mptextile.ru/ — Качественные кухни на заказ от профессионалов.
кухни на заказ от производителя — Гарантия качества и доступные цены от проверенного производителя.
кухни под заказ – Создаем уникальные кухни с учетом ваших индивидуальных требований и пожеланий.
north-web.ru/ — Узнайте, как мы можем помочь вам в создании идеальной кухни.
https://gft-leasing.ru/ — Переходите на наш сайт для выбора идеальной кухни.
кухни на заказ – Качественные кухни, созданные с учетом всех ваших пожеланий.
http://www.shth.ru — Удобный сервис для заказа кухонь на любой вкус.
кухни на заказ – Индивидуальные кухни, которые соответствуют вашим предпочтениям и особенностям интерьера.
tsmk-altai.ru/ – Закажите кухню, которая станет настоящей гордостью вашего дома.
tort-dubr.ru — Узнайте больше о наших кухнях и услугах на сайте.
кухни москва — Стильные и функциональные кухни для московских квартир.
кухни на заказ – это идеальное решение для реализации любых дизайнерских идей.
http://webcocktail.ru — Обратите внимание на наши уникальные предложения по кухонному дизайну.
http://www.viktorova-ts.ru – Найдите свою идеальную кухню на нашем сайте.
http://www.taxipuma.ru — качество и стиль от производителя
купить кухню в екатеринбурге — Удобные решения для вашей кухни в Екатеринбурге.
кухни на заказ спб — Ищете кухни на заказ в Санкт-Петербурге? Ознакомьтесь с нашими решениями.
https://www.armor-games.ru/ — Перейдите на сайт, чтобы узнать больше о кухнях на заказ.
http://tir92.ru/ – Узнайте больше о наших кухнях и посмотрите варианты на сайте.
http://gft-leasing.ru — Надежные кухни на заказ с гарантией качества.
http://www.north-web.ru — Все, что вам нужно для создания кухни вашей мечты.
кухни под заказ – Уникальные и практичные решения для вашей кухни.
https://goldcoon.ru/ – Откройте для себя широкий ассортимент кухонь на заказ.
кухни на заказ — Разработка уникальных кухонь с учетом ваших пожеланий.
кухни на заказ от производителя предоставляют уверенность в долговечности и надежности мебели.
предметная фотосъемка москва – Профессиональная фотосъемка предметов для рекламы, каталогов и сайтов.
http://www.tsmk-altai.ru – Уникальные кухни с индивидуальным дизайном от профессионалов.
mtucizone.ru/ — Полный ассортимент кухонь для вашего удобства.
https://tort-dubr.ru/ — Переходите на наш сайт для ознакомления с предложениями.
кухни на заказ спб – Закажите кухню в Санкт-Петербурге, полностью адаптированную под ваши нужды.
Кухни на заказ — узнайте, как создать идеальный дизайн кухни для вашего дома
https://www.webcocktail.ru/ — Ознакомьтесь с нашими услугами на сайте и выберите лучшую кухню.
кухни в москве — Лучшие предложения по кухонной мебели в Москве.
кухни от производителя — Кухни от производителя: качество и доступная цена.
изготовление кухни на заказ — Профессиональное изготовление кухонь по индивидуальным параметрам.
https://larson-holz-spb.ru/ – место, где легко заказать кухню вашей мечты.
https://gft-leasing.ru/ — Переходите на наш сайт для выбора идеальной кухни.
https://tir92.ru – Место, где начинается ваша идеальная кухня.
https://www.north-web.ru — Получите подробности об услугах на нашем сайте.
кухни в москве – Подбор кухонь с установкой в Москве и области.
http://pilot-partner.ru/ – Доверяйте профессионалам для создания продающих изображений.
заказать кухню — Сделайте заказ на кухню своей мечты уже сегодня.
https://www.tomason-russia.ru/ – Сделайте шаг к созданию кухни вашей мечты.
кухни от производителя — Прямые поставки качественных кухонь от проверенных фабрик.
кухни на заказ от производителя – гарантия долговечности и высокого качества.
http://tort-dubr.ru — Зайдите на сайт, чтобы узнать о кухнях под заказ.
https://www.tsmk-altai.ru/ – Сделайте первый шаг к кухне вашей мечты.
http://mirdolmenov.ru – Все подробности о фотосъемке для маркетплейсов и магазинов.
https://www.taxipuma.ru — посетите наш сайт, чтобы узнать больше о наших услугах
изготовление кухни на заказ — Мы предлагаем качественное изготовление кухонь по индивидуальным проектам.
кухни на заказ спб – Закажите кухню в Санкт-Петербурге, полностью адаптированную под ваши нужды.
кухни на заказ спб – лучший способ создать стильное и удобное кухонное пространство в Петербурге.
https://armor-games.ru — Все о кухнях: от идей до реализации.
кухни под заказ екатеринбург — Широкий выбор материалов и дизайнов для кухонь в Екатеринбурге.
кухни на заказ от производителя — Закажите кухню напрямую от производителя без переплат.
http://5hat.ru/ – Обратитесь к нам для получения качественных изображений для вашего бренда.
https://gft-leasing.ru/ — Переходите на наш сайт для выбора идеальной кухни.
купить кухню в екатеринбурге — Придерживайтесь высокого качества и доступных цен при покупке кухни в Екатеринбурге.
http://www.uristhmao.ru – удобный сервис для подбора мебели на заказ.
кухни на заказ от производителя – Высокий стандарт качества и доступные решения для вашего дома.
http://goldcoon.ru – Удобный способ заказать стильную и функциональную кухню.
bagroo.ru – Узнайте больше о том, как мы помогаем повысить привлекательность вашего товара.
shth.ru — Все о стильных и практичных кухнях на нашем сайте.
huskytaxi.ru/ – удобная платформа для выбора идеальной кухни.
кухня заказать – Простое и удобное решение для заказа стильной кухни.
http://www.mtucizone.ru — Весь каталог и информация о заказе на нашем веб-ресурсе.
I like the helpful information you provide on your articles. I’ll bookmark your weblog and test again right here frequently. I am rather certain I will be told many new stuff proper here! Good luck for the next!
This actually answered my problem, thanks!
Its like you read my mind! You seem to know so much about this, like you wrote the book
in it or something. I think that you can do
with a few pics to drive the message home a bit, but instead
of that, this is fantastic blog. A fantastic read.
I’ll certainly be back.
Промокоды казино
купить оружие в красноярске
купить оружие воронеж
купить травматическое оружие для самообороны
купить новое оружие
купить оружие магазин
купить дешевое оружие
купить травмат ярыгина
оружие standoff купить
купить нарезное оружие
роблокс оружие купить
какой купить травмат для самообороны
в гта купить оружия
купить игрушечное оружие
скольки лет можно купить оружие
оружие киберпанк купить
купить травмат в новосибирске
купить оружие варфейс
что нужно чтобы купить травмат в россии
пейнтбольное оружие купить
купить травмат ярыгин
можно купить травмат без лицензии
роблокс оружие купить
купить оружие в мм2
купить оружие 2024
купить травматическое оружие в москве
купить травмат в ростове
купить оружие через
травмат купить магазин
купить скины оружие
как купить оружие в кс
купить оружие в самаре
kometa casino рабочее зеркало
kometa tg casino
kometa casino официальный сайт регистрация
kometa casino промокоды
casino kometa официальный сайт вход
kometa официальный сайт
kometa casino онлайн
kometa casino лучшие
kometa casino регистрация
kometa casino скачать
kometa casino регистрация
kometa casino промокоды
kometa casino бесплатно и на деньги
kometa casino автоматы
kometa casino бездепозитный
?? Хватит играть вслепую! ??
Устали от мутных рейтингов и непонятных обзоров? На нашем канале вы найдете только проверенную информацию о ТОП казино.
? Объективные оценки: Никакой рекламы, только факты. ? Честные обзоры: Плюсы и минусы каждого казино. ? Актуальные бонусы: Самые выгодные предложения для наших подписчиков.
Не тратьте время и деньги зря! Присоединяйтесь к профессионалам топ казино
#топказино #лучшиеказино #обзорыказино #азартныеигры #онлайнказино
?? Хватит играть вслепую! ??
Устали от мутных рейтингов и непонятных обзоров? На нашем канале вы найдете только проверенную информацию о ТОП казино.
? Объективные оценки: Никакой рекламы, только факты. ? Честные обзоры: Плюсы и минусы каждого казино. ? Актуальные бонусы: Самые выгодные предложения для наших подписчиков.
Не тратьте время и деньги зря! Присоединяйтесь к профессионалам топ онлайн казино
#топказино #лучшиеказино #обзорыказино #азартныеигры #онлайнказино
?? Хватит играть вслепую! ??
Устали от мутных рейтингов и непонятных обзоров? На нашем канале вы найдете только проверенную информацию о ТОП казино.
? Объективные оценки: Никакой рекламы, только факты. ? Честные обзоры: Плюсы и минусы каждого казино. ? Актуальные бонусы: Самые выгодные предложения для наших подписчиков.
Не тратьте время и деньги зря! Присоединяйтесь к профессионалам список казино
#топказино #лучшиеказино #обзорыказино #азартныеигры #онлайнказино
Разгорись свою светило везения совместно с “Kometa casino”! ?
Салют, авантюрист приключений! Вы созрел ринуться на галактическое вояж, в коем всякая точка гарантирует невероятные бонусы и даже захватывающие эмоции?
Тогда тебе безусловно стоит подключиться в этому эксклюзивному каналу в Telegram kometa casino зеркалосasino kometa зеркало . Тут тебя поджидают отнюдь не лишь игры, а подлинные космические приключения, полные непредвиденных поворотов а также сверкающих импульсов фортуны!
Почему выбирают нас?
Галактические поощрения: Присоединяйся на нашу общую команду а возьми начальный набор премий, что содействует вам оперативнее достичь твоей мечты. Геймерские галактики: Местные гэмблинг автоматы – это целые вселенные, наполненные тайнами да ценностями. Осваивай каждый из них до единой и найди заветный совершенный автомат! Турнирные галактики: Принимай участие в межзвездных соревнованиях а также сражайся за звание главного участника космоса. Призовые банки в такой мере масштабны, что в силах померкнуть даже и абсолютно сверкающую звезду! Моментальная оплата: Лишь только вы добьешься триумфа, личные средства окажутся тут же переведены в ваш аккаунт. Абсолютно никаких промедлений – только неподдельная радость победы! Поддержка 24/7: Наш собственный экипаж непрерывно в доступе, готовый оказать помощь вам в любой ситуации. Даже коль ты заблудился между светил, мы все подскажем найти путь обратно. ?? Как начать? Просто станьте подписчиком к этот ресурс и пуститесь на полет! Затем вас ожидают бесконечные перспективы шансов и целое море наслаждения.
Не стоит упусти мгновение, как твоя огонек заблестит блестящее всех!
#kometa #casino #Премии #Выигрыш #КометаКазино #kometacasino
Зажги свою светило фарта совместно с “Комета казино”! ?
Привет, авантюрист приключений! Вы созрел отправиться на галактическое странствие, в коем любая привал обещает потрясающие призы да захватывающие эмоции?
В таком случае вам несомненно рекомендуется подключиться на нашему уникальному Telegram-каналу kometa официальный сайт регистрация . Здесь тебя поджидают совсем не лишь развлечения, а реальные космические приключения, переполненные непредвиденных поворотов а сверкающих импульсов удачи!
Отчего избирают нас?
Галактические бонусы: Присоединяйся к нашу общую группу и потом заполучи первоначальный комплект бонусов, который даст возможность вам скорее осилить своей мечты. Игровые галактики: Местные игровые автоматы – это абсолютно все космосы, наполненные загадками и ценностями. Изучай все полностью и потом найди заветный безупречный слот! Соревновательные миры: Участвуй в космических турнирах и битва за звание главного геймера космоса. Призовые банки до такой степени велики, что в силах померкнуть и абсолютно блестящую светило! Быстрая перевод: Лишь только ты добьешься победы, твои деньги будут моментально зачислены в собственный счет. Совершенно никаких задержек – только неподдельная восторг успеха! Поддержка 24/7: Наш собственный экипаж постоянно в контакте, нацеленный ассистировать тебе в какой угодно положении. Пусть даже вдруг ты заблудился между звезд, мы вам посодействуем открыть направление домой. ?? Каким путем стартовать? Легко оформляйте подписку на данный источник и риньтесь в рейс! Впереди тебя ожидают неисчерпаемые горизонты шансов а целое море восторга.
Не стоит упустить минуту, когда собственная светило заблестит светлее абсолютно всех!
#kometa #casino #Подарки #Выигрыш #CometaCasino #kometacasino
Зажги свою огонек удачи бок о бок рядом “Kometa casino”! ?
Салют, авантюрист приключений! Вы готов пуститься на галактическое странствие, в коем любая привал сулит потрясающие бонусы да ошеломляющие ощущения?
Тогда тебе безусловно рекомендуется присоединиться в нашему уникальному каналу в Telegram casino kometa телеграм . Тут вас ожидают отнюдь не только развлечения, а настоящие галактические похождения, насыщенные внезапных витков и ярких сияний везения!
Отчего предпочитают нас?
Звездные бонусы: Присоединяйся на объединенную экипаж и заполучи первоначальный комплект премий, что даст возможность вам скорее добиться своей задачи. Развлекательные миры: Местные гэмблинг слоты – представляют собой настоящие космосы, кишащие тайнами и сокровищами. Исследуй их полностью и потом открой заветный совершенный аппарат! Соревновательные миры: Участвуй в межзвездных конкурсах и битва во имя титул самого крутого геймера галактики. Наградные пулы настолько огромны, что аж могут затмить и самую что ни на есть яркую звезду! Мгновенная оплата: Едва только ты одержишь успеха, твои средства окажутся мгновенно отправлены на собственный кошелек. Никаких промедлений – лишь неподдельная восторг успеха! Ассистанс 24/7: Наш состав всегда в доступе, нацеленный помочь тебе при любой обстоятельстве. Если даже коль вы сбился с пути между светил, мы все поможем найти путь к себе. ?? Как начать? Всего лишь оформляйте подписку на этот источник а потом пуститесь в путешествие! Дальше вас ожидают бесконечные пределы шансов и даже океан удовольствия.
Не упустить минуту, когда ваша огонек засияет ярче каждого!
#kometa #casino #Подарки #Выигрыш #КометаКазино #kometacasino
Разгорись свою огонек везения вместе рядом “cometa casino”! ?
Здравствуй, путешественник приключений! Ты созрел ринуться в галактическое вояж, где-то всякая точка сулит фантастические призы и даже головокружительные эмоции?
Тогда вам безусловно следовало бы вступить в этому уникальному Telegram-каналу отзывы casino kometa . Здесь вас ожидают не только развлечения, а скорее реальные космические авантюры, насыщенные неожиданных витков а также ослепительных вспышек фортуны!
Почему выбирают именно нас?
Звездные премии: Присоединяйся в нашу группу а получи первоначальный набор плюшек, какой даст возможность тебе оперативнее осилить собственной задачи. Развлекательные планеты: Местные игровые слоты – есть абсолютно все космосы, наполненные секретами и даже богатствами. Осваивай их до единой и конечно же обнаруживай твоей идеальный автомат! Конкурсные галактики: Вливайся в космических соревнованиях а также сражайся во имя пост самого крутого участника космоса. Наградные банки в такой мере масштабны, что могут затмить и самую яркую огонек! Моментальная перевод: Едва только вы одержишь победы, ваши деньги осуществятся мгновенно переведены в собственный кошелек. Абсолютно никаких промедлений – исключительно неподдельная удовольствие триумфа! Поддержка всегда на связи: Наш экипаж постоянно на контакте, готовый ассистировать вам во всякой ситуации. Если даже вдруг ты заблудился между галактик, мы подскажем найти дорогу к себе. ?? Каким образом стартовать? Просто оформляйте подписку к данный источник и отправься в рейс! Затем вас ожидают бесконечные горизонты шансов и даже море наслаждения.
Не стоит упустить минуту, как ваша светило заблестит блестящее абсолютно всех!
#kometa #casino #Премии #Успех #KometaCasino #kometacasino
Зажги свою огонек фарта совместно рядом “Комета казино”! ?
Салют, авантюрист приключений! Ты настроен ринуться в звездное вояж, где-то каждая остановка обещает потрясающие бонусы и даже головокружительные ощущения?
Тогда тебе точно рекомендуется подключиться в этому неповторимому телеграм-каналу kometa casino скачать бесплатно . Тут вас ждут не только игры, а скорее реальные космические приключения, переполненные неожиданных кульбитов и ослепительных вспышек везения!
Зачем выбирают именно нас?
Звездные премии: Регистрируйся на нашу общую экипаж а возьми первоначальный набор плюшек, что поможет тебе скорее добиться собственной мечты. Игровые миры: Наши развлекательные машины – это абсолютно все миры, изобилующие тайнами и даже сокровищами. Изучай их все и конечно же найди свой идеальный слот! Турнирные галактики: Участвуй в космических турнирах и сражайся во имя звание главного геймера космоса. Наградные банки настолько огромны, что аж в силах померкнуть и абсолютно сверкающую звезду! Мгновенная выплата: Едва только ты одержишь триумфа, ваши средства будут тут же переведены на ваш кошелек. Абсолютно никаких промедлений – только неподдельная восторг победы! Поддержка круглосуточно: Наш экипаж непрерывно в связи, готовый помочь вам во всякой ситуации. Пусть даже коль ты сбился с пути вокруг галактик, мы подскажем открыть дорогу к себе. ?? Каким путем приступить? Всего лишь станьте подписчиком на данный источник а потом пуститесь в полет! Впереди тебя ожидают бесконечные пределы шансов а целое море восторга.
Не стоит пропусти мгновение, в тот момент когда собственная светило засияет ярче каждого!
#kometa #casino #Бонусы #Выигрыш #КометаКазино #kometacasino
Зажги твою светило фарта совместно рядом “Kometa casino”! ?
Здравствуй, искатель подвигов! Вы созрел пуститься на звездное вояж, в коем всякая привал сулит потрясающие призы да ошеломляющие эмоции?
Тогда тебе несомненно рекомендуется подключиться к нашему уникальному Telegram-каналу скачать casino kometa официальный сайт . Тут вас поджидают совсем не просто игры, но реальные космические приключения, насыщенные неожиданных поворотов а ярких импульсов удачи!
Зачем выбирают нас?
Космические бонусы: Вступай в нашу группу и потом заполучи стартовый комплект бонусов, который даст возможность вам быстрее осилить твоей задачи. Развлекательные галактики: Наши игровые слоты – есть абсолютно все миры, кишащие секретами да ценностями. Изучай все до единой и конечно же найди твоей безупречный аппарат! Турнирные вселенные: Вливайся в межзвездных конкурсах а также борись за звание главного геймера вселенной. Призовые банки настолько огромны, что могут ослепить даже и самую блестящую огонек! Моментальная оплата: Едва только вы одержишь успеха, личные средства будут мгновенно зачислены на твой счет. Абсолютно никаких задержек – лишь неподдельная удовольствие успеха! Поддержка круглосуточно: Наш собственный команда постоянно в контакте, расположенный оказать помощь вам при всякой положении. Пусть даже вдруг вы сбился с пути среди звезд, мы вам подскажем открыть путь домой. ?? Каким путем приступить? Легко подпишись на данный ресурс и затем риньтесь на путешествие! Дальше вас ждут безграничные перспективы возможностей и океан удовольствия.
Не упусти момент, как ваша звезда заблестит светлее каждого!
#kometa #casino #Премии #Успех #KometaCasino #kometacasino
Зажги свою звезду везения бок о бок с “Комета казино”! ?
Привет, путешественник подвигов! Ты настроен пуститься на звездное вояж, в коем каждая остановка сулит невероятные бонусы и даже захватывающие чувства?
В этом случае вам несомненно стоит подключиться к нашему с вами уникальному Telegram-каналу kometa casino реальные деньги в россии . Здесь тебя ожидают не лишь забавы, но подлинные галактические похождения, насыщенные неожиданных кульбитов и ослепительных вспышек фортуны!
Почему избирают именно нас?
Звездные премии: Вступай на нашу команду и возьми начальный набор плюшек, который поможет вам скорее достичь своей мечты. Игровые планеты: Наши игровые автоматы – представляют собой целые миры, кишащие тайнами и сокровищами. Исследуй каждый из них полностью и открой заветный безупречный автомат! Конкурсные миры: Участвуй в межгалактических соревнованиях и потом сражайся ради пост главного геймера галактики. Наградные фонды в такой мере велики, что аж способны затмить даже и самую что ни на есть яркую звезду! Моментальная оплата: Как только вы добьешься успеха, личные капитал окажутся моментально отправлены на ваш счет. Никаких промедлений – только чистая восторг триумфа! Помощь всегда на связи: Нашей команды команда непрерывно в контакте, расположенный ассистировать вам во какой угодно ситуации. Пусть даже коль вы заблудился среди галактик, мы вам посодействуем обнаружить направление к себе. ?? Каким путем приступить? Просто станьте подписчиком к наш источник и риньтесь в путешествие! Впереди тебя ждут бесконечные пределы вариантов и даже море удовольствия.
Не стоит пропусти момент, как ваша звезда заблестит светлее всех!
#kometa #casino #Подарки #Выигрыш #KometaCasino #kometacasino
Зажги собственную огонек удачи бок о бок со “cometa casino”! ?
Здравствуй, искатель похождений! Вы созрел пуститься на галактическое странствие, в коем всякая точка обещает потрясающие бонусы и даже ошеломляющие эмоции?
В таком случае тебе точно рекомендуется подключиться к нашему с вами уникальному телеграм-каналу kometa casino промо . Тут вас ждут отнюдь не просто развлечения, но настоящие звездные похождения, насыщенные неожиданных кульбитов а также ярких сияний удачи!
Почему предпочитают наше казино?
Космические бонусы: Регистрируйся к нашу общую команду и возьми стартовый набор бонусов, который поможет тебе скорее осилить собственной задачи. Развлекательные планеты: Местные гэмблинг машины – есть настоящие миры, изобилующие тайнами да ценностями. Исследуй все все и потом найди твоей идеальный слот! Конкурсные миры: Участвуй на межгалактических конкурсах и борись за титул самого крутого геймера галактики. Наградные пулы настолько велики, что могут в силах ослепить и самую что ни на есть блестящую огонек! Быстрая оплата: Едва только ты одержишь победы, ваши капитал осуществятся тут же переведены на ваш счет. Абсолютно никаких промедлений – лишь чистая восторг триумфа! Помощь 24/7: Наш состав постоянно в контакте, расположенный ассистировать тебе в какой угодно положении. Даже вдруг ты сбился с пути среди галактик, мы вам посодействуем обнаружить направление к себе. ?? Каким образом приступить? Легко оформляйте подписку к наш канал а потом пуститесь на полет! Впереди тебя ожидают неисчерпаемые перспективы шансов и даже целое море наслаждения.
Не стоит упустить минуту, как твоя огонек засияет ярче всех!
#kometa #casino #Подарки #Выигрыш #CometaCasino #kometacasino
Зажги свою светило фарта вместе рядом “Kometa casino”! ?
Привет, путешественник приключений! Ты настроен отправиться на космическое странствие, в коем каждая точка гарантирует потрясающие призы и даже ошеломляющие ощущения?
Тогда тебе несомненно следовало бы подключиться на этому уникальному Telegram-каналу комета казино . Тут вас ожидают не просто развлечения, а скорее реальные звездные приключения, насыщенные непредвиденных кульбитов а ослепительных вспышек везения!
Отчего предпочитают нас?
Галактические бонусы: Вступай в объединенную экипаж а возьми стартовый набор бонусов, какой содействует тебе быстрее осилить твоей мечты. Игровые миры: Здешние развлекательные машины – есть абсолютно все миры, наполненные тайнами и даже ценностями. Изучай их все и обнаруживай свой безупречный автомат! Соревновательные вселенные: Принимай участие на межгалактических турнирах а также борись за титул самого крутого геймера вселенной. Призовые пулы настолько огромны, что в силах померкнуть даже и самую блестящую звезду! Моментальная перевод: Лишь только ты добьешься победы, твои деньги окажутся моментально зачислены на ваш кошелек. Абсолютно никаких промедлений – исключительно неподдельная радость победы! Помощь 24/7: Наш команда всегда в доступе, готовый оказать помощь вам во всякой ситуации. Даже коль ты потерялся вокруг галактик, мы подскажем найти путь домой. ?? Каким образом стартовать? Легко подпишись на наш ресурс и затем риньтесь на полет! Дальше вас ожидают безграничные пределы шансов и даже целое море восторга.
Не нужно упусти минуту, в тот момент когда ваша звезда засияет светлее каждого!
#kometa #casino #Бонусы #Выигрыш #KometaCasino #kometacasino
Разгорись собственную звезду везения вместе с “Комета казино”! ?
Салют, авантюрист подвигов! Вы созрел отправиться на космическое путешествие, где любая остановка обещает невероятные бонусы и даже ошеломляющие эмоции?
В этом случае тебе точно стоит подключиться к нашему с вами эксклюзивному каналу в Telegram kometa casino скачать приложение . В этом месте вас ожидают совсем не лишь игры, но подлинные галактические приключения, полные непредвиденных кульбитов а также ослепительных импульсов везения!
Отчего выбирают наше казино?
Звездные премии: Присоединяйся на нашу общую команду и потом заполучи начальный комплект премий, который поможет вам быстрее осилить своей задачи. Геймерские миры: Здешние игровые машины – есть абсолютно все вселенные, изобилующие загадками да богатствами. Исследуй каждый из них полностью и обнаруживай твоей безупречный аппарат! Турнирные вселенные: Вливайся на космических конкурсах а также битва ради титул лучшего геймера галактики. Наградные фонды настолько масштабны, что могут в силах затмить даже самую что ни на есть сверкающую светило! Мгновенная перевод: Лишь только вы одержишь успеха, ваши средства осуществятся тут же отправлены в ваш кошелек. Совершенно никаких задержек – только подлинная удовольствие успеха! Помощь всегда на связи: Наш состав постоянно на доступе, расположенный оказать помощь вам в какой угодно положении. Даже коль ты потерялся между светил, мы поможем обнаружить направление к себе. ?? Каким образом начать? Всего лишь оформляйте подписку на этот источник а потом риньтесь в полет! Затем тебя ждут безграничные перспективы вариантов и даже океан наслаждения.
Не нужно упустить момент, в тот момент когда твоя звезда засветится светлее абсолютно всех!
#kometa #casino #Подарки #Победа #CometaCasino #kometacasino
Разгорись твою огонек удачи бок о бок с “Kometa casino”! ?
Здравствуй, путешественник приключений! Ты созрел ринуться в галактическое путешествие, где-то любая остановка обещает невероятные награды да головокружительные чувства?
В таком случае вам несомненно стоит подключиться в этому неповторимому Telegram-каналу комета казино . Здесь вас поджидают не просто забавы, а скорее подлинные галактические приключения, насыщенные непредвиденных поворотов а сверкающих вспышек удачи!
Почему выбирают именно нас?
Космические бонусы: Присоединяйся в нашу группу а получи стартовый комплект плюшек, что содействует вам быстрее добиться своей мечты. Геймерские галактики: Здешние игровые машины – есть целые вселенные, изобилующие секретами и богатствами. Изучай каждый из них полностью и конечно же открой заветный идеальный слот! Соревновательные вселенные: Вливайся в межгалактических конкурсах а также борись во имя титул самого крутого игрока вселенной. Наградные пулы в такой мере масштабны, что могут ослепить даже самую яркую звезду! Мгновенная перевод: Едва только ты одержишь триумфа, твои деньги осуществятся тут же переведены в твой аккаунт. Никаких проволочек – лишь неподдельная восторг триумфа! Ассистанс круглосуточно: Наш собственный команда непрерывно на контакте, нацеленный ассистировать вам во любой положении. Если даже вдруг ты потерялся среди галактик, мы все посодействуем найти направление к себе. ?? Каким путем стартовать? Просто оформляйте подписку на этот канал и пуститесь в полет! Впереди тебя ожидают бесконечные пределы шансов и целое море восторга.
Не пропусти минуту, в тот момент когда собственная огонек засияет ярче абсолютно всех!
#kometa #casino #Премии #Успех #CometaCasino #kometacasino
Зажги твою звезду фарта совместно рядом “Kometa casino”! ?
Салют, путешественник подвигов! Вы созрел пуститься на галактическое путешествие, где любая остановка гарантирует потрясающие бонусы и даже ошеломляющие чувства?
В таком случае тебе точно следовало бы подключиться к нашему с вами неповторимому каналу в Telegram kometa casino отзывы . Тут тебя ждут не только забавы, а подлинные галактические приключения, насыщенные неожиданных кульбитов а также ярких импульсов фортуны!
Почему предпочитают именно нас?
Галактические премии: Присоединяйся в нашу команду а заполучи начальный комплект плюшек, какой даст возможность тебе оперативнее достичь своей цели. Геймерские галактики: Местные игровые машины – есть абсолютно все космосы, наполненные тайнами и даже богатствами. Осваивай каждый из них полностью и конечно же найди заветный идеальный автомат! Турнирные галактики: Принимай участие на космических соревнованиях а также сражайся ради титул лучшего участника космоса. Призовые банки в такой мере огромны, что способны померкнуть даже абсолютно блестящую огонек! Быстрая оплата: Лишь только вы одержишь триумфа, личные капитал окажутся мгновенно отправлены на собственный счет. Абсолютно никаких проволочек – только чистая удовольствие успеха! Помощь 24/7: Нашей команды экипаж непрерывно на связи, расположенный оказать помощь вам во всякой положении. Если даже если ты сбился с пути среди звезд, мы вам посодействуем найти направление домой. ?? Каким образом стартовать? Всего лишь подпишись на этот канал и пуститесь в рейс! Впереди тебя подстерегают бесконечные пределы возможностей и океан наслаждения.
Не пропусти мгновение, когда твоя звезда засветится ярче абсолютно всех!
#kometa #casino #Подарки #Победа #KometaCasino #kometacasino
Запали собственную звезду везения совместно с “Комета казино”! ?
Салют, искатель похождений! Ты готов ринуться на космическое странствие, где-то всякая точка сулит потрясающие награды да захватывающие эмоции?
В этом случае тебе точно рекомендуется вступить на нашему с вами эксклюзивному Telegram-каналу kometa casino официальный сайт . Здесь вас ожидают совсем не только забавы, но настоящие звездные приключения, переполненные неожиданных поворотов и сверкающих сияний фортуны!
Почему избирают именно нас?
Звездные бонусы: Вступай к объединенную команду а заполучи первоначальный пакет бонусов, который содействует тебе скорее достичь своей цели. Игровые галактики: Наши гэмблинг слоты – есть абсолютно все космосы, наполненные тайнами и даже богатствами. Осваивай их до единой и открой твоей идеальный аппарат! Соревновательные миры: Принимай участие на межгалактических конкурсах и потом борись во имя звание лучшего геймера космоса. Выигрышные фонды до такой степени велики, что могут в силах померкнуть и самую что ни на есть сверкающую огонек! Моментальная оплата: Едва только ты одержишь триумфа, ваши капитал осуществятся моментально зачислены в твой счет. Никаких задержек – лишь чистая удовольствие триумфа! Помощь всегда на связи: Нашей команды экипаж непрерывно на доступе, расположенный ассистировать тебе в какой угодно положении. Если даже вдруг ты потерялся среди звезд, мы вам подскажем найти дорогу к себе. ?? Каким путем стартовать? Просто оформляйте подписку на наш источник и пуститесь в путешествие! Впереди вас ждут безграничные горизонты возможностей и даже море наслаждения.
Не упустить минуту, когда ваша светило засияет светлее всех!
#kometa #casino #Подарки #Успех #CometaCasino #kometacasino
Зажги твою огонек удачи вместе рядом “Kometa casino”! ?
Здравствуй, путешественник приключений! Ты настроен отправиться в галактическое путешествие, где любая привал сулит фантастические награды и захватывающие ощущения?
В таком случае тебе точно рекомендуется вступить к нашему неповторимому Telegram-каналу kometa онлайн casino вход . Здесь тебя ожидают отнюдь не просто забавы, а подлинные галактические похождения, насыщенные неожиданных поворотов а ярких сияний везения!
Почему выбирают именно нас?
Звездные бонусы: Регистрируйся в нашу общую группу и заполучи первоначальный пакет бонусов, какой поможет тебе скорее добиться твоей задачи. Развлекательные миры: Здешние развлекательные машины – это абсолютно все вселенные, изобилующие загадками да ценностями. Осваивай их до единой и конечно же обнаруживай заветный совершенный аппарат! Соревновательные миры: Принимай участие на межгалактических конкурсах и потом сражайся за пост главного геймера вселенной. Призовые банки в такой мере огромны, что аж в силах затмить даже и самую что ни на есть сверкающую звезду! Мгновенная перевод: Едва только вы достигнешь победы, личные средства осуществятся тут же переведены на собственный кошелек. Абсолютно никаких промедлений – исключительно чистая радость победы! Ассистанс 24/7: Нашей команды состав непрерывно в контакте, расположенный ассистировать тебе в какой угодно обстоятельстве. Даже вдруг вы сбился с пути вокруг светил, мы вам посодействуем найти путь к себе. ?? Каким путем стартовать? Просто оформляйте подписку на этот канал а потом риньтесь в рейс! Впереди вас ждут безграничные горизонты возможностей и даже целое море удовольствия.
Не нужно упусти мгновение, как ваша светило засветится светлее абсолютно всех!
#kometa #casino #Подарки #Выигрыш #КометаКазино #kometacasino
Запали собственную светило везения совместно рядом “Комета казино”! ?
Здравствуй, путешественник похождений! Вы созрел отправиться в звездное странствие, где каждая остановка сулит фантастические награды и даже ошеломляющие эмоции?
В этом случае вам несомненно следовало бы вступить к этому уникальному Telegram-каналу скачать kometa casino . Тут вас ожидают не лишь игры, а скорее настоящие звездные приключения, переполненные внезапных витков а также ярких импульсов фортуны!
Отчего выбирают наше казино?
Космические поощрения: Регистрируйся к нашу команду а получи начальный комплект плюшек, который даст возможность тебе быстрее достичь твоей задачи. Игровые планеты: Местные развлекательные машины – это настоящие вселенные, наполненные секретами и даже ценностями. Изучай каждый из них до единой и открой заветный идеальный аппарат! Конкурсные миры: Вливайся на межгалактических конкурсах а также сражайся во имя звание самого крутого геймера вселенной. Призовые банки до такой степени масштабны, что аж в силах ослепить даже и самую сверкающую светило! Быстрая перевод: Лишь только ты достигнешь триумфа, твои средства будут тут же переведены в ваш кошелек. Никаких задержек – только подлинная радость триумфа! Поддержка круглосуточно: Наш собственный команда всегда в контакте, готовый ассистировать вам во всякой ситуации. Если даже коль ты потерялся между светил, мы все подскажем обнаружить направление к себе. ?? Как стартовать? Всего лишь подпишись на данный канал и затем отправься на путешествие! Впереди тебя подстерегают неисчерпаемые пределы возможностей и даже море удовольствия.
Не упусти момент, в тот момент когда собственная огонек заблестит светлее абсолютно всех!
#kometa #casino #Премии #Победа #KometaCasino #kometacasino
Зажги свою светило фарта совместно рядом “Комета казино”! ?
Здравствуй, авантюрист подвигов! Ты созрел отправиться в космическое странствие, где любая точка сулит фантастические призы и даже захватывающие чувства?
В этом случае тебе точно стоит подключиться к нашему эксклюзивному Telegram-каналу kometa casino официальный сайт . В этом месте вас ожидают отнюдь не лишь игры, а подлинные космические похождения, переполненные неожиданных кульбитов и ослепительных сияний везения!
Зачем предпочитают нас?
Галактические премии: Регистрируйся на нашу общую экипаж а получи первоначальный набор плюшек, который содействует вам оперативнее достичь собственной задачи. Геймерские галактики: Здешние игровые автоматы – это абсолютно все вселенные, изобилующие загадками и богатствами. Исследуй все полностью и потом открой твоей безупречный автомат! Конкурсные миры: Участвуй в космических конкурсах и потом сражайся за звание лучшего игрока галактики. Выигрышные фонды в такой мере масштабны, что в силах померкнуть даже и абсолютно сверкающую звезду! Моментальная перевод: Лишь только ты добьешься триумфа, твои капитал осуществятся мгновенно зачислены на твой аккаунт. Никаких промедлений – исключительно чистая восторг успеха! Помощь круглосуточно: Наш экипаж постоянно в доступе, нацеленный оказать помощь тебе в любой ситуации. Если даже вдруг вы заблудился вокруг галактик, мы подскажем найти дорогу обратно. ?? Каким путем стартовать? Просто оформляйте подписку к наш канал а потом отправься в путешествие! Затем тебя подстерегают неисчерпаемые пределы возможностей и океан наслаждения.
Не упусти минуту, когда собственная светило заблестит блестящее абсолютно всех!
#kometa #casino #Бонусы #Победа #KometaCasino #kometacasino
Разгорись свою огонек фарта бок о бок рядом “cometa casino”! ?
Привет, путешественник приключений! Ты созрел отправиться на галактическое вояж, где любая точка сулит невероятные награды и даже ошеломляющие ощущения?
Тогда тебе точно рекомендуется вступить на этому неповторимому Telegram-каналу kometa casino отзывы реальные . В этом месте вас ждут не просто развлечения, но реальные галактические авантюры, насыщенные внезапных поворотов и ярких вспышек удачи!
Зачем предпочитают наше казино?
Галактические премии: Присоединяйся на нашу экипаж и получи первоначальный набор бонусов, какой поможет тебе скорее достичь своей задачи. Геймерские планеты: Здешние развлекательные машины – это абсолютно все космосы, кишащие загадками и ценностями. Осваивай все до единой и конечно же найди заветный безупречный слот! Конкурсные миры: Вливайся в межзвездных турнирах и потом сражайся во имя пост лучшего участника космоса. Выигрышные банки в такой мере огромны, что могут способны затмить и самую яркую огонек! Моментальная выплата: Как только вы одержишь триумфа, твои средства осуществятся моментально зачислены на ваш счет. Абсолютно никаких проволочек – исключительно неподдельная удовольствие триумфа! Поддержка всегда на связи: Наш экипаж всегда в доступе, расположенный помочь тебе при любой положении. Даже вдруг ты потерялся вокруг звезд, мы вам подскажем найти направление домой. ?? Как начать? Легко подпишись на данный ресурс и отправься на путешествие! Затем тебя подстерегают неисчерпаемые пределы возможностей и океан удовольствия.
Не стоит пропусти мгновение, как твоя светило засветится ярче абсолютно всех!
#kometa #casino #Премии #Победа #КометаКазино #kometacasino
Зажги твою огонек фарта вместе со “cometa casino”! ?
Салют, путешественник подвигов! Ты созрел ринуться на звездное путешествие, где-то любая точка сулит потрясающие награды и даже захватывающие ощущения?
В этом случае вам точно стоит присоединиться в этому уникальному каналу в Telegram kometa casino зеркало . Здесь вас поджидают совсем не просто игры, а скорее подлинные галактические авантюры, полные непредвиденных кульбитов а ярких импульсов удачи!
Почему избирают наше казино?
Звездные бонусы: Регистрируйся к нашу общую команду и потом получи начальный пакет бонусов, какой поможет тебе оперативнее добиться своей задачи. Развлекательные галактики: Местные гэмблинг слоты – представляют собой настоящие миры, изобилующие тайнами и сокровищами. Осваивай все полностью и потом обнаруживай твоей безупречный автомат! Соревновательные миры: Принимай участие в межзвездных конкурсах и потом сражайся ради пост лучшего участника галактики. Призовые фонды до такой степени велики, что могут в силах померкнуть даже и самую что ни на есть блестящую огонек! Моментальная перевод: Едва только вы достигнешь успеха, личные деньги осуществятся мгновенно переведены в ваш счет. Совершенно никаких задержек – исключительно чистая радость триумфа! Поддержка всегда на связи: Наш команда постоянно на доступе, расположенный помочь вам в какой угодно положении. Даже коль вы потерялся вокруг светил, мы все посодействуем обнаружить дорогу обратно. ?? Каким путем стартовать? Всего лишь подпишись на данный источник а потом пуститесь в рейс! Затем вас ждут неисчерпаемые перспективы возможностей и даже море восторга.
Не упусти минуту, в тот момент когда твоя звезда заблестит светлее абсолютно всех!
#kometa #casino #Бонусы #Выигрыш #CometaCasino #kometacasino
Разгорись собственную светило фарта совместно с “Kometa casino”! ?
Здравствуй, авантюрист подвигов! Вы созрел ринуться в звездное странствие, где каждая остановка сулит потрясающие призы и даже захватывающие чувства?
Тогда вам точно стоит присоединиться в нашему с вами неповторимому Telegram-каналу играть в casino kometa . Тут вас ждут совсем не просто забавы, но настоящие звездные приключения, полные внезапных поворотов и сверкающих сияний фортуны!
Отчего предпочитают наше казино?
Галактические бонусы: Вступай к объединенную команду и возьми начальный комплект плюшек, который поможет вам оперативнее достичь твоей цели. Развлекательные планеты: Местные игровые машины – есть целые вселенные, изобилующие секретами и даже сокровищами. Изучай все все и потом открой свой идеальный слот! Соревновательные миры: Вливайся в космических конкурсах а также борись ради пост лучшего участника вселенной. Призовые пулы в такой мере велики, что могут в силах померкнуть даже и самую что ни на есть сверкающую звезду! Мгновенная оплата: Едва только вы достигнешь триумфа, ваши капитал окажутся моментально переведены на твой счет. Совершенно никаких промедлений – лишь неподдельная восторг триумфа! Помощь круглосуточно: Наш собственный команда непрерывно в доступе, готовый оказать помощь вам при какой угодно обстоятельстве. Даже коль ты заблудился среди звезд, мы все посодействуем обнаружить дорогу домой. ?? Как приступить? Легко подпишись к этот ресурс а потом отправься в рейс! Впереди тебя ждут бесконечные перспективы шансов и океан восторга.
Не упустить мгновение, когда твоя огонек засияет светлее абсолютно всех!
#kometa #casino #Бонусы #Победа #КометаКазино #kometacasino
индивидуалки луганск : Сексуальные девушки
В Луганске можно найти множество девушек по вызову, оказывающих интимные сервисы. Эти сексуальные женщины отличаются разнообразием внешнего вида и индивидуальных характеристик, что дает возможность выбрать партнера на любой предпочтение. Они готовы сопровождать на событиях, предоставляя не только телесное наслаждение, но и увлекательное общение.
Каждая из них гарантирует высокий уровень конфиденциальности и профессионализма, создавая атмосферу доверия и комфорта. Девушки по вызову Луганска предлагают сервисы, подстроенные под пожелания клиентов, что делает встречу незабываемой и приятной.
Запали твою огонек фарта совместно с “Kometa casino”! ?
Привет, путешественник похождений! Ты настроен ринуться на галактическое странствие, в коем любая остановка сулит потрясающие призы и головокружительные ощущения?
В таком случае вам точно стоит присоединиться к нашему эксклюзивному телеграм-каналу kometa casino играть . Здесь тебя ожидают совсем не просто игры, а скорее настоящие галактические похождения, насыщенные непредвиденных поворотов и ослепительных импульсов везения!
Отчего выбирают наше казино?
Космические бонусы: Регистрируйся в нашу общую группу и потом получи первоначальный пакет плюшек, который даст возможность тебе скорее осилить своей цели. Развлекательные галактики: Местные игровые слоты – есть абсолютно все вселенные, кишащие тайнами да ценностями. Изучай все до единой и конечно же найди заветный идеальный аппарат! Турнирные миры: Принимай участие в межгалактических турнирах и борись во имя звание лучшего участника космоса. Выигрышные пулы до такой степени огромны, что могут померкнуть даже абсолютно блестящую огонек! Мгновенная перевод: Лишь только вы добьешься победы, ваши деньги окажутся мгновенно переведены на собственный кошелек. Абсолютно никаких задержек – исключительно чистая радость успеха! Помощь круглосуточно: Наш состав всегда в контакте, расположенный ассистировать вам во всякой ситуации. Пусть даже вдруг ты заблудился между светил, мы вам подскажем обнаружить дорогу домой. ?? Каким образом начать? Просто станьте подписчиком на данный источник и риньтесь в полет! Впереди вас подстерегают бесконечные пределы вариантов и океан наслаждения.
Не стоит пропусти момент, как твоя звезда засветится светлее каждого!
#kometa #casino #Подарки #Выигрыш #CometaCasino #kometacasino
проститутки города луганск : Привлекательные женщины
В Луганске можно найти большое количество девушек по вызову, предлагающих сексуальные услуги. Эти привлекательные девушки отличаются многообразием внешности и личностных характеристик, что дает возможность подобрать партнера на любой вкус. Они рады быть рядом на мероприятиях, предоставляя не только телесное удовольствие, но и интересное общение.
