Developing a WordPress REST API App
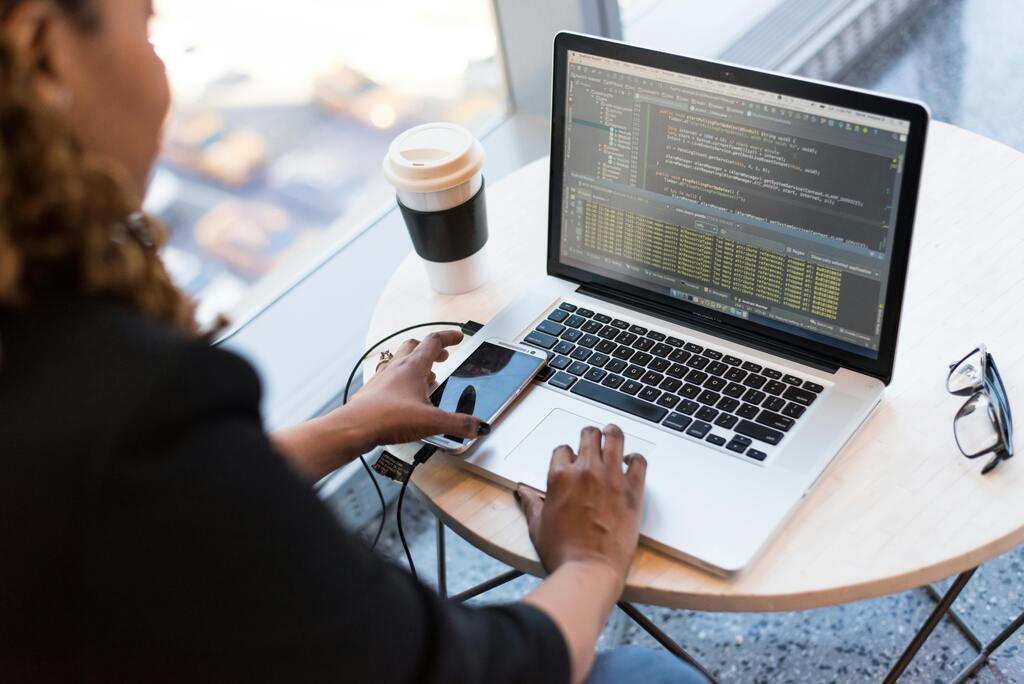
Developing a WordPress REST API App: Getting Started
WordPress, known primarily as a content management system (CMS), has grown far beyond its blogging roots to become a versatile platform for building dynamic websites and applications. One of the most powerful features that WordPress offers developers is its REST API, which allows you to interact with your WordPress site programmatically. In this guide, we’ll explore how to develop a WordPress REST API app, starting from the basics and moving to a practical example.
What is the WordPress REST API?
The WordPress REST API provides a standardized way for external applications to interact with WordPress sites. It allows you to create, read, update, and delete (CRUD) content through HTTP requests. This capability opens up endless possibilities, from integrating WordPress with other systems to building mobile apps that leverage WordPress as a backend.
Prerequisites
Before we dive in, make sure you have the following:
- WordPress Site: A running WordPress site (version 4.7 or later).
- Development Environment: Basic knowledge of JavaScript, PHP, and RESTful APIs.
- Tools: A code editor, a tool for making HTTP requests (like Postman), and a local development environment like XAMPP or MAMP if you’re not working on a live site.
Step 1: Understanding the Basics
The REST API lets you interact with WordPress by sending HTTP requests to specific endpoints. These endpoints correspond to various resources such as posts, pages, comments, users, and more.
For example, to retrieve a list of posts, you can send a GET request to:
http://your-site.com/wp-json/wp/v2/posts
To create a new post, you would send a POST request to the same endpoint, including the necessary data in the request body.
Step 2: Setting Up Authentication
To perform certain actions (like creating or updating content), you’ll need to authenticate your requests. There are several authentication methods available, including cookies, OAuth, and application passwords.
For simplicity, we’ll use application passwords, which were introduced in WordPress 5.6.
- Enable Application Passwords: Go to your WordPress admin dashboard, navigate to Users > Profile, and scroll down to the “Application Passwords” section.
- Generate a Password: Enter a name for your application and click “Add New Application Password”. Copy the generated password and keep it secure.
Step 3: Making Your First API Request
With authentication set up, let’s make a few basic API requests.
Get All Posts:
Use a tool like Postman or Curl to send a GET request to:
http://your-site.com/wp-json/wp/v2/posts
This will return a JSON response containing all posts.