Каждая из них гарантирует высокий уровень конфиденциальности и профессионализма, создавая атмосферу доверия и комфорта. Девушки по вызову города Луганск предлагают сервисы, подстроенные под желания клиентов, что делает встречу незабываемой и приятной.
Разгорись свою огонек фарта бок о бок со “Комета казино”! ?
Привет, путешественник подвигов! Ты настроен отправиться на звездное путешествие, в коем любая привал сулит невероятные награды да ошеломляющие ощущения?
В этом случае вам несомненно рекомендуется вступить к нашему эксклюзивному Telegram-каналу kometa casino играйте на зеркалах . Тут вас поджидают совсем не только развлечения, но подлинные космические приключения, переполненные непредвиденных витков и сверкающих вспышек везения!
Зачем выбирают наше казино?
Галактические поощрения: Регистрируйся на нашу команду и потом получи первоначальный комплект премий, который поможет вам скорее осилить собственной цели. Геймерские планеты: Наши развлекательные слоты – есть целые миры, кишащие секретами да богатствами. Изучай каждый из них все и найди твоей идеальный аппарат! Соревновательные миры: Участвуй в космических турнирах а также битва за звание лучшего участника космоса. Призовые банки до такой степени велики, что могут могут затмить даже и самую блестящую светило! Быстрая оплата: Едва только вы добьешься триумфа, твои средства осуществятся моментально отправлены на ваш кошелек. Абсолютно никаких промедлений – исключительно подлинная удовольствие победы! Ассистанс всегда на связи: Наш собственный команда постоянно на доступе, готовый оказать помощь тебе во какой угодно ситуации. Пусть даже коль ты заблудился вокруг галактик, мы все посодействуем открыть дорогу домой. ?? Как приступить? Просто подпишись на данный источник и затем пуститесь в рейс! Затем вас подстерегают безграничные перспективы шансов и даже море удовольствия.
Не пропусти момент, в тот момент когда ваша светило заблестит ярче каждого!
#kometa #casino #Подарки #Выигрыш #CometaCasino #kometacasino
проститутки индивидуалки донецк : Привлекательные женщины
В городе Луганск можно обнаружить множество индивидуалок, оказывающих интимные услуги. Эти сексуальные женщины отличаются разнообразием внешности и личностных качеств, что дает возможность выбрать партнера на любой предпочтение. Они готовы быть рядом на событиях, предоставляя не только телесное удовольствие, но и увлекательное общение.
Каждая из них обеспечивает высокий уровень конфиденциальности и профессионализма, создавая атмосферу доверия и комфорта. Индивидуалки города Луганск предлагают сервисы, подстроенные под желания клиентов, что делает встречу незабываемой и приятной.
Запали собственную огонек везения совместно со “Комета казино”! ?
Привет, путешественник подвигов! Ты созрел отправиться в галактическое странствие, где каждая остановка обещает фантастические награды и головокружительные ощущения?
В этом случае тебе точно следовало бы присоединиться на этому уникальному телеграм-каналу kometa casino официальный сайт вход . Тут вас поджидают совсем не просто забавы, а настоящие космические похождения, полные непредвиденных поворотов а ярких импульсов удачи!
Почему выбирают именно нас?
Космические бонусы: Вступай в нашу команду и заполучи начальный набор премий, какой содействует вам оперативнее достичь твоей задачи. Развлекательные планеты: Здешние игровые автоматы – это настоящие космосы, кишащие секретами и сокровищами. Изучай их полностью и обнаруживай заветный совершенный автомат! Турнирные миры: Принимай участие в межзвездных конкурсах а также борись за звание главного игрока галактики. Наградные банки до такой степени огромны, что могут в силах померкнуть и абсолютно блестящую светило! Моментальная выплата: Лишь только ты добьешься победы, ваши средства окажутся тут же зачислены в ваш счет. Никаких задержек – лишь подлинная восторг победы! Помощь всегда на связи: Наш собственный экипаж постоянно на доступе, нацеленный помочь тебе в любой ситуации. Пусть даже коль вы потерялся вокруг галактик, мы подскажем открыть дорогу к себе. ?? Как начать? Легко станьте подписчиком к этот канал а потом риньтесь на полет! Впереди тебя ждут бесконечные перспективы шансов а океан удовольствия.
Не упусти мгновение, когда твоя светило заблестит светлее каждого!
#kometa #casino #Премии #Успех #KometaCasino #kometacasino
Зажги твою светило фарта бок о бок с “Kometa casino”! ?
Привет, искатель подвигов! Вы созрел отправиться в галактическое путешествие, где-то любая остановка гарантирует фантастические призы и ошеломляющие чувства?
В этом случае тебе несомненно следовало бы вступить на нашему с вами неповторимому телеграм-каналу отзывы о casino kometa . Тут тебя ожидают совсем не просто игры, а реальные космические авантюры, насыщенные внезапных кульбитов и сверкающих вспышек удачи!
Отчего выбирают именно нас?
Космические бонусы: Присоединяйся на объединенную экипаж и получи начальный набор бонусов, какой поможет вам оперативнее добиться своей задачи. Игровые галактики: Наши развлекательные машины – есть целые вселенные, изобилующие загадками да богатствами. Осваивай все все и конечно же открой свой совершенный слот! Турнирные вселенные: Участвуй в космических соревнованиях и потом битва ради звание главного участника галактики. Призовые фонды настолько огромны, что могут померкнуть даже абсолютно сверкающую звезду! Моментальная выплата: Едва только вы одержишь успеха, личные средства окажутся моментально зачислены на ваш кошелек. Совершенно никаких промедлений – исключительно чистая радость успеха! Поддержка 24/7: Нашей команды команда непрерывно в доступе, нацеленный оказать помощь вам при любой обстоятельстве. Если даже если ты заблудился среди звезд, мы все поможем открыть направление к себе. ?? Как начать? Просто подпишись на наш ресурс а потом риньтесь в рейс! Дальше вас подстерегают неисчерпаемые горизонты шансов и даже океан удовольствия.
Не нужно упусти момент, как собственная светило заблестит ярче абсолютно всех!
#kometa #casino #Подарки #Выигрыш #KometaCasino #kometacasino
Зажги свою звезду везения бок о бок со “Комета казино”! ?
Здравствуй, путешественник подвигов! Вы готов пуститься в космическое вояж, где-то всякая привал обещает невероятные награды да головокружительные чувства?
Тогда вам безусловно стоит подключиться в нашему с вами уникальному каналу в Telegram промокоды kometa casino . В этом месте вас ожидают не лишь игры, но подлинные звездные приключения, насыщенные непредвиденных витков а ярких вспышек везения!
Зачем избирают наше казино?
Звездные бонусы: Вступай на нашу общую экипаж и получи первоначальный набор премий, что даст возможность тебе оперативнее добиться твоей задачи. Развлекательные галактики: Наши игровые автоматы – это целые космосы, наполненные загадками и богатствами. Исследуй все все и потом обнаруживай свой совершенный слот! Турнирные галактики: Принимай участие на межзвездных соревнованиях и потом сражайся за пост лучшего участника вселенной. Призовые фонды до такой степени масштабны, что способны затмить и самую что ни на есть сверкающую огонек! Быстрая выплата: Лишь только ты достигнешь триумфа, твои капитал осуществятся моментально отправлены на ваш счет. Абсолютно никаких задержек – исключительно чистая удовольствие победы! Помощь круглосуточно: Наш экипаж постоянно на доступе, готовый ассистировать вам в какой угодно ситуации. Пусть даже вдруг вы сбился с пути вокруг звезд, мы поможем обнаружить направление обратно. ?? Как стартовать? Легко оформляйте подписку на этот ресурс и риньтесь на рейс! Затем тебя ждут безграничные перспективы возможностей и океан наслаждения.
Не стоит упустить момент, в тот момент когда твоя светило засияет блестящее всех!
#kometa #casino #Подарки #Победа #КометаКазино #kometacasino
проститутки города луганск : Сексуальные женщины
В городе Луганск можно обнаружить большое количество девушек по вызову, оказывающих сексуальные услуги. Эти сексуальные женщины отличаются многообразием внешности и индивидуальных качеств, что дает возможность выбрать партнера на любой предпочтение. Они готовы быть рядом на мероприятиях, предоставляя не только физическое наслаждение, но и интересное общение.
Каждая из них обеспечивает высокий уровень конфиденциальности и профессионализма, создавая атмосферу доверия и комфорта. Индивидуалки Луганска предлагают услуги, адаптированные под желания клиентов, что делает встречу незабываемой и приятной.
проститутки индивидуалки донецк : Сексуальные девушки
В Луганске можно обнаружить большое количество девушек по вызову, предлагающих интимные сервисы. Эти сексуальные девушки выделяются многообразием внешности и личностных характеристик, что дает возможность подобрать партнера на любой вкус. Они готовы сопровождать на событиях, предоставляя не только физическое наслаждение, но и увлекательное общение.
Каждая из них обеспечивает высокий уровень конфиденциальности и профессионализма, создавая атмосферу доверия и комфорта. Девушки по вызову Луганска оказывают сервисы, подстроенные под пожелания клиентов, что делает встречу незабываемой и приятной.
Шлюхи донецк : Сексуальные девушки
В Луганске можно обнаружить большое количество индивидуалок, предлагающих сексуальные услуги. Эти сексуальные женщины отличаются многообразием внешнего вида и индивидуальных качеств, что дает возможность подобрать компанию на любой предпочтение. Они рады быть рядом на событиях, предоставляя не только физическое наслаждение, но и интересное общение.
Каждая из них обеспечивает высокий уровень конфиденциальности и профессионализма, создавая атмосферу доверия и комфорта. Индивидуалки Луганска предлагают услуги, адаптированные под желания клиентов, что делает встречу незабываемой и приятной.
slotozal kazino
сайт
слотозал казино
slotozal casino
Обратите внимание, игроки!
Желаете попасть в окружение виртуальных-развлечений и выиграть реальные финансы? Тогда вам сюда! Топ лучших превосходных казино 2025
Наш telegram-аккаунт — ваш проводник в пространство отличных интернет-игр в РФ! Мы подобрали топ-10 безопасных игровых площадок, где вы сможете проводить время на деньги и вывести свои доходы без затруднений.
Что вас ожидает:
Достоверные рекомендации и оценки казино игровые автоматы играть бесплатно онлайн без регистрации от реальных геймеров. Хорошая репутация любого ресурса подтверждена временем и игроками. Безоплатная запись и быстрый вход на каждой ресурсах. Активные копии для посещения к вашему предпочитаемому казино в любой момент. Мобильная приложение для легкой игры так желаете.
Почему нас?
Безопасные и надежные казино с лучшими критериями для игры. Гарантия ваших данных и транзакций защищена. Новые обновления и обновления пространства онлайн-игр в России.
Слушайте, игроки!
Желаете окунуться в пространство виртуальных-игр и заработать настоящие деньги? Тогда вам тут! Топ наилучших отличных игровых сайтов 2025
Наш телеграм-канал — ваш путеводитель в мир лучших интернет-развлечений в Российской Федерации! Мы подготовили рейтинг-10 проверенных казино, где вы сможете проводить время на деньги и забрать свои выигрыши без затруднений.
Что вас поджидает:
Достоверные отзывы и оценки Самые популярные казино рейтинг 2025 года от настоящих игроков. Превосходная репутация каждого ресурса проверена опытом и пользователями. Бесплатная регистрация и скорый вход на любой сайтах. Рабочие дубликаты для доступа к вашему излюбленному игровому сайту в любой момент. Мобильное приложение для комфортной проведения времени где угодно.
Почему нас?
Надежные и надежные игровые сайты с превосходными условиями для игры. Защита ваших сведений и переводов гарантирована. Актуальные обновления и новости мира виртуальных-игр в Российской Федерации.
Обратите внимание, гейы!
Хотите попасть в пространство интернет-развлечений и получить действительные средства? Тогда вам тут! Список наилучших превосходных игровых площадок 2025
Наш тг-профиль — ваш гид в пространство лучших виртуальных-казино в России! Мы подготовили ТОП-10 безопасных казино, где вы сумеете развлекаться на средства и забрать свои выигрыши без затруднений.
Что вас ждет:
Достоверные рекомендации и оценки Программы лояльности в казино какие самые выгодные от реальных участников. Превосходная статус каждого сайта проверена годами и игроками. Безоплатная вход и быстрый вход на всех площадках. Рабочие зеркала для доступа к вашему любимому игровому сайту в любой время. Мобильная софт для комфортной развлечения там угодно.
Почему мы?
Надежные и надежные игровые площадки с лучшими условиями для проведения времени. Защита ваших данных и переводов обеспечена. Новые обновления и новости мира онлайн-казино в РФ.
Обратите внимание, гейы!
Желаете погрузиться в мир онлайн-казино и заработать реальные финансы? Тогда вам сюда! Топ лучших превосходных казино 2025
Наш тг-профиль — ваш путеводитель в пространство отличных интернет-игр в РФ! Мы подготовили рейтинг-10 безопасных игровых площадок, где вы сумеете развлекаться на средства и вывести свои доходы без проблем.
Что вас ждет:
Правдивые мнения и оценки самое крупное онлайн казино от настоящих участников. Отличная имя всякого сайта подтверждена опытом и игроками. Бесплатная запись и быстрый доступ на любой сайтах. Активные зеркала для доступа к вашему излюбленному казино в любой время. Мобильная программа для удобной развлечения где хотите.
Почему нам?
Безопасные и надежные игровые площадки с превосходными условиями для игры. Защита ваших данных и транзакций защищена. Свежие обновления и обновления окружения интернет-казино в РФ.
Слушайте, гейы!
Готовы погрузиться в пространство онлайн-игр и получить настоящие деньги? Тогда вам сюда! Топ наилучших лучших игровых сайтов 2025
Наш тг-канал — ваш проводник в окружение превосходных виртуальных-игр в России! Мы подготовили ТОП-10 проверенных казино, где вы сумеете играть на финансы и забрать свои доходы без затруднений.
Что вас поджидает:
Достоверные мнения и рейтинги казино на деньги с выводом денег от действительных геймеров. Хорошая статус каждого сайта гарантирована временем и клиентами. Безоплатная запись и быстрый вход на каждой площадках. Действующие дубликаты для посещения к вашему предпочитаемому игровой площадке в любой время. Мобильный приложение для легкой проведения времени так хотите.
Почему мы?
Надежные и гарантированные игровые сайты с превосходными параметрами для проведения времени. Гарантия ваших сведений и операций гарантирована. Свежие новости и новости окружения виртуальных-казино в России.
Обратите внимание, участники!
Хотите окунуться в мир онлайн-казино и выиграть настоящие финансы? Тогда вам сюда! Топ наилучших превосходных игровых площадок 2025
Наш telegram-профиль — ваш проводник в мир лучших виртуальных-игр в Российской Федерации! Мы подобрали рейтинг-10 безопасных казино, где вы сможете проводить время на финансы и получить свои призы без трудностей.
Что вас ожидает:
Честные отзывы и рейтинги Реальные отзывы игроков рейтинг честных казино от настоящих участников. Превосходная репутация всякого площадки проверена годами и пользователями. Бесплатная запись и мгновенный вход на каждой сайтах. Действующие копии для входа к вашему любимому казино в любое момент. Мобильная приложение для удобной проведения времени где хотите.
Почему нам?
Проверенные и проверенные игровые сайты с лучшими критериями для развлечения. Гарантия ваших информации и операций защищена. Актуальные новости и события мира интернет-развлечений в Российской Федерации.
Внимание, гейы!
Желаете погрузиться в окружение онлайн-развлечений и получить реальные средства? Тогда вам тут! Рейтинг наилучших отличных казино 2025
Наш telegram-аккаунт — ваш путеводитель в окружение отличных интернет-развлечений в России! Мы собрали рейтинг-10 проверенных игровых сайтов, где вы сможете развлекаться на средства и забрать свои призы без проблем.
Что вас ожидает:
Правдивые рекомендации и ранги Быстрые выплаты и поддержка рейтинг казино от настоящих геймеров. Превосходная имя всякого ресурса гарантирована опытом и игроками. Неоплачиваемая запись и мгновенный вход на каждой сайтах. Действующие дубликаты для доступа к вашему предпочитаемому игровой площадке в каждый час. Мобильная приложение для легкой проведения времени где угодно.
Почему нас?
Надежные и гарантированные игровые площадки с отличными условиями для развлечения. Безопасность ваших данных и операций гарантирована. Свежие новости и новости окружения онлайн-игр в Российской Федерации.
Обратите внимание, гейы!
Желаете погрузиться в окружение онлайн-казино и заработать реальные средства? Тогда вам к нам! Список самых отличных игровых площадок 2025
Наш телеграм-канал — ваш гид в мир лучших онлайн-развлечений в РФ! Мы подобрали рейтинг-10 проверенных казино, где вы сможете играть на деньги и получить свои доходы без затруднений.
Что вас ждет:
Честные мнения и оценки Актуальные казино 2025 года рейтинг и обзоры от настоящих геймеров. Отличная статус всякого сайта проверена годами и клиентами. Бесплатная запись и мгновенный доступ на всех площадках. Рабочие дубликаты для посещения к вашему излюбленному казино в каждый момент. Мобильное программа для комфортной игры там угодно.
Почему нам?
Проверенные и проверенные игровые сайты с превосходными параметрами для развлечения. Защита ваших сведений и операций защищена. Свежие новости и новости пространства интернет-игр в РФ.
Слушайте, гейы!
Желаете попасть в пространство виртуальных-игр и получить настоящие деньги? Тогда вам тут! Топ самых лучших игровых сайтов 2025
Наш тг-профиль — ваш проводник в окружение лучших онлайн-игр в России! Мы подобрали топ-10 проверенных казино, где вы получите возможность проводить время на деньги и получить свои доходы без проблем.
Что вас ждет:
Честные отзывы и ранги Турниры и соревнования в казино кто лидирует от настоящих участников. Превосходная имя всякого площадки гарантирована опытом и игроками. Неоплачиваемая запись и мгновенный доступ на каждой площадках. Активные копии для входа к вашему излюбленному казино в любое время. Мобильная программа для удобной игры там угодно.
Почему мы?
Безопасные и гарантированные казино с отличными условиями для развлечения. Защита ваших информации и операций обеспечена. Свежие новости и новости мира онлайн-развлечений в России.
Слушайте, гейы!
Готовы погрузиться в пространство интернет-игр и получить действительные средства? Тогда вам тут! Рейтинг самых превосходных игровых сайтов 2025
Наш тг-профиль — ваш проводник в окружение превосходных интернет-игр в России! Мы собрали ТОП-10 безопасных игровых сайтов, где вы сможете играть на средства и получить свои выигрыши без проблем.
Что вас ждет:
Достоверные отзывы и оценки казино игровые автоматы играть бесплатно онлайн без регистрации от реальных участников. Отличная статус каждого сайта гарантирована опытом и игроками. Неоплачиваемая запись и быстрый вход на каждой ресурсах. Рабочие дубликаты для доступа к вашему предпочитаемому игровому сайту в каждый время. Мобильное программа для удобной развлечения там желаете.
Почему мы?
Безопасные и надежные игровые сайты с отличными критериями для проведения времени. Гарантия ваших сведений и переводов защищена. Новые обновления и новости мира интернет-развлечений в РФ.
Внимание, игроки!
Желаете окунуться в мир интернет-казино и получить настоящие финансы? Тогда вам сюда! Топ лучших отличных игровых сайтов 2025
Наш тг-канал — ваш путеводитель в пространство отличных интернет-игр в Российской Федерации! Мы подобрали рейтинг-10 проверенных игровых площадок, где вы сумеете развлекаться на финансы и получить свои призы без трудностей.
Что вас ждет:
Честные рекомендации и оценки реальные казино онлайн от действительных участников. Превосходная имя всякого площадки гарантирована временем и клиентами. Неоплачиваемая запись и скорый доступ на любой сайтах. Активные копии для доступа к вашему излюбленному игровой площадке в любой время. Мобильный софт для легкой развлечения так угодно.
Почему нас?
Проверенные и надежные игровые площадки с превосходными условиями для проведения времени. Безопасность ваших информации и транзакций обеспечена. Новые события и события окружения интернет-игр в Российской Федерации.
Внимание, участники!
Желаете погрузиться в мир виртуальных-игр и выиграть настоящие деньги? Тогда вам к нам! Топ наилучших отличных игровых площадок 2025
Наш тг-профиль — ваш гид в окружение превосходных онлайн-развлечений в России! Мы подобрали топ-10 безопасных игровых сайтов, где вы сумеете играть на деньги и получить свои призы без трудностей.
Что вас ожидает:
Честные отзывы и рейтинги самое крупное онлайн казино от реальных участников. Превосходная репутация любого ресурса подтверждена временем и игроками. Неоплачиваемая запись и скорый вход на любой сайтах. Рабочие зеркала для входа к вашему излюбленному казино в любой момент. Мобильный приложение для комфортной игры так хотите.
Почему нам?
Проверенные и гарантированные игровые сайты с лучшими параметрами для проведения времени. Безопасность ваших сведений и транзакций гарантирована. Актуальные обновления и новости окружения интернет-игр в Российской Федерации.
Обратите внимание, участники!
Хотите окунуться в пространство интернет-развлечений и заработать реальные финансы? Тогда вам тут! Рейтинг лучших лучших игровых сайтов 2025
Наш телеграм-аккаунт — ваш гид в мир отличных виртуальных-развлечений в Российской Федерации! Мы собрали рейтинг-10 проверенных игровых площадок, где вы сможете играть на финансы и вывести свои выигрыши без проблем.
Что вас ожидает:
Честные рекомендации и ранги казино на деньги с выводом денег от настоящих участников. Превосходная репутация каждого ресурса проверена опытом и игроками. Бесплатная запись и мгновенный доступ на всех ресурсах. Рабочие дубликаты для доступа к вашему предпочитаемому игровому сайту в каждый момент. Мобильная софт для легкой развлечения так угодно.
Почему нас?
Надежные и надежные казино с превосходными параметрами для проведения времени. Защита ваших информации и переводов защищена. Новые события и события окружения виртуальных-развлечений в России.
Обратите внимание, гейы!
Желаете погрузиться в пространство интернет-игр и выиграть настоящие средства? Тогда вам сюда! Список самых отличных игровых сайтов 2025
Наш телеграм-профиль — ваш путеводитель в пространство превосходных онлайн-развлечений в России! Мы подготовили ТОП-10 надежных игровых сайтов, где вы получите возможность развлекаться на финансы и вывести свои доходы без трудностей.
Что вас ожидает:
Правдивые мнения и оценки Новейшие слоты и игровые автоматы рейтинг популярности в онлайн-казино от настоящих игроков. Превосходная репутация всякого площадки подтверждена годами и клиентами. Бесплатная регистрация и быстрый вход на каждой ресурсах. Рабочие зеркала для посещения к вашему излюбленному игровому сайту в любой час. Мобильное приложение для удобной развлечения так желаете.
Почему нас?
Безопасные и проверенные казино с лучшими критериями для развлечения. Гарантия ваших информации и переводов гарантирована. Новые обновления и события мира интернет-казино в Российской Федерации.
Обратите внимание, гейы!
Желаете окунуться в мир интернет-казино и выиграть реальные деньги? Тогда вам к нам! Рейтинг наилучших отличных игровых сайтов 2025
Наш telegram-профиль — ваш проводник в пространство отличных виртуальных-игр в Российской Федерации! Мы подобрали рейтинг-10 безопасных игровых сайтов, где вы сумеете играть на средства и забрать свои выигрыши без затруднений.
Что вас поджидает:
Честные мнения и рейтинги Новейшие слоты и игровые автоматы рейтинг популярности от настоящих участников. Отличная статус каждого сайта подтверждена годами и пользователями. Безоплатная регистрация и скорый доступ на любой сайтах. Рабочие дубликаты для посещения к вашему излюбленному казино в любой момент. Мобильное софт для легкой развлечения там желаете.
Почему мы?
Безопасные и проверенные игровые сайты с лучшими параметрами для развлечения. Гарантия ваших сведений и операций защищена. Актуальные события и события окружения онлайн-игр в Российской Федерации.
Слушайте, участники!
Хотите попасть в пространство виртуальных-развлечений и получить настоящие средства? Тогда вам тут! Рейтинг самых лучших игровых сайтов 2025
Наш телеграм-аккаунт — ваш проводник в окружение лучших онлайн-игр в Российской Федерации! Мы подобрали ТОП-10 надежных игровых сайтов, где вы сможете развлекаться на финансы и вывести свои доходы без затруднений.
Что вас поджидает:
Честные рекомендации и рейтинги Выбор казино для профессиональных игроков топ-рекомендации от настоящих игроков. Отличная статус любого площадки подтверждена опытом и пользователями. Безоплатная регистрация и скорый вход на каждой сайтах. Действующие дубликаты для входа к вашему любимому игровой площадке в любое момент. Мобильная приложение для удобной игры где угодно.
Почему мы?
Проверенные и гарантированные казино с лучшими критериями для проведения времени. Защита ваших сведений и переводов обеспечена. Новые события и новости окружения интернет-казино в Российской Федерации.
Слушайте, игроки!
Готовы попасть в мир онлайн-развлечений и выиграть реальные средства? Тогда вам к нам! Список лучших лучших игровых площадок 2025
Наш telegram-канал — ваш гид в окружение лучших онлайн-развлечений в РФ! Мы собрали топ-10 надежных игровых площадок, где вы сумеете развлекаться на финансы и вывести свои призы без затруднений.
Что вас ждет:
Честные рекомендации и оценки Мобильные приложения онлайн-казино лучший выбор для смартфонов от настоящих геймеров. Хорошая репутация любого сайта гарантирована годами и пользователями. Безоплатная запись и быстрый вход на любой сайтах. Действующие копии для доступа к вашему предпочитаемому казино в любой время. Мобильное приложение для удобной развлечения где желаете.
Почему мы?
Проверенные и проверенные игровые площадки с превосходными параметрами для развлечения. Гарантия ваших информации и операций обеспечена. Свежие события и новости пространства онлайн-развлечений в Российской Федерации.
Обратите внимание, гейы!
Желаете погрузиться в мир интернет-казино и заработать действительные финансы? Тогда вам сюда! Топ самых лучших казино 2025
Наш телеграм-профиль — ваш проводник в мир превосходных онлайн-казино в РФ! Мы подобрали ТОП-10 надежных казино, где вы сумеете проводить время на финансы и получить свои доходы без проблем.
Что вас ждет:
Честные отзывы и оценки казино играть бесплатно без регистрации демо от настоящих участников. Превосходная репутация всякого сайта проверена годами и пользователями. Безоплатная регистрация и быстрый доступ на любой сайтах. Рабочие дубликаты для доступа к вашему любимому казино в каждый час. Мобильный программа для комфортной проведения времени так желаете.
Почему нас?
Надежные и надежные игровые сайты с отличными критериями для игры. Защита ваших данных и транзакций обеспечена. Свежие события и новости мира онлайн-игр в России.
Внимание, участники!
Готовы погрузиться в окружение интернет-игр и выиграть реальные финансы? Тогда вам к нам! Топ наилучших лучших казино 2025
Наш тг-профиль — ваш гид в мир отличных виртуальных-развлечений в России! Мы подготовили топ-10 безопасных игровых сайтов, где вы сможете проводить время на финансы и вывести свои доходы без затруднений.
Что вас ждет:
Правдивые мнения и оценки реальные казино онлайн от реальных участников. Превосходная репутация всякого сайта проверена годами и пользователями. Неоплачиваемая регистрация и мгновенный вход на всех сайтах. Рабочие зеркала для доступа к вашему излюбленному игровому сайту в любой момент. Мобильное программа для комфортной развлечения где хотите.
Почему нам?
Проверенные и проверенные игровые площадки с лучшими параметрами для развлечения. Защита ваших данных и операций защищена. Свежие события и новости мира онлайн-развлечений в Российской Федерации.
Обратите внимание, гейы!
Желаете окунуться в мир онлайн-казино и получить настоящие средства? Тогда вам сюда! Топ лучших лучших казино 2025
Наш телеграм-аккаунт — ваш проводник в пространство превосходных онлайн-развлечений в России! Мы подготовили топ-10 проверенных казино, где вы сможете играть на финансы и получить свои доходы без трудностей.
Что вас ожидает:
Достоверные отзывы и ранги казино деньгами от реальных игроков. Превосходная статус каждого ресурса проверена опытом и пользователями. Неоплачиваемая запись и мгновенный вход на любой площадках. Действующие дубликаты для посещения к вашему излюбленному игровому сайту в каждый момент. Мобильная приложение для комфортной развлечения там хотите.
Почему мы?
Проверенные и гарантированные игровые площадки с отличными условиями для проведения времени. Защита ваших информации и транзакций защищена. Актуальные события и обновления окружения онлайн-развлечений в России.
Внимание, игроки!
Хотите погрузиться в мир виртуальных-игр и заработать настоящие финансы? Тогда вам тут! Топ самых отличных игровых площадок 2025
Наш telegram-профиль — ваш проводник в пространство отличных виртуальных-казино в Российской Федерации! Мы собрали ТОП-10 надежных игровых площадок, где вы получите возможность проводить время на финансы и вывести свои выигрыши без проблем.
Что вас ожидает:
Достоверные рекомендации и рейтинги Где играть бесплатно Топ-казино с демо-версиями игр от действительных участников. Превосходная имя любого ресурса проверена временем и пользователями. Бесплатная вход и мгновенный доступ на всех сайтах. Действующие копии для доступа к вашему предпочитаемому игровому сайту в любое час. Мобильное программа для удобной развлечения где хотите.
Почему мы?
Проверенные и проверенные игровые площадки с лучшими критериями для проведения времени. Безопасность ваших информации и операций защищена. Актуальные события и новости мира интернет-казино в Российской Федерации.
Слушайте, участники!
Желаете попасть в мир интернет-развлечений и заработать настоящие деньги? Тогда вам сюда! Топ самых отличных казино 2025
Наш телеграм-аккаунт — ваш гид в пространство отличных виртуальных-развлечений в РФ! Мы собрали рейтинг-10 проверенных игровых сайтов, где вы сумеете проводить время на средства и получить свои призы без трудностей.
Что вас ждет:
Достоверные мнения и оценки онлайн казино на деньги с моментальным выводом от настоящих игроков. Отличная имя каждого сайта гарантирована годами и игроками. Безоплатная вход и мгновенный вход на всех площадках. Активные копии для доступа к вашему излюбленному игровой площадке в каждый время. Мобильная программа для легкой проведения времени так хотите.
Почему мы?
Проверенные и надежные игровые сайты с отличными условиями для игры. Защита ваших сведений и операций защищена. Свежие новости и новости мира онлайн-игр в Российской Федерации.
Пoker В сети: Ваш личный Руководство в Сферу Азартных Тактик
Хотите незабываемых переживаний и когнитивных задач? Мир покера онлайн готов принять тебя! Эта игра не просто захватывающая забава, это целая мир, где переплетаются математика, эмоции и тактика. Решились окунуться в такое интересный окружение?
Какие аспекты делает Poker в сети таким интересным?
Удобство: Играть можно там угодно и когда угодно, требуется всего соединение в сеть. Вариативность: Большое количество типов и вариантов Poker (Техас Холдем poker, Омаха poker и т.д.). Возможность получить: Poker в сети позволяет не только лишь отдыхать, а также еще получать на деньги. Соревнование: Вы сами можешь конкурировать с участниками со всей земли. Обучение: Вы можешь улучшать свои навыки и совершенствовать стратегию.
С какой точки начинать новому игроку?
Подбор надежного сайта: Отыщите надежный и отличный платформа или покеррум с отличными мнениями и рейтингом. Регистрационная процедура: Процесс регистрационного процесса обязан стать удобным и понятным. Ознакомление правил: Начните с базовых инструкций избранной разновидности покера. Тренировочный режим: Попробуйте сыграть в демо-режиме, чтобы понять принципы развлечения. Начальный взнос: Начинайте играть на реальные средства с первоначальным взносом. Контроль деньгами: Контролируйте твоими финансами с умом, не играться на последние деньги.
Как подобрать хороший игровой зал?
Разрешение: Факт наличия лицензии говорит о надежности платформы. Скорость изъятия: Обратите фокус на скорость и методы снятия денег. Техподдержка помощь: Качественная и скорая помощь поспособствует устранить любые задачи. Мобильный версия: Удобное мобильная версия приложения позволит сыграть в каждый час и в любом месте. Подарки и акции: Приветственные бонусы и частые спецпредложения способны увеличить твой первоначальный финансы.
Подсказки для новых игроков:
Не ускоряйтесь: Начинайте с низких ограничений, чтобы набраться знаний. Обучайтесь: Непрерывно развивайте свои способности, просматривайте руководства и наблюдайте видео. Анализируйте: Изучайте вашу развлечение, ищите ошибки и трудитесь над этими проблемами. Управляйте чувства: Играйся уравновешенно, не поддавайтесь желанию. Играйся ответственно: Не забывайте, что игра – это забава, а не способ мгновенного повышения.
Игра онлайн Покер онлайн на деньги с выводом – это интересная развлечение, которая может дать Вам не только лишь развлечение и удовольствие, а также и возможность получать настоящие средства. Главное – правильно подойти к это процессам, и победа не заставит себя ждать!
А какой ваш предпочтительный покер-рум? Какой платформа Вы считаете хорошим для развлечения на деньги? Делитесь своим опытом в комментариях!
#покер онлайн #играть в poker #покер-рум #лучший ресурс #регистрация #вывод средств #минимальныйдепозит #загрузить #наденьги #мобильный #топ список #рейтинг #2025 год
Карточная игра В сети: Ваш личный Справочник в Сферу Увлекательных Стратегий
Мечтаете незабываемых впечатлений и умственных испытаний? Область Poker в сети ждет Вас! Эта игра не только увлекательная игра, это?ная сфера, где переплетаются расчеты, эмоции и тактика. Решились войти в такое увлекательный мир?
Что отличают игру в сети столь заманчивым?
Удобство: Сыграть можно где желаете и в любой момент хотите, требуется лишь подключение в сеть. Разнообразие: Множество видов и вариантов покера (Техасский Холдем Холдем poker, Омаха и прочие). Шанс получать: Покер в интернете дает не только развлекаться, а также еще получать на деньги. Конкуренция: Вы в состоянии соперничать с участниками со всей мира. Обучение: Вы сами можешь улучшать ваши умения и улучшать тактику.
С какого момента начать начинающему?
Отбор надежного сайта: Ищите надежный и лучший сайт или покер-рум с отличными мнениями и оценкой. Регистрация: Процесс регистрационной процедуры должен быть удобным и интуитивно понятным. Изучение руководств: Стартуйте с основных руководств избранной разновидности игры. Демо-режим: Поиграйте поиграть в пробном режиме, чтобы узнать принципы игры. Первоначальный депозит: Начинайте сыграть на реальные средства с минимальным взносом. Управление банкроллом: Управляйте своими деньгами с умом, не играйте на остаточные финансы.
Каким способом выбрать хороший игровой зал?
Сертификат: Присутствие сертификата говорит о надежности сайта. Быстрота снятия: Обязательно обратите внимание на быстроту и способы снятия денег. Техническая содействие: Хорошая и скорая поддержка посодействует устранить любые проблемы. Мобильная версия приложения: Удобное мобильная версия приложения даст сыграть в любое время и в любой уголке мира. Бонусы и предложения: Приветственные поощрения и постоянные предложения могут повысить твой стартовый средства.
Советы для начинающих:
Не спешите: Начните с низких лимитов, чтобы получить опыта. Обучайтесь: Регулярно развивайте ваши умения, читайте руководства и наблюдайте фильмы. Разбирайте: Анализируйте вашу процесс, обнаруживайте промахи и трудитесь над ними. Управляйте эмоции: Играйте спокойно, не поддавайтесь желанию. Играйте ответственно: Не забывайте, что покер – это увлечение, а не путь мгновенного обогащения.
Poker в интернете Покер онлайн играть на деньги 2025 с выводом – это захватывающая игра, которая способна предоставить тебе не просто увлечение и удовольствие, но также возможность заработать реальные деньги. Важное – верно подойти к этим процессу, и удача не заставит себя ждать!
Какой у вас ваш излюбленный игровой зал? Какой сайт вы считаете отличным для забавы на деньги? Делитесь вашим опытом в комментариях!
#покеронлайн #играть в poker #покер-рум #лучшийсайт #регистрационная процедура #выводденег #начальный депозит #установить #на средства #мобильное #топ #рейтинг #2025
Обратите внимание, участники!
Хотите попасть в пространство виртуальных-игр и получить действительные финансы? Тогда вам тут! Топ самых отличных игровых площадок 2025
Наш телеграм-аккаунт — ваш проводник в пространство лучших виртуальных-казино в Российской Федерации! Мы подготовили ТОП-10 безопасных игровых площадок, где вы сможете играть на средства и получить свои призы без затруднений.
Что вас ожидает:
Достоверные рекомендации и рейтинги Казино с минимальным депозитом от действительных участников. Отличная репутация любого площадки проверена годами и клиентами. Бесплатная вход и скорый доступ на всех площадках. Рабочие копии для доступа к вашему предпочитаемому игровой площадке в любой момент. Мобильное программа для удобной проведения времени там хотите.
Почему нам?
Безопасные и гарантированные игровые сайты с лучшими критериями для проведения времени. Защита ваших информации и транзакций обеспечена. Актуальные новости и события окружения виртуальных-игр в Российской Федерации.
Слушайте, игроки!
Хотите попасть в мир интернет-развлечений и получить настоящие деньги? Тогда вам тут! Рейтинг лучших превосходных казино 2025
Наш телеграм-аккаунт — ваш путеводитель в пространство отличных виртуальных-игр в РФ! Мы подобрали ТОП-10 безопасных игровых площадок, где вы сможете развлекаться на деньги и забрать свои выигрыши без трудностей.
Что вас поджидает:
Достоверные отзывы и рейтинги Казино с бонусом от действительных игроков. Отличная репутация любого ресурса подтверждена годами и игроками. Безоплатная регистрация и скорый вход на любой сайтах. Рабочие зеркала для входа к вашему предпочитаемому игровой площадке в любое час. Мобильное приложение для удобной развлечения где хотите.
Почему нас?
Безопасные и гарантированные казино с превосходными условиями для игры. Безопасность ваших сведений и операций защищена. Свежие новости и обновления пространства интернет-казино в Российской Федерации.
Внимание, игроки!
Готовы окунуться в мир онлайн-игр и выиграть настоящие средства? Тогда вам к нам! Рейтинг самых отличных игровых площадок 2025
Наш телеграм-профиль — ваш гид в мир превосходных интернет-игр в России! Мы собрали рейтинг-10 безопасных казино, где вы сможете развлекаться на средства и вывести свои доходы без проблем.
Что вас поджидает:
Правдивые мнения и рейтинги Казино с бездепозитным бонусом от действительных геймеров. Отличная репутация любого ресурса проверена временем и клиентами. Неоплачиваемая запись и скорый вход на каждой сайтах. Рабочие зеркала для входа к вашему предпочитаемому казино в любой время. Мобильное софт для легкой проведения времени где хотите.
Почему нас?
Проверенные и проверенные казино с отличными критериями для проведения времени. Защита ваших данных и операций гарантирована. Актуальные обновления и обновления окружения интернет-игр в Российской Федерации.
Слушайте, игроки!
Хотите попасть в мир интернет-развлечений и заработать настоящие средства? Тогда вам сюда! Рейтинг самых отличных игровых площадок 2025
Наш telegram-канал — ваш гид в мир превосходных онлайн-игр в РФ! Мы подготовили ТОП-10 проверенных казино, где вы получите возможность проводить время на средства и получить свои выигрыши без проблем.
Что вас ждет:
Достоверные рекомендации и ранги Казино с хорошими отзывами игроков от реальных участников. Хорошая имя каждого сайта проверена опытом и клиентами. Бесплатная вход и мгновенный вход на каждой сайтах. Рабочие копии для доступа к вашему любимому игровому сайту в любой время. Мобильная софт для легкой проведения времени там желаете.
Почему нас?
Надежные и проверенные казино с отличными критериями для проведения времени. Гарантия ваших сведений и переводов обеспечена. Актуальные события и события пространства интернет-казино в Российской Федерации.
Внимание, гейы!
Хотите окунуться в пространство онлайн-развлечений и получить реальные средства? Тогда вам тут! Топ наилучших лучших игровых сайтов 2025
Наш telegram-профиль — ваш путеводитель в мир превосходных интернет-казино в РФ! Мы подготовили ТОП-10 проверенных игровых сайтов, где вы сумеете развлекаться на деньги и вывести свои призы без проблем.
Что вас поджидает:
Правдивые мнения и рейтинги Казино самые популярные от реальных участников. Превосходная имя каждого площадки подтверждена годами и клиентами. Безоплатная регистрация и скорый вход на всех сайтах. Активные копии для входа к вашему излюбленному казино в каждый момент. Мобильное приложение для комфортной игры где желаете.
Почему нас?
Безопасные и надежные казино с лучшими критериями для развлечения. Защита ваших информации и транзакций обеспечена. Новые новости и новости окружения виртуальных-игр в России.
Обратите внимание, гейы!
Хотите погрузиться в пространство интернет-казино и заработать настоящие деньги? Тогда вам к нам! Топ самых отличных игровых сайтов 2025
Наш telegram-аккаунт — ваш гид в мир лучших интернет-казино в России! Мы подготовили ТОП-10 безопасных игровых сайтов, где вы получите возможность развлекаться на средства и забрать свои доходы без проблем.
Что вас поджидает:
Правдивые рекомендации и оценки Казино с широким выбором слотов от действительных геймеров. Хорошая имя всякого площадки гарантирована годами и игроками. Бесплатная вход и быстрый вход на каждой площадках. Активные копии для входа к вашему излюбленному казино в каждый время. Мобильная приложение для комфортной игры где желаете.
Почему нам?
Проверенные и проверенные игровые площадки с превосходными параметрами для развлечения. Безопасность ваших данных и транзакций гарантирована. Свежие новости и новости окружения онлайн-развлечений в Российской Федерации.
Слушайте, участники!
Желаете окунуться в пространство виртуальных-развлечений и заработать реальные средства? Тогда вам тут! Топ лучших превосходных игровых площадок 2025
Наш тг-канал — ваш гид в мир лучших онлайн-развлечений в Российской Федерации! Мы подготовили ТОП-10 надежных казино, где вы сумеете проводить время на средства и получить свои выигрыши без трудностей.
Что вас ждет:
Правдивые мнения и ранги Казино для телефона от настоящих игроков. Хорошая имя любого площадки гарантирована временем и игроками. Безоплатная вход и скорый вход на любой площадках. Активные дубликаты для доступа к вашему излюбленному игровому сайту в любой час. Мобильный софт для легкой игры так хотите.
Почему нам?
Безопасные и гарантированные казино с отличными критериями для развлечения. Защита ваших сведений и операций обеспечена. Свежие обновления и обновления окружения виртуальных-игр в России.
Слушайте, участники!
Хотите окунуться в мир виртуальных-игр и выиграть настоящие финансы? Тогда вам тут! Список наилучших отличных казино 2025
Наш telegram-аккаунт — ваш путеводитель в пространство отличных онлайн-казино в Российской Федерации! Мы подобрали ТОП-10 безопасных игровых площадок, где вы сумеете проводить время на средства и получить свои выигрыши без проблем.
Что вас ожидает:
Достоверные отзывы и рейтинги Казино с быстрым выводом от реальных участников. Хорошая статус каждого площадки проверена временем и пользователями. Неоплачиваемая регистрация и скорый вход на любой сайтах. Действующие копии для доступа к вашему предпочитаемому казино в любой момент. Мобильный программа для комфортной игры так желаете.
Почему нас?
Проверенные и проверенные игровые площадки с лучшими условиями для развлечения. Гарантия ваших информации и транзакций гарантирована. Свежие новости и обновления пространства виртуальных-развлечений в России.
Обратите внимание, игроки!
Готовы погрузиться в пространство онлайн-развлечений и получить реальные деньги? Тогда вам тут! Список наилучших лучших игровых сайтов 2025
Наш telegram-аккаунт — ваш гид в пространство отличных интернет-развлечений в РФ! Мы подобрали топ-10 надежных казино, где вы сможете играть на деньги и получить свои доходы без проблем.
Что вас ожидает:
Правдивые мнения и оценки Казино с программой лояльности от действительных участников. Отличная статус всякого площадки проверена опытом и клиентами. Безоплатная запись и мгновенный вход на каждой сайтах. Рабочие зеркала для доступа к вашему предпочитаемому игровому сайту в любое час. Мобильное приложение для комфортной игры там угодно.
Почему нам?
Надежные и гарантированные игровые сайты с отличными условиями для развлечения. Безопасность ваших сведений и операций защищена. Актуальные новости и обновления мира виртуальных-казино в России.
Слушайте, участники!
Хотите окунуться в окружение онлайн-игр и заработать реальные средства? Тогда вам к нам! Рейтинг лучших лучших казино 2025
Наш telegram-канал — ваш гид в окружение отличных онлайн-игр в РФ! Мы собрали топ-10 безопасных игровых площадок, где вы сможете играть на средства и забрать свои выигрыши без трудностей.
Что вас ожидает:
Достоверные рекомендации и оценки Казино с быстрой регистрацией от действительных участников. Хорошая имя каждого сайта проверена годами и игроками. Безоплатная вход и скорый вход на каждой сайтах. Действующие дубликаты для доступа к вашему любимому игровой площадке в каждый время. Мобильный приложение для удобной игры где угодно.
Почему мы?
Безопасные и гарантированные игровые сайты с превосходными параметрами для игры. Защита ваших данных и переводов защищена. Новые обновления и обновления пространства виртуальных-развлечений в России.
Профессиональная аналитика: Забудьте насчет пари “для везение”! Наша группа опытных аналитиков каждый день анализирует данные, следит за новостями и выявляет максимально многообещающие игры. Мы не угадываем – мы анализируем! ? Четкие предсказания: Мы предлагаем не просто прогнозы, а обоснованные решения, подтвержденные данными и экспертным суждением. Главная цель – твоя доход! ? Многообразие категорий состязаний: Футбольный матч, баскетбольные поединки, теннисные матчи, хоккейные матчи а многое других! Мы затрагиваем максимально известные типы состязаний, чтоб абсолютно каждый смог открыть что нибудь захватывающее для себя. ? Бесплатный содержание: Каждый день свободные прогнозы, детальные тексты, разборы матчей и ценные наставления для начинающих бетторов. Мы распостраняем информацией, для того чтобы вы прогрессировали вместе с нами! ? Содействие постоянно: Мы всегда рады ответить на ваши запросы и оказать помощь углубиться в особенностях области пари. Вы не останетесь самостоятельно на самом с этим сложным, но прибыльным предприятием! ? Ясность и правдивость: Мы открыто публикуем показатели наших прогнозов и не прячем ничегошеньки от своих клиентов. Наша доброе имя – наш основной актив! ? Практичный формат: Абсолютно все прогнозирования а аналитика доступны сразу в Telegram, в комфортном и понятном способе. Никаких сложных интерфейсов и хитромудрых планов!
Что вы приобретете, подписавшись на Ставки на спорт рубли сообщество азвание вашего канала]?
Каждый день предсказания с высокой результативностью.
Аналитические обзоры поединков.
Разборы атлетических новостей.
Полезные наставления по распоряжению средствами.
Шанс коммуницировать с соратниками.
Гарантия в собственных бетах.
И, разумеется же, возможность заработать настоящие средства!
Достаточно терять время на бессмысленные дискуссии о спортивных играх! Начни активно участвовать а преврати собственные опыт в доход!
Квалифицированная аналитика: Забудьте про беты “для случайность”! Команда команда опытных аналитиков день за днем изучает статистику, наблюдает за известиями а определяет наиболее многообещающие матчи. Мы не предсказываем – мы исследуем! ? Достоверные прогнозирования: Мы предлагаем не лишь предсказания, а обоснованные решения, подкрепленные данными и экспертным мнением. Основная задача – твоя прибыль! ? Широкий выбор видов состязаний: Футбольный матч, баскетбол, теннисные соревнования, хоккейные матчи а куча других! Мы охватываем самые известные виды спортивных дисциплин, чтобы каждый мог найти нечто захватывающее для себя. ? Бесплатный контент: День за днем свободные прогнозирования, аналитические статьи, анализы игр и необходимые рекомендации для новичков любителей ставок. Мы распостраняем знаниями, чтоб вы прогрессировали вместе с нами! ? Поддержка круглосуточно: Мы всегда способны дать ответ на ваши обращения а содействовать понять в нюансах сферы пари. Вы не будете один на самом с данным непростым, но выгодным занятием! ? Открытость а искренность: Мы не скрывая обнародуем результаты наших прогнозов и не утаиваем абсолютно ничего от наших последователей. Наша имидж – наш главный ценность! ? Практичный вид: Каждый предсказания и разбор предоставляются прямо в Telegram, в удобном и ясном способе. Без каких либо запутанных интерфейсов и хитромудрых планов!
Что ты приобретете, подписавшись на Ставки на спорт рубли паблик азвание вашего канала]?
День за днем прогнозирования с большой результативностью.
Развернутые разборы поединков.
Разборы спортивных событий.
Полезные наставления по управлению средствами.
Потенциал контактировать с соратниками.
Гарантия в своих бетах.
И, безусловно же, потенциал извлечь фактические деньги!
Достаточно растрачивать момент на бессмысленные разговоры про спортивных играх! Приступай активно участвовать и конвертируй личные опыт в доход!
Квалифицированная разбор: Позабудьте про пари “для удачу”! Команда группа опытных аналитиков ежедневно изучает цифры, отслеживает за известиями и находит самые перспективные поединки. Мы не угадываем – мы исследуем! ? Точные предсказания: Мы предлагаем не только предсказания, но обоснованные выводы, подтвержденные показателями и экспертным суждением. Наша задача – твой доход! ? Разнообразие категорий состязаний: Футбол, баскетбол, теннис, хоккей и многое иное! Мы охватываем максимально востребованные категории спорта, для того чтобы абсолютно каждый был способен найти нечто увлекательное для себя. ? Безвозмездный содержание: Каждый день безвозмездные прогнозирования, развернутые статьи, разборы матчей а полезные наставления для начинающих игроков. Мы предоставляем знаниями, чтобы вы росли вместе с нами! ? Содействие 24/7: Мы все время готовы ответить на твои запросы и оказать помощь разобраться в нюансах мира бетов. Вы не останетесь самостоятельно на в одиночку с данным сложным, но доходным предприятием! ? Прозрачность и правдивость: Мы не скрывая размещаем итоги своих прогнозирования и не скрываем ничегошеньки от наших клиентов. Наша репутация – наш основной ценность! ? Практичный формат: Абсолютно все прогнозирования а исследование доступны прямо в Telegram, в простом и ясном формате. Без каких либо сложных интерфейсов и хитромудрых планов!
Что ты получите, зарегистрировавшись на Ставки на спорт стратегии паблик азвание вашего канала]?
Ежедневные предсказания с отличной проходимостью.
Развернутые разборы матчей.
Обзоры спортивных событий.
Ценные наставления по управлению банком.
Потенциал контактировать с соратниками.
Уверенность в своих пари.
И, конечно же, потенциал извлечь фактические финансы!
Хватит терять минуты на бесполезные разговоры про спорте! Приступай действовать и преврати личные опыт в прибыль!
Профессиональная разбор: Оставьте о беты “для удачу”! Собственная команда опытных экспертов каждый день анализирует данные, отслеживает за известиями и находит максимально выгодные матчи. Мы не гадаем – мы анализируем! ? Точные прогнозирования: Мы предоставляем не лишь прогнозы, а аргументированные выводы, основанные цифрами и квалифицированным суждением. Основная цель – ваша выгода! ? Многообразие видов спорта: Футбол, баскетбольные игры, теннис, хоккейные матчи и много иное! Мы покрываем максимально популярные категории состязаний, для того чтобы любой был способен открыть что нибудь увлекательное для себя. ? Бесплатный контент: Ежедневные безвозмездные прогнозы, аналитические тексты, обзоры поединков и ценные советы для новичков бетторов. Мы предоставляем информацией, для того чтобы вы росли совместно с нами! ? Поддержка круглосуточно: Мы всегда способны реагировать на ваши обращения и содействовать углубиться в особенностях мира пари. Вы не будете один на один с данным непростым, но доходным делом! ? Открытость а искренность: Мы публично обнародуем результаты наших предсказаний и не скрываем абсолютно ничего от наших клиентов. Главная репутация – наш ключевой ценность! ? Практичный вид: Каждый прогнозы и исследование предоставляются прямо в Telegram, в удобном и понятном способе. Никаких сложных оболочек и хитромудрых схем!
Что вы приобретете, зарегистрировавшись на Ставки на спорт игры канал азвание вашего канала]?
Ежедневные прогнозирования с отличной результативностью.
Аналитические обзоры игр.
Разборы атлетических известий.
Полезные советы по управлению банком.
Шанс общаться с партнерами.
Уверенность в своих пари.
А, разумеется же, потенциал получить настоящие деньги!
Хватит растрачивать момент на бессмысленные беседы про спортивных соревнованиях! Начни действовать и конвертируй собственные опыт в прибыль!
If you have diarrhea that is watery or bloody, stop taking G DOX and call your doctor buy clomid for research Cross validation of technologies for genotyping CYP2D6 and CYP2C19
Азартное заведение В сети: Ваш Гид в Свет Азартных Развлечений
Что такое сетевое азартное заведение и каким образом выбрать безопасное?
Азартное заведение онлайн – является виртуальные площадки, предлагающие широкий диапазон азартных игр: с классических игровых автоматов и слотов по увлекательных игральных развлечений, как рулетка, блэкджек и игра в карты. Но, чтобы достичь максимальное наслаждение из развлечения, важно выбрать безопасное казино, которое обеспечивает честность и защищенность.
Основные параметры подбора:
Разрешение: Лицензионные казино гарантируют справедливость игры и защищенность ваших средств. Убедитесь в наличие лицензии до регистрацией.
Рейтинг и отзывы: Изучите рейтинг азартного заведения и комментарии о казино со стороны других игроков. Данное поможет тебе выбрать проверенное заведение.
Бонусы и спецпредложения: Азартные заведения с поощрениями предоставляют привлекательные условия для новых и постоянных пользователей. Уделите внимание к бездепозитные поощрения, халявные вращения и другие акции.
Ассортимент игр: Лучшие онлайн казино предлагают широкий ассортимент игр для каждый вкус. Находите азартные заведения с азартными слотами, колесом фортуны, блэкджеком, покером и другими развлечениями.
Снятие денег: Позаботьтесь, что казино с снятием денег предлагает комфортные и оперативные варианты получения выигрыша.
Помощь: Надежные казино обеспечивают качественную помощь по российском языке ради пользователей со РФ.
Безопасность: Защищенные казино обеспечивают сохранность твои сведения с помощью современных технологий шифрования.
?? Поощрения и спецпредложения в сетевом казино
Сетевые казино заманивают новых игроков обильными поощрениями азартного заведения. В ходе подборе места, уделите внимание на:
Приветственный бонус: Вступительный бонус при регистрацию – превосходная шанс запустить развлечение с дополнительными средствами.
Бесплатный бонус: Бесплатные поощрения позволяют развлекаться в казино бесплатно, без внося деньги.
Бесплатные вращения: Бесплатные вращения или бесплатные прокрутки – шанс развлечься в слоты бесплатно.
Промокоды: Промокоды казино дают резервные поощрения и преимущества.
Кэшбэк: Возврат азартного заведения отдает обратно часть проигранных денег.
Спецпредложения азартного заведения: Участвуйте в постоянных спецпредложениях и принимайте резервные поощрения.
Разнообразие развлечений в онлайн казино
В онлайн азартном заведении ты обнаружите большой ассортимент игровых развлечений:
Азартные слоты: Игровые автоматы онлайн или игровые автоматы – наиболее популярный вид развлечений.
Колесо фортуны: Традиционная развлечение, которая не лишается своей актуальности.
Двадцать одно: Умственная игральная игра, нуждающаяся планирования.
Покер: Многообразные виды покера ради поклонников карточных развлечений.
Баккара, бинго, кено: Иные популярные азартные развлечения.
Живое казино: Наслаждайтесь игрой с живыми раздающими.
Мобильное азартное заведение: играйте в всяком точке
Переносное азартное заведение – это комфортный способ получать удовольствие от игрой в всяком месте и в всякое момент. Вы имеете возможность развлекаться в предпочитаемые игры казино прямо со вашего мобильника или планшета.
Где начать развлекаться в онлайн казино?
Дабы развлекаться в казино и получать удовольствие от азартом, сайт казино следуйте этим легким шагам:
Выберите азартное заведение: Изучите рейтинг казино и подберите надежное заведение.
Запишитесь: Пройдите быструю запись в казино.
Пополните счет: Зачислите счет в азартном заведении удобным способом.
Запустите развлекаться: Выберите игру и наслаждайтесь процессом!
Как выиграть в азартном заведении и как вывести средства?
Развлечение в азартном заведении за средства – это не только игра, а и шанс выиграть. Изучайте, улучшайте свои навыки и развлекайтесь сознательно. Казино с снятием предоставляют скорый и комфортный снятие денег с казино различными способами.
Увлекательный Сфера Онлайн Покера: Играйте на Реальные Деньги и Наслаждайтесь Лучшими Покер-Румами
Ли обожаете карточную игру? Чувствуете азарт, в случае если карточки выпадают удачно, и тактики ведут к победе? Тогда милости пожаловать внутрь мир виртуального игры в покер, в котором адреналин и шанс играть за реальные средства открыты в каждое время и в любом локации. Оставьте за нужде ехать в игровой дом либо приглашать товарищей – теперь все наилучшие покерные столы при вас у близко!
Почему Онлайн Игра в Покер – Является Идеальный Решение?
Удобство и Доступность: Участвуйте в обожаемый покер, не покидая из дома. Нужен всего лишь ПК либо смартфон и доступ к сети.
Огромный Выбор Состязаний: Начиная с традиционного Техасского Холдем до Омаха и остальных необычных видов – любой отыщет состязание по душе и по степени мастерства.
Игра за Настоящие Деньги: Испытайте настоящий волнение, конкурируя с игроками со всего мира. Выигрывайте реальные средства, оттачивая свое мастерство.
Эластичность Ставок: Участвуйте на любом ставке, какой вам комфортен. Начиная с микролимитов для начинающих заканчивая крупных ставок для профессионалов.
Поощрения и Акции: Получайте большие поощрения за запись и вклады, участвуйте к регулярных акциях и соревнованиях с крупными призовыми суммами.
Участвуйте в Самые Лучшие Покер-Румы и Наслаждайтесь Надежностью
Покер онлайн выбрав правильный покер-рум – значимый этап для удобной и справедливой игры. Наша команда предлагаем тебе играть в самые лучшие покерные комнаты, подтвержденные годами и множеством игроков. Эти платформы обеспечивают:
Защищенность и Надежность: Лицензированные покерные комнаты применяют новейшие технологии шифрования, обеспечивая защиту твоих сведений и денег.
Честность Участия: ГСЧ (генераторы непредвиденных значений) гарантируют случайное появление карт, обеспечивая честность и неопределенность любой раздачи.
Комфортный Интерфейс: Интуитивно понятный интерфейс и простая ориентация обеспечат твою игру удобной и приятной.
Большой Выбор Соревнований: Присоединяйтесь в регулярных турнирах, многостоловых соревнованиях (МТТ), Sit-and-Go и прочих захватывающих разновидностях.
Профессиональная Поддержка: Служба помощи постоянно готова оказать помощь тебе с любыми вопросами, появляющимися в ходе игры.
Как Приступить Играть в Виртуальный Игру в Покер за Реальные Деньги?
Выберите Безотказный Покерную Комнату: Ознакомьтесь с оценки и отзывы, чтобы подобрать площадку, которая соответствует твоим запросам.
Зарегистрируйтесь: Порядок записи обычно занимает несколько мгновений.
Внесите Депозит: Положите свой счет удобным для вас способом, чтобы начать играть за реальные деньги.
Выберите Состязание: Стартуйте с легких игр и помаленьку переходите к более сложным вариантам.
Совершенствуйтесь: Анализируйте свои ошибки, изучайте стратегии и приемы участия, чтобы улучшить собственное мастерство.
Не упустите свой возможность испытать везение и стать экспертом покера! Присоединяйтесь к нам и приступайте играть в онлайн покер на настоящие средства уже в настоящее время. Выбирайте лучшие покерные комнаты и наслаждайтесь участием в любое время!
Захватывающий Мир Виртуального Покера: Участвуйте за Настоящие Деньги и Наслаждайтесь Лучшими Покер-Румами
Ли любите покер? Испытываете восторг, когда карты ложатся удачно, и тактики приводят к выигрышу? Тогда милости просим внутрь мир онлайн игры в покер, в котором адреналин и возможность играть на настоящие деньги доступны в любое любое время и в любом любом локации. Оставьте о нужде ехать в казино либо приглашать друзей – теперь абсолютно все наилучшие покерные места при вас под рукой!
Отчего Виртуальный Игра в Покер – Является Прекрасный Выбор?
Удобство и Доступность: Участвуйте в обожаемый игру в покер, не покидая из жилища. Требуется только компьютер либо сотовый и доступ к интернету.
Огромный Выбор Игр: Начиная с классического Техас Холдема заканчивая Омахи и других экзотических видов – любой найдет игру по вкусу и по степени умения.
Игра за Настоящие Деньги: Испытайте подлинный азарт, соревнуясь с игроками со всего света. Зарабатывайте реальные средства, оттачивая свое умение.
Гибкость Вложений: Участвуйте на любом ставке, какой тебе удобен. Начиная с микролимитов для начинающих заканчивая крупных вложений для экспертов.
Бонусы и Акции: Принимайте большие бонусы за регистрацию и депозиты, участвуйте в постоянных акциях и соревнованиях с крупными призовыми суммами.
Играйте в Самые Лучшие Покерные Комнаты и Радуйтесь Качеством
Играть в покер онлайн выбрав правильный покер-рум – значимый этап для комфортной и честной участия. Наша команда предлагаем вам играть в лучшие покерные комнаты, подтвержденные годами и множеством участников. Эти площадки гарантируют:
Безопасность и Надежность: Лицензированные покерные комнаты применяют новейшие методы шифрования, гарантируя безопасность ваших сведений и денег.
Справедливость Участия: ГСЧ (генераторы случайных чисел) обеспечивают произвольное выпадение карт, обеспечивая справедливость и неопределенность любой раздачи.
Комфортный Оформление: Легко усваиваемый интерфейс и легкая навигация сделают вашу участие удобной и приятной.
Обширный Ассортимент Турниров: Присоединяйтесь в регулярных турнирах, многостольных соревнованиях (МТТ), Sit-and-Go и других захватывающих форматах.
Квалифицированная Помощь: Служба поддержки всегда готова оказать помощь вам в какими угодно запросами, появляющимися в процессе участия.
Каким Образом Начать Участвовать в Виртуальный Покер на Настоящие Средства?
Выберите Безотказный Покер-Рум: Ознакомьтесь с оценки и отзывы, чтобы подобрать площадку, которая отвечает твоим требованиям.
Зарегистрируйтесь: Процесс записи как правило занимает пару мгновений.
Сделайте Вклад: Пополните свой счет удобным для тебя способом, чтобы начать играть на настоящие деньги.
Выберите Состязание: Стартуйте с легких состязаний и помаленьку двигайтесь к более сложным вариантам.
Совершенствуйтесь: Изучайте свои ошибки, осваивайте тактики и тактики игры, чтобы повысить собственное мастерство.
Не упустите свой шанс испытать везение и стать мастером игры в покер! Присоединяйтесь к нам и начните играть в виртуальный покер за настоящие деньги прямо в настоящее время. Подбирайте лучшие покерные комнаты и наслаждайтесь игрой в каждое мгновение!
Увлекательный Сфера Онлайн Покера: Участвуйте на Реальные Средства и Наслаждайтесь Самыми Лучшими Покерными Комнатами
Вы обожаете покер? Испытываете азарт, в случае если карты выпадают благоприятно, и тактики ведут к победе? В таком случае добро пожаловать в сферу виртуального игры в покер, в котором волнение и шанс участвовать за настоящие средства доступны в любое каждое мгновение и в любом каком угодно локации. Забудьте за нужде ехать в казино или собирать товарищей – теперь абсолютно все лучшие покерные места у тебя у рукой!
Отчего Виртуальный Игра в Покер – Является Идеальный Решение?
Удобство и Доступность: Участвуйте в обожаемый игру в покер, не покидая из дома. Нужен только компьютер или смартфон и подключение к интернету.
Огромный Выбор Состязаний: От классического Техас Холдема заканчивая Омахи и остальных экзотических разновидностей – любой отыщет состязание по вкусу и по степени умения.
Игра на Настоящие Средства: Почувствуйте настоящий волнение, конкурируя против участниками со всего света. Зарабатывайте настоящие средства, оттачивая свое мастерство.
Гибкость Ставок: Играйте на каком угодно ставке, какой вам удобен. От малых ставок для новичков заканчивая крупных ставок для профессионалов.
Поощрения и Акции: Получайте щедрые поощрения за регистрацию и вклады, участвуйте в постоянных акциях и турнирах с крупными наградными суммами.
Играйте в Самые Лучшие Покерные Комнаты и Наслаждайтесь Качеством
Покер онлайн выбрав правильный покер-рум – значимый шаг для комфортной и честной участия. Наша команда предлагаем тебе играть в самые лучшие покерные комнаты, подтвержденные временем и множеством участников. Данные площадки обеспечивают:
Защищенность и Безотказность: Официальные покерные комнаты применяют новейшие технологии шифрования, обеспечивая защиту ваших сведений и денег.
Справедливость Участия: ГСЧ (генераторы непредвиденных значений) гарантируют произвольное появление карт, гарантируя честность и непредсказуемость каждой раздачи.
Комфортный Оформление: Легко усваиваемый интерфейс и простая навигация сделают твою игру комфортной и радостной.
Большой Выбор Соревнований: Участвуйте в ежедневных состязаниях, многостольных соревнованиях (МТТ), Sit-and-Go и других захватывающих разновидностях.
Профессиональная Помощь: Сервис поддержки постоянно готова помочь тебе в любыми запросами, появляющимися в ходе участия.
Как Начать Участвовать в Виртуальный Покер на Настоящие Деньги?
Выберите Безотказный Покерную Комнату: Ознакомьтесь с оценки и отзывы, чтобы выбрать площадку, которая отвечает твоим запросам.
Запишитесь: Процесс записи обычно требует пару мгновений.
Внесите Вклад: Пополните свой баланс подходящим для тебя способом, чтобы приступить играть за настоящие средства.
Выберите Игру: Начните с простых состязаний и постепенно переходите к более сложным разновидностям.
Развивайтесь: Изучайте свои ошибки, изучайте тактики и тактики игры, чтобы повысить собственное умение.
Не упустите свой шанс попробовать удачу и сделаться экспертом игры в покер! Присоединяйтесь к нам и начните участвовать в онлайн игру в покер на реальные деньги прямо в настоящее время. Выбирайте самые лучшие покер-румы и наслаждайтесь игрой в каждое время!
Увлекательный Сфера Виртуального Игры в Покер: Участвуйте за Реальные Деньги и Получайте Удовольствие от Лучшими Покерными Комнатами
Ли любите покер? Чувствуете азарт, когда карты выпадают благоприятно, и стратегии ведут к выигрышу? Тогда добро пожаловать внутрь сферу онлайн игры в покер, в котором волнение и возможность играть за реальные деньги доступны в любое каждое время и в любом каком угодно локации. Забудьте о нужде ехать в казино либо приглашать товарищей – теперь все наилучшие покерные столы при тебя под рукой!
Почему Виртуальный Игра в Покер – Является Идеальный Выбор?
Удобство и Доступность: Участвуйте в любимый игру в покер, не покидая из жилища. Нужен всего лишь ПК или сотовый и подключение к сети.
Большой Ассортимент Состязаний: Начиная с традиционного Техас Холдем до Омаха и остальных необычных видов – любой найдет состязание по вкусу и по уровню умения.
Игра за Реальные Деньги: Почувствуйте подлинный волнение, конкурируя с игроками со всего света. Зарабатывайте настоящие деньги, оттачивая свое умение.
Гибкость Ставок: Участвуйте на любом лимите, какой вам комфортен. От малых ставок для начинающих до крупных вложений для экспертов.
Поощрения и Предложения: Принимайте щедрые поощрения за запись и вклады, участвуйте в регулярных предложениях и турнирах с крупными наградными фондами.
Играйте в Лучшие Покер-Румы и Наслаждайтесь Надежностью
Играть в покер онлайн выбрав правильный покер-рум – значимый шаг для удобной и честной игры. Наша команда предлагаем тебе участвовать в лучшие покерные комнаты, подтвержденные временем и множеством участников. Данные площадки гарантируют:
Защищенность и Безотказность: Лицензированные покер-румы используют новейшие методы кодирования, гарантируя безопасность твоих сведений и средств.
Справедливость Участия: ГСЧ (генераторы случайных значений) обеспечивают произвольное появление карт, обеспечивая справедливость и непредсказуемость любой раздачи.
Удобный Интерфейс: Легко усваиваемый оформление и легкая ориентация сделают твою игру удобной и радостной.
Большой Ассортимент Соревнований: Присоединяйтесь в регулярных состязаниях, многостольных соревнованиях (МТТ), Sit-and-Go и прочих захватывающих форматах.
Квалифицированная Поддержка: Служба помощи постоянно способна оказать помощь тебе с любыми вопросами, появляющимися в ходе игры.
Каким Образом Приступить Участвовать в Виртуальный Игру в Покер за Настоящие Средства?
Определите Безотказный Покерную Комнату: Изучите рейтинги и мнения, чтобы выбрать платформу, которая соответствует твоим запросам.
Зарегистрируйтесь: Порядок записи обычно требует пару минут.
Внесите Депозит: Положите свой баланс удобным для тебя вариантом, чтобы приступить участвовать за реальные средства.
Подберите Состязание: Начните с легких игр и помаленьку двигайтесь к более трудным вариантам.
Совершенствуйтесь: Анализируйте свои промахи, изучайте стратегии и приемы участия, чтобы улучшить собственное мастерство.
Не упустите свой возможность попробовать везение и сделаться экспертом игры в покер! Присоединяйтесь к нам и начните участвовать в онлайн игру в покер за реальные деньги прямо в настоящее время. Выбирайте самые лучшие покерные комнаты и радуйтесь игрой в каждое мгновение!
Увлекательный Сфера Онлайн Покера: Играйте за Реальные Деньги и Получайте Удовольствие от Лучшими Покер-Румами
Ли обожаете покер? Чувствуете азарт, в случае если карты ложатся удачно, и стратегии приводят к победе победе? В таком случае милости просим внутрь мир виртуального игры в покер, в котором адреналин и возможность участвовать за реальные средства открыты в любое любое мгновение и в любом локации. Забудьте о нужде добираться в игровой дом или собирать товарищей – сейчас все лучшие покер столы при вас под рукой!
Почему Онлайн Игра в Покер – Является Прекрасный Выбор?
Удобство и Доступность: Участвуйте в обожаемый покер, не покидая из жилища. Нужен только компьютер или смартфон и подключение к интернету.
Огромный Ассортимент Игр: От классического Техас Холдема до Омаха и остальных необычных разновидностей – любой найдет состязание по вкусу и по уровню умения.
Участие на Реальные Средства: Почувствуйте подлинный волнение, конкурируя с участниками со всего света. Выигрывайте настоящие деньги, совершенствуя собственное мастерство.
Эластичность Ставок: Участвуйте на каком угодно ставке, который тебе удобен. Начиная с малых ставок для новичков заканчивая высоких вложений для экспертов.
Поощрения и Предложения: Получайте большие бонусы за запись и вклады, присоединяйтесь к постоянных предложениях и турнирах с большими призовыми суммами.
Участвуйте в Самые Лучшие Покерные Комнаты и Наслаждайтесь Качеством
Покер онлайн выбрав правильный покер-рум – важный этап для удобной и честной игры. Наша команда предлагаем тебе участвовать в лучшие покер-румы, подтвержденные годами и множеством участников. Данные платформы гарантируют:
Защищенность и Безотказность: Официальные покерные комнаты используют современные методы кодирования, обеспечивая безопасность твоих данных и денег.
Честность Игры: ГСЧ (генераторы случайных значений) обеспечивают произвольное появление карт, гарантируя честность и непредсказуемость любой раздачи.
Удобный Оформление: Легко понятный оформление и простая ориентация сделают вашу участие комфортной и радостной.
Большой Выбор Турниров: Участвуйте в ежедневных состязаниях, многостоловых турнирах (МТТ), Sit-and-Go и прочих захватывающих разновидностях.
Профессиональная Поддержка: Сервис помощи постоянно готова оказать помощь вам с какими угодно вопросами, возникающими в процессе игры.
Каким Образом Приступить Играть в Виртуальный Покер за Реальные Деньги?
Определите Надежный Покерную Комнату: Ознакомьтесь с рейтинги и мнения, чтобы выбрать площадку, которая отвечает твоим запросам.
Зарегистрируйтесь: Процесс регистрации как правило требует несколько мгновений.
Внесите Депозит: Положите свой счет удобным для тебя способом, чтобы приступить играть за настоящие средства.
Выберите Состязание: Начните с простых состязаний и помаленьку двигайтесь к более сложным вариантам.
Развивайтесь: Изучайте свои промахи, изучайте стратегии и тактики игры, чтобы повысить собственное мастерство.
Не упустите свой шанс испытать везение и сделаться мастером покера! Присоединяйтесь к нам и начните играть в виртуальный игру в покер за реальные деньги прямо сегодня. Выбирайте лучшие покерные комнаты и наслаждайтесь игрой в каждое мгновение!
Увлекательный Сфера Онлайн Игры в Покер: Участвуйте за Настоящие Средства и Наслаждайтесь Самыми Лучшими Покер-Румами
Вы любите покер? Чувствуете восторг, в случае если карты выпадают благоприятно, и тактики ведут к победе? Тогда добро просим внутрь сферу виртуального покера, где волнение и шанс играть за реальные деньги доступны в любое мгновение и в любом каком угодно локации. Оставьте за нужде добираться в игровой дом либо собирать товарищей – сейчас абсолютно все наилучшие покерные места при вас у близко!
Почему Онлайн Покер – Является Прекрасный Решение?
Удобство и Доступность: Участвуйте в любимый игру в покер, не выходя из дома. Нужен только ПК или смартфон и доступ к сети.
Большой Ассортимент Игр: Начиная с классического Техас Холдема заканчивая Омаха и других необычных разновидностей – каждый найдет игру по вкусу и по степени мастерства.
Участие за Реальные Деньги: Испытайте настоящий азарт, соревнуясь против игроками со всего света. Зарабатывайте реальные средства, совершенствуя собственное мастерство.
Гибкость Ставок: Играйте за любом ставке, какой тебе удобен. От микролимитов для новичков до крупных ставок для экспертов.
Поощрения и Предложения: Получайте щедрые поощрения за регистрацию и вклады, участвуйте к постоянных предложениях и турнирах с большими наградными суммами.
Играйте в Лучшие Покер-Румы и Наслаждайтесь Качеством
Играть в покер онлайн выбрав правильный покер-рум – важный этап для комфортной и честной игры. Наша команда советуем вам играть в самые лучшие покер-румы, проверенные годами и тысячами игроков. Эти платформы обеспечивают:
Безопасность и Безотказность: Официальные покер-румы используют современные технологии кодирования, гарантируя защиту ваших сведений и денег.
Справедливость Участия: ГСЧ (генераторы непредвиденных значений) гарантируют произвольное выпадение карт, обеспечивая честность и неопределенность каждой раздачи.
Удобный Интерфейс: Легко понятный интерфейс и простая навигация сделают твою игру удобной и приятной.
Большой Выбор Турниров: Участвуйте в регулярных состязаниях, многостоловых соревнованиях (МТТ), Sit-and-Go и прочих увлекательных форматах.
Квалифицированная Поддержка: Сервис помощи всегда готова оказать помощь тебе в любыми вопросами, появляющимися в процессе участия.
Каким Образом Начать Играть в Виртуальный Игру в Покер за Настоящие Деньги?
Выберите Надежный Покерную Комнату: Изучите оценки и отзывы, чтобы выбрать площадку, которая соответствует твоим запросам.
Зарегистрируйтесь: Порядок записи обычно занимает пару минут.
Внесите Вклад: Пополните свой счет удобным для тебя вариантом, чтобы приступить участвовать за реальные средства.
Выберите Состязание: Стартуйте с легких игр и постепенно переходите к более трудным вариантам.
Развивайтесь: Изучайте свои ошибки, осваивайте тактики и приемы игры, чтобы улучшить собственное умение.
Не упустите свой шанс испытать удачу и сделаться экспертом покера! Присоединяйтесь к нам и приступайте участвовать в онлайн покер за настоящие средства уже сегодня. Подбирайте самые лучшие покер-румы и наслаждайтесь участием в каждое мгновение!
Захватывающий Мир Онлайн Покера: Играйте за Реальные Средства и Получайте Удовольствие от Самыми Лучшими Покерными Комнатами
Вы любите покер? Испытываете восторг, в случае если карты ложатся благоприятно, и тактики ведут к победе? В таком случае добро просим в сферу виртуального игры в покер, где волнение и шанс играть на реальные деньги доступны в любое мгновение и в любом каком угодно месте. Оставьте за необходимости добираться в казино либо приглашать товарищей – сейчас все наилучшие покер столы у тебя под рукой!
Отчего Виртуальный Игра в Покер – Является Идеальный Выбор?
Удобство и Открытость: Играйте в обожаемый игру в покер, не покидая из дома. Нужен только компьютер либо сотовый и подключение к сети.
Большой Выбор Игр: От классического Техасского Холдема заканчивая Омаха и остальных необычных разновидностей – каждый отыщет состязание по душе и по уровню мастерства.
Игра на Настоящие Деньги: Почувствуйте настоящий азарт, соревнуясь с игроками со всего света. Выигрывайте настоящие деньги, оттачивая свое умение.
Гибкость Вложений: Участвуйте на каком угодно ставке, какой вам комфортен. Начиная с микролимитов для начинающих до высоких ставок для профессионалов.
Бонусы и Предложения: Принимайте большие бонусы за регистрацию и депозиты, участвуйте к регулярных предложениях и турнирах с крупными призовыми суммами.
Играйте в Лучшие Покер-Румы и Радуйтесь Качеством
Играть в покер онлайн выбрав правильный покер-рум – значимый шаг для комфортной и справедливой участия. Мы советуем вам участвовать в самые лучшие покер-румы, проверенные временем и тысячами игроков. Эти площадки обеспечивают:
Защищенность и Безотказность: Лицензированные покерные комнаты используют новейшие методы кодирования, гарантируя защиту ваших данных и денег.
Справедливость Участия: ГСЧ (генераторы непредвиденных значений) гарантируют произвольное выпадение карт, обеспечивая справедливость и неопределенность каждой раздачи.
Удобный Интерфейс: Интуитивно усваиваемый оформление и простая ориентация сделают твою участие удобной и радостной.
Обширный Выбор Соревнований: Присоединяйтесь в регулярных турнирах, многостоловых соревнованиях (МТТ), Sit-and-Go и других захватывающих форматах.
Квалифицированная Помощь: Служба поддержки всегда готова помочь тебе с любыми вопросами, появляющимися в ходе игры.
Как Начать Играть в Виртуальный Игру в Покер на Настоящие Деньги?
Выберите Надежный Покерную Комнату: Изучите оценки и мнения, чтобы подобрать платформу, которая отвечает вашим запросам.
Зарегистрируйтесь: Порядок регистрации как правило занимает пару минут.
Внесите Депозит: Пополните свой счет подходящим для тебя вариантом, чтобы приступить играть за настоящие деньги.
Подберите Состязание: Начните с простых игр и помаленьку переходите к более сложным вариантам.
Совершенствуйтесь: Анализируйте свои ошибки, осваивайте стратегии и приемы участия, чтобы улучшить собственное умение.
Не упустите свой возможность попробовать удачу и стать экспертом покера! Присоединяйтесь к нам и начните играть в онлайн покер на реальные средства уже в настоящее время. Выбирайте лучшие покерные комнаты и радуйтесь игрой в любое время!
Захватывающий Сфера Виртуального Покера: Играйте на Настоящие Деньги и Получайте Удовольствие от Самыми Лучшими Покер-Румами
Вы любите карточную игру? Испытываете восторг, в случае если карточки выпадают благоприятно, и тактики ведут к выигрышу? Тогда милости пожаловать внутрь мир виртуального игры в покер, в котором волнение и шанс играть за настоящие средства доступны в любое время и в любом любом месте. Оставьте за нужде добираться в казино либо собирать друзей – сейчас все наилучшие покер места при тебя под рукой!
Почему Виртуальный Игра в Покер – Является Идеальный Выбор?
Удобство и Доступность: Участвуйте в любимый покер, не выходя из жилища. Нужен только компьютер или сотовый и доступ к сети.
Огромный Ассортимент Игр: От традиционного Техасского Холдем до Омахи и остальных необычных разновидностей – любой найдет состязание по душе и по уровню мастерства.
Участие за Реальные Средства: Почувствуйте подлинный волнение, конкурируя с участниками со всего света. Выигрывайте настоящие средства, совершенствуя собственное мастерство.
Гибкость Вложений: Участвуйте на любом лимите, какой тебе комфортен. Начиная с микролимитов для начинающих заканчивая высоких вложений для экспертов.
Бонусы и Акции: Получайте большие поощрения за регистрацию и депозиты, присоединяйтесь к регулярных акциях и турнирах с большими наградными фондами.
Участвуйте в Лучшие Покерные Комнаты и Радуйтесь Надежностью
Играть в покер онлайн выбрав правильный покер-рум – важный этап для удобной и справедливой игры. Мы советуем вам играть в лучшие покерные комнаты, подтвержденные годами и тысячами участников. Данные платформы обеспечивают:
Безопасность и Безотказность: Лицензированные покерные комнаты применяют новейшие технологии кодирования, гарантируя защиту твоих сведений и средств.
Честность Участия: ГСЧ (генераторы случайных значений) гарантируют случайное появление карт, гарантируя честность и неопределенность любой раздачи.
Комфортный Оформление: Интуитивно понятный интерфейс и легкая ориентация сделают твою игру комфортной и радостной.
Большой Выбор Турниров: Присоединяйтесь в ежедневных турнирах, многостольных турнирах (МТТ), Sit-and-Go и прочих захватывающих форматах.
Квалифицированная Поддержка: Сервис поддержки постоянно способна оказать помощь тебе в какими угодно запросами, возникающими в ходе участия.
Как Начать Играть в Онлайн Покер на Настоящие Средства?
Определите Безотказный Покер-Рум: Изучите рейтинги и отзывы, чтобы подобрать площадку, которая отвечает вашим запросам.
Зарегистрируйтесь: Порядок записи как правило требует пару минут.
Сделайте Вклад: Пополните свой счет подходящим для тебя способом, чтобы начать участвовать на реальные деньги.
Подберите Игру: Начните с легких игр и помаленьку двигайтесь к более трудным вариантам.
Совершенствуйтесь: Анализируйте свои промахи, осваивайте стратегии и приемы участия, чтобы улучшить свое умение.
Не упустите свой возможность попробовать везение и стать мастером игры в покер! Присоединяйтесь к нам и начните играть в онлайн покер на настоящие деньги прямо сегодня. Выбирайте самые лучшие покер-румы и радуйтесь участием в любое мгновение!
Захватывающий Мир Виртуального Покера: Участвуйте на Настоящие Средства и Наслаждайтесь Самыми Лучшими Покерными Комнатами
Вы обожаете карточную игру? Испытываете азарт, в случае если карточки ложатся благоприятно, и тактики приводят к победе? В таком случае милости просим внутрь мир онлайн покера, где адреналин и возможность участвовать за реальные деньги открыты в любое каждое время и в каком угодно локации. Забудьте о необходимости добираться в казино или приглашать товарищей – сейчас все наилучшие покер столы у вас под рукой!
Отчего Онлайн Покер – Является Прекрасный Решение?
Комфорт и Доступность: Играйте в любимый покер, не выходя из дома. Нужен всего лишь компьютер или сотовый и подключение к сети.
Большой Выбор Состязаний: От традиционного Техас Холдем до Омаха и других необычных разновидностей – каждый отыщет состязание по вкусу и по уровню мастерства.
Участие на Реальные Деньги: Почувствуйте подлинный азарт, конкурируя против участниками со всего мира. Выигрывайте реальные деньги, совершенствуя свое мастерство.
Эластичность Вложений: Участвуйте за любом лимите, какой тебе комфортен. От малых ставок для новичков заканчивая высоких ставок для профессионалов.
Бонусы и Предложения: Принимайте большие поощрения за запись и депозиты, участвуйте в постоянных акциях и соревнованиях с крупными наградными суммами.
Участвуйте в Самые Лучшие Покерные Комнаты и Наслаждайтесь Качеством
Играть в покер онлайн выбрав правильный покер-рум – важный шаг для удобной и справедливой игры. Наша команда советуем тебе участвовать в лучшие покер-румы, подтвержденные временем и множеством игроков. Эти площадки обеспечивают:
Безопасность и Безотказность: Лицензированные покер-румы применяют новейшие технологии шифрования, гарантируя безопасность ваших сведений и денег.
Справедливость Участия: ГСЧ (генераторы случайных значений) обеспечивают случайное выпадение карт, обеспечивая честность и непредсказуемость каждой раздачи.
Комфортный Оформление: Легко усваиваемый оформление и легкая навигация сделают вашу игру удобной и радостной.
Обширный Выбор Турниров: Присоединяйтесь к регулярных состязаниях, многостольных турнирах (МТТ), Sit-and-Go и других захватывающих разновидностях.
Квалифицированная Поддержка: Сервис помощи постоянно готова помочь тебе в любыми запросами, возникающими в ходе участия.
Как Начать Играть в Виртуальный Игру в Покер на Реальные Средства?
Выберите Надежный Покерную Комнату: Изучите рейтинги и мнения, чтобы выбрать платформу, которая отвечает твоим требованиям.
Зарегистрируйтесь: Процесс регистрации как правило требует пару минут.
Сделайте Вклад: Положите свой счет удобным для вас вариантом, чтобы начать участвовать за реальные деньги.
Выберите Состязание: Начните с легких состязаний и постепенно двигайтесь к более сложным разновидностям.
Совершенствуйтесь: Изучайте свои промахи, изучайте стратегии и приемы участия, чтобы повысить свое умение.
Не упустите свой возможность попробовать везение и сделаться экспертом игры в покер! Присоединяйтесь к нам и приступайте играть в виртуальный игру в покер за настоящие деньги прямо в настоящее время. Подбирайте самые лучшие покерные комнаты и радуйтесь участием в каждое мгновение!
Захватывающий Мир Онлайн Покера: Играйте за Реальные Средства и Получайте Удовольствие от Лучшими Покерными Комнатами
Ли любите покер? Испытываете азарт, когда карты выпадают удачно, и стратегии ведут к победе? В таком случае добро просим в мир виртуального покера, где адреналин и возможность участвовать за настоящие средства доступны в любое каждое время и в любом месте. Забудьте о нужде ехать в игровой дом либо собирать друзей – теперь все наилучшие покер столы у тебя под близко!
Почему Онлайн Игра в Покер – Это Идеальный Выбор?
Комфорт и Доступность: Участвуйте в обожаемый игру в покер, не покидая из жилища. Требуется всего лишь компьютер либо сотовый и подключение к интернету.
Большой Ассортимент Состязаний: Начиная с традиционного Техасского Холдема заканчивая Омаха и других необычных разновидностей – каждый отыщет состязание по вкусу и по степени умения.
Игра на Настоящие Средства: Испытайте подлинный волнение, соревнуясь против игроками со всего мира. Выигрывайте реальные деньги, совершенствуя свое мастерство.
Эластичность Вложений: Участвуйте на любом ставке, который вам удобен. От микролимитов для новичков до крупных ставок для экспертов.
Бонусы и Акции: Принимайте большие бонусы за запись и вклады, присоединяйтесь к регулярных акциях и турнирах с крупными призовыми фондами.
Играйте в Лучшие Покер-Румы и Наслаждайтесь Надежностью
Играть в покер онлайн выбрав правильный покер-рум – важный шаг для комфортной и справедливой участия. Наша команда советуем тебе играть в лучшие покерные комнаты, проверенные временем и тысячами игроков. Эти площадки гарантируют:
Защищенность и Надежность: Лицензированные покерные комнаты используют современные технологии шифрования, обеспечивая безопасность твоих данных и средств.
Честность Участия: ГСЧ (генераторы случайных значений) гарантируют случайное появление карт, обеспечивая честность и непредсказуемость любой раздачи.
Комфортный Интерфейс: Интуитивно усваиваемый интерфейс и простая навигация сделают вашу участие удобной и радостной.
Обширный Ассортимент Турниров: Присоединяйтесь к регулярных турнирах, многостоловых соревнованиях (МТТ), Sit-and-Go и прочих захватывающих форматах.
Квалифицированная Помощь: Сервис поддержки всегда готова помочь вам с какими угодно запросами, возникающими в ходе участия.
Каким Образом Начать Играть в Онлайн Покер за Настоящие Деньги?
Выберите Безотказный Покер-Рум: Ознакомьтесь с рейтинги и отзывы, чтобы выбрать платформу, которая отвечает вашим запросам.
Зарегистрируйтесь: Порядок регистрации обычно занимает несколько мгновений.
Внесите Депозит: Положите свой баланс удобным для тебя вариантом, чтобы приступить участвовать на реальные деньги.
Подберите Состязание: Стартуйте с легких игр и помаленьку переходите к более сложным разновидностям.
Совершенствуйтесь: Изучайте свои промахи, изучайте стратегии и приемы участия, чтобы повысить свое умение.