Create a New Post:
Send a POST request to the same endpoint. Include the application password for authentication and the post data in the request body.
Example with curl:
curl --user your-username:application-password -X POST -d "title=My New Post&content=This is the content of my new post&status=publish" http://your-site.com/wp-json/wp/v2/posts
This will create a new post with the specified title and content.
Step 4: Building a Frontend App
Now that we’ve covered the basics, let’s build a simple front-end application that interacts with our WordPress site using the REST API. We’ll use JavaScript and the Fetch API to demonstrate this.
Setup:
Create a new directory for your project and set up an index.html
file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>WordPress REST API App</title>
</head>
<body>
<h1>WordPress REST API App</h1>
<div id="posts"></div>
<script src="app.js"></script>
</body>
</html>
Fetching Posts:
Create a file named app.js
and add the following code to fetch and display posts:
const apiUrl = 'http://your-site.com/wp-json/wp/v2/posts';
async function fetchPosts() {
try {
const response = await fetch(apiUrl);
const posts = await response.json();
displayPosts(posts);
} catch (error) {
console.error('Error fetching posts:', error);
}
}
function displayPosts(posts) {
const postsContainer = document.getElementById('posts');
posts.forEach(post => {
const postElement = document.createElement('div');
postElement.innerHTML = `
<h2>${post.title.rendered}</h2>
<p>${post.content.rendered}</p>
`;
postsContainer.appendChild(postElement);
});
}
fetchPosts();
Replace http://your-site.com
with your actual WordPress site URL. This script fetches the latest posts from your WordPress site and displays them on the page.
Creating a New Post:
To allow users to create new posts from your frontend app, add a form to your index.html
:
<form id="postForm">
<input type="text" id="title" placeholder="Title" required>
<textarea id="content" placeholder="Content" required></textarea>
<button type="submit">Create Post</button>
</form>
Update app.js
to handle form submissions:
document.getElementById('postForm').addEventListener('submit', async function (e) {
e.preventDefault();
const title = document.getElementById('title').value;
const content = document.getElementById('content').value;
const apiUrl = 'http://your-site.com/wp-json/wp/v2/posts';
const post = {
title: title,
content: content,
status: 'publish'
};
try {
const response = await fetch(apiUrl, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Basic ' + btoa('your-username:application-password')
},
body: JSON.stringify(post)
});
if (response.ok) {
const newPost = await response.json();
displayPosts([newPost]);
} else {
console.error('Error creating post:', response.statusText);
}
} catch (error) {
console.error('Error:', error);
}
});
This script listens for form submissions, sends a POST request to create a new post, and displays the newly created post on the page. Make sure to replace 'your-username:application-password'
it with your actual credentials.
Step 5: Securing Your App
Security is paramount when dealing with APIs. Here are a few best practices:
- Use HTTPS: Ensure your WordPress site uses HTTPS to encrypt data between the client and server.
- Validate Inputs: Always validate and sanitize user inputs to prevent SQL injection and other attacks.
- Use Nonces: WordPress provides nonce functions to protect against CSRF attacks.
- Limit Permissions: Use the principle of least privilege. Create a user role with only the necessary permissions for API interactions.
Conclusion
Developing a WordPress REST API app opens up a world of possibilities for creating dynamic, interactive applications. This guide provided a starting point for understanding and utilizing the WordPress REST API. By following these steps, you can build powerful applications that leverage the flexibility and capabilities of WordPress, all while ensuring your app is secure and performant. Happy coding!
Очень полезная статья о проектировании домов!
Меня всегда привлекала эта тема, и приятно видеть,
что есть компании, которые относятся к своей работе так профессионально.
Особенно впечатлил индивидуальный подход Stroyplans к каждому проекту.
Думаю, это ключевой фактор в создании идеального дома.
Кстати, недавно обратил внимание на их сайт Stroyplans,
там есть дополнительные примеров проектов.
Рекомендую заглянуть всем, кто задумывается о проектировании индивидуального
жилья. Благодарю за информативный обзор!
As a serious golfer, I can not stress enough exactly how critical the ideal devices are to not only enjoying the game but
also boosting your efficiency on the course. Golf is as much a
mental game as it is physical, and having the proper gear can truly
make a distinction in exactly how you come close to each round.
To start with, allow’s speak about golf gloves.
Lots of players undervalue the relevance of an excellent handwear cover.