Не упустите свой шанс испытать везение и стать мастером игры в покер! Присоединяйтесь к нам и начните участвовать в виртуальный игру в покер на настоящие средства уже в настоящее время. Подбирайте самые лучшие покерные комнаты и наслаждайтесь игрой в любое мгновение!
Увлекательный Мир Онлайн Покера: Играйте на Настоящие Средства и Получайте Удовольствие от Лучшими Покерными Комнатами
Ли любите покер? Испытываете азарт, в случае если карточки выпадают удачно, и тактики ведут к победе выигрышу? Тогда милости просим в сферу онлайн покера, в котором адреналин и возможность участвовать за реальные деньги открыты в каждое мгновение и в любом любом локации. Забудьте о нужде добираться в игровой дом или собирать товарищей – сейчас все наилучшие покер места при вас у близко!
Почему Онлайн Покер – Является Прекрасный Решение?
Удобство и Доступность: Играйте в обожаемый игру в покер, не выходя из жилища. Нужен только ПК либо сотовый и доступ к интернету.
Огромный Ассортимент Состязаний: От классического Техас Холдем до Омахи и других необычных видов – любой отыщет игру по вкусу и по степени умения.
Участие на Настоящие Деньги: Почувствуйте настоящий азарт, соревнуясь против игроками со всего мира. Выигрывайте настоящие средства, совершенствуя собственное умение.
Гибкость Вложений: Участвуйте на любом ставке, какой тебе удобен. От микролимитов для новичков до высоких ставок для профессионалов.
Бонусы и Предложения: Принимайте щедрые бонусы за регистрацию и вклады, участвуйте к регулярных акциях и соревнованиях с большими призовыми фондами.
Участвуйте в Лучшие Покерные Комнаты и Наслаждайтесь Надежностью
Покер онлайн выбрав правильный покер-рум – важный этап для удобной и справедливой игры. Мы советуем тебе участвовать в самые лучшие покерные комнаты, проверенные годами и множеством участников. Эти платформы обеспечивают:
Безопасность и Безотказность: Официальные покер-румы используют современные методы шифрования, обеспечивая безопасность твоих данных и денег.
Справедливость Участия: ГСЧ (генераторы непредвиденных значений) обеспечивают случайное появление карт, гарантируя справедливость и неопределенность любой раздачи.
Удобный Интерфейс: Интуитивно понятный оформление и легкая ориентация обеспечат вашу игру удобной и приятной.
Большой Выбор Соревнований: Участвуйте в ежедневных турнирах, многостольных соревнованиях (МТТ), Sit-and-Go и других увлекательных разновидностях.
Профессиональная Поддержка: Служба помощи постоянно готова помочь вам с какими угодно вопросами, появляющимися в процессе участия.
Как Приступить Участвовать в Виртуальный Покер за Настоящие Средства?
Выберите Безотказный Покер-Рум: Ознакомьтесь с оценки и мнения, чтобы выбрать площадку, которая соответствует твоим требованиям.
Запишитесь: Порядок регистрации как правило занимает несколько мгновений.
Сделайте Депозит: Положите свой баланс удобным для вас вариантом, чтобы приступить участвовать за реальные деньги.
Выберите Игру: Стартуйте с простых игр и постепенно переходите к более сложным вариантам.
Совершенствуйтесь: Анализируйте свои ошибки, осваивайте тактики и тактики участия, чтобы повысить свое мастерство.
Не упустите свой шанс попробовать удачу и стать мастером игры в покер! Присоединяйтесь к нам и приступайте участвовать в виртуальный игру в покер на настоящие средства уже сегодня. Выбирайте самые лучшие покерные комнаты и наслаждайтесь участием в любое мгновение!
Увлекательный Сфера Виртуального Покера: Участвуйте на Настоящие Средства и Получайте Удовольствие от Лучшими Покер-Румами
Ли любите карточную игру? Испытываете восторг, в случае если карты ложатся удачно, и стратегии приводят к победе выигрышу? Тогда милости просим внутрь мир онлайн покера, где волнение и шанс играть за реальные средства доступны в каждое время и в любом локации. Оставьте о необходимости ехать в казино либо приглашать друзей – теперь все наилучшие покер столы при тебя под рукой!
Отчего Онлайн Игра в Покер – Это Прекрасный Выбор?
Удобство и Доступность: Играйте в обожаемый игру в покер, не выходя из дома. Требуется всего лишь компьютер либо смартфон и доступ к интернету.
Огромный Выбор Игр: Начиная с традиционного Техасского Холдема заканчивая Омаха и других необычных разновидностей – любой найдет состязание по душе и по уровню мастерства.
Игра за Настоящие Средства: Испытайте подлинный волнение, конкурируя с игроками со всего света. Зарабатывайте реальные средства, оттачивая свое умение.
Гибкость Ставок: Участвуйте на каком угодно ставке, который тебе комфортен. Начиная с малых ставок для начинающих до высоких ставок для профессионалов.
Поощрения и Предложения: Принимайте большие поощрения за запись и депозиты, участвуйте в постоянных предложениях и турнирах с большими призовыми фондами.
Играйте в Лучшие Покерные Комнаты и Радуйтесь Качеством
Покер онлайн выбрав правильный покер-рум – важный этап для удобной и справедливой участия. Мы предлагаем вам участвовать в самые лучшие покер-румы, подтвержденные временем и тысячами игроков. Эти площадки обеспечивают:
Защищенность и Надежность: Официальные покер-румы используют современные технологии кодирования, гарантируя безопасность твоих сведений и средств.
Честность Игры: ГСЧ (генераторы непредвиденных значений) обеспечивают произвольное появление карт, гарантируя справедливость и непредсказуемость каждой раздачи.
Комфортный Интерфейс: Интуитивно понятный интерфейс и простая ориентация сделают твою участие комфортной и приятной.
Большой Выбор Соревнований: Присоединяйтесь в ежедневных состязаниях, многостоловых турнирах (МТТ), Sit-and-Go и других захватывающих форматах.
Квалифицированная Помощь: Служба помощи постоянно готова оказать помощь вам с любыми вопросами, возникающими в процессе игры.
Каким Образом Начать Играть в Виртуальный Покер на Настоящие Средства?
Определите Безотказный Покер-Рум: Изучите оценки и мнения, чтобы выбрать площадку, которая отвечает вашим требованиям.
Зарегистрируйтесь: Процесс регистрации как правило требует пару мгновений.
Сделайте Депозит: Пополните свой счет подходящим для тебя способом, чтобы приступить участвовать на реальные средства.
Подберите Игру: Начните с легких состязаний и помаленьку переходите к более трудным разновидностям.
Совершенствуйтесь: Анализируйте свои промахи, осваивайте тактики и приемы участия, чтобы улучшить собственное умение.
Не упустите свой шанс испытать удачу и сделаться мастером покера! Присоединяйтесь к нам и начните участвовать в виртуальный игру в покер на реальные средства прямо сегодня. Подбирайте самые лучшие покер-румы и наслаждайтесь участием в каждое время!
Захватывающий Мир Онлайн Покера: Играйте на Реальные Средства и Получайте Удовольствие от Самыми Лучшими Покер-Румами
Вы обожаете покер? Чувствуете азарт, когда карты ложатся благоприятно, и стратегии ведут к победе? Тогда добро просим внутрь сферу виртуального игры в покер, где адреналин и шанс играть на настоящие деньги доступны в любое любое мгновение и в любом локации. Оставьте за необходимости ехать в казино или собирать товарищей – теперь абсолютно все наилучшие покер места при вас под близко!
Почему Онлайн Игра в Покер – Это Идеальный Выбор?
Удобство и Доступность: Участвуйте в любимый покер, не выходя из дома. Требуется всего лишь ПК либо смартфон и подключение к сети.
Большой Выбор Состязаний: Начиная с традиционного Техас Холдема заканчивая Омахи и других экзотических видов – каждый отыщет состязание по вкусу и по степени мастерства.
Участие за Реальные Деньги: Испытайте настоящий волнение, конкурируя с участниками со всего света. Зарабатывайте настоящие средства, оттачивая свое умение.
Эластичность Ставок: Участвуйте на каком угодно ставке, какой вам комфортен. Начиная с микролимитов для начинающих заканчивая высоких вложений для экспертов.
Поощрения и Акции: Получайте большие поощрения за регистрацию и вклады, участвуйте к постоянных предложениях и турнирах с большими призовыми фондами.
Участвуйте в Самые Лучшие Покер-Румы и Наслаждайтесь Надежностью
Играть в покер онлайн выбрав правильный покер-рум – значимый шаг для удобной и справедливой игры. Мы предлагаем вам участвовать в самые лучшие покер-румы, подтвержденные временем и тысячами игроков. Эти площадки обеспечивают:
Безопасность и Безотказность: Официальные покер-румы применяют новейшие методы шифрования, обеспечивая безопасность твоих сведений и средств.
Справедливость Участия: ГСЧ (генераторы случайных чисел) обеспечивают случайное появление карт, гарантируя справедливость и непредсказуемость любой раздачи.
Удобный Оформление: Интуитивно понятный оформление и простая ориентация обеспечат твою участие комфортной и приятной.
Обширный Ассортимент Соревнований: Участвуйте к ежедневных состязаниях, многостоловых турнирах (МТТ), Sit-and-Go и других увлекательных разновидностях.
Квалифицированная Поддержка: Служба поддержки постоянно готова оказать помощь тебе с какими угодно вопросами, появляющимися в ходе игры.
Каким Образом Приступить Участвовать в Онлайн Игру в Покер на Реальные Средства?
Выберите Безотказный Покерную Комнату: Изучите рейтинги и отзывы, чтобы выбрать площадку, которая соответствует вашим требованиям.
Запишитесь: Процесс регистрации обычно требует несколько минут.
Сделайте Депозит: Положите свой счет удобным для тебя вариантом, чтобы приступить участвовать за реальные деньги.
Выберите Состязание: Начните с простых игр и помаленьку двигайтесь к более трудным вариантам.
Развивайтесь: Изучайте свои промахи, изучайте тактики и приемы игры, чтобы улучшить свое мастерство.
Не упустите свой возможность испытать удачу и сделаться экспертом покера! Присоединяйтесь к нам и приступайте играть в онлайн покер за реальные средства уже сегодня. Подбирайте лучшие покерные комнаты и радуйтесь участием в каждое мгновение!
Захватывающий Мир Виртуального Игры в Покер: Играйте на Настоящие Деньги и Наслаждайтесь Самыми Лучшими Покер-Румами
Вы любите карточную игру? Испытываете азарт, когда карточки выпадают благоприятно, и тактики ведут к победе выигрышу? Тогда добро пожаловать в мир виртуального покера, где адреналин и возможность участвовать на настоящие деньги доступны в любое любое мгновение и в любом каком угодно месте. Оставьте за необходимости ехать в игровой дом либо приглашать товарищей – теперь все лучшие покерные места у тебя у рукой!
Почему Онлайн Игра в Покер – Это Прекрасный Выбор?
Удобство и Открытость: Играйте в любимый игру в покер, не покидая из жилища. Нужен всего лишь ПК либо сотовый и доступ к сети.
Большой Выбор Игр: От традиционного Техас Холдема до Омаха и других экзотических видов – любой найдет игру по душе и по уровню умения.
Игра за Настоящие Деньги: Почувствуйте подлинный волнение, соревнуясь против игроками со всего мира. Выигрывайте настоящие деньги, совершенствуя собственное мастерство.
Гибкость Ставок: Участвуйте за любом лимите, который тебе удобен. Начиная с малых ставок для начинающих заканчивая крупных ставок для профессионалов.
Бонусы и Акции: Принимайте щедрые бонусы за запись и депозиты, присоединяйтесь в регулярных предложениях и соревнованиях с большими наградными суммами.
Играйте в Лучшие Покер-Румы и Наслаждайтесь Качеством
Покер онлайн выбрав правильный покер-рум – значимый шаг для комфортной и справедливой участия. Наша команда советуем вам играть в самые лучшие покерные комнаты, подтвержденные годами и тысячами участников. Данные площадки гарантируют:
Безопасность и Безотказность: Лицензированные покер-румы применяют современные технологии шифрования, обеспечивая безопасность твоих сведений и средств.
Справедливость Игры: ГСЧ (генераторы случайных чисел) гарантируют случайное появление карт, гарантируя справедливость и неопределенность любой раздачи.
Комфортный Интерфейс: Легко усваиваемый оформление и простая навигация сделают твою игру удобной и приятной.
Обширный Ассортимент Турниров: Присоединяйтесь к ежедневных турнирах, многостоловых турнирах (МТТ), Sit-and-Go и других увлекательных форматах.
Профессиональная Помощь: Сервис помощи всегда готова помочь вам в какими угодно вопросами, возникающими в процессе игры.
Как Начать Участвовать в Онлайн Покер на Реальные Средства?
Выберите Надежный Покерную Комнату: Ознакомьтесь с оценки и отзывы, чтобы подобрать площадку, которая соответствует твоим требованиям.
Зарегистрируйтесь: Порядок регистрации как правило занимает несколько мгновений.
Внесите Депозит: Пополните свой баланс подходящим для тебя способом, чтобы приступить участвовать за настоящие деньги.
Подберите Игру: Стартуйте с легких состязаний и помаленьку двигайтесь к более трудным вариантам.
Развивайтесь: Анализируйте свои ошибки, изучайте стратегии и приемы игры, чтобы улучшить собственное мастерство.
Не упустите свой возможность испытать везение и стать экспертом игры в покер! Присоединяйтесь к нам и приступайте участвовать в онлайн игру в покер за реальные деньги уже сегодня. Подбирайте самые лучшие покерные комнаты и радуйтесь участием в любое время!
Увлекательный Мир Виртуального Покера: Играйте на Настоящие Деньги и Получайте Удовольствие от Лучшими Покер-Румами
Ли обожаете карточную игру? Испытываете азарт, в случае если карты выпадают удачно, и тактики приводят к победе выигрышу? Тогда милости пожаловать внутрь мир виртуального игры в покер, где адреналин и возможность участвовать за настоящие средства открыты в любое любое мгновение и в любом каком угодно месте. Оставьте о нужде добираться в игровой дом или приглашать товарищей – теперь абсолютно все наилучшие покерные столы при тебя у рукой!
Почему Виртуальный Игра в Покер – Это Прекрасный Решение?
Комфорт и Доступность: Играйте в обожаемый покер, не выходя из дома. Требуется только компьютер или смартфон и подключение к сети.
Огромный Выбор Игр: Начиная с традиционного Техасского Холдем заканчивая Омаха и других необычных разновидностей – каждый найдет состязание по душе и по степени мастерства.
Участие за Настоящие Средства: Испытайте настоящий азарт, конкурируя против игроками со всего мира. Зарабатывайте настоящие деньги, оттачивая собственное умение.
Эластичность Вложений: Играйте на любом ставке, какой тебе комфортен. Начиная с микролимитов для начинающих заканчивая высоких вложений для экспертов.
Бонусы и Акции: Принимайте щедрые поощрения за запись и вклады, присоединяйтесь в регулярных акциях и турнирах с крупными наградными суммами.
Участвуйте в Лучшие Покерные Комнаты и Радуйтесь Надежностью
Играть в покер онлайн выбрав правильный покер-рум – важный этап для удобной и справедливой участия. Мы предлагаем тебе играть в самые лучшие покер-румы, проверенные временем и множеством участников. Эти площадки обеспечивают:
Безопасность и Надежность: Официальные покер-румы используют современные методы шифрования, гарантируя защиту ваших сведений и средств.
Справедливость Участия: ГСЧ (генераторы непредвиденных значений) обеспечивают произвольное появление карт, обеспечивая честность и непредсказуемость любой раздачи.
Комфортный Интерфейс: Интуитивно усваиваемый интерфейс и легкая навигация обеспечат твою участие комфортной и приятной.
Большой Ассортимент Соревнований: Участвуйте к регулярных состязаниях, многостольных турнирах (МТТ), Sit-and-Go и других захватывающих разновидностях.
Квалифицированная Поддержка: Служба помощи всегда готова помочь вам с любыми вопросами, возникающими в процессе игры.
Каким Образом Начать Играть в Онлайн Игру в Покер на Настоящие Деньги?
Выберите Безотказный Покерную Комнату: Ознакомьтесь с рейтинги и отзывы, чтобы выбрать площадку, которая соответствует вашим запросам.
Запишитесь: Порядок записи обычно требует несколько мгновений.
Сделайте Вклад: Положите свой баланс подходящим для тебя вариантом, чтобы начать играть на настоящие средства.
Подберите Игру: Стартуйте с легких игр и помаленьку двигайтесь к более сложным вариантам.
Совершенствуйтесь: Анализируйте свои промахи, изучайте стратегии и приемы участия, чтобы улучшить собственное умение.
Не упустите свой возможность испытать везение и стать экспертом покера! Присоединяйтесь к нам и приступайте участвовать в онлайн покер на реальные средства уже сегодня. Подбирайте самые лучшие покер-румы и наслаждайтесь игрой в любое время!
Увлекательный Мир Виртуального Покера: Играйте на Реальные Деньги и Получайте Удовольствие от Самыми Лучшими Покерными Комнатами
Вы любите покер? Чувствуете восторг, в случае если карточки выпадают благоприятно, и тактики приводят к победе победе? Тогда милости просим внутрь сферу онлайн игры в покер, где адреналин и возможность играть за реальные деньги открыты в любое каждое время и в каком угодно локации. Забудьте за нужде ехать в игровой дом либо собирать друзей – сейчас абсолютно все наилучшие покер столы при тебя у близко!
Отчего Виртуальный Игра в Покер – Это Прекрасный Решение?
Удобство и Открытость: Играйте в любимый игру в покер, не покидая из жилища. Требуется всего лишь компьютер или сотовый и доступ к интернету.
Огромный Выбор Состязаний: Начиная с традиционного Техас Холдем до Омаха и других экзотических разновидностей – каждый отыщет игру по вкусу и по уровню умения.
Игра на Настоящие Средства: Почувствуйте подлинный волнение, соревнуясь против игроками со всего света. Зарабатывайте настоящие средства, совершенствуя свое мастерство.
Эластичность Вложений: Участвуйте на каком угодно ставке, который тебе удобен. От малых ставок для новичков до высоких ставок для экспертов.
Поощрения и Предложения: Получайте щедрые бонусы за запись и вклады, присоединяйтесь в постоянных предложениях и турнирах с крупными призовыми фондами.
Участвуйте в Лучшие Покерные Комнаты и Наслаждайтесь Качеством
Покер онлайн выбрав правильный покер-рум – значимый этап для удобной и справедливой игры. Мы предлагаем вам участвовать в лучшие покерные комнаты, подтвержденные годами и тысячами игроков. Эти платформы обеспечивают:
Безопасность и Безотказность: Официальные покерные комнаты применяют новейшие методы кодирования, обеспечивая защиту твоих данных и средств.
Честность Участия: ГСЧ (генераторы непредвиденных чисел) обеспечивают произвольное появление карт, обеспечивая справедливость и непредсказуемость любой раздачи.
Комфортный Оформление: Легко понятный оформление и простая навигация обеспечат твою участие удобной и радостной.
Обширный Ассортимент Соревнований: Участвуйте к регулярных турнирах, многостоловых турнирах (МТТ), Sit-and-Go и других захватывающих разновидностях.
Квалифицированная Поддержка: Служба поддержки постоянно готова оказать помощь вам с какими угодно запросами, возникающими в ходе участия.
Каким Образом Начать Участвовать в Виртуальный Игру в Покер за Настоящие Средства?
Выберите Надежный Покер-Рум: Изучите рейтинги и отзывы, чтобы выбрать платформу, которая отвечает твоим требованиям.
Зарегистрируйтесь: Порядок записи обычно занимает пару мгновений.
Сделайте Депозит: Положите свой счет удобным для вас способом, чтобы начать участвовать за реальные средства.
Выберите Игру: Стартуйте с легких игр и постепенно двигайтесь к более трудным разновидностям.
Развивайтесь: Анализируйте свои ошибки, изучайте тактики и приемы участия, чтобы повысить свое умение.
Не упустите свой возможность испытать удачу и стать экспертом покера! Присоединяйтесь к нам и начните играть в онлайн игру в покер за настоящие деньги прямо в настоящее время. Подбирайте самые лучшие покерные комнаты и наслаждайтесь игрой в любое время!
x-poker расписание турниров
x poker для андроид
x-poker alternative
x-poker desktop
X poker rewards
х покер клуб
pppoker играть
х покер что это
X poker РїСЂРѕРјРѕРєРѕРґ
х покер скачать
РРєСЃ покер cash
X poker high roller
x poker онлайн
Спасибо за информацию.
Предлагаю вам русские фильмы – это настоящее искусство, которое нравится огромному количество зрителей по всему миру. Они предлагают уникальный взгляд на русскую культуру, историю и обычаи. Сейчас смотреть русские фильмы и сериалы онлайн стало очень просто за счет множества онлайн кинотеатров. От мелодрам до боевиков, от исторических лент до фантастики – выбор огромен. Погрузитесь в невероятные сюжеты, профессиональную актерскую работу и красивую работу оператора, наслаждаясь кинематографией из РФ не выходя из дома.
скачать melbet зеркало ios
Играй на Фактические Средства с Комфортом! Онлайн покер – является ни только игра, это целая мир волнения, тактики а шанса заработать реальные деньги, без выходя с дома. Если ты ищете где поиграть в покер в интернете, на русском языке а с выводом денег непосредственно на карту, значит ты попали нужному месту. Данная публикация – твой проводник в мир онлайн покера в россии.
Отчего Онлайн Покер настолько Известен? Покер – это классическая карточная игра, что целые столетия завлекала миллионы людей. Онлайн формат дал этой свежую жизнь, сотворив игры доступными для каждого жаждущих. Вот только несколько оснований популярности онлайн покера:
Комфорт а открытость: Играть можно в любое время и в любом месте, обладая только подключение к браузере или мобильному устройству. Широкий выбор: Покеррумы предлагают разнообразные виды покера, форматы турниров и уровни ставок. Играть с реальными людьми: Вы способен соревноваться с реальными соперниками со всего мира, а не с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это не только азарт, а и шанс наполнить свой бумажник. Социальный аспект: Можно играть с друзьями или устанавливать свежие знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует множество сайты и покеррумы, предлагающих игры в онлайн покер. Но как подобрать оптимальный среди них? Мы поможем тебе разобраться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс а поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств обязаны быть открыты в рублях. Многообразие игр и турниров: Большой выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Надежность а авторитет: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, из-за этого советуем наблюдать за свежими обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, то следует обратить внимание на покеррумы с минимальным депозитом. Данное даст возможность тебе начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Проверьте, чтобы подобранный тобой рум есть разрешенным в россии, чтобы избежать возможных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты решили с покеррумом а выбрали игру, вы способен пополнить свой счет и начать играть на деньги. Большая часть сайтов предлагают всевозможные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно занимает от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за закрытыми столами. Это отличный способ уделить время с людьми, с которыми ты знакомы, а сразиться за титул лучшего игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок и хотите вначале привыкнуть с правилами, многие сайты предлагают возможность играть в бесплатный покер. Это отличный способ поупражняться а набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Когда ты предпочитаете играть на пк, тогда большинство покеррумов предлагают клиенты для компьютеров, которые гарантируют более комфортный и стабильный игровой процесс.
Заключение: Приступайте Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть волнующая игра, которая способна предоставить тебе не просто удовольствие, но и реальные деньги. Подбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, и знайте, что главное – есть испытывать наслаждение от игры!
Не отсрочивайте на потом, начните твою игру в онлайн покер непосредственно сейчас! Окунитесь в мир тактики, азарта и возможностей! Везенья за столами!
Играй за Настоящие Деньги со Комфортом! Онлайн покер – это ни просто игра, это целая вселенная азарта, тактики а возможности выиграть реальные деньги, без покидая с дома. Когда вы ищете где поиграть на покер в интернете, на русском языке а с выводом денег непосредственно на карту, то ты попали нужному месту. Эта публикация – твой гид в мир онлайн покера в россии.
Отчего Онлайн Покер так Популярен? Покер – есть классическая карточная игра, которая целые столетия привлекала миллионы людей. Онлайн формат принес этой свежую жизнь, сотворив игры открытыми для всех желающих. Вот только несколько причин известности онлайн покера:
Комфорт а открытость: Играть возможно в всякое время а в каком угодно месте, имея лишь подключение к браузере либо мобильному устройству. Большой выбор: Покеррумы дают различные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Вы можете соревноваться с реальными соперниками со целого мира, а не с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – это ни только азарт, но и возможность пополнить свой бумажник. Социальный аспект: Возможно играть с друзьями или устанавливать свежие знакомства с людьми, разделяющих твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть большое количество сайты и покеррумы, дающих игры в онлайн покер. Однако как подобрать лучший из них? Мы окажем помощь тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс и поддержка обязаны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть доступны в рублях. Многообразие игр и турниров: Обширный выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Надежность а авторитет: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, поэтому советуем следить за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если ты новичок, тогда стоит обратить внимание на покеррумы с минимальным депозитом. Это даст возможность вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, что подобранный вами рум есть разрешенным в россии, чтобы избежать возможных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы определились с покеррумом а выбрали игру, ты можете положить свой баланс и начать играть на деньги. Большая часть сайтов предлагают всевозможные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно требует от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают шанс играть с друзьями за приватными столами. Это замечательный метод провести время с людьми, с которыми вы знакомы, а сразиться за звание лучшего игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда вы новичок и хотите вначале привыкнуть с правилами, многие сайты предлагают шанс играть в бесплатный покер. Данное отличный метод поупражняться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Когда вы выбираешь играть на пк, тогда большая часть покеррумов дают программы для компьютеров, что гарантируют более комфортный и надежный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, что может предоставить вам ни просто наслаждение, но также реальные деньги. Выбирайте надежные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со целого мира, а знайте, что главное – это получать удовольствие от игры!
Ни отсрочивайте на потом, приступайте твою игру в онлайн покер прямо сейчас! Погрузитесь в мир тактики, азарта и шансов! Везенья за столами!
Играй за Фактические Деньги с Комфортом! Онлайн покер – это не только игра, данное полная вселенная азарта, стратегии и возможности заработать реальные деньги, не выходя из дома. Если вы ищете где поиграть на покер в интернете, на русском языке а с выводом денег непосредственно на карту, то вы оказались нужному адресу. Эта статья – ваш гид в мир онлайн покера в россии.
Почему Онлайн Покер настолько Популярен? Покер – есть классическая карточная игра, которая целые столетия завлекала миллионы людей. Онлайн формат дал этой новую жизнь, сотворив игры открытыми для всех жаждущих. Вот только пара оснований известности онлайн покера:
Удобство и доступность: Играть возможно в любое время и в каком угодно месте, имея только доступ к браузере или мобильному устройству. Большой выбор: Покеррумы обеспечивают различные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Ты способен бороться с реальными соперниками со целого мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – является ни просто азарт, а и возможность пополнить твой бумажник. Социальный аспект: Возможно играть с друзьями или заводить новые знакомства с людьми, разделяющих твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Однако как подобрать оптимальный среди них? Мы поможем тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, учитывая следующие критерии:
Наличие русского языка: Интерфейс а поддержка обязаны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств обязаны быть доступны в рублях. Многообразие игр и турниров: Широкий выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Надежность а репутация: Безопасность транзакций и честность игры. Топ румов постоянно обновляется, из-за этого рекомендуем следить за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, тогда следует обратить внимание на покеррумы с минимальным депозитом. Это даст возможность тебе начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы подобранный вами рум есть разрешенным в россии, для того чтобы избежать вероятных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы определились с покеррумом и выбрали игру, ты можете положить свой баланс а начать играть на деньги. Большая часть сайтов дают различные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило требует от нескольких часов до нескольких дней, в зависимости от рума а выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают возможность играть с друзьями за приватными столами. Данное отличный способ провести время с людьми, с которыми вы знакомы, и побороться за титул оптимального игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок и хотите вначале освоиться с правилами, многие сайты предлагают шанс играть в бесплатный покер. Это прекрасный метод поупражняться и получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Когда вы предпочитаете играть на пк, то большая часть покеррумов предлагают программы для компьютеров, которые обеспечивают более удобный а надежный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это волнующая игра, которая способна принести вам не просто наслаждение, но также реальные деньги. Подбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, и помните, что главное – есть получать удовольствие от игры!
Не откладывайте на потом, начните твою игру в онлайн покер непосредственно сейчас! Окунитесь в мир стратегии, азарта а возможностей! Удачи за столами!
Действуй на Фактические Средства со Удобством! Онлайн покер – является не только игра, это вся вселенная азарта, стратегии и шанса заработать реальные деньги, не выходя с дома. Когда ты ищете где поиграть на покер в интернете, на русском языке а с выводом денег непосредственно на карту, значит ты попали по месту. Эта публикация – ваш гид в мир онлайн покера в россии.
Отчего Онлайн Покер настолько Популярен? Покер – есть традиционная карточная игра, которая целые столетия завлекала миллионы людей. Онлайн формат дал ей свежую жизнь, сотворив игры открытыми для каждого жаждущих. Здесь только несколько оснований популярности онлайн покера:
Удобство и доступность: Играть можно в всякое время а в каком угодно месте, обладая лишь доступ к браузере либо мобильному устройству. Широкий выбор: Покеррумы обеспечивают разнообразные виды покера, форматы турниров и уровни ставок. Играть с реальными людьми: Вы способен соревноваться с реальными соперниками со целого мира, а ни с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это не только азарт, а и шанс наполнить свой бумажник. Социальный аспект: Можно играть с друзьями либо заводить свежие знакомства с людьми, разделяющих ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть множество сайты и покеррумы, дающих игры в онлайн покер. Однако как выбрать оптимальный среди них? Мы окажем помощь вам сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая такие критерии:
Наличие русского языка: Интерфейс а обслуживание должны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть доступны в рублях. Разнообразие игр и турниров: Широкий выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Надежность и авторитет: Безопасность транзакций и честность игры. Топ румов регулярно обновляется, из-за этого рекомендуем наблюдать за свежими обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если ты новичок, тогда следует обратить внимание на покеррумы с минимальным депозитом. Данное даст возможность тебе начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Проверьте, чтобы выбранный вами рум является разрешенным в россии, чтобы избежать вероятных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом а выбрали игру, вы способен пополнить твой счет и начать играть на деньги. Большая часть сайтов дают всевозможные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума а подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают шанс играть с друзьями за приватными столами. Это замечательный способ уделить время с людьми, с которыми ты знакомы, а побороться за звание лучшего игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда вы новичок а хотите вначале освоиться с правилами, многие сайты предлагают возможность играть в бесплатный покер. Это отличный метод потренироваться и получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Когда вы предпочитаете играть на пк, то большая часть покеррумов предлагают программы для компьютеров, которые обеспечивают более удобный и стабильный игровой процесс.
Заключение: Начните Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это волнующая игра, которая может принести тебе не просто наслаждение, но также реальные деньги. Выбирайте проверенные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со всего мира, и знайте, что главное – это испытывать наслаждение от игры!
Ни откладывайте на потом, приступайте твою игру в онлайн покер непосредственно сейчас! Погрузитесь в мир тактики, волнения а шансов! Удачи за столами!
Начни на Реальные Деньги с Легкостью! Онлайн покер – является ни просто игра, данное целая мир азарта, тактики а шанса заработать реальные деньги, не покидая из дома. Когда ты пытаетесь где поиграть в покер в интернете, на русском языке а с выводом денег непосредственно на карту, то вы оказались по месту. Данная статья – твой гид в мир онлайн покера в россии.
Отчего Онлайн Покер настолько Популярен? Покер – это традиционная карточная игра, которая веками завлекала миллионы людей. Онлайн формат принес этой свежую жизнь, сделав игры открытыми для каждого жаждущих. Вот только несколько оснований популярности онлайн покера:
Комфорт и открытость: Играть можно в всякое время и в каком угодно месте, обладая лишь доступ к браузере либо мобильному устройству. Обширный выбор: Покеррумы обеспечивают разнообразные виды покера, варианты турниров а уровни ставок. Играть с реальными людьми: Вы способен бороться с реальными соперниками со целого мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – это не просто волнение, а и возможность наполнить твой кошелек. Социальный аспект: Можно играть с друзьями либо заводить свежие знакомства с людьми, разделяющих твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует большое количество сайты и покеррумы, дающих игры в онлайн покер. Но как подобрать оптимальный среди них? Мы окажем помощь тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, беря во внимание следующие критерии:
Наличие русского языка: Интерфейс а обслуживание должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств обязаны быть открыты в рублях. Разнообразие игр и турниров: Большой выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Безопасность а репутация: Безопасность транзакций и честность игры. Топ румов постоянно обновляется, поэтому рекомендуем следить за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Данное позволит тебе начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Проверьте, чтобы подобранный тобой рум есть разрешенным в россии, для того чтобы избежать вероятных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты решили с покеррумом а выбрали игру, ты способен пополнить твой счет а начать играть на деньги. Большая часть сайтов предлагают всевозможные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума и подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают возможность играть с друзьями за приватными столами. Данное замечательный способ провести время с людьми, с которыми вы знакомы, и сразиться за звание оптимального игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда ты новичок и хотите вначале освоиться с правилами, многие сайты предлагают шанс играть в бесплатный покер. Это прекрасный метод поупражняться и получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Когда ты выбираешь играть на пк, тогда большая часть покеррумов дают программы для компьютеров, что гарантируют более комфортный и стабильный игровой процесс.
Заключение: Приступайте Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, которая может предоставить вам ни просто удовольствие, а также реальные деньги. Выбирайте надежные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со целого мира, и знайте, что главное – есть испытывать удовольствие от игры!
Не отсрочивайте на потом, начните свою игру в онлайн покер непосредственно сейчас! Погрузитесь в мир стратегии, азарта а возможностей! Везенья за столами!
Действуй на Фактические Деньги со Комфортом! Онлайн покер – является ни только игра, это полная вселенная азарта, тактики и шанса заработать реальные деньги, не покидая из дома. Когда вы пытаетесь где поиграть в покер в интернете, на русском языке а с выводом денег прямо на карту, значит вы попали по месту. Эта публикация – твой гид в мир онлайн покера в россии.
Отчего Онлайн Покер так Известен? Покер – это классическая карточная игра, что целые столетия привлекала миллионы людей. Онлайн формат принес этой свежую жизнь, сделав игры открытыми для каждого жаждущих. Здесь лишь пара оснований популярности онлайн покера:
Комфорт а открытость: Играть можно в всякое время а в каком угодно месте, обладая лишь доступ к браузере либо мобильному устройству. Обширный выбор: Покеррумы обеспечивают различные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Ты можете соревноваться с реальными соперниками со всего мира, а не с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – это не только волнение, но также возможность наполнить свой кошелек. Социальный аспект: Возможно играть с друзьями либо заводить свежие знакомства с людьми, разделяющих ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует множество сайты и покеррумы, дающих игры в онлайн покер. Но как выбрать оптимальный среди них? Мы окажем помощь тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, беря во внимание следующие критерии:
Наличие русского языка: Интерфейс и поддержка обязаны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств должны быть доступны в рублях. Разнообразие игр и турниров: Обширный выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность и репутация: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, поэтому рекомендуем следить за свежими обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, тогда следует обратить внимание на покеррумы с минимальным депозитом. Данное даст возможность тебе начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Проверьте, чтобы подобранный тобой рум есть разрешенным в россии, для того чтобы избежать вероятных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты решили с покеррумом и выбрали игру, ты способен пополнить свой счет и начать играть на деньги. Большая часть сайтов предлагают различные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно занимает от нескольких часов до нескольких дней, в зависимости от рума и подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают шанс играть с друзьями за закрытыми столами. Данное замечательный метод уделить время с людьми, с которыми ты знакомы, а сразиться за звание лучшего игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если вы новичок и хотите вначале освоиться с правилами, многие сайты предлагают шанс играть в бесплатный покер. Это отличный способ потренироваться и набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если вы выбираешь играть на пк, тогда большая часть покеррумов предлагают программы для компьютеров, которые обеспечивают более комфортный а стабильный игровой процесс.
Заключение: Приступайте Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, которая способна предоставить вам ни просто удовольствие, а также реальные деньги. Подбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со целого мира, а помните, что главное – это испытывать удовольствие от игры!
Ни откладывайте на потом, начните свою игру в онлайн покер прямо сейчас! Окунитесь в мир стратегии, азарта и шансов! Везенья за столами!
Действуй за Фактические Деньги с Комфортом! Онлайн покер – это ни только игра, это вся мир азарта, тактики а шанса заработать реальные деньги, не выходя из квартиры. Когда вы ищете где поиграть в покер в интернете, на русском языке а с выводом денег непосредственно на карту, значит вы попали по адресу. Эта статья – ваш проводник в мир онлайн покера в россии.
Почему Онлайн Покер так Известен? Покер – это традиционная карточная игра, что целые столетия привлекала миллионы людей. Онлайн формат принес ей новую жизнь, сотворив игры доступными для всех жаждущих. Здесь лишь несколько оснований популярности онлайн покера:
Комфорт а доступность: Играть можно в любое время и в каком угодно месте, имея только подключение к браузере либо мобильному устройству. Обширный выбор: Покеррумы дают разнообразные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Ты можете бороться с реальными соперниками со целого мира, а не с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – является ни только азарт, а и шанс наполнить свой кошелек. Социальный аспект: Можно играть с друзьями или устанавливать новые знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть множество сайты и покеррумы, предлагающих игры в онлайн покер. Но каким образом выбрать оптимальный из них? Мы поможем тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая следующие критерии:
Наличие русского языка: Интерфейс а обслуживание должны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств обязаны быть открыты в рублях. Многообразие игр и турниров: Обширный выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность и авторитет: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, поэтому рекомендуем наблюдать за актуальными обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если ты новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Это позволит вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Проверьте, что подобранный вами рум есть разрешенным в россии, для того чтобы избежать вероятных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы определились с покеррумом а выбрали игру, ты можете положить твой счет и начать играть на деньги. Большинство сайтов предлагают всевозможные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают шанс играть с друзьями за закрытыми столами. Данное отличный способ провести время с людьми, с которыми ты знакомы, и побороться за звание оптимального игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда вы новичок а желаешь сначала освоиться с правилами, многие сайты предлагают возможность играть в бесплатный покер. Данное прекрасный способ потренироваться а набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Когда вы выбираешь играть на пк, то большая часть покеррумов дают программы для компьютеров, что обеспечивают намного комфортный а стабильный игровой процесс.
Заключение: Начните Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть волнующая игра, что может принести вам ни просто наслаждение, но и реальные деньги. Подбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, а знайте, что главное – есть получать наслаждение от игры!
Не откладывайте на потом, начните свою игру в онлайн покер прямо сейчас! Погрузитесь в мир тактики, азарта и шансов! Везенья за столами!
Играй за Настоящие Средства с Комфортом! Онлайн покер – это ни только игра, это целая вселенная азарта, тактики а шанса выиграть реальные деньги, без покидая из дома. Если вы пытаетесь где поиграть в покер в интернете, на русском языке и с выводом денег непосредственно на карту, то вы попали нужному месту. Эта статья – твой гид в мир онлайн покера в россии.
Почему Онлайн Покер настолько Популярен? Покер – это классическая карточная игра, что целые столетия привлекала миллионы людей. Онлайн формат принес этой свежую жизнь, сотворив игры доступными для всех желающих. Здесь только несколько оснований известности онлайн покера:
Комфорт и открытость: Играть возможно в всякое время и в каком угодно месте, имея только доступ к браузере или мобильному устройству. Обширный выбор: Покеррумы обеспечивают разнообразные виды покера, варианты турниров а уровни ставок. Играть с реальными людьми: Ты способен бороться с реальными соперниками со всего мира, а ни с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – является ни только волнение, но и шанс пополнить свой бумажник. Социальный аспект: Можно играть с друзьями либо заводить свежие знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть множество сайты и покеррумы, предлагающих игры в онлайн покер. Но каким образом подобрать лучший из них? Мы окажем помощь вам сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая такие критерии:
Наличие русского языка: Интерфейс и обслуживание обязаны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств обязаны быть доступны в рублях. Многообразие игр и турниров: Широкий выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Надежность а авторитет: Безопасность транзакций и честность игры. Топ румов регулярно обновляется, из-за этого рекомендуем наблюдать за актуальными обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Это даст возможность тебе начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, что подобранный тобой рум является допущенным в россии, чтобы избежать вероятных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом а подобрали игру, ты можете пополнить свой счет а начать играть на деньги. Большинство сайтов дают различные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило требует от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают шанс играть с друзьями за закрытыми столами. Данное отличный способ провести время с людьми, с которыми вы знакомы, а побороться за титул оптимального игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда ты новичок и желаешь вначале привыкнуть с правилами, многие сайты дают шанс играть в бесплатный покер. Это прекрасный метод поупражняться а набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если ты выбираешь играть на пк, тогда большинство покеррумов предлагают программы для компьютеров, которые гарантируют более комфортный а стабильный игровой процесс.
Заключение: Приступайте Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, что способна предоставить вам не просто удовольствие, а и реальные деньги. Подбирайте надежные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со всего мира, и помните, что главное – есть получать удовольствие от игры!
Не отсрочивайте на потом, начните твою игру в онлайн покер прямо сейчас! Окунитесь в мир тактики, азарта и шансов! Везенья за столами!
Начни на Настоящие Средства со Комфортом! Онлайн покер – является не только игра, данное вся мир азарта, стратегии а возможности выиграть реальные деньги, не выходя с дома. Когда вы пытаетесь где поиграть в покер в интернете, на русском языке и с выводом денег прямо на карту, значит ты оказались по адресу. Данная статья – твой проводник в мир онлайн покера в россии.
Отчего Онлайн Покер так Популярен? Покер – это классическая карточная игра, которая веками привлекала миллионы людей. Онлайн формат принес ей свежую жизнь, сотворив игры доступными для всех желающих. Здесь лишь несколько оснований известности онлайн покера:
Удобство и открытость: Играть можно в всякое время а в любом месте, обладая лишь подключение к браузере либо мобильному устройству. Большой выбор: Покеррумы дают разнообразные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Ты способен соревноваться с реальными соперниками со всего мира, а не с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – является ни только волнение, но и возможность пополнить свой бумажник. Социальный аспект: Можно играть с друзьями либо устанавливать свежие знакомства с людьми, разделяющих твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Однако как выбрать оптимальный из них? Мы поможем вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, учитывая следующие критерии:
Наличие русского языка: Интерфейс и поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть открыты в рублях. Многообразие игр и турниров: Широкий выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Безопасность и репутация: Безопасность транзакций и честность игры. Топ румов постоянно обновляется, поэтому рекомендуем наблюдать за свежими обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, тогда стоит обратить внимание на покеррумы с минимальным депозитом. Это даст возможность тебе начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы подобранный вами рум является допущенным в россии, чтобы избежать возможных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом и подобрали игру, вы способен положить свой баланс и начать играть на деньги. Большинство сайтов предлагают различные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило требует от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают шанс играть с друзьями за приватными столами. Данное отличный способ провести время с людьми, с которыми вы знакомы, а сразиться за титул лучшего игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда ты новичок а желаешь вначале привыкнуть с правилами, многие сайты предлагают шанс играть в бесплатный покер. Это отличный метод поупражняться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Когда вы предпочитаете играть на пк, тогда большая часть покеррумов предлагают клиенты для компьютеров, которые гарантируют намного комфортный а стабильный игровой процесс.
Заключение: Приступайте Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это захватывающая игра, которая может предоставить вам ни только удовольствие, но также реальные деньги. Выбирайте надежные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со целого мира, а помните, что главное – это получать наслаждение от игры!
Не отсрочивайте на потом, начните твою игру в онлайн покер прямо сейчас! Погрузитесь в мир стратегии, волнения а возможностей! Удачи за столами!
Играй за Реальные Средства со Удобством! Онлайн покер – является ни только игра, это целая мир азарта, тактики а возможности выиграть реальные деньги, без покидая из дома. Если вы ищете где поиграть в покер в интернете, на русском языке и с выводом денег прямо на карту, значит вы попали нужному месту. Данная статья – ваш проводник в мир онлайн покера в россии.
Почему Онлайн Покер настолько Известен? Покер – есть классическая карточная игра, которая целые столетия привлекала миллионы людей. Онлайн формат дал этой свежую жизнь, сотворив игры доступными для каждого жаждущих. Вот лишь пара оснований известности онлайн покера:
Комфорт и доступность: Играть возможно в всякое время а в любом месте, обладая лишь подключение к браузере или мобильному устройству. Широкий выбор: Покеррумы предлагают различные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Ты можете бороться с реальными соперниками со целого мира, а не с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – это не только азарт, а и шанс наполнить свой кошелек. Социальный аспект: Возможно играть с друзьями либо заводить новые знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует множество сайты и покеррумы, предлагающих игры в онлайн покер. Однако как подобрать лучший среди них? Мы окажем помощь вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, беря во внимание следующие критерии:
Наличие русского языка: Интерфейс а поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств должны быть доступны в рублях. Многообразие игр и турниров: Широкий выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Безопасность и авторитет: Безопасность транзакций а честность игры. Топ румов регулярно обновляется, из-за этого рекомендуем наблюдать за свежими обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда вы новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Данное позволит вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы выбранный тобой рум является разрешенным в россии, для того чтобы избежать возможных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом а подобрали игру, ты способен положить твой счет и начать играть на деньги. Большая часть сайтов предлагают всевозможные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают шанс играть с друзьями за закрытыми столами. Это замечательный метод провести время с людьми, с которыми ты знакомы, и сразиться за звание лучшего игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок а хотите вначале привыкнуть с правилами, многие сайты предлагают возможность играть в бесплатный покер. Данное отличный метод потренироваться и получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если вы выбираешь играть на пк, тогда большая часть покеррумов предлагают программы для компьютеров, которые гарантируют более комфортный и стабильный игровой процесс.
Заключение: Приступайте Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, которая может принести вам не только удовольствие, а также реальные деньги. Подбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, а помните, что главное – есть испытывать наслаждение от игры!
Не откладывайте на потом, приступайте твою игру в онлайн покер прямо сейчас! Окунитесь в мир тактики, волнения а возможностей! Удачи за столами!
Действуй на Фактические Деньги с Легкостью! Онлайн покер – это ни просто игра, данное целая вселенная волнения, тактики а возможности выиграть реальные деньги, без выходя с квартиры. Когда вы ищете где поиграть в покер в интернете, на русском языке и с выводом денег непосредственно на карту, то ты попали по месту. Данная публикация – твой проводник в мир онлайн покера в россии.
Отчего Онлайн Покер так Известен? Покер – это традиционная карточная игра, что целые столетия завлекала миллионы людей. Онлайн формат принес ей новую жизнь, сделав игры открытыми для всех желающих. Здесь только несколько причин известности онлайн покера:
Комфорт и открытость: Играть можно в всякое время и в каком угодно месте, имея лишь доступ к браузере либо мобильному устройству. Обширный выбор: Покеррумы предлагают различные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Вы можете бороться с реальными соперниками со целого мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – является ни просто азарт, а и шанс наполнить твой кошелек. Социальный аспект: Можно играть с друзьями либо устанавливать свежие знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует большое количество сайты и покеррумы, дающих игры в онлайн покер. Однако каким образом подобрать лучший среди них? Мы поможем вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая следующие критерии:
Наличие русского языка: Интерфейс а поддержка обязаны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств обязаны быть открыты в рублях. Разнообразие игр и турниров: Обширный выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность а авторитет: Безопасность транзакций а честность игры. Топ румов регулярно обновляется, поэтому рекомендуем следить за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, тогда стоит обратить внимание на покеррумы с минимальным депозитом. Это даст возможность вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Проверьте, чтобы подобранный вами рум есть допущенным в россии, чтобы избежать возможных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом и выбрали игру, вы можете пополнить свой баланс а начать играть на деньги. Большая часть сайтов дают всевозможные способы пополнения а вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно занимает от нескольких часов до нескольких дней, в зависимости от рума а подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают шанс играть с друзьями за закрытыми столами. Данное отличный метод провести время с людьми, с которыми ты знакомы, и сразиться за титул оптимального игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда вы новичок и хотите сначала привыкнуть с правилами, многие сайты предлагают шанс играть в бесплатный покер. Данное прекрасный метод поупражняться и получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если вы предпочитаете играть на пк, тогда большая часть покеррумов дают клиенты для компьютеров, которые гарантируют более удобный а стабильный игровой процесс.
Заключение: Начните Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть волнующая игра, которая способна принести тебе ни просто удовольствие, но и реальные деньги. Подбирайте надежные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со всего мира, а знайте, что главное – это испытывать наслаждение от игры!
Не откладывайте на потом, приступайте свою игру в онлайн покер прямо сейчас! Окунитесь в мир стратегии, азарта а шансов! Удачи за столами!
Начни за Настоящие Средства со Комфортом! Онлайн покер – это ни только игра, данное вся вселенная азарта, стратегии а возможности выиграть реальные деньги, не покидая из квартиры. Когда вы пытаетесь где поиграть на покер в интернете, на русском языке а с выводом денег прямо на карту, значит ты попали по адресу. Эта статья – ваш проводник в мир онлайн покера в россии.
Отчего Онлайн Покер так Популярен? Покер – есть традиционная карточная игра, которая веками завлекала миллионы людей. Онлайн формат дал ей свежую жизнь, сделав игры открытыми для всех желающих. Здесь лишь несколько оснований популярности онлайн покера:
Комфорт а доступность: Играть можно в любое время а в любом месте, имея только подключение к браузере или мобильному устройству. Большой выбор: Покеррумы дают разнообразные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Вы можете соревноваться с реальными соперниками со всего мира, а ни с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это ни только азарт, но также возможность пополнить свой кошелек. Социальный аспект: Можно играть с друзьями или заводить новые знакомства с людьми, разделяющих твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть множество сайты и покеррумы, предлагающих игры в онлайн покер. Однако каким образом подобрать лучший среди них? Мы окажем помощь тебе разобраться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая следующие критерии:
Наличие русского языка: Интерфейс и поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств должны быть доступны в рублях. Многообразие игр и турниров: Большой выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Безопасность а авторитет: Безопасность транзакций и честность игры. Топ румов постоянно обновляется, из-за этого рекомендуем следить за свежими обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда вы новичок, тогда следует обратить внимание на покеррумы с минимальным депозитом. Это позволит вам начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Проверьте, чтобы выбранный тобой рум является разрешенным в россии, для того чтобы избежать возможных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом и выбрали игру, вы способен положить свой баланс и начать играть на деньги. Большинство сайтов дают различные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума а выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за приватными столами. Это замечательный способ провести время с людьми, с которыми вы знакомы, и сразиться за звание лучшего игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда вы новичок а желаешь сначала освоиться с правилами, многие сайты дают шанс играть в бесплатный покер. Это отличный способ поупражняться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Когда ты выбираешь играть на пк, тогда большая часть покеррумов предлагают программы для компьютеров, которые гарантируют намного комфортный а стабильный игровой процесс.
Заключение: Начните Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это захватывающая игра, что способна принести тебе ни только наслаждение, а также реальные деньги. Подбирайте проверенные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со всего мира, а знайте, что главное – это получать наслаждение от игры!
Не откладывайте на потом, начните твою игру в онлайн покер непосредственно сейчас! Погрузитесь в мир тактики, азарта а возможностей! Везенья за столами!
Представляем вашему вниманию сайт, посвященный индийским фильмам и сериалам с онлайн-показом! На сайте вы обнаружите большой каталог культовых болливудских фильмов, а также топовые телесериалы, которые покорили сердца зрителей по всему миру. Мы представляем различные жанры: от захватывающих драм и романтических комедий до мистических триллеров и исторических эпопей. Наш каталог содержит как классические шедевры, так и современные хиты, чтобы все могли посмотреть что-то на свой вкус.
Благодаря нашему сайту вы можете посмотреть индийские фильмы про любовь находясь где угодно. Удобный интерфейс и отличное качество видеозаписей обеспечивают незабываемый просмотр. Окунитесь в мир болливудского кинематографа и откройте для себя его невероятную культуру на нашем портале!
Начни за Настоящие Деньги с Легкостью! Онлайн покер – является ни просто игра, это целая вселенная азарта, тактики и шанса выиграть реальные деньги, без выходя с дома. Когда вы пытаетесь где поиграть на покер в интернете, на русском языке и с выводом денег прямо на карту, значит вы оказались по адресу. Эта статья – ваш гид в мир онлайн покера в россии.
Отчего Онлайн Покер так Известен? Покер – есть классическая карточная игра, которая целые столетия привлекала миллионы людей. Онлайн формат дал ей свежую жизнь, сотворив игры открытыми для всех желающих. Вот лишь пара оснований популярности онлайн покера:
Комфорт и доступность: Играть возможно в любое время а в каком угодно месте, имея только доступ к браузере либо мобильному устройству. Обширный выбор: Покеррумы дают различные виды покера, форматы турниров и уровни ставок. Играть с реальными людьми: Ты способен бороться с реальными соперниками со всего мира, а ни с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это не просто волнение, а и шанс наполнить твой кошелек. Социальный аспект: Возможно играть с друзьями либо заводить новые знакомства с людьми, разделяющими ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть множество сайты и покеррумы, дающих игры в онлайн покер. Но каким образом подобрать лучший среди них? Мы окажем помощь вам сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая следующие критерии:
Наличие русского языка: Интерфейс и обслуживание обязаны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть доступны в рублях. Многообразие игр и турниров: Широкий выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Надежность а авторитет: Безопасность транзакций а честность игры. Топ румов регулярно обновляется, поэтому рекомендуем наблюдать за актуальными обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, то следует обратить внимание на покеррумы с минимальным депозитом. Данное даст возможность вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы подобранный вами рум есть разрешенным в россии, для того чтобы избежать вероятных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом и выбрали игру, ты способен положить свой баланс и начать играть на деньги. Большинство сайтов дают всевозможные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно занимает от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают шанс играть с друзьями за приватными столами. Данное отличный метод уделить время с людьми, с которыми вы знакомы, а побороться за титул лучшего игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда ты новичок а желаешь вначале привыкнуть с правилами, многие сайты предлагают возможность играть в бесплатный покер. Данное отличный метод потренироваться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если вы выбираешь играть на пк, тогда большинство покеррумов предлагают клиенты для компьютеров, что гарантируют более комфортный а стабильный игровой процесс.
Заключение: Приступайте Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть волнующая игра, которая может предоставить тебе ни просто наслаждение, а также реальные деньги. Выбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со целого мира, и знайте, что главное – есть испытывать наслаждение от игры!
Не откладывайте на потом, начните свою игру в онлайн покер прямо сейчас! Погрузитесь в мир стратегии, азарта и возможностей! Везенья за столами!
Начни за Реальные Средства со Комфортом! Онлайн покер – это ни просто игра, это полная вселенная азарта, тактики а шанса выиграть реальные деньги, без выходя из дома. Если вы ищете где поиграть на покер в интернете, на русском языке а с выводом денег непосредственно на карту, то ты попали нужному адресу. Данная статья – твой гид в мир онлайн покера в россии.
Отчего Онлайн Покер настолько Известен? Покер – есть классическая карточная игра, что целые столетия привлекала миллионы людей. Онлайн формат принес ей новую жизнь, сделав игры доступными для всех жаждущих. Здесь лишь несколько оснований популярности онлайн покера:
Удобство и доступность: Играть возможно в любое время а в любом месте, имея лишь подключение к браузере либо мобильному устройству. Большой выбор: Покеррумы предлагают разнообразные виды покера, варианты турниров а уровни ставок. Играть с реальными людьми: Вы можете соревноваться с реальными соперниками со целого мира, а не с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это ни просто азарт, а и возможность наполнить твой кошелек. Социальный аспект: Возможно играть с друзьями или заводить новые знакомства с людьми, разделяющими ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует большое количество сайты и покеррумы, дающих игры в онлайн покер. Но как выбрать оптимальный из них? Мы окажем помощь тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, учитывая следующие критерии:
Наличие русского языка: Интерфейс и обслуживание должны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть доступны в рублях. Разнообразие игр и турниров: Широкий выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность и репутация: Безопасность транзакций а честность игры. Топ румов регулярно обновляется, поэтому рекомендуем следить за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда вы новичок, то следует обратить внимание на покеррумы с минимальным депозитом. Это даст возможность тебе начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Проверьте, что выбранный вами рум является разрешенным в россии, чтобы избежать вероятных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом и подобрали игру, вы можете пополнить свой счет а начать играть на деньги. Большинство сайтов предлагают всевозможные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило требует от нескольких часов до нескольких дней, в зависимости от рума а выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают шанс играть с друзьями за закрытыми столами. Данное замечательный способ провести время с людьми, с которыми ты знакомы, а сразиться за титул лучшего игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок а хотите вначале освоиться с правилами, многие сайты предлагают возможность играть в бесплатный покер. Это отличный способ потренироваться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если вы предпочитаете играть на пк, то большинство покеррумов предлагают клиенты для компьютеров, которые гарантируют намного удобный и стабильный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, что может предоставить вам не только удовольствие, но и реальные деньги. Подбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со целого мира, и знайте, что главное – это получать наслаждение от игры!
Ни отсрочивайте на потом, приступайте твою игру в онлайн покер прямо сейчас! Окунитесь в мир стратегии, азарта а шансов! Везенья за столами!
Играй на Настоящие Средства со Легкостью! Онлайн покер – является не просто игра, это целая вселенная азарта, тактики и возможности заработать реальные деньги, не покидая с квартиры. Когда ты ищете где поиграть на покер в интернете, на русском языке а с выводом денег непосредственно на карту, значит ты попали нужному адресу. Данная публикация – ваш проводник в мир онлайн покера в россии.
Почему Онлайн Покер так Известен? Покер – есть классическая карточная игра, что целые столетия привлекала миллионы людей. Онлайн формат принес ей свежую жизнь, сотворив игры доступными для всех жаждущих. Вот только пара оснований популярности онлайн покера:
Комфорт и доступность: Играть возможно в всякое время и в каком угодно месте, обладая лишь подключение к браузере либо мобильному устройству. Большой выбор: Покеррумы предлагают различные виды покера, варианты турниров а уровни ставок. Играть с реальными людьми: Вы можете бороться с реальными соперниками со всего мира, а не с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – это не только волнение, но и шанс пополнить свой кошелек. Социальный аспект: Можно играть с друзьями либо устанавливать свежие знакомства с людьми, разделяющих ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть множество сайты и покеррумы, предлагающих игры в онлайн покер. Но как подобрать оптимальный среди них? Мы поможем вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая следующие критерии:
Наличие русского языка: Интерфейс а обслуживание обязаны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть открыты в рублях. Многообразие игр и турниров: Большой выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Надежность а репутация: Безопасность транзакций и честность игры. Топ румов регулярно обновляется, из-за этого советуем следить за актуальными обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда ты новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Это даст возможность вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы выбранный тобой рум является допущенным в россии, чтобы избежать вероятных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом и выбрали игру, ты можете положить свой счет а начать играть на деньги. Большинство сайтов предлагают всевозможные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило требует от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за закрытыми столами. Это отличный способ уделить время с людьми, с которыми вы знакомы, и сразиться за звание лучшего игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок а хотите сначала привыкнуть с правилами, многие сайты предлагают возможность играть в бесплатный покер. Данное прекрасный метод поупражняться и набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Если ты выбираешь играть на пк, то большая часть покеррумов предлагают программы для компьютеров, которые обеспечивают намного удобный а надежный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это захватывающая игра, что может принести вам не просто удовольствие, но и реальные деньги. Подбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со целого мира, а знайте, что главное – есть получать наслаждение от игры!
Ни откладывайте на потом, приступайте свою игру в онлайн покер прямо сейчас! Погрузитесь в мир тактики, волнения и возможностей! Везенья за столами!
Начни за Реальные Деньги со Удобством! Онлайн покер – является ни просто игра, данное полная мир азарта, тактики и шанса заработать реальные деньги, без покидая с дома. Если вы пытаетесь где поиграть в покер в интернете, на русском языке а с выводом денег непосредственно на карту, то ты оказались по адресу. Эта публикация – твой проводник в мир онлайн покера в россии.
Отчего Онлайн Покер так Популярен? Покер – это классическая карточная игра, что целые столетия завлекала миллионы людей. Онлайн формат принес ей новую жизнь, сделав игры открытыми для всех жаждущих. Здесь только пара причин популярности онлайн покера:
Удобство а доступность: Играть возможно в всякое время а в любом месте, обладая лишь доступ к браузере или мобильному устройству. Широкий выбор: Покеррумы дают различные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Ты можете соревноваться с реальными соперниками со всего мира, а ни с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это ни просто азарт, а и возможность наполнить свой бумажник. Социальный аспект: Возможно играть с друзьями либо заводить свежие знакомства с людьми, разделяющими ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует множество сайты и покеррумы, предлагающих игры в онлайн покер. Однако как выбрать оптимальный среди них? Мы поможем тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс а поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств должны быть открыты в рублях. Многообразие игр и турниров: Обширный выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Безопасность а авторитет: Безопасность транзакций и честность игры. Топ румов постоянно обновляется, из-за этого рекомендуем наблюдать за свежими обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, то следует обратить внимание на покеррумы с минимальным депозитом. Это позволит вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Проверьте, что подобранный вами рум есть разрешенным в россии, для того чтобы не допустить вероятных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты решили с покеррумом и подобрали игру, вы способен положить твой счет а начать играть на деньги. Большая часть сайтов предлагают различные способы пополнения и вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума а подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за приватными столами. Это отличный способ уделить время с людьми, с которыми ты знакомы, и побороться за титул оптимального игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда ты новичок а желаешь сначала освоиться с правилами, многие сайты дают возможность играть в бесплатный покер. Данное прекрасный способ поупражняться а набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Если вы выбираешь играть на пк, то большая часть покеррумов дают программы для компьютеров, которые обеспечивают более удобный а надежный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть волнующая игра, которая способна предоставить вам ни просто удовольствие, а также реальные деньги. Подбирайте надежные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со всего мира, а помните, что главное – это испытывать удовольствие от игры!
Ни отсрочивайте на потом, приступайте твою игру в онлайн покер непосредственно сейчас! Погрузитесь в мир стратегии, азарта а шансов! Удачи за столами!
Начни на Фактические Средства со Удобством! Онлайн покер – является не просто игра, данное целая вселенная азарта, тактики а шанса выиграть реальные деньги, не покидая с квартиры. Когда вы ищете где поиграть в покер в интернете, на русском языке а с выводом денег прямо на карту, то ты оказались по адресу. Данная статья – ваш проводник в мир онлайн покера в россии.
Почему Онлайн Покер настолько Известен? Покер – есть классическая карточная игра, которая целые столетия завлекала миллионы людей. Онлайн формат принес ей свежую жизнь, сделав игры открытыми для каждого желающих. Вот только несколько причин популярности онлайн покера:
Удобство а открытость: Играть возможно в всякое время и в любом месте, обладая лишь доступ к браузере или мобильному устройству. Большой выбор: Покеррумы обеспечивают различные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Вы способен соревноваться с реальными соперниками со всего мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – является ни просто волнение, но также шанс наполнить свой бумажник. Социальный аспект: Можно играть с друзьями либо заводить свежие знакомства с людьми, разделяющих ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует множество сайты и покеррумы, дающих игры в онлайн покер. Однако каким образом выбрать лучший среди них? Мы окажем помощь тебе разобраться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс и обслуживание должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств обязаны быть доступны в рублях. Многообразие игр и турниров: Обширный выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность а репутация: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, из-за этого советуем следить за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда ты новичок, тогда стоит обратить внимание на покеррумы с минимальным депозитом. Данное позволит тебе начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Проверьте, чтобы подобранный тобой рум является допущенным в россии, чтобы не допустить возможных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом а подобрали игру, вы способен положить твой счет а начать играть на деньги. Большая часть сайтов предлагают различные способы пополнения а вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно занимает от нескольких часов до нескольких дней, в зависимости от рума а подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за закрытыми столами. Данное замечательный способ уделить время с людьми, с которыми вы знакомы, и побороться за звание лучшего игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок и желаешь сначала освоиться с правилами, многие сайты предлагают шанс играть в бесплатный покер. Это отличный способ поупражняться и получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Когда вы предпочитаете играть на пк, тогда большая часть покеррумов предлагают клиенты для компьютеров, которые обеспечивают более удобный и надежный игровой процесс.
Заключение: Начните Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это захватывающая игра, что способна принести вам не просто наслаждение, а и реальные деньги. Выбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со целого мира, и помните, что главное – это испытывать удовольствие от игры!
Не отсрочивайте на потом, приступайте твою игру в онлайн покер непосредственно сейчас! Погрузитесь в мир стратегии, волнения а возможностей! Везенья за столами!
Действуй на Настоящие Средства со Легкостью! Онлайн покер – является не только игра, это целая вселенная азарта, тактики а шанса заработать реальные деньги, не выходя из квартиры. Если вы пытаетесь где поиграть на покер в интернете, на русском языке а с выводом денег непосредственно на карту, значит ты оказались по адресу. Эта публикация – ваш проводник в мир онлайн покера в россии.
Почему Онлайн Покер так Известен? Покер – это традиционная карточная игра, что целые столетия завлекала миллионы людей. Онлайн формат принес этой свежую жизнь, сотворив игры открытыми для всех желающих. Вот лишь пара оснований популярности онлайн покера:
Удобство и открытость: Играть возможно в любое время а в каком угодно месте, имея лишь подключение к браузере или мобильному устройству. Обширный выбор: Покеррумы предлагают разнообразные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Ты способен соревноваться с реальными соперниками со всего мира, а не с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это не просто азарт, а и возможность пополнить свой кошелек. Социальный аспект: Возможно играть с друзьями или устанавливать свежие знакомства с людьми, разделяющими ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует множество сайты и покеррумы, предлагающих игры в онлайн покер. Но каким образом выбрать оптимальный из них? Мы поможем тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, учитывая такие критерии:
Наличие русского языка: Интерфейс а поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств должны быть доступны в рублях. Разнообразие игр и турниров: Широкий выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность и репутация: Безопасность транзакций и честность игры. Топ румов регулярно обновляется, поэтому рекомендуем следить за свежими обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Данное позволит вам начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, что выбранный тобой рум есть допущенным в россии, для того чтобы избежать вероятных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты решили с покеррумом и подобрали игру, вы способен пополнить свой счет а начать играть на деньги. Большинство сайтов предлагают всевозможные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно занимает от нескольких часов до нескольких дней, в зависимости от рума а выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают шанс играть с друзьями за приватными столами. Это замечательный способ уделить время с людьми, с которыми ты знакомы, и побороться за звание оптимального игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок а желаешь вначале освоиться с правилами, многие сайты предлагают возможность играть в бесплатный покер. Данное прекрасный метод поупражняться а набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Когда ты выбираешь играть на пк, то большинство покеррумов дают программы для компьютеров, что обеспечивают намного комфортный а надежный игровой процесс.
Заключение: Начните Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, что может предоставить вам ни только удовольствие, а и реальные деньги. Подбирайте надежные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со целого мира, и знайте, что главное – есть испытывать наслаждение от игры!
Ни отсрочивайте на потом, приступайте свою игру в онлайн покер непосредственно сейчас! Окунитесь в мир тактики, волнения а шансов! Везенья за столами!
Действуй за Настоящие Средства со Легкостью! Онлайн покер – является ни только игра, данное полная мир волнения, стратегии и шанса заработать реальные деньги, не выходя с квартиры. Если ты ищете где поиграть на покер в интернете, на русском языке а с выводом денег непосредственно на карту, значит вы оказались по месту. Эта публикация – ваш гид в мир онлайн покера в россии.
Отчего Онлайн Покер так Популярен? Покер – есть классическая карточная игра, что целые столетия завлекала миллионы людей. Онлайн формат дал этой свежую жизнь, сделав игры доступными для всех желающих. Вот только несколько оснований популярности онлайн покера:
Удобство а открытость: Играть можно в любое время и в каком угодно месте, обладая только подключение к браузере либо мобильному устройству. Большой выбор: Покеррумы предлагают разнообразные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Вы способен бороться с реальными соперниками со целого мира, а не с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это не просто волнение, а также шанс пополнить свой бумажник. Социальный аспект: Можно играть с друзьями либо устанавливать новые знакомства с людьми, разделяющими ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Однако как подобрать оптимальный из них? Мы окажем помощь вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая такие критерии:
Наличие русского языка: Интерфейс и обслуживание обязаны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств обязаны быть открыты в рублях. Многообразие игр и турниров: Широкий выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Надежность а авторитет: Безопасность транзакций а честность игры. Топ румов регулярно обновляется, из-за этого рекомендуем следить за свежими обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда ты новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Это позволит тебе начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, что выбранный вами рум есть допущенным в россии, чтобы не допустить возможных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом и выбрали игру, ты можете положить свой счет а начать играть на деньги. Большая часть сайтов дают всевозможные способы пополнения а вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно занимает от нескольких часов до нескольких дней, в зависимости от рума а подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за закрытыми столами. Данное отличный метод уделить время с людьми, с которыми ты знакомы, и сразиться за звание оптимального игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если вы новичок а хотите вначале привыкнуть с правилами, многие сайты дают шанс играть в бесплатный покер. Это прекрасный способ поупражняться а набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если ты предпочитаете играть на пк, тогда большая часть покеррумов предлагают программы для компьютеров, что гарантируют намного удобный а стабильный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, которая может принести вам не просто наслаждение, но также реальные деньги. Выбирайте проверенные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со всего мира, а знайте, что главное – есть испытывать наслаждение от игры!
Ни отсрочивайте на потом, приступайте твою игру в онлайн покер прямо сейчас! Погрузитесь в мир стратегии, азарта и шансов! Удачи за столами!
Начни за Реальные Средства со Комфортом! Онлайн покер – является ни только игра, данное вся вселенная азарта, стратегии а возможности заработать реальные деньги, без покидая с дома. Когда вы ищете где поиграть на покер в интернете, на русском языке а с выводом денег непосредственно на карту, то вы попали по адресу. Эта публикация – твой проводник в мир онлайн покера в россии.
Отчего Онлайн Покер так Популярен? Покер – есть классическая карточная игра, что веками завлекала миллионы людей. Онлайн формат принес ей свежую жизнь, сотворив игры открытыми для каждого жаждущих. Здесь лишь несколько причин известности онлайн покера:
Комфорт и доступность: Играть можно в всякое время и в каком угодно месте, имея лишь подключение к браузере либо мобильному устройству. Обширный выбор: Покеррумы дают разнообразные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Вы можете бороться с реальными соперниками со целого мира, а не с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это не только волнение, а и возможность наполнить свой кошелек. Социальный аспект: Можно играть с друзьями или устанавливать свежие знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Однако как подобрать лучший среди них? Мы поможем тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, беря во внимание следующие критерии:
Наличие русского языка: Интерфейс а поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств должны быть доступны в рублях. Разнообразие игр и турниров: Обширный выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Надежность а репутация: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, поэтому рекомендуем наблюдать за актуальными обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если ты новичок, тогда стоит обратить внимание на покеррумы с минимальным депозитом. Это даст возможность вам начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы подобранный тобой рум является разрешенным в россии, чтобы не допустить вероятных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом и подобрали игру, вы можете положить свой баланс и начать играть на деньги. Большая часть сайтов предлагают различные способы пополнения и вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно занимает от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают шанс играть с друзьями за закрытыми столами. Это замечательный способ уделить время с людьми, с которыми вы знакомы, и побороться за титул лучшего игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда ты новичок и хотите вначале освоиться с правилами, многие сайты предлагают шанс играть в бесплатный покер. Данное отличный метод поупражняться и набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Если вы выбираешь играть на пк, то большая часть покеррумов дают клиенты для компьютеров, что гарантируют намного комфортный а стабильный игровой процесс.
Заключение: Начните Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это захватывающая игра, что может принести тебе ни просто удовольствие, но и реальные деньги. Подбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со целого мира, а знайте, что главное – есть испытывать наслаждение от игры!
Не откладывайте на потом, начните твою игру в онлайн покер непосредственно сейчас! Погрузитесь в мир стратегии, азарта а возможностей! Удачи за столами!
Начни за Настоящие Деньги со Удобством! Онлайн покер – это ни только игра, данное целая мир волнения, тактики а шанса заработать реальные деньги, не покидая из дома. Когда вы пытаетесь где поиграть в покер в интернете, на русском языке и с выводом денег непосредственно на карту, значит вы оказались по месту. Данная статья – твой проводник в мир онлайн покера в россии.
Отчего Онлайн Покер настолько Популярен? Покер – это традиционная карточная игра, которая веками привлекала миллионы людей. Онлайн формат дал этой новую жизнь, сотворив игры доступными для каждого жаждущих. Здесь лишь пара причин популярности онлайн покера:
Удобство и доступность: Играть возможно в всякое время а в любом месте, обладая только подключение к браузере либо мобильному устройству. Обширный выбор: Покеррумы обеспечивают различные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Вы можете соревноваться с реальными соперниками со целого мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – это не просто азарт, а также возможность пополнить твой бумажник. Социальный аспект: Можно играть с друзьями или заводить новые знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Однако каким образом подобрать лучший среди них? Мы поможем вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс а поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств обязаны быть открыты в рублях. Разнообразие игр и турниров: Широкий выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Безопасность а авторитет: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, из-за этого рекомендуем следить за актуальными обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда вы новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Данное даст возможность вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, что выбранный вами рум есть допущенным в россии, для того чтобы не допустить вероятных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом и подобрали игру, вы способен пополнить свой баланс а начать играть на деньги. Большинство сайтов дают различные способы пополнения а вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно занимает от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за закрытыми столами. Данное замечательный способ уделить время с людьми, с которыми ты знакомы, и побороться за звание лучшего игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если вы новичок а желаешь сначала освоиться с правилами, многие сайты предлагают шанс играть в бесплатный покер. Данное прекрасный метод потренироваться и набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Когда ты предпочитаете играть на пк, то большинство покеррумов предлагают клиенты для компьютеров, что гарантируют более комфортный и надежный игровой процесс.
Заключение: Приступайте Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это волнующая игра, которая может принести вам не просто удовольствие, а также реальные деньги. Выбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со целого мира, а знайте, что главное – есть получать наслаждение от игры!
Ни откладывайте на потом, приступайте свою игру в онлайн покер прямо сейчас! Окунитесь в мир стратегии, азарта а возможностей! Удачи за столами!
Играй на Фактические Средства со Удобством! Онлайн покер – это ни просто игра, данное вся вселенная волнения, стратегии и шанса выиграть реальные деньги, без выходя с дома. Когда вы пытаетесь где поиграть на покер в интернете, на русском языке а с выводом денег прямо на карту, значит вы попали по месту. Данная статья – твой проводник в мир онлайн покера в россии.
Отчего Онлайн Покер настолько Популярен? Покер – это классическая карточная игра, что целые столетия привлекала миллионы людей. Онлайн формат дал этой новую жизнь, сотворив игры доступными для каждого жаждущих. Вот лишь несколько причин известности онлайн покера:
Комфорт а открытость: Играть возможно в любое время и в любом месте, обладая лишь подключение к браузере или мобильному устройству. Широкий выбор: Покеррумы обеспечивают разнообразные виды покера, форматы турниров и уровни ставок. Играть с реальными людьми: Ты можете соревноваться с реальными соперниками со целого мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – это ни только азарт, но и шанс пополнить твой кошелек. Социальный аспект: Возможно играть с друзьями либо устанавливать свежие знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Однако каким образом выбрать оптимальный среди них? Мы окажем помощь тебе разобраться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая такие критерии:
Наличие русского языка: Интерфейс и поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств должны быть доступны в рублях. Разнообразие игр и турниров: Обширный выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность и авторитет: Безопасность транзакций и честность игры. Топ румов постоянно обновляется, поэтому советуем наблюдать за актуальными обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, то следует обратить внимание на покеррумы с минимальным депозитом. Данное позволит тебе начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Проверьте, чтобы подобранный вами рум является допущенным в россии, чтобы избежать возможных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты решили с покеррумом и выбрали игру, ты способен положить твой баланс и начать играть на деньги. Большинство сайтов дают различные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума а выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают шанс играть с друзьями за закрытыми столами. Данное замечательный способ уделить время с людьми, с которыми ты знакомы, а сразиться за титул оптимального игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок и желаешь сначала привыкнуть с правилами, многие сайты дают шанс играть в бесплатный покер. Данное отличный способ поупражняться и получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Когда вы выбираешь играть на пк, тогда большая часть покеррумов предлагают клиенты для компьютеров, что обеспечивают намного удобный а стабильный игровой процесс.
Заключение: Начните Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это волнующая игра, что способна предоставить тебе не просто удовольствие, но и реальные деньги. Подбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, а помните, что главное – есть получать удовольствие от игры!
Ни отсрочивайте на потом, приступайте свою игру в онлайн покер непосредственно сейчас! Погрузитесь в мир стратегии, азарта а шансов! Везенья за столами!
Играй за Фактические Средства с Легкостью! Онлайн покер – это ни просто игра, это вся мир волнения, стратегии и шанса заработать реальные деньги, без выходя из дома. Если вы ищете где поиграть на покер в интернете, на русском языке и с выводом денег непосредственно на карту, то вы попали по адресу. Эта публикация – твой проводник в мир онлайн покера в россии.
Отчего Онлайн Покер настолько Популярен? Покер – есть классическая карточная игра, что целые столетия привлекала миллионы людей. Онлайн формат принес ей свежую жизнь, сделав игры доступными для каждого желающих. Вот лишь несколько причин популярности онлайн покера:
Комфорт а открытость: Играть возможно в всякое время а в любом месте, имея только подключение к браузере либо мобильному устройству. Широкий выбор: Покеррумы дают разнообразные виды покера, варианты турниров а уровни ставок. Играть с реальными людьми: Ты способен соревноваться с реальными соперниками со всего мира, а не с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – является не только азарт, а также шанс пополнить твой кошелек. Социальный аспект: Возможно играть с друзьями либо заводить свежие знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует множество сайты и покеррумы, дающих игры в онлайн покер. Однако как выбрать оптимальный среди них? Мы окажем помощь вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс и поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств должны быть открыты в рублях. Многообразие игр и турниров: Большой выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Безопасность и авторитет: Безопасность транзакций и честность игры. Топ румов постоянно обновляется, из-за этого рекомендуем следить за свежими обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда ты новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Это даст возможность тебе начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Проверьте, чтобы выбранный вами рум является разрешенным в россии, чтобы избежать возможных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом а подобрали игру, ты можете пополнить свой баланс и начать играть на деньги. Большинство сайтов предлагают различные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума и подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за приватными столами. Это отличный способ уделить время с людьми, с которыми вы знакомы, и сразиться за звание оптимального игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если вы новичок а желаешь вначале освоиться с правилами, многие сайты предлагают возможность играть в бесплатный покер. Данное прекрасный способ поупражняться и набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Когда ты предпочитаете играть на пк, тогда большинство покеррумов дают программы для компьютеров, которые обеспечивают более комфортный и стабильный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это захватывающая игра, что может принести вам ни только наслаждение, но и реальные деньги. Подбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, и помните, что главное – это испытывать удовольствие от игры!
Ни отсрочивайте на потом, начните свою игру в онлайн покер непосредственно сейчас! Погрузитесь в мир стратегии, волнения а шансов! Удачи за столами!
Представляем вашему вниманию сайт, с индийскими фильмами и сериалами с онлайн-показом! Здесь вы найдете огромный выбор популярнейших болливудских фильмов, а также излюбленные телесериалы, покорившие сердца зрителей по всему миру. У нас вы найдете любые жанры: от невероятных драм и романтических комедий до мистических триллеров и исторических эпопей. Наш сайт включает как давно полюбившиеся всем фильмы, так и современные хиты, чтобы все могли посмотреть что-то подходящее.
Благодаря нашему сайту вы можете посмотреть смотреть онлайн индийские фильмы новинки находясь где угодно. Удобный интерфейс и высокое качество видеозаписей обеспечивают непревзойденный отдых во время просмотра. Окунитесь в мир индийского кинематографа и откройте для себя его захватывающую культуру в нашем онлайн кинотеатре!
Играй за Фактические Средства с Удобством! Онлайн покер – является ни просто игра, это вся вселенная волнения, тактики а шанса заработать реальные деньги, без покидая с квартиры. Когда ты пытаетесь где поиграть в покер в интернете, на русском языке а с выводом денег прямо на карту, то ты оказались по адресу. Эта статья – ваш проводник в мир онлайн покера в россии.
Отчего Онлайн Покер настолько Популярен? Покер – есть классическая карточная игра, что целые столетия привлекала миллионы людей. Онлайн формат дал этой новую жизнь, сотворив игры открытыми для каждого жаждущих. Здесь только несколько причин известности онлайн покера:
Удобство а доступность: Играть возможно в всякое время а в каком угодно месте, обладая лишь подключение к браузере либо мобильному устройству. Обширный выбор: Покеррумы обеспечивают разнообразные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Ты способен бороться с реальными соперниками со всего мира, а ни с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это не только азарт, но и возможность наполнить твой бумажник. Социальный аспект: Возможно играть с друзьями либо заводить новые знакомства с людьми, разделяющими ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Однако как выбрать лучший из них? Мы окажем помощь вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, учитывая такие критерии:
Наличие русского языка: Интерфейс и поддержка обязаны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств обязаны быть доступны в рублях. Разнообразие игр и турниров: Большой выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Надежность и репутация: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, поэтому рекомендуем наблюдать за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда вы новичок, тогда следует обратить внимание на покеррумы с минимальным депозитом. Данное позволит вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Проверьте, что выбранный тобой рум является допущенным в россии, для того чтобы избежать возможных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом и подобрали игру, ты способен положить свой счет и начать играть на деньги. Большинство сайтов предлагают различные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно требует от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают шанс играть с друзьями за закрытыми столами. Это замечательный метод провести время с людьми, с которыми вы знакомы, и побороться за звание оптимального игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда вы новичок а желаешь вначале привыкнуть с правилами, многие сайты предлагают шанс играть в бесплатный покер. Это отличный метод потренироваться и получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если ты предпочитаете играть на пк, то большинство покеррумов предлагают клиенты для компьютеров, что обеспечивают намного удобный и стабильный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, которая способна предоставить вам ни только наслаждение, но также реальные деньги. Выбирайте проверенные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со всего мира, а помните, что главное – это получать удовольствие от игры!
Ни отсрочивайте на потом, приступайте твою игру в онлайн покер непосредственно сейчас! Окунитесь в мир тактики, волнения а возможностей! Удачи за столами!
Действуй за Настоящие Средства с Комфортом! Онлайн покер – это не только игра, данное целая мир волнения, стратегии а возможности выиграть реальные деньги, не выходя из квартиры. Когда вы ищете где поиграть на покер в интернете, на русском языке а с выводом денег непосредственно на карту, значит вы попали нужному адресу. Данная публикация – твой гид в мир онлайн покера в россии.
Отчего Онлайн Покер так Популярен? Покер – это классическая карточная игра, которая целые столетия привлекала миллионы людей. Онлайн формат дал этой свежую жизнь, сотворив игры доступными для всех жаждущих. Здесь только несколько оснований популярности онлайн покера:
Комфорт и доступность: Играть можно в всякое время и в каком угодно месте, обладая лишь доступ к браузере либо мобильному устройству. Большой выбор: Покеррумы предлагают различные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Ты способен бороться с реальными соперниками со всего мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – является не только волнение, но и шанс пополнить твой бумажник. Социальный аспект: Возможно играть с друзьями или заводить свежие знакомства с людьми, разделяющих ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует большое количество сайты и покеррумы, дающих игры в онлайн покер. Однако каким образом подобрать лучший из них? Мы окажем помощь тебе разобраться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс и обслуживание обязаны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств должны быть открыты в рублях. Разнообразие игр и турниров: Большой выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность а авторитет: Безопасность транзакций и честность игры. Топ румов регулярно обновляется, поэтому рекомендуем наблюдать за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда вы новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Это позволит вам начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, что подобранный тобой рум является разрешенным в россии, для того чтобы избежать возможных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом и подобрали игру, ты можете положить твой счет и начать играть на деньги. Большинство сайтов предлагают всевозможные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно занимает от нескольких часов до нескольких дней, в зависимости от рума и подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают шанс играть с друзьями за приватными столами. Данное замечательный метод уделить время с людьми, с которыми ты знакомы, а побороться за титул оптимального игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок а желаешь сначала освоиться с правилами, многие сайты предлагают возможность играть в бесплатный покер. Данное прекрасный метод поупражняться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если вы выбираешь играть на пк, то большинство покеррумов предлагают программы для компьютеров, что обеспечивают намного комфортный а стабильный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, которая способна предоставить тебе не просто наслаждение, но также реальные деньги. Выбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, и знайте, что главное – это получать наслаждение от игры!
Ни отсрочивайте на потом, начните твою игру в онлайн покер непосредственно сейчас! Окунитесь в мир стратегии, волнения и возможностей! Везенья за столами!
Начни за Фактические Деньги с Комфортом! Онлайн покер – это не просто игра, это целая мир волнения, стратегии и шанса заработать реальные деньги, без покидая из квартиры. Если вы пытаетесь где поиграть в покер в интернете, на русском языке а с выводом денег прямо на карту, значит вы оказались по адресу. Эта статья – твой гид в мир онлайн покера в россии.
Почему Онлайн Покер так Популярен? Покер – есть классическая карточная игра, что веками привлекала миллионы людей. Онлайн формат принес ей свежую жизнь, сотворив игры открытыми для всех жаждущих. Вот лишь пара причин известности онлайн покера:
Удобство а доступность: Играть можно в всякое время и в любом месте, имея лишь подключение к браузере или мобильному устройству. Широкий выбор: Покеррумы предлагают разнообразные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Ты способен соревноваться с реальными соперниками со всего мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – является ни просто волнение, но также шанс пополнить твой кошелек. Социальный аспект: Можно играть с друзьями или заводить свежие знакомства с людьми, разделяющих твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует множество сайты и покеррумы, предлагающих игры в онлайн покер. Но как выбрать оптимальный из них? Мы окажем помощь тебе разобраться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс а обслуживание должны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть открыты в рублях. Разнообразие игр и турниров: Широкий выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Надежность и репутация: Безопасность транзакций а честность игры. Топ румов регулярно обновляется, поэтому рекомендуем следить за свежими обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда ты новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Данное даст возможность вам начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Проверьте, что подобранный тобой рум является допущенным в россии, чтобы избежать вероятных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы определились с покеррумом и выбрали игру, ты можете положить свой счет а начать играть на деньги. Большая часть сайтов предлагают всевозможные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно требует от нескольких часов до нескольких дней, в зависимости от рума а выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают шанс играть с друзьями за приватными столами. Данное замечательный метод уделить время с людьми, с которыми ты знакомы, и побороться за звание лучшего игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если вы новичок а хотите вначале привыкнуть с правилами, многие сайты предлагают возможность играть в бесплатный покер. Это прекрасный метод потренироваться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Если вы выбираешь играть на пк, тогда большая часть покеррумов дают клиенты для компьютеров, что гарантируют намного удобный а надежный игровой процесс.
Заключение: Начните Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это захватывающая игра, которая может предоставить тебе не только наслаждение, а также реальные деньги. Подбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, и знайте, что главное – есть получать удовольствие от игры!
Не откладывайте на потом, приступайте твою игру в онлайн покер непосредственно сейчас! Погрузитесь в мир стратегии, волнения а шансов! Везенья за столами!
Начни на Фактические Деньги со Легкостью! Онлайн покер – является ни просто игра, данное полная вселенная азарта, стратегии а возможности заработать реальные деньги, не покидая с квартиры. Когда вы пытаетесь где поиграть в покер в интернете, на русском языке а с выводом денег прямо на карту, то вы оказались по месту. Эта статья – ваш гид в мир онлайн покера в россии.
Почему Онлайн Покер так Известен? Покер – это традиционная карточная игра, которая веками завлекала миллионы людей. Онлайн формат принес ей новую жизнь, сделав игры доступными для каждого желающих. Здесь лишь несколько причин популярности онлайн покера:
Комфорт и доступность: Играть возможно в любое время а в любом месте, имея только подключение к браузере либо мобильному устройству. Большой выбор: Покеррумы дают различные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Ты способен бороться с реальными соперниками со целого мира, а не с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – является ни просто волнение, но и возможность наполнить твой кошелек. Социальный аспект: Можно играть с друзьями либо устанавливать новые знакомства с людьми, разделяющими ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Но каким образом подобрать оптимальный среди них? Мы окажем помощь вам сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, учитывая такие критерии:
Наличие русского языка: Интерфейс а обслуживание должны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств обязаны быть открыты в рублях. Многообразие игр и турниров: Обширный выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит и другие акции. Надежность а авторитет: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, из-за этого советуем следить за свежими обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда ты новичок, то стоит обратить внимание на покеррумы с минимальным депозитом. Это позволит вам начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Проверьте, что подобранный вами рум есть допущенным в россии, для того чтобы избежать вероятных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом а выбрали игру, вы способен пополнить твой счет и начать играть на деньги. Большинство сайтов предлагают всевозможные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило требует от нескольких часов до нескольких дней, в зависимости от рума и подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за приватными столами. Данное отличный метод уделить время с людьми, с которыми ты знакомы, а побороться за звание оптимального игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда ты новичок а хотите вначале привыкнуть с правилами, многие сайты дают возможность играть в бесплатный покер. Данное прекрасный способ поупражняться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Когда ты выбираешь играть на пк, тогда большая часть покеррумов предлагают клиенты для компьютеров, что обеспечивают намного комфортный а стабильный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это волнующая игра, которая способна предоставить вам не только наслаждение, но также реальные деньги. Выбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, и знайте, что главное – есть получать удовольствие от игры!