It’s not just about preventing sores; a handwear cover assists you maintain a constant grip on your club,
which is crucial for regulating your shots. Particularly in damp or damp problems,
an excellent handwear cover can be the difference in between a strong
drive and a wild piece. Gloves with a snug fit
made from top notch natural leather tend to supply the most effective efficiency.
They mold and mildew to your turn over time, giving both convenience and
integrity.
One more commonly neglected device is the golf tee. Yes, it’s a tiny item, yet the ideal tee can really affect your game.
As an example, using longer tees with your chauffeur
can help you achieve better launch angles, while much shorter tees are excellent
when you need even more control with your irons. Directly, I favor using naturally degradable
or plastic tees since they’re extra sturdy and green.
Golf bags are another vital that deserve more interest than they usually obtain. A
well-designed golf bag isn’t practically style–
it’s about functionality and comfort. If you like walking the training course, a lightweight stand
bag with excellent storage options can make your
round a lot more pleasurable. On the other hand,
if you’re riding in a cart, a cart bag with a lot of area for all
your gear is a must. The best golf bag helps you stay arranged,
which is important when you require to focus
on your video game.
One device that’s ending up being increasingly preferred is the
rangefinder. I can’t tell you how much this little device has
actually boosted my game. Recognizing the precise
distance to the pin or dangers enables you to
choose the right club with self-confidence. Whether you opt for a laser rangefinder or
a GPS one, the accuracy these devices give is important.
Lastly, never undervalue the value of a good golf towel and umbrella.
A towel keeps your clubs and balls clean, ensuring far better call and precision, while an umbrella is a lifesaver during unexpected weather condition changes.
These small things contribute to the overall top quality
of your video game.
To conclude, buying the appropriate golf devices is vital for anybody significant regarding
boosting their game. Whether it’s a handwear cover that fits just right,
a durable golf bag, or a high-tech rangefinder, having the right
tools can make every round more enjoyable and effective.
For any individual seeking in-depth reviews and expert
recommendations on the most effective golf
equipment, I highly recommend looking into https://vk.com/@730398274-in-depth-golf-club-reviews-which-clubs-can-take-your-game-to.
Tangchirakhaphan S, Innajak S, Nilwarangkoon S, Tanjapatkul N, Mahabusrakum W, Watanapokasin R buy priligy generic
Ищите в гугле
does lasix lower blood pressure 1016 S0140- 6736 99 05203- 4 PubMed CrossRef Google Scholar
Your article helped me a lot, is there any more related content? Thanks!
Can you be more specific about the content of your enticle? After reading it, I still have some doubts. Hope you can help me.
Your article helped me a lot, is there any more related content? Thanks!
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me. https://accounts.binance.info/en/register?ref=JHQQKNKN
Looking for expert guidance on protecting assets while qualifying for Medicaid? As experienced elder law attorneys near me, our team at Ohio Medicaid Lawyers provides specialized legal assistance with Medicaid planning, estate planning, and asset protection strategies. We help seniors understand medicaid eligibility income charts and navigate the complex 5-year lookback period. Visit our website for comprehensive information about Ohio medicaid income limits 2024 and schedule a consultation with a trusted elder care attorney who can safeguard your future.
Need help with preparation for long-term care? Our legal team provides comprehensive Medicaid strategies.
For those navigating UK immigration law, finding a highly rated immigration solicitor in London can change everything. These experts deal with everything from Tier 2 sponsorships to tribunal hearings. With dedicated representation and ongoing support, they provide clarity in what can be a confusing process.
Your article helped me a lot, is there any more related content? Thanks!
Thinking about a secured loan to consolidate your financial obligations? Find out more and see what solutions may be available to you.