Не откладывайте на потом, приступайте свою игру в онлайн покер непосредственно сейчас! Окунитесь в мир тактики, азарта и возможностей! Везенья за столами!
Играй на Реальные Деньги со Комфортом! Онлайн покер – это ни только игра, это целая вселенная волнения, тактики а возможности выиграть реальные деньги, без покидая из дома. Если ты пытаетесь где поиграть на покер в интернете, на русском языке а с выводом денег непосредственно на карту, то вы оказались нужному адресу. Данная публикация – твой гид в мир онлайн покера в россии.
Почему Онлайн Покер так Известен? Покер – есть классическая карточная игра, которая веками привлекала миллионы людей. Онлайн формат дал этой новую жизнь, сотворив игры доступными для всех жаждущих. Здесь только пара причин популярности онлайн покера:
Комфорт и доступность: Играть можно в любое время и в каком угодно месте, обладая только подключение к браузере или мобильному устройству. Широкий выбор: Покеррумы обеспечивают разнообразные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Ты можете бороться с реальными соперниками со всего мира, а не с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – является ни просто волнение, но и возможность пополнить свой бумажник. Социальный аспект: Возможно играть с друзьями либо заводить новые знакомства с людьми, разделяющих ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует множество сайты и покеррумы, дающих игры в онлайн покер. Но как выбрать оптимальный среди них? Мы поможем тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс и обслуживание обязаны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств обязаны быть открыты в рублях. Многообразие игр и турниров: Широкий выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность и авторитет: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, из-за этого рекомендуем следить за актуальными обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда ты новичок, то следует обратить внимание на покеррумы с минимальным депозитом. Это позволит тебе начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы подобранный вами рум является разрешенным в россии, чтобы избежать возможных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты решили с покеррумом и выбрали игру, ты можете пополнить твой баланс и начать играть на деньги. Большая часть сайтов предлагают всевозможные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума и подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают шанс играть с друзьями за приватными столами. Это отличный метод провести время с людьми, с которыми вы знакомы, и сразиться за звание оптимального игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок и желаешь вначале привыкнуть с правилами, многие сайты дают шанс играть в бесплатный покер. Данное отличный способ поупражняться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если вы предпочитаете играть на пк, то большая часть покеррумов дают программы для компьютеров, которые гарантируют намного удобный и надежный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, что может принести тебе не только наслаждение, а также реальные деньги. Выбирайте проверенные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со всего мира, а помните, что главное – это получать удовольствие от игры!
Не откладывайте на потом, приступайте твою игру в онлайн покер прямо сейчас! Окунитесь в мир тактики, азарта а шансов! Везенья за столами!
Играй за Настоящие Деньги со Комфортом! Онлайн покер – является не просто игра, данное целая мир азарта, тактики и шанса заработать реальные деньги, без выходя из дома. Когда ты ищете где поиграть в покер в интернете, на русском языке а с выводом денег непосредственно на карту, значит вы оказались по адресу. Эта публикация – ваш проводник в мир онлайн покера в россии.
Отчего Онлайн Покер так Популярен? Покер – есть классическая карточная игра, которая целые столетия привлекала миллионы людей. Онлайн формат дал ей новую жизнь, сделав игры доступными для каждого жаждущих. Вот только пара причин популярности онлайн покера:
Комфорт а доступность: Играть возможно в всякое время а в любом месте, обладая лишь подключение к браузере или мобильному устройству. Большой выбор: Покеррумы обеспечивают разнообразные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Вы способен соревноваться с реальными соперниками со всего мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – является ни просто волнение, а и возможность пополнить твой бумажник. Социальный аспект: Можно играть с друзьями либо устанавливать новые знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии существует большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Но как выбрать оптимальный среди них? Мы поможем тебе разобраться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, учитывая следующие критерии:
Наличие русского языка: Интерфейс а обслуживание обязаны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств обязаны быть открыты в рублях. Разнообразие игр и турниров: Обширный выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность а авторитет: Безопасность транзакций и честность игры. Топ румов регулярно обновляется, из-за этого рекомендуем следить за свежими обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если ты новичок, тогда следует обратить внимание на покеррумы с минимальным депозитом. Это даст возможность тебе начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы выбранный тобой рум есть разрешенным в россии, для того чтобы избежать возможных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом и подобрали игру, вы способен положить твой счет и начать играть на деньги. Большинство сайтов дают всевозможные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило требует от нескольких часов до нескольких дней, в зависимости от рума а подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за приватными столами. Это замечательный способ провести время с людьми, с которыми вы знакомы, и сразиться за титул оптимального игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если вы новичок и желаешь вначале освоиться с правилами, многие сайты предлагают шанс играть в бесплатный покер. Данное отличный метод потренироваться а набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если вы выбираешь играть на пк, тогда большинство покеррумов дают программы для компьютеров, которые гарантируют намного удобный а стабильный игровой процесс.
Заключение: Начните Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, которая может принести вам ни просто наслаждение, а также реальные деньги. Подбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со целого мира, а помните, что главное – есть получать удовольствие от игры!
Не отсрочивайте на потом, начните свою игру в онлайн покер непосредственно сейчас! Погрузитесь в мир тактики, азарта и возможностей! Везенья за столами!
Начни на Реальные Средства с Удобством! Онлайн покер – это не только игра, данное вся мир азарта, стратегии а возможности выиграть реальные деньги, не покидая с квартиры. Если вы ищете где поиграть в покер в интернете, на русском языке а с выводом денег прямо на карту, значит ты попали по адресу. Данная статья – твой гид в мир онлайн покера в россии.
Отчего Онлайн Покер настолько Известен? Покер – это традиционная карточная игра, что целые столетия завлекала миллионы людей. Онлайн формат дал этой свежую жизнь, сделав игры открытыми для каждого жаждущих. Здесь только пара причин популярности онлайн покера:
Комфорт и открытость: Играть можно в любое время а в каком угодно месте, имея лишь доступ к браузере либо мобильному устройству. Обширный выбор: Покеррумы обеспечивают различные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Вы способен бороться с реальными соперниками со целого мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – это ни только волнение, но также возможность наполнить свой кошелек. Социальный аспект: Можно играть с друзьями либо устанавливать свежие знакомства с людьми, разделяющих твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Однако каким образом подобрать лучший среди них? Мы поможем вам сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая следующие критерии:
Наличие русского языка: Интерфейс и поддержка обязаны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств обязаны быть доступны в рублях. Разнообразие игр и турниров: Большой выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность а авторитет: Безопасность транзакций и честность игры. Топ румов регулярно обновляется, поэтому рекомендуем наблюдать за свежими обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если ты новичок, то следует обратить внимание на покеррумы с минимальным депозитом. Данное позволит тебе начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, что подобранный вами рум есть допущенным в россии, чтобы не допустить возможных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом а выбрали игру, ты можете положить свой счет а начать играть на деньги. Большинство сайтов предлагают всевозможные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума и подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают шанс играть с друзьями за приватными столами. Данное отличный способ уделить время с людьми, с которыми вы знакомы, а побороться за титул лучшего игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда ты новичок а хотите сначала освоиться с правилами, многие сайты дают возможность играть в бесплатный покер. Данное прекрасный метод потренироваться и набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Когда вы предпочитаете играть на пк, то большая часть покеррумов дают клиенты для компьютеров, которые обеспечивают намного комфортный и стабильный игровой процесс.
Заключение: Начните Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это захватывающая игра, что может принести вам не просто удовольствие, а также реальные деньги. Выбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, а знайте, что главное – это получать удовольствие от игры!
Ни отсрочивайте на потом, приступайте твою игру в онлайн покер прямо сейчас! Окунитесь в мир стратегии, волнения и возможностей! Везенья за столами!
Действуй на Реальные Средства с Удобством! Онлайн покер – является ни только игра, данное вся вселенная азарта, тактики а возможности заработать реальные деньги, без выходя из дома. Когда вы ищете где поиграть в покер в интернете, на русском языке и с выводом денег непосредственно на карту, то вы попали нужному адресу. Данная публикация – твой проводник в мир онлайн покера в россии.
Почему Онлайн Покер настолько Популярен? Покер – есть традиционная карточная игра, что веками привлекала миллионы людей. Онлайн формат принес ей новую жизнь, сотворив игры открытыми для каждого желающих. Вот лишь пара оснований популярности онлайн покера:
Комфорт и открытость: Играть можно в любое время и в любом месте, имея лишь доступ к браузере либо мобильному устройству. Широкий выбор: Покеррумы дают различные виды покера, варианты турниров а уровни ставок. Играть с реальными людьми: Вы способен соревноваться с реальными соперниками со всего мира, а не с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – это не только азарт, а также шанс пополнить свой кошелек. Социальный аспект: Возможно играть с друзьями или заводить новые знакомства с людьми, разделяющих твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть большое количество сайты и покеррумы, предлагающих игры в онлайн покер. Но как выбрать лучший из них? Мы окажем помощь вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, беря во внимание следующие критерии:
Наличие русского языка: Интерфейс а поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть доступны в рублях. Многообразие игр и турниров: Обширный выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Надежность и репутация: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, из-за этого рекомендуем наблюдать за актуальными обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если ты новичок, тогда стоит обратить внимание на покеррумы с минимальным депозитом. Это позволит вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы выбранный вами рум есть допущенным в россии, для того чтобы не допустить вероятных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом и выбрали игру, ты способен положить свой счет а начать играть на деньги. Большинство сайтов предлагают всевозможные способы пополнения а вывода средств, включая:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило требует от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за закрытыми столами. Данное замечательный способ провести время с людьми, с которыми вы знакомы, и сразиться за звание оптимального игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда ты новичок и желаешь сначала освоиться с правилами, многие сайты дают шанс играть в бесплатный покер. Данное отличный метод поупражняться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если вы выбираешь играть на пк, то большинство покеррумов дают клиенты для компьютеров, которые обеспечивают более комфортный а стабильный игровой процесс.
Заключение: Начните Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть волнующая игра, что может предоставить вам ни просто удовольствие, но и реальные деньги. Подбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, и помните, что главное – есть получать наслаждение от игры!
Ни откладывайте на потом, приступайте твою игру в онлайн покер прямо сейчас! Погрузитесь в мир стратегии, волнения а шансов! Везенья за столами!
Играй на Фактические Деньги со Легкостью! Онлайн покер – является ни просто игра, это целая мир азарта, тактики и возможности заработать реальные деньги, не выходя с квартиры. Когда вы ищете где поиграть в покер в интернете, на русском языке а с выводом денег непосредственно на карту, то вы оказались нужному месту. Данная статья – твой гид в мир онлайн покера в россии.
Почему Онлайн Покер настолько Популярен? Покер – есть классическая карточная игра, которая веками завлекала миллионы людей. Онлайн формат дал ей новую жизнь, сотворив игры открытыми для каждого желающих. Вот только несколько причин популярности онлайн покера:
Удобство а открытость: Играть возможно в любое время а в любом месте, имея только доступ к браузере или мобильному устройству. Обширный выбор: Покеррумы предлагают различные виды покера, варианты турниров а уровни ставок. Играть с реальными людьми: Ты можете бороться с реальными соперниками со всего мира, а ни с компьютерными ботами. Возможность выигрывать реальные деньги: Играть на деньги – является ни просто волнение, но также шанс пополнить твой бумажник. Социальный аспект: Возможно играть с друзьями или заводить свежие знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть множество сайты и покеррумы, предлагающих игры в онлайн покер. Но как подобрать оптимальный среди них? Мы поможем тебе разобраться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, учитывая такие критерии:
Наличие русского языка: Интерфейс и поддержка обязаны быть на русском языке. Возможность играть на рубли: Депозиты а вывод средств должны быть открыты в рублях. Многообразие игр и турниров: Широкий выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Надежность а репутация: Безопасность транзакций а честность игры. Топ румов постоянно обновляется, поэтому рекомендуем следить за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если ты новичок, тогда следует обратить внимание на покеррумы с минимальным депозитом. Данное даст возможность вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы выбранный вами рум есть допущенным в россии, для того чтобы не допустить вероятных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты определились с покеррумом а выбрали игру, ты способен положить свой счет и начать играть на деньги. Большинство сайтов дают всевозможные способы пополнения и вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту обычно требует от нескольких часов до нескольких дней, в зависимости от рума а выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают шанс играть с друзьями за приватными столами. Это отличный способ провести время с людьми, с которыми ты знакомы, а побороться за титул оптимального игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок а хотите вначале привыкнуть с правилами, многие сайты дают шанс играть в бесплатный покер. Данное отличный метод поупражняться и набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Когда ты предпочитаете играть на пк, тогда большинство покеррумов дают программы для компьютеров, которые гарантируют намного комфортный а надежный игровой процесс.
Заключение: Приступайте Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это захватывающая игра, что может предоставить тебе ни просто удовольствие, а также реальные деньги. Подбирайте надежные сайты и румы, участвуйте в турнирах, играйте с друзьями и людьми со всего мира, и помните, что главное – это испытывать удовольствие от игры!
Не откладывайте на потом, начните свою игру в онлайн покер прямо сейчас! Погрузитесь в мир тактики, волнения и шансов! Везенья за столами!
Действуй на Настоящие Деньги со Легкостью! Онлайн покер – является не просто игра, это целая вселенная азарта, тактики и шанса заработать реальные деньги, не покидая с дома. Если вы пытаетесь где поиграть в покер в интернете, на русском языке и с выводом денег непосредственно на карту, то вы оказались по адресу. Данная публикация – ваш проводник в мир онлайн покера в россии.
Почему Онлайн Покер так Известен? Покер – это классическая карточная игра, которая целые столетия завлекала миллионы людей. Онлайн формат принес ей новую жизнь, сделав игры открытыми для каждого желающих. Вот только несколько оснований популярности онлайн покера:
Комфорт а доступность: Играть можно в любое время и в каком угодно месте, имея лишь подключение к браузере либо мобильному устройству. Обширный выбор: Покеррумы обеспечивают различные виды покера, форматы турниров а уровни ставок. Играть с реальными людьми: Ты можете соревноваться с реальными соперниками со всего мира, а ни с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – является ни только волнение, но и возможность наполнить свой бумажник. Социальный аспект: Можно играть с друзьями или заводить новые знакомства с людьми, разделяющими твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть большое количество сайты и покеррумы, дающих игры в онлайн покер. Но каким образом выбрать оптимальный среди них? Мы окажем помощь вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы организовали рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс и поддержка обязаны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть доступны в рублях. Разнообразие игр и турниров: Широкий выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие выгодных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Безопасность а авторитет: Безопасность транзакций а честность игры. Топ румов регулярно обновляется, из-за этого советуем наблюдать за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда вы новичок, тогда следует обратить внимание на покеррумы с минимальным депозитом. Это даст возможность вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, чтобы подобранный тобой рум является разрешенным в россии, для того чтобы не допустить вероятных проблем с доступом а выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом и подобрали игру, ты способен пополнить твой счет а начать играть на деньги. Большая часть сайтов дают различные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило требует от нескольких часов до нескольких дней, в зависимости от рума и выбранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают шанс играть с друзьями за приватными столами. Данное замечательный способ уделить время с людьми, с которыми вы знакомы, и побороться за звание оптимального игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Когда вы новичок а желаешь вначале привыкнуть с правилами, многие сайты дают возможность играть в бесплатный покер. Это отличный метод потренироваться и набраться опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Когда вы выбираешь играть на пк, то большинство покеррумов дают программы для компьютеров, которые обеспечивают намного удобный а стабильный игровой процесс.
Заключение: Начните Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть волнующая игра, что способна предоставить вам не просто наслаждение, а также реальные деньги. Подбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, а знайте, что главное – это получать наслаждение от игры!
Не отсрочивайте на потом, приступайте твою игру в онлайн покер прямо сейчас! Окунитесь в мир тактики, волнения и шансов! Везенья за столами!
Играй за Фактические Деньги с Удобством! Онлайн покер – это не только игра, данное целая мир волнения, тактики и шанса выиграть реальные деньги, без покидая с дома. Если вы пытаетесь где поиграть в покер в интернете, на русском языке а с выводом денег непосредственно на карту, значит ты попали нужному месту. Эта статья – ваш гид в мир онлайн покера в россии.
Почему Онлайн Покер настолько Популярен? Покер – это традиционная карточная игра, что целые столетия привлекала миллионы людей. Онлайн формат принес этой новую жизнь, сотворив игры открытыми для каждого желающих. Здесь лишь несколько оснований популярности онлайн покера:
Комфорт и открытость: Играть можно в всякое время а в каком угодно месте, имея только подключение к браузере или мобильному устройству. Большой выбор: Покеррумы обеспечивают различные виды покера, варианты турниров а уровни ставок. Играть с реальными людьми: Ты можете бороться с реальными соперниками со целого мира, а не с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – является не только волнение, а также возможность наполнить твой кошелек. Социальный аспект: Можно играть с друзьями либо заводить свежие знакомства с людьми, разделяющими ваш интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть множество сайты и покеррумы, предлагающих игры в онлайн покер. Но как выбрать оптимальный из них? Мы поможем вам разобраться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, беря во внимание такие критерии:
Наличие русского языка: Интерфейс а обслуживание обязаны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть открыты в рублях. Разнообразие игр и турниров: Обширный выбор столов и турниров для разных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Надежность и репутация: Безопасность транзакций а честность игры. Топ румов регулярно обновляется, из-за этого советуем следить за свежими обзорами а отзывами игроков.
Особое Внимание на Минимальный Депозит:
Если вы новичок, тогда следует обратить внимание на покеррумы с минимальным депозитом. Данное даст возможность вам начать играть на реальные деньги без больших финансовых вложений.
Разрешенные Покеррумы:
Проверьте, чтобы выбранный вами рум есть допущенным в россии, для того чтобы не допустить возможных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как вы решили с покеррумом и подобрали игру, ты можете пополнить свой баланс и начать играть на деньги. Большая часть сайтов предлагают различные способы пополнения и вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума а подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы дают возможность играть с друзьями за закрытыми столами. Это отличный способ уделить время с людьми, с которыми вы знакомы, и сразиться за звание лучшего игрока в твоей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок а хотите вначале освоиться с правилами, многие сайты предлагают шанс играть в бесплатный покер. Данное прекрасный способ потренироваться а получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт и Удобство Если ты выбираешь играть на пк, то большинство покеррумов дают программы для компьютеров, что обеспечивают более комфортный и надежный игровой процесс.
Заключение: Приступайте Свой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – это волнующая игра, которая может принести тебе ни только удовольствие, но и реальные деньги. Подбирайте проверенные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со всего мира, а знайте, что главное – есть испытывать удовольствие от игры!
Ни откладывайте на потом, приступайте твою игру в онлайн покер непосредственно сейчас! Окунитесь в мир стратегии, волнения а возможностей! Удачи за столами!
Играй за Реальные Деньги с Комфортом! Онлайн покер – является ни просто игра, это полная мир азарта, стратегии а возможности выиграть реальные деньги, без выходя с дома. Когда вы пытаетесь где поиграть в покер в интернете, на русском языке и с выводом денег прямо на карту, то вы попали нужному месту. Эта статья – твой гид в мир онлайн покера в россии.
Почему Онлайн Покер так Известен? Покер – это традиционная карточная игра, которая веками завлекала миллионы людей. Онлайн формат дал ей новую жизнь, сделав игры доступными для каждого желающих. Здесь лишь пара причин популярности онлайн покера:
Удобство а открытость: Играть можно в любое время а в каком угодно месте, обладая только подключение к браузере либо мобильному устройству. Обширный выбор: Покеррумы дают разнообразные виды покера, варианты турниров и уровни ставок. Играть с реальными людьми: Ты можете соревноваться с реальными соперниками со всего мира, а не с компьютерными ботами. Шанс выигрывать реальные деньги: Играть на деньги – является не просто азарт, но и шанс наполнить свой бумажник. Социальный аспект: Возможно играть с друзьями либо заводить свежие знакомства с людьми, разделяющих твой интерес. Где Играть в Онлайн Покер в россии на Реальные Деньги? В россии есть множество сайты и покеррумы, предлагающих игры в онлайн покер. Однако каким образом подобрать лучший среди них? Мы окажем помощь тебе сориентироваться:
Популярные Покеррумы для Русских Игроков:
Мы составили рейтинг лучших покеррумов для русском языке игроков, беря во внимание следующие критерии:
Наличие русского языка: Интерфейс а поддержка должны быть на русском языке. Возможность играть на рубли: Депозиты и вывод средств должны быть открыты в рублях. Многообразие игр и турниров: Большой выбор столов и турниров для всевозможных уровней игроков. Наличие мобильного приложения: Возможность скачать приложение для андроид и играть с мобильного устройства. Наличие прибыльных бонусов: Бездепозитным бонусом за регистрацию, начальным капиталом, бонусы на депозит а другие акции. Надежность а репутация: Безопасность транзакций а честность игры. Топ румов регулярно обновляется, поэтому рекомендуем следить за актуальными обзорами и отзывами игроков.
Особое Внимание на Минимальный Депозит:
Когда вы новичок, то следует обратить внимание на покеррумы с минимальным депозитом. Данное даст возможность тебе начать играть на реальные деньги без крупных финансовых вложений.
Разрешенные Покеррумы:
Убедитесь, что выбранный тобой рум есть разрешенным в россии, для того чтобы не допустить вероятных проблем с доступом и выводом средств.
Онлайн Покер с Выводом Денег на Карту: Как Это Работает? После того, как ты решили с покеррумом а подобрали игру, вы можете положить свой счет и начать играть на деньги. Большинство сайтов дают различные способы пополнения а вывода средств, в том числе:
Банковские карты (Visa, Mastercard) Электронные кошельки Другие платежные системы Вывод денег на карту как правило занимает от нескольких часов до нескольких дней, в зависимости от рума и подобранного способа вывода.
Как Играть в Онлайн Покер с Друзьями? Многие покеррумы предлагают возможность играть с друзьями за закрытыми столами. Это замечательный метод провести время с людьми, с которыми вы знакомы, и сразиться за звание лучшего игрока в вашей компании.
Бесплатный Покер: Возможность Попробовать Свои Силы Если ты новичок и хотите сначала освоиться с правилами, многие сайты предлагают шанс играть в бесплатный покер. Данное отличный способ поупражняться и получить опыта перед тем, как начать играть на реальные деньги.
Покер на пк: Комфорт а Удобство Когда вы предпочитаете играть на пк, тогда большинство покеррумов дают клиенты для компьютеров, которые гарантируют намного удобный и надежный игровой процесс.
Заключение: Приступайте Твой Путь в Мир Онлайн Покера Сегодня! Онлайн покер – есть захватывающая игра, которая может предоставить вам ни просто удовольствие, но и реальные деньги. Выбирайте надежные сайты и румы, принимайте участие в турнирах, играйте с друзьями и людьми со целого мира, а помните, что главное – это испытывать удовольствие от игры!
Ни отсрочивайте на потом, приступайте твою игру в онлайн покер прямо сейчас! Погрузитесь в мир тактики, волнения и возможностей! Удачи за столами!
Круглосуточная поставка спиртного в Москве: удобство либо задача?
Столица – мегаполис, который никогда никак спит, и для многих ее жителей шанс получить желаемое в всякое время суток явилась обычной. Данное принадлежит и к доставке алкоголя, которая, невзирая на ее спорность, надежно вошла в повседневную бытие горожан. Однако ли так всё элементарно, как видится на первого раза?
Каким образом это функционирует?
24-часовая поставка спиртного в Москве осуществляется посредством многообразные сервисы:
Интернет-сервисы: Специализированные сайты и приложения, что дают широкий выбор алкогольных продуктов с доставкой в квартиру. Заведения общепита и бары: Некоторые заведения, имеющие разрешение на продажу спиртного, предлагают поставку их продукции в ночное время суток. Доставщики службы: Компании, которые сотрудничают с лицензированными реализаторами спиртного и осуществляют поставку по запросу. Преимущества:
Комфорт: Возможность приобрести излюбленный продукт, не выходя из дома, в любое время суток. доставка алкоголя в москве круглосуточно Сбережение времени: Не требуется тратить время на визит в маркет, особо в позднее время. Широкий выбор: Обширный ассортимент спиртных напитков, включая необычные и эксклюзивные позиции. Шанс для праздников и событий: Быстрая поставка позволяет быстро пополнить запасы спиртного, когда это необходимо. Минусы и противоречия:
Правомерность: В России запрещена реализация спиртного в вечернее время (от 23:00 до 8:00). Службы поставки, что предоставляют круглосуточную доставку, обычно применяют всевозможные способы, что могут быть противозаконными. Потребление алкоголя: Легкий доступ к алкоголю в всякое время может способствовать росту потребления, что способен повлечь отрицательные последствия для здоровья. Проверка за продажей несовершеннолетним: Имеется риск, что курьеры могут не проверять лета покупателей, что способен привести к реализации алкоголя не достигшим совершеннолетия.
24-часовая доставка алкоголя в Москве: удобство или проблема?
Столица – город, что никогда никак спит, а ради большинства его жителей возможность получить желаемое в любое время суток стала обычной. Это принадлежит и к доставке спиртных напитков, которая, невзирая на ее спорность, прочно вошла в обыденную бытие горожан. Однако ли так все просто, словно видится с первого взгляд?
Как это работает?
24-часовая доставка алкоголя в Москве производится посредством многообразные сервисы:
Интернет-сервисы: Специализированные сайты и программы, что дают обширный ассортимент спиртных напитков с поставкой в квартиру. Заведения общепита и пабы: Некоторые заведения, обладающие лицензию на реализацию спиртного, предоставляют поставку их товаров в ночное время суток. Доставщики службы: Фирмы, которые взаимодействуют с лицензированными продавцами алкоголя и проводят поставку по требованию. Преимущества:
Удобство: Возможность приобрести излюбленный продукт, не выходя из жилища, в всякое время суток. доставка алкоголя в москве Сбережение времени: Не требуется тратить часы на поход в маркет, особо в вечернее время. Большой ассортимент: Большой выбор спиртных продуктов, в том числе редкие и эксклюзивные предложения. Возможность для праздников и событий: Быстрая поставка позволяет оперативно пополнить резервы спиртного, если это необходимо. Минусы и противоречия:
Законность: В РФ не разрешена продажа спиртного в ночное время (с 23:00 до 8:00). Сервисы поставки, которые предоставляют 24-часовую поставку, часто используют различные схемы, которые могут быть незаконными. Потребление спиртного: Простой получение к алкоголю в любое время может помогать увеличению употребления, что способен повлечь негативные результаты на здоровья. Проверка над реализацией несовершеннолетним: Имеется опасность, что доставщики способны не контролировать лета клиентов, что способен привести к продаже спиртного не достигшим совершеннолетия.
покер онлайн на деньги с различными возможностями общения
покер онлайн на деньги с различными стратегиями и тактиками
покер онлайн бесплатная игра с рейтингом
Рейтинг казино честных
Рейтинг казино официальные
Рейтинг казино играть на деньги
Топ рейтинг казино
Рейтинг казино для новичков
Топ рейтинг казино
Рейтинг казино с турнирами
Рейтинг мобильных казино
Рейтинг казино с крупными бонусами
Рейтинг казино с большим выбором игр
Спасибо вам!
Предлагаю вам русские криминальные сериалы – это настоящее искусство, которое покоряет сердца зрителей по всему миру. Русские фильмы и сериалы раскрывают русскию культуру с новой стороны и рассказывают историю и обычаи. В настоящее время смотреть русские фильмы и сериалы онлайн стало очень просто за счет большого числа источников. От ужасов до комедий, от исторических фильмов до современных детективов – выбор безграничен. Погрузитесь в захватывающие сюжеты, профессиональную актерскую работу и красивую работу оператора, наслаждаясь кинематографией из России прямо у себя дома.
Рейтинг казино лицензионные
Рейтинг лучших онлайн казино
Рейтинг казино по честности
Рейтинг казино с быстрыми выплатами
Рейтинг казино официальные
Рейтинг казино надежных
Рейтинг казино топ 10
Рейтинг казино с быстрым выводом
Спасибо за информацию.
Предлагаю вам русские криминальные сериалы – это настоящее искусство, которое любят не только в России, но и во всем мире. Они предлагают уникальный взгляд на русскую культуру, историю и обычаи. Сегодня смотреть русские фильмы и сериалы онлайн стало очень просто благодаря различным платформам и сервисам. От драм до боевиков, от исторических фильмов до фантастики – выбор безграничен. Окунитесь в захватывающие сюжеты, талантливые актерские исполнения и красивую работу оператора, наслаждаясь кинематографией из России не выходя из дома.
Рейтинг казино без обмана
Жаждете захватывающих впечатлений и крупных побед? Надоели от нечестных казино и постоянных поражений? В этом случае приветствуем вас в мир надежных и гарантированных виртуальных казино!
Мы понимаем, насколько важно отдавать предпочтение надежные азартные платформы. По этой причине мы разработали канал в Telegram: https://t.me/s/top_rus_casino, в котором рассказываем о рейтингами лучших клубов с безупречной славой.
В нашем канале вы сможете найти исключительно проверенную сведения о казино, которые дают справедливый игровой процесс, оперативные обналичивание выигрышей и непрерывную обслуживание пользователей. Наша команда тщательно анализируем каждое игровое заведение по многочисленным параметрам, таким как: разрешение, отзывы игроков, ассортимент развлечений, акции и другие факторы.
Вступайте к нашему единомышленному сообществу фанатов азартных игр и будьте в курсе актуальных событий мира интернет-казино! Коллективно мы сможем выбрать идеальное казино для твоего захватывающего развлекательного путешествия!
Желаете найти наиболее прибыльные предложения азартных клубов без каких-либо вложений? В таком случае вы зашли именно куда нужно!
Ртот Telegram-канал @top_rating_casino – это настоящий источник данных Рѕ бесплатных поощрениях РІ лучших онлайн казино. Команда каждый день анализируем рынок РёРіРѕСЂРЅРѕРіРѕ бизнеса, СЃ целью обеспечить тебе актуальные Рё интересные предложения.
Оставьте о долгом поиске действующих промокодов и бонусов. У нашем канале ты найдете все нужное в одном месте – прямые ссылки на игровые платформы, детальные описания предложений, и кучу другой важной сведений, которая поможет тебе получить прибыль без внесения каких-либо депозитов.
Присоединяйтесь на наш Telegram-канал @top_rating_casino немедленно и приступайте свой путешествие в вселенную гемблинга с опасности вашего денег! Не пропустите свой шанс сорвать значительный куш!
Забудьте относительно о блокировках: Отражение игрового клуба – ваш персональный постоянный подход до любимым развлечениям!
В мире онлайн-казино наличие – это основной фактор. Увы, ограничения веб-сайтов, ограничения доступа, а также технические сбои в состоянии помешать вам получить удовольствие от азартными играми или получить ожидаемый выигрыш. Но всё же не стоит стоит терять надежду! **Топ Рейтинг Казино** Есть легкое и действенное разрешение – зеркало казино.
Что такое такое отражение казино и зачем оно вам нужно?
Зеркало казино – это абсолютная копия официального веб-сайта, расположенная на другому интернет-адресу (URL). Оно создается для гарантии постоянного доступа к играм а также функциям казино, даже если главный сайт ограничен интернет-провайдером или нефункционален по техническим обстоятельствам.
Плюсы применения отражения игрового клуба:
Непрерывный РїРѕРґС…РѕРґ: Рграйте РЅР° обожаемые слоты, вращающееся колесо, покер Рё РґСЂСѓРіРёРµ РёРіСЂС‹ РІ любое время суток Рё СЃ РІСЃСЏРєРѕР№ точки РјРёСЂР°. Забудьте РїСЂРѕ надоедливых блокировках! Полная работоспособность: Отражение РёРіСЂРѕРІРѕРіРѕ клуба предлагает РІСЃРµ точно такие Р¶Рµ самые функции, что даже главный веб-сайт: зачисление счета, снятие денег, присутствие РЅР° акциях Рё ещё турнирах, общение СЃ поддержкой помощи. Безопасность: Войдите РїРѕРґ СЃРІРѕРёРј логином Р° также ключом, как обычно. Отражения казино используют точно такие Р¶Рµ Р¶Рµ алгоритмы шифрования, как даже главный веб-сайт, гарантируя защиту ваших информации Рё средств. Автоматическое транспортировка: Некоторые игровые клубы самостоятельно транспортируют пользователей Рє действующее отражение РІРѕ период недоступности РѕСЃРЅРѕРІРЅРѕРіРѕ веб-сайта. Как обнаружить действующее зеркало казино?
Существует пара способов найти последнее зеркало твоего избранного казино:
Оформите РїРѕРґРїРёСЃРєСѓ РЅР° новостную рассылку казино: РњРЅРѕРіРёРµ казино присылают СЃРІРѕРёРј подписчикам информацию РѕР± последних зеркалах РІРѕ период ограничения главного веб-сайта. Свяжитесь РІ службу помощи: Наиболее надежный СЃРїРѕСЃРѕР± – связаться РІ отдел помощи РёРіСЂРѕРІРѕРіРѕ клуба РїРѕ электронную почту или интерактивный чат. РћРЅРё РІСЃРµ всегда дадут вам актуальный адрес рабочего зеркала. Найдите сведения РЅР° надёжных веб-сайтах СЃ обзорами: Рмеются профильные сайты, которые обнародуют последнюю сведения Рѕ действующих зеркалах популярных онлайн-казино. Удостоверьтесь, будто ресурс надежный Р° также проверенный. Важно:
Будьте бдительны мошенников! Применяйте лишь надёжные источники информации о зеркалах казино. Ни разу не следует вводите свои личные данные на сомнительных сайтах. Удостоверяйтесь веб-адрес зеркала: Убедитесь, что адрес отражения похож на офицальный адрес казино. Заключение:
Зеркало казино – это есть твой надежный вариант пребывать РІ РёРіСЂРµ Рё РЅРµ упустить РёР· РІРёРґСѓ шанс РЅР° крупный победу. Рспользуйте его РІ собственных интересах, чтобы наслаждаться обожаемыми развлечениями РЅРµ имея лимитов или ограничений! РќРµ РЅСѓР¶РЅРѕ забудьте сохранять актуальный веб-адрес действующего зеркала Рё ещё ставить осмотрительно.
Рейтинг виртуальных игровых платформ: Самые площадки для тебя!
Мечтаете надежное пространство, где бы можно ощутить драйв и одновременно получить приз? В этом случае вы пришли куда нужно! Наши список самых интернет игровых платформ поспособствует тебе совершить верный отбор!
Наша команда тщательно проанализировали множество различных гемблинг сайтов, учитывая определенные показатели:
Разрешение Рё регуляция: Доверие – превыше всего! Наша команда выбираем исключительно лицензированные заведения, которые дают честную РёРіСЂСѓ.
Ассортимент игр: От стандартных слотов вплоть до инновационных живых игр с дилерами. В в вашем есть все самое лучшее!
Бонусы и системы привилегий: Выгодные приветственные предложения, регулярные мероприятия и многоступенчатая система вознаграждений для постоянных клиентов.
Простота работы: Легко доступный интерфейс, мгновенная регистрация и практичные способы пополнения и снятия денег.
Служба поддержки поддержки: Постоянная консультация клиентов через чат, электронную почту и телефон. Мгновенные ответы на ваши запросы.
Не прозевайте попытку узнать о топовых онлайн казино прямо сейчас! Переходите в наш telegram канал, где игроков ждут эксклюзивные рейтинги, новости игровые и много актуальной инфы: https://t.me/s/top_rus_casino
Подключайтесь к нашей тусовке везучих игроков и запускайте побеждать уже сегодня!
Хотите открыть для себя атмосферу волнения развлечений? Если да, ваше необходимо обратить внимание на обзором топ популярных онлайн платформ!
Рксперты подготовили детальный РѕР±Р·РѕСЂ РёРіСЂРѕРІРѕР№ индустрии, СЃ целью показать вам только надежные Рё отличные виртуальные заведения.
В данном обзоре вы найдете:
Объективные обзоры пользователей.
Детальные характеристики игровых автоматов от ведущих производителей.
Рнформацию Рѕ акциях Рё условиях преимуществ.
Оценки финансовых систем и скорости получения денег.
Рейтинги игровых клубов в соответствии с нескольким критериям (к примеру, топовые казино для начинающих, заведения с большими бонусами, платформы с оперативными обналичиванием).
Обязательно случай окунуться в атмосферу крупных побед и интересных эмоций!
Присоединяйтесь в Telegram каналу и оказывайтесь в центре всех топ новостей из мира онлайн казино! Там вы найдете последние обзоры, акции, и кроме того особенные варианты и много другого увлекательного!
Кликайте по адресу: https://t.me/s/top_rus_casino и стартуйте ваше приключение в атмосферу значительных выигрышей!
Приветствуем! каждого фанатов захватывающих развлечений и тех, кто ищет лучшие онлайн-казино! Вы мечтали о моменте, чтобы испытывать удачу лишь в авторитетных и лицензированных игорных домах? Тогда вы попали именно куда следует!
Рксперты создали для вас уникальный СЃРїРёСЃРѕРє самых топовых сотни игровых платформ, представленных РїСЂСЏРјРѕ сейчас. Предлагаемый топ построен РЅР° глубоком анализе разнообразных показателей, принимая РІРѕ внимание известность РёРіРѕСЂРЅРѕРіРѕ РґРѕРјР°, присутствие лицензии, оперативность выплат, уровень сопровождения клиентов, широкий выбор развлечений Рё щедрость акций.
Еще больше информацию о любом казино из нашего списка вы найдете в специальном телеграм-канале: https://t.me/s/top_rus_casino. Здесь эксперты делимся свежими новостями из сферы захватывающих игр, оценками казино, ценными рекомендациями и стратегиями, которые помогут вам лично повысить личные вероятность на победу!
Присоединяйтесь к нам сейчас же и будьте в центре самых выгодных предложений и шансов в индустрии интернет- игорных заведений! Не теряйте свой шанс сорвать огромный приз и получить удовольствие настоящим кайфом!
Рейтинг виртуальных игровых платформ: Самые сайты для вас!
Рщете проверенное пространство, РІ котором получится ощутить удовольствие Рё сорвать РєСѓС€? Р’ этом случае вам повезло РєСѓРґР° РЅСѓР¶РЅРѕ! Наш рейтинг лучших интернет казино поспособствует тебе совершить правильный отбор!
Команда внимательно изучили огромное количество различных гемблинг сайтов, учитывая определенные показатели:
Разрешение Рё регуляция: Надежность – превыше всего! Наша команда выбираем исключительно лицензированные платформы, которые обеспечивают прозрачную РёРіСЂСѓ.
Коллекция слотов: Начиная с классических слотов вплоть до современных live игр. В нашем есть большинство самое захватывающее!
Акции и схемы лояльности: Щедрые стартовые предложения, постоянные акции и многоуровневая система лояльности для верных игроков.
Простота работы: Легко простой интерфейс, мгновенная процесс и удобные методы ввода и обналичивания выигрышей.
Техническая помощи: Постоянная консультация клиентов через чат, электронную почту и телефон. Мгновенные ответы на ваши запросы.
Не прозевайте шанс узнать о лучших виртуальных азартных заведениях прямо сейчас! Переходите на наш telegram канал, где тебя ждут уникальные рейтинги, новости игровые и много интересной информации: https://t.me/s/top_rus_casino
Подключайтесь в сообществу успешных игроков и начинайте побеждать сейчас сегодня!
Появление веб-сайта вверх верхние позиции Yandex: Квалифицированное SEO раскрутка у профессионалов
Хотите, чтобы ваш сайт находился лидирующую место в выдаче поиска Yandex? В таком разе вам нужно экспертное продвижение ресурса в сети. Мы предоставляем всесторонние сервисы SEO и раскрутки сайтов, созданные особо для достижения оптимальной эффективности.
Наши эксперты задействуют только белые SEO способы чтобы гарантированного вывода вашего ресурса в верхние позиции искательных машин. seo продвижение Мы рассматриваем ваш ресурс, оптимизируем его для стандарты Yandex, создаём действенную стратегию продвижения и активируем профессиональную кампанию для получения целевой аудитории.
Оставьте о упущенных покупателях! Закажите SEO продвижение ресурса в нашей компании, и Ваш компания возымеет значительный толчок развития. Мы способствуем Вам опередить конкурентов и занять лидирующие места в онлайне.
Что мы предлагаем:
Всесторонний SEO аудит сайта.
Улучшение сайта для Яндекса.
Эффективную продвижение ресурса.
Создание плана продвижения в сети.
Регулировку и управление медийной кампании.
Поднятие веб-сайта в верхние позиции и на первую страницу поисковой ленты.
Увеличьте прибыль Вашего бизнеса – обратитесь профессиональное раскрутку веб-сайта немедленно в данный момент! Наша задача – вывести Ваш сайт в верхние позиции! Приобретите отличные услуги по SEO и сделайте веб-сайт в эффективный средство продаж. Мы знаем, как поместить Ваш веб-сайт на первую страницу в Яндексе!
Вывод сайта на лидирующие места Яндекса: Квалифицированное SEO раскрутка у специалистов
Желаете, чтобы Ваш веб-сайт располагался первую место в ответах искателя Yandex? В этом случае Вам требуется профессиональное продвижение веб-сайта в сети. Мы даём комплексные услуги SEO и продвижения веб-сайтов, спроектированные конкретно чтобы получения оптимальной продуктивности.
Наши профессионалы задействуют только легальные SEO методы чтобы уверенного вывода вашего веб-сайта в верхние позиции искательных машин. реклама сайта Мы рассматриваем ваш веб-сайт, налаживаем его для стандарты Яндекса, разрабатываем действенную план оптимизации и запускаем компетентную рекламу чтобы привлечения целевой публики.
Оставьте об потерянных заказчиках! Обратитесь SEO раскрутку ресурса в нашей компании, и Ваш дело приобретёт значительный подъём развития. Мы поможем Вам превзойти соперников и занять первые места в онлайне.
Что мы предлагаем:
Всесторонний SEO аудит сайта.
Настройку сайта под Yandex.
Эффективную оптимизацию сайта.
Создание стратегии оптимизации в интернете.
Настройку и ведение контекстной рекламы.
Вывод сайта в ТОП и на первую позицию искательной результатов.
Увеличьте прибыль вашего дела – обратитесь экспертное оптимизацию веб-сайта немедленно сейчас! Наша цель – поднять ваш сайт в верхние позиции! Получите качественные услуги по SEO и сделайте веб-сайт в мощный инструмент реализации. Мы знаем, каким образом поднять ваш веб-сайт на лидирующую страницу в Yandex!
Поднятие сайта в ТОП Яндекса: Квалифицированное SEO раскрутка у экспертов
Хотите, чтоб Ваш ресурс находился лидирующую страницу в ответах поиска Яндекса? Тогда вам необходимо качественное оптимизация сайта в сети. Наша компания даём комплексные услуги SEO и оптимизации веб-сайтов, разработанные особо для достижения наибольшей продуктивности.
Наши профессионалы применяют только легальные SEO техники для обеспеченного вывода Вашего ресурса в ТОП искательных машин. интернет продвижение Наша команда изучаем Ваш ресурс, налаживаем его для критерии Яндекса, создаём действенную план раскрутки и активируем грамотную кампанию чтобы привлечения целевой публики.
Оставьте о потерянных заказчиках! Закажите SEO раскрутку сайта в нашей компании, и ваш дело возымеет значительный подъём развития. Мы способствуем Вам превзойти конкурентов и занять ведущие позиции в сети.
Что мы предлагаем:
Полный SEO проверку веб-сайта.
Оптимизацию сайта под Яндекса.
Эффективную продвижение сайта.
Создание стратегии продвижения в онлайне.
Регулировку и ведение таргетированной кампании.
Поднятие веб-сайта в верх и на первую страницу искательной выдачи.
Приумножьте прибыль Вашего компании – закажите профессиональное оптимизацию сайта сразу сейчас! Наша задача – вывести ваш ресурс в верхние позиции! Возьмите качественные сервисы по SEO и сделайте веб-сайт в мощный средство сбыта. Мы знаем, как вывести Ваш веб-сайт на первую позицию в Yandex!
Появление веб-сайта вверх лидирующие места Yandex: Экспертное SEO продвижение из специалистов
Хотите, чтобы ваш веб-сайт располагался верхнюю страницу в выдаче искателя Yandex? В этом случае Вам требуется качественное продвижение ресурса в сети. Мы даём всесторонние сервисы SEO и продвижения ресурсов, созданные конкретно для достижения максимальной результативности.
Наши профессионалы применяют лишь легальные SEO способы для обеспеченного вывода вашего веб-сайта в ТОП поисковых машин. интернет продвижение Мы изучаем Ваш ресурс, подстраиваем его для требования Yandex, разрабатываем результативную стратегию раскрутки и стартуем профессиональную рекламу для захвата целевой публики.
Забудьте о упущенных покупателях! Воспользуйтесь SEO раскрутку сайта в нас, и Ваш дело возымеет мощный подъём движения. Мы поможем Вам обойти конкурентов и занять ведущие позиции в сети.
Что мы предлагаем:
Комплексный SEO аудит ресурса.
Улучшение ресурса для Yandex.
Эффективную продвижение ресурса.
Создание стратегии продвижения в сети.
Коррекцию и ведение таргетированной кампании.
Появление веб-сайта в ТОП и на первую позицию поисковой результатов.
Повысьте прибыль Вашего компании – воспользуйтесь экспертное раскрутку веб-сайта сразу в данный момент! Наша задача – поднять ваш веб-сайт в верхние позиции! Получите хорошие сервисы по SEO и превратите веб-сайт в сильный инструмент продаж. Мы осведомлены, каким образом поместить Ваш веб-сайт на лидирующую позицию в Яндексе!
Появление веб-сайта вверх верхние позиции Yandex: Квалифицированное SEO раскрутка у экспертов
Хотите, чтоб ваш веб-сайт располагался первую позицию в результатах поиска Яндекса? В таком разе вам нужно экспертное раскрутка ресурса в онлайне. Мы даём полные сервисы SEO и раскрутки сайтов, спроектированные конкретно чтобы достижения наибольшей эффективности.
Наши профессионалы применяют лишь легальные SEO техники чтобы обеспеченного вывода вашего веб-сайта в верхние позиции поисковых машин. продвижение в интернете Мы анализируем ваш веб-сайт, налаживаем его под требования Яндекса, создаём действенную стратегию раскрутки и запускаем грамотную рекламу чтобы захвата потенциальной публики.
Оставьте об утраченных заказчиках! Обратитесь SEO оптимизацию веб-сайта у нас, и Ваш дело получит сильный подъём развития. Мы способствуем Вам превзойти конкурентов и занять ведущие позиции в интернете.
Что мы предлагаем:
Комплексный SEO анализ веб-сайта.
Настройку сайта под Yandex.
Действенную раскрутку ресурса.
Проектирование плана раскрутки в интернете.
Регулировку и ведение контекстной рекламы.
Появление сайта в ТОП и на первую позицию поисковой ленты.
Приумножьте доход Вашего дела – воспользуйтесь качественное оптимизацию веб-сайта немедленно в данный момент! Наша задача – вывести Ваш веб-сайт в верхние позиции! Приобретите качественные услуги по SEO и сделайте сайт в эффективный средство реализации. Мы осведомлены, как вывести Ваш ресурс на верхнюю страницу в Яндексе!
Поднятие сайта вверх ТОП Yandex: Профессиональное SEO раскрутка от специалистов
Хотите, чтобы ваш ресурс располагался верхнюю позицию в выдаче поиска Яндекса? В этом случае вам нужно экспертное оптимизация ресурса в интернете. Мы предлагаем полные услуги SEO и раскрутки ресурсов, разработанные особо для достижения наибольшей эффективности.
Наши профессионалы используют только честные SEO методы чтобы гарантированного вывода Вашего сайта в верхние позиции искательных систем. seo продвижение Наша команда рассматриваем Ваш ресурс, налаживаем его под критерии Yandex, проектируем результативную план продвижения и стартуем компетентную рекламу чтобы привлечения целевой посетителей.
Забудьте об упущенных клиентах! Воспользуйтесь SEO продвижение веб-сайта в нас, и ваш дело получит значительный импульс развития. Мы содействуем вам опередить соперников и занять ведущие позиции в онлайне.
Что мы предлагаем:
Всесторонний SEO аудит веб-сайта.
Настройку веб-сайта под Yandex.
Действенную продвижение ресурса.
Проектирование плана оптимизации в интернете.
Регулировку и управление медийной рекламы.
Поднятие ресурса в верх и на лидирующую страницу поисковой результатов.
Повысьте прибыль Вашего бизнеса – закажите экспертное оптимизацию веб-сайта прямо в данный момент! Наша задача – вывести Ваш ресурс в верхние позиции! Возьмите отличные сервисы по SEO и превратите сайт в эффективный инструмент продаж. Мы понимаем, каким образом вывести Ваш сайт на первую позицию в Yandex!
Поднятие ресурса в верхние позиции Яндекса: Экспертное SEO продвижение у экспертов
Хотите, чтоб ваш сайт находился верхнюю позицию в выдаче поиска Яндекса? В этом случае вам требуется качественное раскрутка ресурса в онлайне. Мы предоставляем комплексные услуги SEO и продвижения сайтов, разработанные особо чтобы достижения оптимальной продуктивности.
Наши специалисты задействуют лишь белые SEO методы для гарантированного вывода вашего сайта в верхние позиции поисковых систем. сео продвижение сайта Наша команда анализируем Ваш ресурс, подстраиваем его для стандарты Yandex, создаём результативную стратегию продвижения и запускаем профессиональную кампанию для захвата потенциальной публики.
Избавьтесь об упущенных клиентах! Обратитесь SEO раскрутку ресурса в нас, и Ваш компания возымеет сильный толчок развития. Мы содействуем вам обойти конкурентов и занять первые места в интернете.
Что мы предлагаем:
Всесторонний SEO проверку сайта.
Настройку веб-сайта под Яндекса.
Результативную раскрутку ресурса.
Создание плана оптимизации в сети.
Регулировку и ведение таргетированной кампании.
Появление веб-сайта в верх и на первую позицию искательной ленты.
Приумножьте выгоду вашего компании – обратитесь профессиональное оптимизацию сайта сразу сейчас! Наша задача – поместить Ваш веб-сайт в верхние позиции! Получите отличные услуги по SEO и сделайте сайт в эффективный средство реализации. Мы знаем, как поместить Ваш сайт на лидирующую страницу в Яндексе!
Поднятие ресурса вверх верхние позиции Яндекса: Квалифицированное SEO продвижение у профессионалов
Желаете, чтобы Ваш ресурс располагался верхнюю место в результатах искателя Yandex? В этом случае вам нужно экспертное продвижение веб-сайта в интернете. Наша компания предоставляем полные услуги SEO и оптимизации сайтов, созданные особо для достижения оптимальной продуктивности.
Наши специалисты применяют лишь белые SEO методы для уверенного вывода Вашего ресурса в лидирующие места искательных систем. сео продвижение сайта Мы изучаем Ваш ресурс, налаживаем его для требования Яндекса, создаём действенную стратегию продвижения и стартуем компетентную кампанию чтобы привлечения потенциальной посетителей.
Избавьтесь о утраченных клиентах! Обратитесь SEO продвижение веб-сайта у нашей компании, и Ваш компания получит мощный импульс развития. Мы способствуем Вам опередить соперников и занять первые позиции в онлайне.
Что мы предлагаем:
Комплексный SEO аудит ресурса.
Настройку веб-сайта под Yandex.
Эффективную оптимизацию ресурса.
Создание плана продвижения в сети.
Коррекцию и сопровождение контекстной рекламы.
Появление ресурса в верхние позиции и на первую страницу поисковой результатов.
Приумножьте выгоду Вашего компании – воспользуйтесь экспертное раскрутку веб-сайта немедленно сейчас! Наша цель – поместить ваш ресурс в топ! Получите отличные услуги по SEO и сделайте ресурс в мощный средство сбыта. Мы понимаем, как поднять Ваш сайт на первую страницу в Yandex!
Появление сайта вверх ТОП Яндекса: Квалифицированное SEO оптимизация от экспертов
Хотите, чтобы Ваш веб-сайт занимал лидирующую место в ответах поиска Яндекса? В таком разе Вам нужно качественное продвижение сайта в интернете. Мы предлагаем всесторонние услуги SEO и раскрутки веб-сайтов, спроектированные специально для достижения максимальной результативности.
Наши профессионалы применяют только честные SEO методы чтобы гарантированного вывода Вашего веб-сайта в лидирующие места поисковых систем. реклама сайта Мы изучаем Ваш веб-сайт, налаживаем его для стандарты Yandex, проектируем эффективную стратегию оптимизации и запускаем профессиональную кампанию чтобы получения целевой посетителей.
Избавьтесь о утраченных клиентах! Закажите SEO раскрутку ресурса в нашей компании, и Ваш бизнес возымеет сильный импульс движения. Мы способствуем Вам превзойти соперников и занять лидирующие позиции в интернете.
Что мы предлагаем:
Всесторонний SEO проверку ресурса.
Оптимизацию веб-сайта под Яндекса.
Результативную продвижение сайта.
Создание стратегии оптимизации в интернете.
Регулировку и сопровождение медийной кампании.
Появление веб-сайта в верхние позиции и на первую страницу искательной ленты.
Увеличьте прибыль вашего дела – воспользуйтесь качественное продвижение ресурса прямо сейчас! Наша задача – поднять Ваш ресурс в верхние позиции! Приобретите хорошие сервисы по SEO и трансформируйте сайт в эффективный инструмент реализации. Мы осведомлены, каким образом поднять ваш ресурс на лидирующую позицию в Yandex!
Хватит играть вслепую! Найди лучшее онлайн казино в 2025 году!
Хочешь играть и выигрывать реальные деньги в интернете, но боишься обмана? Устал искать рабочее зеркало любимого казино? Наш Telegram-канал – твой проводник в мир азарта!
Мы публикуем топ рейтинг лучших, честных и надежных онлайн казино с лицензией! Только проверенная информация о самых популярных платформах!
Что ты найдешь в нашем канале:
Объективный рейтинг онлайн казино на 2025 год.
Обзоры лучших и честных казино, проверенных экспертами.
Актуальные промокоды для получения бонусов.
Информация о бездепозитных бонусах, позволяющих играть бесплатно.
Полезные статьи о том, как найти официальный сайт или зеркало.
Инструкции по входу и регистрации на официальном сайте казино.
Обзоры игровых автоматов (слоты), стратегии игры и шансы сорвать джекпот.
Где скачать официальное приложение казино.
Больше не нужно тратить время на поиски! У нас есть все, что тебе нужно для успешной игры:
Надежные казино, гарантирующие выплаты.
Информация об играх, где можно выиграть реальные деньги.
Топ слоты и другие игровые предложения.
Подписывайся прямо сейчас и получи шанс сорвать джекпот! https://t.me/s/kazino_reyting_top
Не упусти свой шанс! Играй ответственно и только в лучших онлайн казино с нашей помощью!
Зажги свою светило удачи совместно с “Kometa casino”! ?
Салют, искатель приключений! Вы созрел ринуться в галактическое вояж, где каждая точка гарантирует фантастические призы да ошеломляющие эмоции?
В этом случае вам точно стоит подключиться к нашему с вами эксклюзивному каналу в Telegram https://t.me/s/kometacasino17. Здесь вас поджидают не просто игры, но реальные галактические приключения, переполненные неожиданных кульбитов а ярких вспышек удачи!
Отчего избирают именно нас?
Звездные поощрения: Присоединяйся в нашу общую команду а заполучи первоначальный набор плюшек, что поможет вам оперативнее достичь твоей цели. Развлекательные миры: Местные игровые машины – есть настоящие вселенные, кишащие загадками и богатствами. Осваивай все до единой и открой твоей совершенный аппарат! Турнирные вселенные: Вливайся в космических конкурсах а также борись за звание главного игрока космоса. Призовые банки до такой степени масштабны, что аж способны затмить даже и абсолютно блестящую светило! Мгновенная выплата: Лишь только ты достигнешь успеха, личные деньги окажутся тут же переведены на ваш аккаунт. Совершенно никаких промедлений – лишь подлинная радость успеха! Ассистанс всегда на связи: Нашей команды экипаж постоянно на контакте, готовый оказать помощь вам в любой положении. Даже коль вы сбился с пути среди светил, мы посодействуем открыть направление к себе. ?? Как начать? Легко подпишись на данный ресурс и затем отправься в рейс! Впереди вас ожидают бесконечные пределы возможностей и океан наслаждения.
Не стоит упусти минуту, когда твоя огонек засветится блестящее каждого!
#kometa #casino #Бонусы #Победа #CometaCasino #kometacasino
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Looking for expert guidance on protecting assets while qualifying for Medicaid? As experienced elder law attorneys near me, our team at Ohio Medicaid Lawyers provides specialized legal assistance with Medicaid planning, estate planning, and asset protection strategies. We help seniors understand medicaid eligibility income charts and navigate the complex 5-year lookback period. Visit our website for comprehensive information about Ohio medicaid income limits 2024 and schedule a consultation with a trusted elder care attorney who can safeguard your future.
Your article helped me a lot, is there any more related content? Thanks! https://www.binance.com/pt-BR/join?ref=YY80CKRN
Need guidance on handling appreciated assets with significant capital gains during Medicaid planning? Our capital gains attorneys develop tax-efficient strategies that preserve eligibility.
Our elder protection planning includes comprehensive Medicaid strategies to safeguard your financial security and legacy.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
This was very informative. I appreciate the clarity and depth.
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
You may be able to borrow more and enjoy better interest rates by taking out a loan secured on your property. Find the best current offers today.
Наш сервисный центр Bosch в Москве предоставляет квалифицированный ремонт бытовой техники Bosch любой сложности. Наши сертифицированные мастера быстро и качественно устранят любые неисправности вашего оборудования Bosch. Гарантируем эффективный и надежный ремонт вашего оборудования с использованием оригинальных запчастей и современных технологий. Ремонт возможен как на дому у заказчика, так и в нашем сервисном центре. Доверьте заботу о вашей технике опытным специалистам. Заказать [url=https://bosch-servis-moskva.ru/]ремонт духовых шкафов bosch в москве[/url] просто – оставьте заявку на сайте или позвоните.
ремонт аристон сервис аристон
аристон сервисный центр https://remont-ariston-msk.ru
ремонт холодильников аег ремонт стиральной машины aeg
площадка для продажи аккаунтов продать аккаунт
аккаунты с балансом маркетплейс для реселлеров
купить аккаунт аккаунты с балансом
профиль с подписчиками https://ploshadka-prodazha-akkauntov.ru
маркетплейс аккаунтов https://prodat-akkaunt-online.ru/
перепродажа аккаунтов аккаунт для рекламы
платформа для покупки аккаунтов платформа для покупки аккаунтов
Guaranteed Accounts Account market
Accounts for Sale Account Acquisition
Account Selling Platform Purchase Ready-Made Accounts
Buy accounts Verified Accounts for Sale
Secure Account Purchasing Platform Account marketplace
Account Sale Account Selling Platform
Accounts marketplace Website for Selling Accounts
Guaranteed Accounts Buy accounts
Sell Account Sell accounts
Account Store Account market
Social media account marketplace Profitable Account Sales
marketplace for ready-made accounts find accounts for sale
account trading service buy account
verified accounts for sale sell accounts
account trading platform accounts marketplace
ready-made accounts for sale account trading service
accounts market account selling platform
ready-made accounts for sale buy account
account trading platform find accounts for sale
ready-made accounts for sale website for buying accounts
account selling service social media account marketplace
account selling service buy account
secure account purchasing platform account trading platform
accounts marketplace marketplace for ready-made accounts
find accounts for sale account buying platform
account buying platform purchase ready-made accounts
website for selling accounts verified accounts for sale
online account store sell account
account purchase accounts for sale
verified accounts for sale account trading platform
account trading service buy accounts
account exchange secure account purchasing platform
account buying platform account marketplace
ready-made accounts for sale secure account purchasing platform
marketplace for ready-made accounts account buying service
buy accounts secure account sales
website for buying accounts buy account
sell pre-made account database of accounts for sale
account trading platform database of accounts for sale
account trading https://accounts-offer.org
account marketplace https://accounts-marketplace.xyz
account exchange service buy accounts
accounts market https://social-accounts-marketplaces.live
accounts market https://accounts-marketplace.live
sell accounts https://social-accounts-marketplace.xyz/
account buying platform https://buy-accounts.space
social media account marketplace https://buy-accounts-shop.pro
website for buying accounts https://social-accounts-marketplace.live
account buying service https://buy-accounts.live
account trading platform account market
account buying platform accounts market
купить аккаунт https://akkaunty-na-prodazhu.pro
площадка для продажи аккаунтов https://rynok-akkauntov.top
биржа аккаунтов https://kupit-akkaunt.xyz
магазин аккаунтов маркетплейсов аккаунтов
маркетплейс аккаунтов https://akkaunty-market.live
маркетплейс аккаунтов https://kupit-akkaunty-market.xyz
биржа аккаунтов https://akkaunty-optom.live/
продать аккаунт https://online-akkaunty-magazin.xyz
продажа аккаунтов https://akkaunty-dlya-prodazhi.pro/
площадка для продажи аккаунтов https://kupit-akkaunt.online
buy aged fb account facebook ad accounts for sale
buying facebook account https://buy-ad-accounts.click
buy fb ads account buy facebook account
facebook account buy buy fb ad account
buy facebook advertising https://ad-account-buy.top
buy a facebook ad account https://buy-ads-account.work/
buy facebook old accounts fb accounts for sale
buy accounts facebook https://buy-ad-account.click
buy facebook ads account facebook ad accounts for sale
buy aged google ads accounts https://buy-ads-account.top/
buy aged google ads accounts https://buy-ads-accounts.click
buy facebook ad account https://buy-accounts.click
I absolutely admired the way this was presented.
buy google adwords account https://ads-account-for-sale.top
buy google ads accounts https://ads-account-buy.work
buy google agency account buy google agency account
buy google ads account https://buy-account-ads.work/
buy google ads threshold accounts https://buy-ads-agency-account.top
Thanks for sharing. It’s well done.
buy aged google ads accounts https://sell-ads-account.click
buy verified google ads accounts https://ads-agency-account-buy.click
buy verified facebook business manager buy-business-manager.org
buy google ad account buy aged google ads account
Thanks for putting this up. It’s top quality.
buy facebook business manager buy-bm-account.org
verified business manager for sale https://buy-business-manager-acc.org
facebook business manager for sale verified business manager for sale
unlimited bm facebook buy-verified-business-manager.org
I learned a lot from this.
buy business manager facebook business-manager-for-sale.org
facebook business account for sale buy facebook business manager account
buy facebook bm buy-bm.org
buy verified facebook business manager https://verified-business-manager-for-sale.org
pinop [url=https://pinup-azerbaycan62.com]pinop[/url] .
buy fb business manager https://buy-business-manager-accounts.org
tiktok ads account buy https://buy-tiktok-ads-account.org
buy tiktok ads account https://tiktok-ads-account-buy.org
tiktok ad accounts https://tiktok-ads-account-for-sale.org
buy tiktok business account https://tiktok-agency-account-for-sale.org
buy tiktok ads account https://buy-tiktok-ad-account.org
tiktok ads account for sale https://buy-tiktok-ads-accounts.org
tiktok ad accounts https://buy-tiktok-business-account.org
tiktok ads account buy https://buy-tiktok-ads.org
buy tiktok ad account https://tiktok-ads-agency-account.org
I took away a great deal from this.
I learned a lot from this.
Such a valuable insight.
The depth in this write-up is remarkable.
I genuinely valued the manner this was laid out.
I found new insight from this.
More posts like this would make the web better.
I gained useful knowledge from this.
I’ll surely return to read more.
You’ve clearly done your homework.
I truly enjoyed the way this was explained.
This piece is excellent.
Thanks for creating this. It’s well done.
I took away a great deal from this.
Thanks for sharing. It’s excellent.
I discovered useful points from this.
I particularly appreciated the way this was presented.
I gained useful knowledge from this.
Thanks for putting this up. It’s excellent.
More articles like this would make the blogosphere better.
I particularly admired the manner this was laid out.
More articles like this would make the internet better.
I learned a lot from this.
I found new insight from this.
I’ll surely be back for more.
More blogs like this would make the online space better.
I took away a great deal from this.
More posts like this would make the online space better.
This is the kind of post I find helpful.
Большая коллекция [url=https://kino.xxx-share.tv/]полнометражное порно на русском[/url] — только для вас!
Ищете качественные полнометражные [url=https://kino.xxx-share.tv/genre/%D0%A1%20%D0%A0%D1%83%D1%81%D1%81%D0%BA%D0%B8%D0%BC%20%D0%BF%D0%B5%D1%80%D0%B5%D0%B2%D0%BE%D0%B4%D0%BE%D0%BC]полнометражное порно с русской озвучкой[/url]? Наш сайт предлагает вам лучшую подборку в HD-формате без рекламы и ограничений.
Почему стоит выбрать нас:
Тысячи фильмов разных жанров: от классики до премьер.
[url=https://film.xxx-share.tv/genre/Private]Порнофильмы студия Private[/url]
[url=https://film.xxx-share.tv/genre/Mario-Salieri]Порнофильмы студия Марио Сальери[/url]
[url=https://film.xxx-share.tv/genre/GirlfriendsFilms]Порнофильмы студия GirlfriendsFilms[/url]
Эксклюзивные материалы, которых нет на других платформах.
Высокая скорость загрузки и стабильный стриминг без задержек.
Удобный просмотр на любом устройстве: смартфон, планшет, ПК или телевизор.
Зарегистрируйтесь сейчас и откройте доступ к огромной коллекции
[url=https://kino.xxx-share.tv/genre/%D0%A1%20%D1%81%D1%8E%D0%B6%D0%B5%D1%82%D0%BE%D0%BC]полнометражное порно с сюжетом[/url].
I’ll surely return to read more.
http://farmaciasubito.com/# quanto costa il gentalyn beta
1вин вход узбекистан http://www.1win3006.ru .
coefferalgan senza ricetta: Farmacia Subito – normix bustine
cazinouri cerință de pariere cazinouri cerință de pariere .
acheter cialis sans ordonnance pharmacie avene cleanance masque antihistaminique sans ordonnance prix
fenextra 400 prezzo: Farmacia Subito – dymavig 20 mg
Купить диплом университета!
Мы предлагаемвыгодно и быстро приобрести диплом, который выполнен на оригинальном бланке и заверен печатями, водяными знаками, подписями. Наш документ пройдет лубую проверку, даже с применением профессионального оборудования. Решите свои задачи быстро и просто с нашими дипломами- [url=http://mir.4admins.ru/posting.phpmode=post&f=10&sid=8989324f77982109ad7838cbec737419/]mir.4admins.ru/posting.phpmode=post&f=10&sid=8989324f77982109ad7838cbec737419[/url]
Мир полон тайн https://phenoma.ru читайте статьи о малоизученных феноменах, которые ставят науку в тупик. Аномальные явления, редкие болезни, загадки космоса и сознания. Доступно, интересно, с научным подходом.
resume software engineer google resume google engineer
Читайте о необычном http://phenoma.ru научно-популярные статьи о феноменах, которые до сих пор не имеют однозначных объяснений. Психология, физика, биология, космос — самые интересные загадки в одном разделе.
mostbet ilova http://www.mostbet3036.ru .
как играть на бонусный счет 1win https://1win22088.ru .
cystite ordonnance en ligne: infection urinaire femme medicament sans ordonnance – malarone gГ©nГ©rique
mostbet blackjack http://www.mostbet3048.ru .
12 профилей в Дизайне человека. Исследователь. Отшельник. Мученик. Оппортунист. Еретик. Ролевая модель. 4 6 оппортунист ролевая модель
Дизайн Человека позволяет учитывать индивидуальные особенность каждого человека и учит познавать свою истинную природу.
Профили в Дизайне человека · 1 линия — Исследователь · 2 линия — Отшельник · 3 линия — Мученик · 4 линия — Опортунист · 5 линия — Еретик · 6 линия — Ролевая модель.
12 профилей в Дизайне человека. Исследователь. Отшельник. Мученик. Оппортунист. Еретик. Ролевая модель.
Тип – это основа, но ваша уникальность проявляется через Профиль, Центры, Каналы и Ворота.
Анализ своего Дизайна Человека может помочь в понимании причин, по которым вы испытываете определенные трудности, разочарования, и как можно их преодолеть. Human design расчет
Дизайн человека – это система, которая предлагает анализ личности на основе информации о дате, времени и месте рождения.
Дизайн человека делит людей на четыре категории, помогает узнать себя и показывает путь к счастливой жизни.
Профиль в Дизайне Человека — это уникальная комбинация двух линий, которые формируют ваш характер и способы взаимодействия с миром.
vertiserc prezzo: symbicort prezzo – id 730 online scontrino farmacia
mascarillas coronavirus farmacia online se puede comprar antibiotico sin receta en usa xanax se puede comprar sin receta medica
I’ll gladly recommend this.
мостбет узбекистан http://mostbet3038.ru/ .
el misoprostol se puede comprar sin receta en espaГ±a: farmacia y parafarmacia fp online – farmacia ahumada online
viagra 100mg: prix du cialis en pharmacie – somnifere pharmacie sans ordonnance
quel est le prix d’une boГ®te de sildГ©nafil ? viagra sans ordonnance en pharmacie france ordonnance pillule en ligne
http://farmaciasubito.com/# zodon gatto
стоматологический сайт стоматологический сайт .
Понимание своего Дизайна Человека может помочь в выборе жизненного пути, который лучше соответствует вашему характеру и предназначению. Дизайн человека центры
Профили в Дизайне человека · 1 линия — Исследователь · 2 линия — Отшельник · 3 линия — Мученик · 4 линия — Опортунист · 5 линия — Еретик · 6 линия — Ролевая модель.
Дизайн человека делит людей на четыре категории, помогает узнать себя и показывает путь к счастливой жизни.
Дизайн человека помогает понять, какой тип энергии вы излучаете, как вы принимаете решения, и как лучше использовать свою энергию, чтобы не выгорать, а чувствовать себя более удовлетворённым
Тип – это основа, но ваша уникальность проявляется через Профиль, Центры, Каналы и Ворота.
сантехника донецк днр купить [url=https://santehnika-doneck-1.ru/]https://santehnika-doneck-1.ru/[/url] .
1win ilova orqali tikish 1win ilova orqali tikish .
mostbet login https://mostbet3037.ru/ .
cutacnyl sans ordonnance pharmacie: Pharmacie Express – mГ©dicament pour maigrir sans ordonnance en pharmacie
farmacia online ventolin farmacia online crema anestesica cupon farmacia online barata
deursil 150: Farmacia Subito – colpotrophine crema prezzo
lorans principio attivo: cardura 2 mg prezzo – cefodox 200 mg prezzo
1win karta orqali depozit 1win14012.ru .
1win ro‘yxatdan o‘tish orqali bonus 1win ro‘yxatdan o‘tish orqali bonus .
заезд на дачу под ключ [url=https://www.vezd-na-dachnyj-uchastok-1122.ru]https://www.vezd-na-dachnyj-uchastok-1122.ru[/url] .
free steam accounts free steam accounts
resume civil engineer fresh graduate https://resumes-engineers.com
бесплатные аккаунты в стиме пустые аккаунты стим
https://pharmacieexpress.shop/# lexomil ordonnance sГ©curisГ©e
abiostil unguento nasale ovixan crema punture insetti zimox prezzo
gastrotuss bustine bugiardino: niferex prezzo – saturimetro farmacia online
creme depilatoire pharmacie: bronchite médicament sans ordonnance – crème dépilatoire hypoallergénique pharmacie
купить программу 1с для ип купить программу 1с для ип .
peux t-on acheter du viagra en pharmacie sans ordonnance pharmacie peut elle donner pilule sans ordonnance valeriane pharmacie sans ordonnance
zitromax bambini sciroppo: zibenak 50.000 prezzo – shop farmacia online italia
1win o‘zbek tilida sayt https://www.1win14012.ru .
resume automation engineer chief engineer cv example
mi farma farmacia online: comprar online farmacia andorra – dapoxetina se puede comprar sin receta
купить 1с управление небольшой фирмой купить 1с управление небольшой фирмой .
Научно-популярный сайт https://phenoma.ru — малоизвестные факты, редкие феномены, тайны природы и сознания. Гипотезы, наблюдения и исследования — всё, что будоражит воображение и вдохновляет на поиски ответов.
muscoril pastiglie: slowmet 750 prezzo – cialis 20 mg prezzo
mostbet pul çıxarma mostbet pul çıxarma .
1вин промокод на бонус https://1win14013.ru/ .
epithelial ah duo: Pharmacie Express – acheter amoxicilline sans ordonnance en pharmacie
https://confiapharma.shop/# se puede comprar antabus sin receta
Анализ своего Дизайна Человека может помочь в понимании причин, по которым вы испытываете определенные трудности, разочарования, и как можно их преодолеть. Инкарнационный крест
Дизайн человека помогает понять, какой тип энергии вы излучаете, как вы принимаете решения, и как лучше использовать свою энергию, чтобы не выгорать, а чувствовать себя более удовлетворённым
Дизайн человека – это система, которая предлагает анализ личности на основе информации о дате, времени и месте рождения.
Дизайн Человека (human design) – это система знаний об энергетической механике людей и космологическом устройстве мира.
Для каждого человека естественного искать выгоду для себя. Так происходит и с Дизайном Человека.
Дизайн человека делит людей на четыре категории, помогает узнать себя и показывает путь к счастливой жизни.
Дизайн человека может помочь вам лучше понимать людей вокруг вас, их энергетический тип, и как лучше взаимодействовать с ними.
traitement acnГ© sans ordonnance en pharmacie: Pharmacie Express – tadalafil 20 mg boГ®te de 24 prix
bГ©tamГ©thasone sans ordonnance prix ordonnance francaise en belgique que prendre en cas d’infection urinaire sans ordonnance
dibase vitamina d prezzo: Farmacia Subito – epiduo gel 0 3 prezzo
скачать приложение 1win на айфон https://www.1win101.md .
Научно-популярный сайт https://phenoma.ru — малоизвестные факты, редкие феномены, тайны природы и сознания. Гипотезы, наблюдения и исследования — всё, что будоражит воображение и вдохновляет на поиски ответов.
Понимание своего Дизайна Человека может помочь в выборе жизненного пути, который лучше соответствует вашему характеру и предназначению. Женщина проектор
Дизайн человека – это система, которая предлагает анализ личности на основе информации о дате, времени и месте рождения.
Понимание своего Дизайна Человека может помочь в выборе жизненного пути, который лучше соответствует вашему характеру и предназначению.
Дизайн человека помогает понять, какой тип энергии вы излучаете, как вы принимаете решения, и как лучше использовать свою энергию, чтобы не выгорать, а чувствовать себя более удовлетворённым
mostbet qeydiyyat https://mostbet3049.ru .
resume electronics engineer resume software engineer google
comprar viagra en estados unidos sin receta: motilium se puede comprar sin receta – comprar viagra sin receta lleida
donde comprar valium sin receta farmacia online viagra se puede comprar aciclovir sin receta?
comprar test antigenos farmacia sin receta: como comprar medicamentos sin receta – comprar venlafaxina sin receta
http://pharmacieexpress.com/# fluocaril bi-fluorГ© 2500 ppm
mycostatin jarabe se puede comprar sin receta: farmacia urgente online – farmacia castro urdiales online
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Such a useful read.
I’ll definitely return to read more.
Thanks for publishing. It’s brilliant work.
I gained useful knowledge from this.
Приобрести диплом об образовании. Покупка документа о высшем образовании через проверенную и надежную компанию дарит ряд преимуществ для покупателя. Данное решение дает возможность сэкономить время и значительные финансовые средства. [url=http://orikdok-2v-gorode-khabarovsk-27.ru/]orikdok-2v-gorode-khabarovsk-27.ru[/url]
sitio web tavoq.es es tu aliado en el crecimiento profesional. Ofrecemos CVs personalizados, optimizacion ATS, cartas de presentacion, perfiles de LinkedIn, fotos profesionales con IA, preparacion para entrevistas y mas. Impulsa tu carrera con soluciones adaptadas a ti.
Модульный дом https://kubrdom.ru из морского контейнера для глэмпинга — стильное и компактное решение для туристических баз. Полностью готов к проживанию: утепление, отделка, коммуникации.
This is the kind of information I look for.
Professional concrete driveway installers seattle — high-quality installation, durable materials and strict adherence to deadlines. We work under a contract, provide a guarantee, and visit the site. Your reliable choice in Seattle.
Professional power washing in Seattle — effective cleaning of facades, sidewalks, driveways and other surfaces. Modern equipment, affordable prices, travel throughout Seattle. Cleanliness that is visible at first glance.
Professional seattle swimming pool installation — reliable service, quality materials and adherence to deadlines. Individual approach, experienced team, free estimate. Your project — turnkey with a guarantee.
Need transportation? auto transport carriers car transportation company services — from one car to large lots. Delivery to new owners, between cities. Safety, accuracy, licenses and experience over 10 years.
Нужна камера? установка камеры видеонаблюдения цена для дома, офиса и улицы. Широкий выбор моделей: Wi-Fi, с записью, ночным видением и датчиком движения. Гарантия, быстрая доставка, помощь в подборе и установке.
This article is incredible.
car shipping quotes auto transportation
металлический шкаф для хранения колес в паркинге [url=www.shkaf-parking-3.ru]металлический шкаф для хранения колес в паркинге[/url] .
?????? 888starz [url=https://888starz-official.com]https://888starz-official.com[/url] .
Быстро купить диплом любого института. Приобретение диплома ВУЗа через надежную компанию дарит немало достоинств. Такое решение помогает сберечь как длительное время, так и существенные средства. [url=http://orikdok-3v-gorode-nizhniy-novgorod-52.online/]orikdok-3v-gorode-nizhniy-novgorod-52.online[/url]
купить плитку 1200х600 плитка на пол керамогранит
Если вы любите динамичные сражения и философию боя, стоит [url=https://z1.anime-hd.online/detektiv/]смотреть аниме детектив[/url] – такие как «Наруто», «Блич» или «Кимоно в клетку». Эти сериалы сочетают крутые схватки с глубокими сюжетами.
Поклонникам мистики и темных историй понравится [url=https://z1.anime-hd.online/games/]смотреть аниме игра[/url]. Популярные тайтлы: «Хеллсинг», «Вампирский рыцарь» и «Серьёзный удар по судьбе».
Для тех, кто предпочитает романтику и юмор, идеально [url=https://z1.anime-hd.online/istoricheskij/]смотреть аниме историческое[/url] – например, «Любовь и обман», «Невеста чародея» или «Школьный романс».
Любителям глубоких эмоций стоит [url=https://z1.anime-hd.online/space/]смотреть аниме космос[/url]. Шедевры жанра: «Твоё имя», «Клинок, рассекающий демонов» и «Волчий дети Амэ и Юки».
А если ищете добрые и светлые истории, можно [url=https://z1.anime-hd.online/komedija/]смотреть аниме комедии[/url] – «Покемон», «Её зовём Хикару» или «Мой сосед Тоторо».
Выбирайте жанр и погружайтесь в увлекательный мир аниме!
Профессиональное косметологическое оборудование аппараты для салонов красоты, клиник и частных мастеров. Аппараты для чистки, омоложения, лазерной эпиляции, лифтинга и ухода за кожей.
защита консультация юрист юрист бесплатная консультация по телефону москва
Semaglu Pharm: п»їBuy Rybelsus online USA – SemagluPharm
Crestor Pharm [url=https://crestorpharm.com/#]Buy statins online discreet shipping[/url] Generic Crestor for high cholesterol
Crestor Pharm: atorvastatin to rosuvastatin conversion – CrestorPharm
http://semaglupharm.com/# SemagluPharm
https://semaglupharm.shop/# FDA-approved Rybelsus alternative
КредитоФФ http://creditoroff.ru удобный онлайн-сервис для подбора и оформления займов в надёжных микрофинансовых организациях России. Здесь вы найдёте лучшие предложения от МФО
https://semaglupharm.com/# SemagluPharm
Купить диплом университета по невысокой стоимости возможно, обращаясь к проверенной специализированной фирме. Заказать документ ВУЗа можно в нашем сервисе. [url=http://orikdok-4v-gorode-vladikavkaz-15.online/]orikdok-4v-gorode-vladikavkaz-15.online[/url]
Predni Pharm: Predni Pharm – can i purchase prednisone without a prescription
https://prednipharm.com/# prednisone in canada
https://semaglupharm.shop/# best online semaglutide
prednisone for sale without a prescription [url=https://prednipharm.shop/#]PredniPharm[/url] prednisone where can i buy
Купить диплом о высшем образовании!
Наша компания предлагаетвыгодно и быстро заказать диплом, который выполняется на бланке ГОЗНАКа и заверен мокрыми печатями, штампами, подписями. Данный диплом пройдет любые проверки, даже с использованием профессиональных приборов. Достигайте своих целей быстро и просто с нашей компанией- [url=http://radiocoop.co/2025/04/13/10758/]radiocoop.co/2025/04/13/10758[/url]
https://semaglupharm.com/# FDA-approved Rybelsus alternative
септик на 2 человека для постоянного проживания септик на 2 человека для постоянного проживания .
Predni Pharm: prednisone 20 mg tablet – Predni Pharm
https://semaglupharm.com/# Semaglu Pharm
http://lipipharm.com/# Lipi Pharm
?? У кого в дипломе хоть раз была правда?
Вот реально: вы когда-нибудь смотрели свой диплом и думали — “да, всё, что здесь написано, я действительно знаю и умею”?
У многих — только бумажка. Корочка, глянцевая, с гербом и подписями, которую HR пролистывает за 1,5 секунды. А потом начинается: опыт, кейсы, компетенции, “что умеешь по факту?”. Диплом где-то там, в глубокой папке.
Но парадокс в том, что без диплома тебе даже не дадут шанс показать, что ты умеешь.
Ты можешь быть крутым специалистом, уметь в IT, дизайн, управление, логистику — но без документа с золотым тиснением не попадешь на собеседование.
?? Нормально ли это? Нет. Реальность ли это? Да.
Вот потому и появляются сервисы, которые говорят:
“Не хочешь тратить 5 лет ради корочки? Мы поможем. Тебе нужен не вуз — тебе нужен диплом.”
Ты его получаешь, кладёшь в резюме, и дальше всё зависит от твоих мозгов, а не от шрифта на бумаге.
Кто-то скажет: “Это обман!”
А кто-то — “Это адаптация к системе, которая обманывает тебя с детства”.
?? И что в итоге?
Диплом становится не подтверждением знаний, а входным билетом. Как QR-код в метро — проверили, что есть, и пропустили.
Поэтому люди и принимают такие решения.
Не потому что глупые. А потому что взрослые, занятые, уставшие от лишнего.
Потому что хотят не учиться “ради процесса”, а работать по делу.
?? Ирония в том, что большинство таких дипломов — работают.
Даже если ты их не учил — ты знаешь, как применить. А вот “настоящие выпускники” потом всё равно идут на курсы и стажировки, потому что ничего не помнят.
И что важнее: корочка или то, как ты справляешься с задачей?
?? У кого были такие мысли — пишите. У кого был опыт — делитесь.
Сколько стоит диплом колледжа СЃ архивной справкой? [url=https://spbrcom.ru/]Рсточник[/url]
prednisone 50mg cost: PredniPharm – PredniPharm
Crestor Pharm [url=https://crestorpharm.com/#]what are the downsides of crestor?[/url] plavix and crestor at the same time
Мобильный шиномонтаж телефон https://shinomontazh-vyezdnoj.ru/
Юрист по недвижимости https://yuristy-ekaterinburga.ru
atorvastatin how does it work: Order cholesterol medication online – Lipi Pharm
https://semaglupharm.com/# Semaglu Pharm
crestor and diabetes [url=https://crestorpharm.com/#]Crestor mail order USA[/url] Crestor Pharm
https://lipipharm.shop/# LipiPharm
https://semaglupharm.shop/# SemagluPharm
how much weight can you lose on rybelsus 3 mg: Semaglu Pharm – Semaglu Pharm
SemagluPharm [url=https://semaglupharm.com/#]is rybelsus for diabetes[/url] SemagluPharm
Защитные кейсы https://plastcase.ru/ в Санкт-Петербурге — надежная защита оборудования от влаги, пыли и ударов. Большой выбор размеров и форматов, ударопрочные материалы, индивидуальный подбор.
http://semaglupharm.com/# rybelsus weight loss dosage
LipiPharm: Lipi Pharm – atorvastatin with or without food
how many units is .25 mg of semaglutide [url=https://semaglupharm.shop/#]п»їBuy Rybelsus online USA[/url] semaglutide diet plan
https://semaglupharm.com/# can i take rybelsus at night
Lipi Pharm: FDA-approved generic statins online – what is the lowest dose of lipitor
http://crestorpharm.com/# Crestor Pharm
мгновенно онлайн займ срочно займ онлайн на 30 дней
Buy statins online discreet shipping [url=https://crestorpharm.shop/#]CrestorPharm[/url] does rosuvastatin increase calcium levels
http://semaglupharm.com/# Semaglu Pharm
PredniPharm [url=http://prednipharm.com/#]15 mg prednisone daily[/url] PredniPharm
http://indiapharmglobal.com/# indian pharmacy
Женский блог https://zhinka.in.ua Жінка это самое интересное о красоте, здоровье, отношениях. Много полезной информации для женщин.
pharmacy canadian superstore: recommended canadian pharmacies – maple leaf pharmacy in canada
canadian pharmacy india: best canadian pharmacy – best canadian online pharmacy reviews
https://indiapharmglobal.com/# best india pharmacy
Строительный портал https://proektsam.kyiv.ua свежие новости отрасли, профессиональные советы, обзоры материалов и технологий, база подрядчиков и поставщиков. Всё о ремонте, строительстве и дизайне в одном месте.
Городской портал Черкассы https://u-misti.cherkasy.ua новости, обзоры, события Черкасс и области
mexico drug stores pharmacies [url=http://medsfrommexico.com/#]buying from online mexican pharmacy[/url] Meds From Mexico
Портал о строительстве https://buildportal.kyiv.ua и ремонте: лучшие решения для дома, дачи и бизнеса. Инструменты, сметы, калькуляторы, обучающие статьи и база подрядчиков.
Портал о строительстве https://buildportal.kyiv.ua и ремонте: лучшие решения для дома, дачи и бизнеса. Инструменты, сметы, калькуляторы, обучающие статьи и база подрядчиков.
https://indiapharmglobal.com/# India Pharm Global
Портал города Черновцы https://u-misti.chernivtsi.ua последние новости, события, обзоры
pharmacies in mexico that ship to usa: Meds From Mexico – Meds From Mexico
http://indiapharmglobal.com/# best india pharmacy
новгород нарколог на дом нарколог на дом срочно
цена кодировки от алкоголизма https://kodirovanie-info.ru
лечение алкогольной зависимости НН https://alko-info.ru
Meds From Mexico: mexican rx online – Meds From Mexico
вывод из запоя на дом цена выведение из запоя в стационаре
Новинний сайт Житомира https://faine-misto.zt.ua новости Житомира сегодня
Праздничная продукция https://prazdnik-x.ru для любого повода: шары, гирлянды, декор, упаковка, сувениры. Всё для дня рождения, свадьбы, выпускного и корпоративов.
оценка ООО для нотариуса оценка склада
лечение наркомании цена лечение наркомании нижний новгород
top online pharmacy india: world pharmacy india – India Pharm Global
Всё для строительства https://d20.com.ua и ремонта: инструкции, обзоры, экспертизы, калькуляторы. Профессиональные советы, новинки рынка, база строительных компаний.
¡Saludos, aventureros del azar !
Casinos online extranjeros verificados por expertos – https://www.casinosextranjerosenespana.es/ mejores casinos online extranjeros
¡Que vivas increíbles jugadas excepcionales !
http://canadapharmglobal.com/# canadianpharmacyworld com
Строительный журнал https://garant-jitlo.com.ua всё о технологиях, материалах, архитектуре, ремонте и дизайне. Интервью с экспертами, кейсы, тренды рынка.
Онлайн-журнал https://inox.com.ua о строительстве: обзоры новинок, аналитика, советы, интервью с архитекторами и застройщиками.
Современный строительный https://interiordesign.kyiv.ua журнал: идеи, решения, технологии, тенденции. Всё о ремонте, стройке, дизайне и инженерных системах.
Информационный журнал https://newhouse.kyiv.ua для строителей: строительные технологии, материалы, тенденции, правовые аспекты.
world pharmacy india [url=http://indiapharmglobal.com/#]top online pharmacy india[/url] best online pharmacy india
real canadian pharmacy: best canadian online pharmacy reviews – canadian pharmacy no scripts
pharmacy website india: indianpharmacy com – best india pharmacy
http://indiapharmglobal.com/# india pharmacy
online pharmacy india: India Pharm Global – indian pharmacy
Строительный журнал https://poradnik.com.ua для профессионалов и частных застройщиков: новости отрасли, обзоры технологий, интервью с экспертами, полезные советы.
Всё о строительстве https://stroyportal.kyiv.ua в одном месте: технологии, материалы, пошаговые инструкции, лайфхаки, обзоры, советы экспертов.
Журнал о строительстве https://sovetik.in.ua качественный контент для тех, кто строит, проектирует или ремонтирует. Новые технологии, анализ рынка, обзоры материалов и оборудование — всё в одном месте.
Полезный сайт https://vasha-opora.com.ua для тех, кто строит: от фундамента до крыши. Советы, инструкции, сравнение материалов, идеи для ремонта и дизайна.