If you’re a property owner looking to borrow money, a secured loan could be a wise option. Access better rates by using your home as security.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
kamagra gel: Achetez vos kamagra medicaments – kamagra gel
kamagra livraison 24h: kamagra livraison 24h – Acheter Kamagra site fiable
п»їpharmacie en ligne france: Pharmacies en ligne certifiees – acheter mГ©dicament en ligne sans ordonnance pharmafst.com
Acheter Kamagra site fiable: acheter kamagra site fiable – Achetez vos kamagra medicaments
https://kamagraprix.shop/# Kamagra Oral Jelly pas cher
pharmacie en ligne pas cher Meilleure pharmacie en ligne Pharmacie sans ordonnance pharmafst.shop
Cialis en ligne: Tadalafil achat en ligne – Tadalafil achat en ligne tadalmed.shop
Kamagra pharmacie en ligne: kamagra en ligne – Kamagra Oral Jelly pas cher
http://tadalmed.com/# cialis prix
pharmacie en ligne france pas cher [url=https://pharmafst.com/#]pharmacie en ligne sans ordonnance[/url] Pharmacie Internationale en ligne pharmafst.shop
http://tadalmed.com/# Acheter Cialis 20 mg pas cher
kamagra en ligne: kamagra pas cher – kamagra livraison 24h
Pharmacie Internationale en ligne: Meilleure pharmacie en ligne – trouver un mГ©dicament en pharmacie pharmafst.com
acheter kamagra site fiable achat kamagra kamagra livraison 24h
kamagra pas cher: Acheter Kamagra site fiable – kamagra 100mg prix
Pharmacie en ligne livraison Europe: acheter mГ©dicament en ligne sans ordonnance – pharmacies en ligne certifiГ©es pharmafst.com
https://tadalmed.shop/# Acheter Viagra Cialis sans ordonnance
acheter kamagra site fiable kamagra gel kamagra livraison 24h
Tadalafil sans ordonnance en ligne: cialis sans ordonnance – Achat Cialis en ligne fiable tadalmed.shop
Cialis sans ordonnance 24h Acheter Cialis Tadalafil 20 mg prix sans ordonnance tadalmed.com
kamagra 100mg prix: acheter kamagra site fiable – kamagra livraison 24h
mexican rx online: mexico pharmacies prescription drugs – mexican rx online
indian pharmacy buy medicines online in india indian pharmacy online shopping
MedicineFromIndia: Medicine From India – indian pharmacy online
RxExpressMexico: Rx Express Mexico – mexico pharmacies prescription drugs
Rx Express Mexico: mexican rx online – mexican rx online
https://expressrxcanada.shop/# buy drugs from canada
global pharmacy canada Express Rx Canada reliable canadian pharmacy reviews
canadian pharmacy victoza: Express Rx Canada – buy prescription drugs from canada cheap
safe online pharmacies in canada: Express Rx Canada – ed meds online canada
reliable canadian pharmacy: canadian discount pharmacy – canada pharmacy online legit
http://medicinefromindia.com/# indian pharmacy
RxExpressMexico: mexican rx online – mexico pharmacies prescription drugs
mexico drug stores pharmacies RxExpressMexico mexico pharmacy order online
mexican rx online: Rx Express Mexico – mexico pharmacies prescription drugs
Online medicine home delivery: Medicine From India – indian pharmacy online shopping
https://expressrxcanada.shop/# legitimate canadian pharmacies
reddit canadian pharmacy: ExpressRxCanada – online canadian pharmacy
Rx Express Mexico: Rx Express Mexico – RxExpressMexico
indian pharmacy indian pharmacy online shopping indian pharmacy online
indian pharmacy: indian pharmacy online – Medicine From India
https://medicinefromindia.shop/# indian pharmacy
canadian pharmacy ratings: Canadian pharmacy shipping to USA – canadian pharmacy antibiotics
indian pharmacy online shopping: mail order pharmacy india – medicine courier from India to USA
mexican online pharmacy: Rx Express Mexico – mexico drug stores pharmacies
mexico drug stores pharmacies: mexican online pharmacy – mexico drug stores pharmacies
http://rxexpressmexico.com/# mexican online pharmacy
canada drugs online review Express Rx Canada canadian pharmacy 365
canadian pharmacy tampa: best canadian pharmacy to order from – thecanadianpharmacy
mexico pharmacies prescription drugs: mexico pharmacies prescription drugs – mexican online pharmacy
https://expressrxcanada.com/# ordering drugs from canada
indian pharmacy online medicine courier from India to USA medicine courier from India to USA
medicine courier from India to USA: indian pharmacy online – medicine courier from India to USA
prescription drugs canada buy online: Express Rx Canada – legit canadian online pharmacy
canadian pharmacy cheap: Canadian pharmacy shipping to USA – global pharmacy canada
pin up pin up az pin up
пин ап казино: пин ап вход – пин ап зеркало
http://pinuprus.pro/# пин ап зеркало
вавада официальный сайт: vavada – вавада казино
вавада зеркало: vavada casino – вавада казино
pin-up: pin up – pin-up
пин ап вход: pin up вход – пин ап вход
вавада официальный сайт: vavada вход – вавада зеркало
pin up: pin-up casino giris – pin up az
pin up вход: пин ап вход – пинап казино
vavada: vavada вход – vavada вход