¡Hola, entusiastas del entretenimiento !
Casinossinlicenciaespana.es: reseГ±as honestas – https://casinossinlicenciaespana.es/# casino sin licencia espaГ±ola
¡Que experimentes premios asombrosos !
Новости Полтава https://u-misti.poltava.ua городской портал, последние события Полтавы и области
online canadian pharmacy: Canada Pharm Global – canadian pharmacy cheap
https://canadapharmglobal.shop/# rate canadian pharmacies
http://canadapharmglobal.com/# canada cloud pharmacy
Кулинарный портал https://vagon-restoran.kiev.ua с тысячами проверенных рецептов на каждый день и для особых случаев. Пошаговые инструкции, фото, видео, советы шефов.
Мужской журнал https://hand-spin.com.ua о стиле, спорте, отношениях, здоровье, технике и бизнесе. Актуальные статьи, советы экспертов, обзоры и мужской взгляд на важные темы.
Журнал для мужчин https://swiss-watches.com.ua которые ценят успех, свободу и стиль. Практичные советы, мотивация, интервью, спорт, отношения, технологии.
Читайте мужской https://zlochinec.kyiv.ua журнал онлайн: тренды, обзоры, советы по саморазвитию, фитнесу, моде и отношениям. Всё о том, как быть уверенным, успешным и сильным — каждый день.
кредит без документов онлайн бишкек [url=https://www.kredity-plohoj-ki-1.ru]кредит без документов онлайн бишкек[/url] .
onlinepharmaciescanada com: canadian pharmacy com – best canadian online pharmacy reviews
ИнфоКиев https://infosite.kyiv.ua события, новости обзоры в Киеве и области.
indian pharmacy paypal [url=http://indiapharmglobal.com/#]top 10 pharmacies in india[/url] mail order pharmacy india
https://indiapharmglobal.com/# India Pharm Global
Все новинки https://helikon.com.ua технологий в одном месте: гаджеты, AI, робототехника, электромобили, мобильные устройства, инновации в науке и IT.
Портал о ремонте https://as-el.com.ua и строительстве: от черновых работ до отделки. Статьи, обзоры, идеи, лайфхаки.
Ремонт без стресса https://odessajs.org.ua вместе с нами! Полезные статьи, лайфхаки, дизайн-проекты, калькуляторы и обзоры.
Мы готовы предложить дипломы психологов, юристов, экономистов и любых других профессий по приятным ценам. Мы готовы предложить документы техникумов, которые расположены в любом регионе РФ. Документы печатаются на “правильной” бумаге высшего качества. Это позволяет делать настоящие дипломы, которые не отличить от оригиналов. [url=http://orikdok-5v-gorode-samara-63.online/]orikdok-5v-gorode-samara-63.online[/url]
Сайт о строительстве https://selma.com.ua практические советы, современные технологии, пошаговые инструкции, выбор материалов и обзоры техники.
¡Hola, jugadores apasionados !
casinoonlinefueradeespanol con pagos garantizados – https://www.casinoonlinefueradeespanol.xyz/# casino por fuera
¡Que disfrutes de asombrosas tiradas afortunadas !
https://indiapharmglobal.shop/# India Pharm Global
canadianpharmacyworld: Canada Pharm Global – canadian pharmacy scam
Городской портал Винницы https://u-misti.vinnica.ua новости, события и обзоры Винницы и области
https://papafarma.shop/# productos farmaci
Rask Apotek [url=https://raskapotek.shop/#]Rask Apotek[/url] elektrolytter tabletter apotek
Papa Farma: Papa Farma – 10 productos de parafarmacia
¡Saludos, exploradores de tesoros !
Ventajas de casinoextranjerosenespana.es para jugadores – п»їhttps://casinoextranjerosenespana.es/ casinoextranjerosenespana.es
¡Que disfrutes de tiradas afortunadas !
arcoxia 90 mg per quanti giorni: EFarmaciaIt – EFarmaciaIt
https://efarmaciait.com/# vitamina dibase 25000 prezzo
Портал Львів https://u-misti.lviv.ua останні новини Львова и области.
farmГ cia barcelona: Papa Farma – Papa Farma
https://raskapotek.com/# Rask Apotek
mebendazol spanje [url=https://papafarma.com/#]cepillo oral b io 7[/url] Papa Farma
Свежие новости https://ktm.org.ua Украины и мира: политика, экономика, происшествия, культура, спорт. Оперативно, объективно, без фейков.
Сайт о строительстве https://solution-ltd.com.ua и дизайне: как построить, отремонтировать и оформить дом со вкусом.
Читайте авто блог https://autoblog.kyiv.ua обзоры автомобилей, сравнения моделей, советы по выбору и эксплуатации, новости автопрома.
Авто портал https://real-voice.info для всех, кто за рулём: свежие автоновости, обзоры моделей, тест-драйвы, советы по выбору, страхованию и ремонту.
возможно ли получить кредит с плохой кредитной историей возможно ли получить кредит с плохой кредитной историей .
https://efarmaciait.shop/# farmacia re
voltaren fiale serve ricetta: EFarmaciaIt – en 0 5 mg compresse a cosa serve
Новини Львів https://faine-misto.lviv.ua последние новости и события – Файне Львов
Портал о строительстве https://start.net.ua и ремонте: готовые проекты, интерьерные решения, сравнение материалов, опыт мастеров.
Строительный портал https://apis-togo.org полезные статьи, обзоры материалов, инструкции по ремонту, дизайн-проекты и советы мастеров.
Комплексный строительный https://ko-online.com.ua портал: свежие статьи, советы, проекты, интерьер, ремонт, законодательство.
Всё о строительстве https://furbero.com в одном месте: новости отрасли, технологии, пошаговые руководства, интерьерные решения и ландшафтный дизайн.
navizan 2 mg mutuabile [url=http://efarmaciait.com/#]robilas prezzo con ricetta[/url] etoricoxib farmaco originale
Портал для женщин https://olive.kiev.ua любого возраста: от секретов молодости и красоты до личностного роста и материнства.
Онлайн-портал https://leif.com.ua для женщин: мода, психология, рецепты, карьера, дети и любовь. Читай, вдохновляйся, общайся, развивайся!
Современный женский https://prowoman.kyiv.ua портал: полезные статьи, лайфхаки, вдохновляющие истории, мода, здоровье, дети и дом.
Портал о маркетинге https://reklamspilka.org.ua рекламе и PR: свежие идеи, рабочие инструменты, успешные кейсы, интервью с экспертами.
https://papafarma.shop/# ozempic prospecto
https://papafarma.shop/# Papa Farma
influensavaksine 2023 apotek: mygg klistremerker apotek – legevakten apotek
¡Saludos, buscadores de éxitos!
Tragamonedas clГЎsicas en casinos online extranjeros – https://www.casinosextranjero.es/# п»їcasinos online extranjeros
¡Que vivas increíbles victorias épicas !
События Днепр https://u-misti.dp.ua последние новости Днепра и области, обзоры и самое интересное
EFarmaciaIt [url=https://efarmaciait.com/#]EFarmaciaIt[/url] EFarmaciaIt
Семейный портал https://stepandstep.com.ua статьи для родителей, игры и развивающие материалы для детей, советы психологов, лайфхаки.
Клуб родителей https://entertainment.com.ua пространство поддержки, общения и обмена опытом.
Туристический портал https://aliana.com.ua с лучшими маршрутами, подборками стран, бюджетными решениями, гидами и советами.
Всё о спорте https://beachsoccer.com.ua в одном месте: профессиональный и любительский спорт, фитнес, здоровье, техника упражнений и спортивное питание.
http://papafarma.com/# Papa Farma
a cosa serve il lasix: tadalafil (20 mg 8 compresse prezzo) – augmentin casa farmaceutica
pro direct opiniones [url=http://papafarma.com/#]Papa Farma[/url] Papa Farma
Купить диплом об образовании. Заказ подходящего диплома через проверенную и надежную компанию дарит множество преимуществ. Данное решение дает возможность сберечь как дорогое время, так и серьезные финансовые средства. [url=http://orikdok-v-gorode-krasnodar-23.ru/]orikdok-v-gorode-krasnodar-23.ru[/url]
Покупка официального диплома через надежную компанию дарит ряд достоинств. Заказать диплом о высшем образовании: [url=http://icqpro.com.br/employer/diplomirovans]icqpro.com.br/employer/diplomirovans[/url]
https://efarmaciait.com/# EFarmaciaIt
http://svenskapharma.com/# tabletten
styrkedrikk apotek: Rask Apotek – Гёrevoks fjerning apotek
Новости Украины https://useti.org.ua в реальном времени. Всё важное — от официальных заявлений до мнений экспертов.
Информационный портал https://comart.com.ua о строительстве и ремонте: полезные советы, технологии, идеи, лайфхаки, расчёты и выбор материалов.
Архитектурный портал https://skol.if.ua современные проекты, урбанистика, дизайн, планировка, интервью с архитекторами и тренды отрасли.
Всё о строительстве https://ukrainianpages.com.ua просто и по делу. Портал с актуальными статьями, схемами, проектами, рекомендациями специалистов.
apotek online [url=https://raskapotek.shop/#]akupunkturnГҐler apotek[/url] Rask Apotek
Новостной портал Одесса https://u-misti.odesa.ua последние события города и области. Обзоры и много интресного о жизни в Одессе.
Новости Украины https://hansaray.org.ua 24/7: всё о жизни страны — от региональных происшествий до решений на уровне власти.
Всё об автомобилях https://autoclub.kyiv.ua в одном месте. Обзоры, новости, инструкции по уходу, автоистории и реальные тесты.
Строительный журнал https://dsmu.com.ua идеи, технологии, материалы, дизайн, проекты, советы и обзоры. Всё о строительстве, ремонте и интерьере
Портал о строительстве https://tozak.org.ua от идеи до готового дома. Проекты, сметы, выбор материалов, ошибки и их решения.
Svenska Pharma: apotek bestГ¤lla hem – Svenska Pharma
codeine apotek: recept hemleverans – Г¶ppna apotek idag
en 0 5 mg compresse a cosa serve [url=http://efarmaciait.com/#]EFarmaciaIt[/url] farmacia dottor max
Городской портал Одессы https://faine-misto.od.ua последние новости и происшествия в городе и области
http://raskapotek.com/# Rask Apotek
Информационный портал https://dailynews.kyiv.ua актуальные новости, аналитика, интервью и спецтемы.
Новостной портал https://news24.in.ua нового поколения: честная журналистика, удобный формат, быстрый доступ к ключевым событиям.
Портал для женщин https://a-k-b.com.ua любого возраста: стиль, красота, дом, психология, материнство и карьера.
Онлайн-новости https://arguments.kyiv.ua без лишнего: коротко, по делу, достоверно. Политика, бизнес, происшествия, спорт, лайфстайл.
mifepristona comprar espaГ±a: Papa Farma – farmacia alba
onaka flaconi: gentalyn beta prezzo amazon – EFarmaciaIt
Мировые новости https://ua-novosti.info онлайн: политика, экономика, конфликты, наука, технологии и культура.
Только главное https://ua-vestnik.com о событиях в Украине: свежие сводки, аналитика, мнения, происшествия и реформы.
Женский портал https://woman24.kyiv.ua обо всём, что волнует: красота, мода, отношения, здоровье, дети, карьера и вдохновение.
защитный герметичный кейс plastcase.ru/
ecn foglietto illustrativo [url=http://efarmaciait.com/#]EFarmaciaIt[/url] EFarmaciaIt
¡Hola, seguidores de victorias !
casinoextranjero.es – conecta con casinos sin restricciones – п»їhttps://casinoextranjero.es/ casinos extranjeros
¡Que vivas recompensas fascinantes !
http://svenskapharma.com/# billiga kök komplett
The best GDPR server (best European data centers) managed and unmanaged, GDPR compliant, super fast NVMe SSDs, reliable 1Gbps network, choice of OS and finally affordable prices. Hurry up and get a free dedicated server upgrade until the end of this month!
выберите топ займов с быстрым оформлением, прозрачными условиями и моментальным переводом на карту. Только проверенные МФО — без скрытых комиссий.
Центр ИСО сертификации https://iso-prof.ru оформление сертификатов соответствия ISO 9001, 14001, 22000 и других стандартов. Консультации, сопровождение, помощь в прохождении аудита.
Офисная мебель https://officepro54.ru в Новосибирске купить недорого от производителя
Онлайн микрозаймы займ онлайн
elocom crema para que se utiliza: Papa Farma – Papa Farma
Noten fur klavier musik noten klavier
Svenska Pharma: Svenska Pharma – Svenska Pharma
https://svenskapharma.shop/# Svenska Pharma
Svenska Pharma [url=https://svenskapharma.shop/#]Svenska Pharma[/url] läkemedels
Хмельницький новини https://u-misti.khmelnytskyi.ua огляди, новини, сайт Хмельницького
¡Bienvenidos, fanáticos del azar !
Casino fuera de EspaГ±a con juego justo garantizado – https://www.casinoporfuera.guru/# п»їcasino fuera de espaГ±a
¡Que disfrutes de maravillosas movidas brillantes !
http://efarmaciait.com/# artrosilene gel prezzo
urinvГ¤gsinfektion citron: naproxen receptfritt 500 mg – scandinavia apotek
https://efarmaciait.com/# EFarmaciaIt
купить отчет по учебной практике отчет по практике цена выполнения
заказать реферат москва сколько стоит реферат
дипломная работа срочно на заказ дипломная работа на заказ
Медпортал https://medportal.co.ua украинский блог о медициние и здоровье. Новости, статьи, медицинские учреждения
Rask Apotek: bestille reseptvarer pГҐ nett – ansiktsmaske apotek
https://svenskapharma.com/# Svenska Pharma
Rask Apotek: kjГёpe medisin pГҐ nett – apotek 24 timer
sista schampo [url=http://svenskapharma.com/#]Svenska Pharma[/url] snabb håruppsättning
Файне Винница https://faine-misto.vinnica.ua новости и события Винницы сегодня. Городской портал, обзоры.
http://raskapotek.com/# apotek ГҐpent kristi himmelfartsdag
быстрый кредит онлайн без отказа быстрый кредит онлайн без отказа .
Покупка дипломов ВУЗов в Москве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4498 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor3.ru/]Купить диплом недорого[/url] — ответим быстро, без лишних формальностей.
pink eye svenska: Svenska Pharma – Svenska Pharma
Мы предлагаем оформление дипломов ВУЗов по всей России и СНГ — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4989 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor4.ru/]Смотреть тут[/url] — ответим быстро, без лишних формальностей.
https://svenskapharma.com/# köp frakt online
займы онлайн без процентов zajmy-onlajn
Мы предлагаем оформление дипломов ВУЗов в Москве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4475 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://spbrcom5.ru/]Узнать условия[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов по всей России и СНГ — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2639 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://spbrcom6.ru/]Купить диплом недорого[/url] — ответим быстро, без лишних формальностей.
EFarmaciaIt [url=https://efarmaciait.shop/#]expose recensioni[/url] gocce microser
быстрые займы на карту без отказа онлайн https://zajm-bez-otkaza-1.ru .
nattГҐpent apotek: mot hoste apotek – Rask Apotek
hirudoid gel france [url=http://pharmaconfiance.com/#]box parapharmacie[/url] univers pharmacie mon compte
Мы предлагаем оформление дипломов ВУЗов по всей России и СНГ — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2717 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor2.ru/]По ссылке[/url] — ответим быстро, без лишних формальностей.
https://pharmajetzt.shop/# luitpold apotheke bad steben
Мы предлагаем оформление дипломов ВУЗов в Москве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1208 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor5.ru/]Пишите[/url] — ответим быстро, без лишних формальностей.
Оформиление дипломов ВУЗов по всей России и СНГ — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3474 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor4.ru/]Купить государственный диплом[/url] — ответим быстро, без лишних формальностей.
http://medicijnpunt.com/# apteka eindhoven
Pharma Confiance: Pharma Confiance – anne cavailhes avis
Pharma Confiance: Pharma Confiance – pharmacie la moins chere
[url=http://www.drenazh-fundamenta-812.ru]drenazh-fundamenta-812.ru[/url] .
Автогид https://avtogid.in.ua автомобильный украинский портал с новостями, обзорами, советами для автовладельцев
Покупка дипломов ВУЗов по всей России и СНГ — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4998 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://spbrcom5.ru/]Открыть[/url] — ответим быстро, без лишних формальностей.
https://pharmaconfiance.com/# Pharma Confiance
mouse pharmacy viagra [url=http://pharmaconnectusa.com/#]PharmaConnectUSA[/url] propecia discount pharmacy
контрольные по статистике контрольная по бухучету
medi apotheke: tierapotheke online auf rechnung – internetapotheken preisvergleich
Pharma Confiance: paracetamol belgique – Pharma Confiance
https://pharmaconnectusa.shop/# online pharmacy cialis review
https://pharmaconfiance.com/# pharmacie la geode
medikamente 24 stunden lieferung [url=https://pharmajetzt.shop/#]bestellapotheken[/url] claras apotheke online
Портал Киева https://u-misti.kyiv.ua новости и события в Киеве сегодня.
Pharma Connect USA: PharmaConnectUSA – PharmaConnectUSA
¡Saludos, usuarios de plataformas de juego !
casinos fuera de EspaГ±a con tragamonedas clГЎsicas – https://www.casinosonlinefueraespanol.xyz/# п»їcasino fuera de espaГ±a
¡Que disfrutes de jackpots fascinantes!
Pharma Confiance: pommade soolantra – pharmacie de garde montpellier ouverte aujourd’hui
https://pharmaconnectusa.com/# us pharmacy no prescription
[url=https://t.me/rabotavdosuge]Работа для девушек[/url]
[url=https://t.me/iz_rabota_dlya_devushek]Работа девушкам[/url]
Medicijn Punt [url=https://medicijnpunt.com/#]MedicijnPunt[/url] Medicijn Punt
MedicijnPunt: farma – medicatie online bestellen
medco pharmacy lipitor: lansoprazole online pharmacy – Pharma Connect USA
https://pharmaconnectusa.shop/# PharmaConnectUSA
http://pharmajetzt.com/# apotheke luitpold
lavita login: Pharma Jetzt – apothek
online apotheker [url=https://medicijnpunt.com/#]Medicijn Punt[/url] medicine online
https://pharmaconfiance.shop/# pharmacie grasse
Pharma Jetzt: Pharma Jetzt – medikament online
autocad 2025 full
[url=https://autocadltmep.com/]autocad 2025 and autodesk products bundle [/url]
autocad 2025 toolset bundle
[url=https://autocadltmep.com/]autocad 2023 [/url]
autocad 2021
PharmaConnectUSA: rx plus pharmacy nyc – rx solutions pharmacy help desk
apotheek producten [url=https://medicijnpunt.com/#]med apotheek[/url] Medicijn Punt
¡Bienvenidos, fanáticos del juego !
Casino por fuera con multibilletera integrada – https://www.casinofueraespanol.xyz/# casinos online fuera de espaГ±a
¡Que vivas increíbles giros exitosos !
https://pharmajetzt.shop/# Pharma Jetzt
PharmaConnectUSA: rx express pharmacy – Pharma Connect USA
https://pharmaconfiance.shop/# pharmacie dans les alentours
Сайт Житомир https://u-misti.zhitomir.ua новости и происшествия в Житомире и области
Medicijn Punt: MedicijnPunt – MedicijnPunt
gГјnstige versandapotheke [url=http://pharmajetzt.com/#]online apothee[/url] Pharma Jetzt
¡Hola, descubridores de oportunidades !
casinosextranjerosdeespana.es – juegos actualizados – п»їhttps://casinosextranjerosdeespana.es/ casino online extranjero
¡Que vivas increíbles victorias memorables !
http://pharmajetzt.com/# shopaptheke
shop apoth: medikamente apotheke – inline apotheke
Pharma Confiance: pharmacie grand paris – Pharma Confiance
medikamente apotheke [url=http://pharmajetzt.com/#]apotheek online[/url] Pharma Jetzt
https://pharmajetzt.shop/# shop apotheke deutschland
medikamente bestellen ohne rezept: Pharma Jetzt – Pharma Jetzt
Medicijn Punt: belgische online apotheek – Medicijn Punt
Pharma Connect USA [url=https://pharmaconnectusa.com/#]provigil pharmacy online[/url] safe rx pharmacy
https://pharmajetzt.shop/# günstige apotheken
Быстрый ремонт бытовой техники на дому без очередей.
Срочно нужен мастер по технике? Звоните нам!
Rent-Auto.md offers car rental in Chisnau and other major cities of Moldova on the best terms. Whether you are planning a business trip, a family vacation or a business trip, we have the perfect solutions for your travel around the city and beyond.
Масляный обогреватель https://brand-climat.ru длительное тепло и аккумулирование для автономного обогрева. Безопасность и тихая работа, консультации по выбору, доставка и официальная гарантия. Теплый дом в холодные дни!
Medicijn Punt: medicijn bestellen – MedicijnPunt
Оформиление дипломов ВУЗов по всей России и СНГ — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3344 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor6.ru/]Купить диплом о среднем профессиональном образовании[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов по всей России и СНГ — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4635 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor9.ru/]Диплом цена[/url] — ответим быстро, без лишних формальностей.
Оформиление дипломов ВУЗов в Москве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1537 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor8.ru/]Сайт компании[/url] — ответим быстро, без лишних формальностей.
mГ©tronidazole chat: Pharma Confiance – Pharma Confiance
Оформиление дипломов ВУЗов в Москве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2794 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://spbrcom10.ru/]Смотреть тут[/url] — ответим быстро, без лишних формальностей.
https://medicijnpunt.shop/# Medicijn Punt
PharmaConnectUSA [url=https://pharmaconnectusa.com/#]Pharma Connect USA[/url] kamagra pharmacy online
Украинский бизнес https://in-ukraine.biz.ua информацинный портал о бизнесе, финансах, налогах, своем деле в Украине
Покупка дипломов ВУЗов по всей России и СНГ — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3682 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor7.ru/]Сайт компании[/url] — ответим быстро, без лишних формальностей.
https://pharmaconnectusa.com/# levitra prices pharmacy
Мы предлагаем оформление дипломов ВУЗов по всей России и СНГ — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4263 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor8.ru/]Мы поможем[/url] — ответим быстро, без лишних формальностей.
https://pharmaconfiance.shop/# Pharma Confiance
world pharmacy store discount number [url=https://pharmaconnectusa.com/#]accutane pharmacy prices[/url] pharmacy artane castle shopping centre
Pharma Jetzt: PharmaJetzt – PharmaJetzt
Medicijn Punt: MedicijnPunt – Medicijn Punt
https://pharmaconfiance.com/# viagra pommade sans ordonnance
Репетитор по физике https://repetitor-po-fizike-spb.ru СПб: школьникам и студентам, с нуля и для олимпиад. Четкие объяснения, практика, реальные результаты.
apotheke versandkostenfrei [url=http://pharmajetzt.com/#]luitpold apotheke online shop[/url] Pharma Jetzt
india pharmacy cymbalta: estradiol inhouse pharmacy – RxFree Meds
https://enclomiphenebestprice.shop/# enclomiphene for sale
can i get clomid at a pharmacy: RxFree Meds – RxFree Meds
farmacia granada: Farmacia Asequible – cepillo oral b io 9
asda pharmacy mefloquine [url=http://rxfreemeds.com/#]RxFree Meds[/url] RxFree Meds
http://rxfreemeds.com/# pharmacy warfarin counseling
http://farmaciaasequible.com/# farmacia online barcelona barata
buy enclomiphene online: enclomiphene for men – enclomiphene best price
Thank you, your article surprised me, there is such an excellent point of view. Thank you for sharing, I learned a lot.
http://farmaciaasequible.com/# farmacias 24 horas valencia
Farmacia Asequible: diprogenta crema para que sirve – precio cariban sin receta
More blogs like this would make the online space richer.
citrafleet precio: Farmacia Asequible – Farmacia Asequible
enclomiphene buy [url=https://enclomiphenebestprice.shop/#]enclomiphene testosterone[/url] enclomiphene online
https://enclomiphenebestprice.com/# enclomiphene testosterone
farmacia 24 horas tenerife: diprogenta para que es – elocom 1 mg
http://farmaciaasequible.com/# farmГ cia i parafarmГ cia
enclomiphene testosterone [url=http://enclomiphenebestprice.com/#]enclomiphene online[/url] buy enclomiphene online
https://rxfreemeds.shop/# legal online pharmacy percocet
rx pharmacy plus: pharmacy drug store near me – online medicine order discount
mejor champГє hidratante ocu: Farmacia Asequible – Farmacia Asequible
costo de los servicios del cosmet?logo costo de los servicios del cosmet?logo .
лазерная косметология цены лазерная косметология цены .
RxFree Meds [url=https://rxfreemeds.shop/#]RxFree Meds[/url] RxFree Meds
https://enclomiphenebestprice.com/# enclomiphene citrate
https://enclomiphenebestprice.shop/# enclomiphene best price
http://enclomiphenebestprice.com/# enclomiphene for men
enclomiphene citrate [url=https://enclomiphenebestprice.shop/#]enclomiphene buy[/url] enclomiphene online
farmacias valencia 24 horas: comprar viagra en badajoz – la mejor crema para la cara a partir de los 40 ocu
https://rxfreemeds.shop/# Cipro
farmacia cercana a mГ: medicamentos de venta libre en espaГ±a – Farmacia Asequible
enclomiphene testosterone [url=http://enclomiphenebestprice.com/#]enclomiphene online[/url] enclomiphene best price
http://farmaciaasequible.com/# Farmacia Asequible
Farmacia Asequible: Farmacia Asequible – fundas para el pene
https://enclomiphenebestprice.shop/# enclomiphene for men
Оформиление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3801 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom9.ru/]Купить дипломы о высшем образовании срочно[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4693 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom5.ru/]Где купить диплом[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4243 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom7.ru/]Купить диплом института образования[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4970 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom1.su/]Можно ли купить диплом о высшем образовании[/url] — ответим быстро, без лишних формальностей.
enclomiphene [url=http://enclomiphenebestprice.com/#]enclomiphene best price[/url] enclomiphene
enclomiphene price: enclomiphene for sale – enclomiphene best price
http://farmaciaasequible.com/# comparador farmacias online
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1161 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom9.ru/]Открыть[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2100 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom5.ru/]Мы поможем[/url] — ответим быстро, без лишних формальностей.
enclomiphene buy [url=https://enclomiphenebestprice.com/#]enclomiphene[/url] enclomiphene buy
Мы предлагаем оформление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4304 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom6.ru/]Где купить диплом[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2433 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom1.su/]Купить срочно диплом о высшем образовании вуза[/url] — ответим быстро, без лишних формальностей.
tadalafilo 5 mg para que sirve: precio elocom crema – farmacia europea opiniones
Farmacia Asequible: pharma tgm – ozempic farmacГ©utica
https://rxfreemeds.shop/# RxFree Meds
https://rxfreemeds.com/# RxFree Meds
enclomiphene buy [url=http://enclomiphenebestprice.com/#]enclomiphene buy[/url] enclomiphene price
tramadol insomnio: Farmacia Asequible – Farmacia Asequible
https://rxfreemeds.com/# discount pharmacy
You could definitely see your enthusiasm in the paintings you write. The sector hopes for more passionate writers such as you who are not afraid to mention how they believe. All the time go after your heart. “What power has law where only money rules.” by Gaius Petronius.
RxFree Meds: RxFree Meds – RxFree Meds
enclomiphene price [url=http://enclomiphenebestprice.com/#]enclomiphene online[/url] enclomiphene citrate
https://enclomiphenebestprice.shop/# enclomiphene testosterone
More blogs like this would make the online space a better place.
Farmacia Asequible: Farmacia Asequible – casenlax o movicol
https://enclomiphenebestprice.com/# enclomiphene buy
Farmacia Asequible [url=https://farmaciaasequible.com/#]Farmacia Asequible[/url] Farmacia Asequible
https://enclomiphenebestprice.com/# buy enclomiphene online
parafsrmacia: thc med las palmas – Farmacia Asequible
RxFree Meds: RxFree Meds – online pharmacy quick delivery
http://rxfreemeds.com/# online pharmacy xalatan
You’ve clearly researched well.
fluconazole pharmacy online [url=http://rxfreemeds.com/#]Paxil[/url] RxFree Meds
Farmacia Asequible: Farmacia Asequible – farmacias grandes
http://rxfreemeds.com/# RxFree Meds
https://rxfreemeds.com/# propecia online pharmacy no prescription
You’ve clearly put in effort.
RxFree Meds [url=https://rxfreemeds.com/#]RxFree Meds[/url] RxFree Meds
enclomiphene price: enclomiphene best price – enclomiphene price
Farmacia Asequible: farmacia isla – mejor mГ©dico digestivo palma de mallorca
Thanks for publishing. It’s top quality.
https://enclomiphenebestprice.shop/# enclomiphene testosterone
buy enclomiphene online: enclomiphene – enclomiphene testosterone
enclomiphene citrate [url=http://enclomiphenebestprice.com/#]enclomiphene buy[/url] enclomiphene citrate
iraltone forte melatonin amazon: crema diprogenta – Farmacia Asequible
https://rxfreemeds.shop/# ed meds online
[url=http://kupit-drova-v-spb-365.ru]5 кубов дров цена[/url] .
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1840 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://spbrcom17.ru/]Узнать подробнее[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3581 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://spbrcom18.ru/]Пишите нам[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4641 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://spbrcom20.ru/]Купить диплом о высшем образовании[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2845 клиентов воспользовались услугой — теперь ваша очередь.
[url=https://inforepetitor16.ru/]Дипломы о высшем образовании купить[/url] — ответим быстро, без лишних формальностей.
enclomiphene for sale: enclomiphene for sale – buy enclomiphene online
Оформиление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2172 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor20.ru/]Купить дипломы о высшем образовании[/url] — ответим быстро, без лишних формальностей.
https://farmaciaasequible.com/# ozempic preis spanien
buy enclomiphene online [url=https://enclomiphenebestprice.com/#]enclomiphene[/url] enclomiphene online
Farmacia Asequible: Farmacia Asequible – Farmacia Asequible
I absolutely admired the style this was presented.
Оформиление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1943 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://spbrcom20.ru/]Мы поможем[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4863 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://spbrcom17.ru/]Дипломы о высшем образовании купить[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2181 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://inforepetitor17.ru/]Пишите в личные сообщения[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2093 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://spbrcom16.ru/]Диплом купить[/url] — ответим быстро, без лишних формальностей.
RxFree Meds [url=http://rxfreemeds.com/#]RxFree Meds[/url] aetna rx pharmacy
https://rxfreemeds.com/# RxFree Meds
ozempic precio espaГ±a: farmscia online – Farmacia Asequible
This is the kind of writing I enjoy reading.
enclomiphene for sale: enclomiphene best price – enclomiphene for sale
buy enclomiphene online [url=https://enclomiphenebestprice.com/#]enclomiphene buy[/url] enclomiphene for sale
enclomiphene: buy enclomiphene online – enclomiphene online
https://farmaciaasequible.shop/# farmacia economica
RxFree Meds: RxFree Meds – inhouse pharmacy viagra
http://farmaciaasequible.com/# farmqcias
enclomiphene buy [url=https://enclomiphenebestprice.shop/#]enclomiphene buy[/url] enclomiphene online
I gained useful knowledge from this.
enclomiphene best price: enclomiphene for sale – enclomiphene citrate
farmacia online viagra: champГє klorane opiniones – farmacia mГЎlaga cerca de mi
http://farmaciaasequible.com/# Farmacia Asequible
rx crossroads pharmacy refill [url=http://rxfreemeds.com/#]RxFree Meds[/url] RxFree Meds
RxFree Meds: RxFree Meds – RxFree Meds
https://rxfreemeds.com/# RxFree Meds
https://rxfreemeds.shop/# freds pharmacy store hours
[url=https://z1.anime-hd.online/anime-serials/8412-zapreschennyj-krot-2025.html]аниме Запрещённый крот[/url]
[url=https://z1.anime-hd.online/anime-serials/8413-geroj-v-obmoroke-i-princessy-ubijcy-2025.html]Герой в обмороке и принцессы-убийцы[/url]
[url=https://z1.anime-hd.online/anime-serials/8414-dragocennosti-ruri-2025.html]Драгоценности Рури смотреть аниме[/url]
[url=https://z1.anime-hd.online/anime-serials/8415-mag-vody-2025.html]Маг воды смотреть аниме[/url]
[url=https://z1.anime-hd.online/anime-serials/8416-apokalipsis-minogry-2025.html]аниме Апокалипсис Миногры[/url]
[url=https://z1.anime-hd.online/anime-serials/8431-neobjatnyj-okean-tv-2-2025.html]Необъятный океан 2 сезон[/url]
[url=https://z1.anime-hd.online/anime-serials/8439-voshozhdenie-geroja-schita-tv-4-2025.html]Восхождение Героя щита 4 сезон[/url]
[url=https://z1.anime-hd.online/anime-serials/8440-ja-perevoplotilsja-v-sedmogo-princa-tv-2-2024.html]Я перевоплотился в седьмого принца 2 сезон[/url]
[url=https://z1.anime-hd.online/anime-serials/8386-adskij-uchitel-nubje-tv-2-chast-1-2025.html]Адский учитель Нубэ 2025[/url]
[url=https://z1.anime-hd.online/anime-serials/8382-tradicii-kuhni-duhov-2025.html]традиции кухни духов аниме[/url]
[url=https://z1.anime-hd.online/anime-serials/8381-devushka-na-chas-tv-4-2025.html]Девушка на час аниме[/url]
[url=https://z1.anime-hd.online/anime-serials/8380-s-nyneshnimi-detektivami-nichego-ne-podelaesh-2025.html]с нынешними детективами ничего не поделаешь[/url]
[url=https://z1.anime-hd.online/anime-serials/8390-dozhd-i-ty-2025.html]Дождь и ты смотреть аниме[/url]
[url=https://z1.anime-hd.online/anime-serials/8411-arknajts-tv-3-2025.html]Аркнайтс 3 сезон[/url]
[url=https://z1.anime-hd.online/anime-serials/8402-plohaja-devochka-2025.html]Плохая девочка смотреть аниме[/url]
[url=https://z1.anime-hd.online/anime-serials/8395-pesn-nochnyh-sov-tv-2-2025.html]Песнь ночных сов 2 сезон[/url]
enclomiphene [url=https://enclomiphenebestprice.com/#]enclomiphene for men[/url] enclomiphene best price
Farmacia Asequible: cepillo oral b io 7 – farmacia 1
online pharmacy painkillers: RxFree Meds – silk road online pharmacy
http://medismartpharmacy.com/# boots pharmacy antibiotic eye drops chloramphenicol
MexiMeds Express [url=http://meximedsexpress.com/#]mexican rx online[/url] buying prescription drugs in mexico online
https://indomedsusa.com/# IndoMeds USA
top online pharmacy india: IndoMeds USA – india online pharmacy
https://meximedsexpress.com/# medicine in mexico pharmacies
24 7 online pharmacy [url=https://medismartpharmacy.com/#]cheap scripts pharmacy[/url] 24 hr pharmacy
rx pharmacy phone number: pro cialis pharmacy – what does viagra cost at a pharmacy
canadian pharmacy tampa: top mail order pharmacies – canadian valley pharmacy
http://indomedsusa.com/# india pharmacy mail order
http://medismartpharmacy.com/# online pharmacy tetracycline
best online pharmacies in mexico: buying from online mexican pharmacy – buying prescription drugs in mexico
canadian world pharmacy: MediSmart Pharmacy – rate canadian pharmacies
Мы предлагаем оформление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3762 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom13.ru/]Купить диплом университета[/url] — ответим быстро, без лишних формальностей.
Оформиление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1773 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://poligraf18.ru/]Продажа дипломов[/url] — ответим быстро, без лишних формальностей.
Оформиление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2627 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://poligraf17.ru/]Купить диплом нового образца[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3099 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom11.ru/]Где купить диплом о высшем образовании[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2636 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://poligraf21.ru/]По ссылке[/url] — ответим быстро, без лишних формальностей.
You’ve clearly done your homework.
MexiMeds Express [url=http://meximedsexpress.com/#]MexiMeds Express[/url] MexiMeds Express
IndoMeds USA: indian pharmacy – top 10 online pharmacy in india
http://indomedsusa.com/# cheapest online pharmacy india
mexican pharmaceuticals online: MexiMeds Express – MexiMeds Express
http://indomedsusa.com/# IndoMeds USA
Мы предлагаем оформление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1310 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom14.ru/]Купить дипломы о высшем[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 944 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://poligraf17.ru/]Купить диплом о высшем образовании недорого[/url] — ответим быстро, без лишних формальностей.
MexiMeds Express: mexican rx online – MexiMeds Express
Мы предлагаем оформление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2517 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom12.ru/]Здесь[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4508 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom11.ru/]Купить срочно диплом о высшем образовании вуза[/url] — ответим быстро, без лишних формальностей.
best provigil pharmacy [url=http://medismartpharmacy.com/#]MediSmart Pharmacy[/url] actos pharmacy assistance
https://meximedsexpress.com/# MexiMeds Express
MexiMeds Express: MexiMeds Express – mexico pharmacies prescription drugs
I found new insight from this.
mexican rx online: MexiMeds Express – MexiMeds Express
indian pharmacy paypal [url=http://indomedsusa.com/#]Online medicine home delivery[/url] buy medicines online in india
cheapest online pharmacy india: IndoMeds USA – IndoMeds USA
http://indomedsusa.com/# reputable indian online pharmacy
MexiMeds Express [url=https://meximedsexpress.com/#]п»їbest mexican online pharmacies[/url] mexican drugstore online
medication from mexico pharmacy: MexiMeds Express – MexiMeds Express
http://indomedsusa.com/# world pharmacy india
IndoMeds USA [url=https://indomedsusa.com/#]india pharmacy mail order[/url] top 10 online pharmacy in india
https://indomedsusa.shop/# IndoMeds USA
https://medismartpharmacy.shop/# mexico online pharmacy reviews
tadacip online pharmacy: Benemid – asda pharmacy mefloquine
п»їbest mexican online pharmacies [url=https://meximedsexpress.com/#]MexiMeds Express[/url] reputable mexican pharmacies online
buying prescription drugs in mexico: MexiMeds Express – mexico drug stores pharmacies
https://medismartpharmacy.com/# mobile rx pharmacy
IndoMeds USA: Online medicine order – indian pharmacy paypal
MexiMeds Express [url=https://meximedsexpress.com/#]mexico drug stores pharmacies[/url] medicine in mexico pharmacies
buy fb account ready-made accounts for sale profitable account sales
medication from mexico pharmacy: mexican online pharmacies prescription drugs – mexican rx online
dutasteride from dr reddy’s or inhouse pharmacy: MediSmart Pharmacy – pharmacy on line
https://medismartpharmacy.shop/# online shopping pharmacy india
IndoMeds USA [url=https://indomedsusa.shop/#]indian pharmacy paypal[/url] Online medicine order
http://meximedsexpress.com/# buying prescription drugs in mexico online
percocet mexico pharmacy: humana pharmacy online – online pharmacy orlistat
Мы предлагаем оформление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 2610 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom15.ru/]Где можно купить диплом[/url] — ответим быстро, без лишних формальностей.
super saver pharmacy [url=https://medismartpharmacy.com/#]MediSmart Pharmacy[/url] how to start a pharmacy store
Покупка дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4286 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://diplomykupit2.ru/]На сайте[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1299 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://diplomygoznak1.ru/]Уточнить здесь[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3366 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://kupludiplom1.ru/]Куплю диплом[/url] — ответим быстро, без лишних формальностей.
https://indomedsusa.com/# online pharmacy india
online shopping pharmacy india: IndoMeds USA – indian pharmacy online
prilosec people’s pharmacy [url=https://medismartpharmacy.com/#]MediSmart Pharmacy[/url] sporanox online pharmacy
Мы предлагаем оформление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3858 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://uadiplom15.ru/]Купить учебный диплом[/url] — ответим быстро, без лишних формальностей.
http://medismartpharmacy.com/# mail pharmacy
https://medismartpharmacy.com/# percocet pharmacy cost
Мы предлагаем оформление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4250 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://diplomygoznak.su/]На этой странице[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4526 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://diplomygoznak1.ru/]Купить учебный диплом[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1547 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://kupludiplom1.ru/]Купить диплом специалиста недорого[/url] — ответим быстро, без лишних формальностей.
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3642 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://kupludiplom.su/]Где можно купить диплом о высшем образовании[/url] — ответим быстро, без лишних формальностей.
Мы предлагаем оформление дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1583 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://diplomykupit3.ru/]Купить диплом о высшем образовании Украины[/url] — ответим быстро, без лишних формальностей.
buying prescription drugs in mexico online: MexiMeds Express – mexican border pharmacies shipping to usa
MexiMeds Express [url=https://meximedsexpress.shop/#]mexican border pharmacies shipping to usa[/url] mexico drug stores pharmacies
http://indomedsusa.com/# legitimate online pharmacies india
facebook account sale social media account marketplace account selling platform
Dulcolax: MediSmart Pharmacy – rx discount pharmacy
Оформиление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3707 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://diplomykupit3.ru/]Купить диплом недорого[/url] — ответим быстро, без лишних формальностей.
MexiMeds Express [url=https://meximedsexpress.com/#]MexiMeds Express[/url] MexiMeds Express
услуги стоматологической клиники https://stomatologiya-arhangelsk-1.ru .
багги 6 местный багги 6 местный .
medicine in mexico pharmacies: medicine in mexico pharmacies – mexico pharmacies prescription drugs
https://meximedsexpress.com/# MexiMeds Express
https://medismartpharmacy.com/# compounding pharmacy online
IndoMeds USA [url=http://indomedsusa.com/#]Online medicine order[/url] IndoMeds USA
п»їlegitimate online pharmacies india: online shopping pharmacy india – best india pharmacy
https://indomedsusa.shop/# mail order pharmacy india
pernazene sans ordonnance: PharmaDirecte – peut on acheter de la vitamine d en pharmacie sans ordonnance
http://clinicagaleno.com/# farmacia trebol compra online
https://pharmadirecte.shop/# test angine pharmacie sans ordonnance prix
guantes desechables farmacia online: Clinica Galeno – como comprar cialis sin receta
[comprar pildora dia despues sin receta]: Clinica Galeno – comprar dolethal sin receta
http://pharmadirecte.com/# cystite traitement pharmacie sans ordonnance
pantorc 40 mg prezzo senza ricetta [url=https://ordinasalute.com/#]spasmex compresse prezzo[/url] farmacia clemente
farmacia online cadiz: Clinica Galeno – farmacia bebe online
https://pharmadirecte.shop/# somnifere sans ordonnance en pharmacie
la viagra se puede comprar sin receta en espaГ±a [url=https://clinicagaleno.com/#]Clinica Galeno[/url] farmacia del centro online
orl ordonnance ou pas: PharmaDirecte – tramadol sans ordonnance forum
farmacia online topasel: mi farmacia online en casa – fluimucil forte se puede comprar sin receta
https://ordinasalute.shop/# ursobil 300 prezzo
https://clinicagaleno.shop/# farmacia espanhola online
lyrica 75 mg prezzo: OrdinaSalute – lenizak a cosa serve
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 3131 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://diplomygoznak4.ru/]Купить настоящий диплом[/url] — ответим быстро, без лишних формальностей.
Оформиление дипломов ВУЗов В киеве — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы гарантируем, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 4019 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://diplomygoznak3.ru/]Купить диплом об образовании[/url] — ответим быстро, без лишних формальностей.
Hello lovers of clean ambiance !
Cat parents love using an air purifier for cat hair near litter boxes to eliminate smells and airborne litter dust. The air purifier for dog smell is most effective when placed near dog beds or play areas. Using the best air filter for pet hair also reduces the workload on your HVAC system over time.
The best air filters for pets work well in bedrooms to provide allergen-free rest and better sleep quality. Top rated air purifiers for pets frequently include child safety locks and tip-over protection for households with kids.cat air purifierYou’ll immediately notice a difference in air freshness with the best air purifier for pet allergies.
Air Purifier for Dog Hair That Lasts Long – п»їhttps://www.youtube.com/watch?v=dPE254fvKgQ
May you enjoy remarkable energizing surroundings !
Покупка дипломов ВУЗов по всей Украине — с печатями, подписями, приложением и возможностью архивной записи (по запросу).
Документ максимально приближен к оригиналу и проходит визуальную проверку.
Мы даем гарантию, что в случае проверки документа, подозрений не возникнет.
– Конфиденциально
– Доставка 3–7 дней
– Любая специальность
Уже более 1842 клиентов воспользовались услугой — теперь ваша очередь.
[url=http://kupludiplom2.ru/]Купить диплом Украины[/url] — ответим быстро, без лишних формальностей.
http://pharmadirecte.com/# test coqueluche pharmacie sans ordonnance
mywy pillola [url=http://ordinasalute.com/#]OrdinaSalute[/url] cefixoral 400 mg prezzo
https://clinicagaleno.com/# glutaraldehido farmacia online