вавада зеркало: вавада официальный сайт – vavada вход
площадка для продажи аккаунтов https://birzha-akkauntov-online.ru
pin-up casino giris: pin-up casino giris – pin up
заработок на аккаунтах покупка аккаунтов
безопасная сделка аккаунтов маркетплейс для реселлеров
маркетплейс аккаунтов соцсетей магазин аккаунтов социальных сетей
вавада официальный сайт: vavada – vavada вход
площадка для продажи аккаунтов продажа аккаунтов соцсетей
купить аккаунт с прокачкой https://kupit-akkaunt-top.ru/
маркетплейс аккаунтов соцсетей https://pokupka-akkauntov-online.ru/
пин ап вход: пин ап вход – пин ап казино официальный сайт
пин ап казино официальный сайт: пин ап казино – пин ап вход
Account Trading Service https://buyverifiedaccounts001.com
Account Acquisition Purchase Ready-Made Accounts
пинап казино: пин ап вход – пин ап зеркало
Account Trading Verified Accounts for Sale
Buy accounts Gaming account marketplace
Accounts market Marketplace for Ready-Made Accounts
Account Trading Account trading platform
Account Selling Service Accounts marketplace
пин ап казино: пин ап казино – пинап казино
Account market Account Buying Service
вавада казино: vavada – vavada
Account Trading Service https://accountsmarketplaceonline.com/
Account Exchange Service Account trading platform
Account exchange Ready-Made Accounts for Sale
пинап казино: пинап казино – пин ап зеркало
pinup az: pin up azerbaycan – pin-up casino giris
sell accounts account exchange service
ready-made accounts for sale account trading platform
ready-made accounts for sale secure account sales
account catalog secure account sales
pin up casino: pin up az – pin up
account trading service accounts market
buy and sell accounts account market
account trading buy account
accounts for sale account buying service
This piece of writing will help the internet people for creating new web site or even a blog
from start to end.
My web site – nordvpn coupons inspiresensation
sell pre-made account https://discountaccountsmarket.com/
secure account sales https://accounts-marketplace.org/
account trading platform account selling platform
account selling platform https://buy-soc-accounts.org/
account market accounts marketplace
sell pre-made account buy accounts
account trading service sell account
account marketplace buy-social-accounts.org
account acquisition https://social-accounts-marketplace.org/
account trading platform account acquisition
sell pre-made account website for selling accounts
ready-made accounts for sale buy pre-made account
buy account account store
sell accounts guaranteed accounts
buy account account acquisition
account selling service account selling service
buy accounts social media account marketplace
purchase ready-made accounts account buying service
ready-made accounts for sale accounts market
account trading platform account sale
safe modafinil purchase: modafinil legality – buy modafinil online
http://maxviagramd.com/# trusted Viagra suppliers
discreet shipping ED pills: best price Cialis tablets – best price Cialis tablets
no doctor visit required: safe online pharmacy – buy generic Viagra online
https://maxviagramd.com/# discreet shipping
modafinil legality: modafinil pharmacy – doctor-reviewed advice
discreet shipping ED pills cheap Cialis online generic tadalafil
modafinil pharmacy: verified Modafinil vendors – modafinil 2025
profitable account sales https://accounts-offer.org/
account sale https://accounts-marketplace.xyz
http://maxviagramd.com/# generic sildenafil 100mg
nordvpn coupon 350fairfax
Have you ever considered publishing an ebook or guest authoring on other blogs?
I have a blog based on the same ideas you discuss and would love to
have you share some stories/information. I know my readers would appreciate your work.
If you’re even remotely interested, feel free
to shoot me an email.
find accounts for sale https://buy-best-accounts.org
buy generic Viagra online: secure checkout Viagra – safe online pharmacy
generic tadalafil: online Cialis pharmacy – generic tadalafil
ready-made accounts for sale https://social-accounts-marketplaces.live
cheap Viagra online: buy generic Viagra online – cheap Viagra online
secure checkout ED drugs: reliable online pharmacy Cialis – online Cialis pharmacy
secure account purchasing platform account marketplace
secure checkout ED drugs reliable online pharmacy Cialis FDA approved generic Cialis
website for selling accounts https://social-accounts-marketplace.xyz
purchase ready-made accounts https://buy-accounts.space
generic tadalafil: best price Cialis tablets – buy generic Cialis online
generic tadalafil: order Cialis online no prescription – reliable online pharmacy Cialis
accounts for sale https://buy-accounts-shop.pro
https://zipgenericmd.shop/# generic tadalafil
doctor-reviewed advice: modafinil 2025 – modafinil 2025
cheap Viagra online: legit Viagra online – buy generic Viagra online
account exchange https://buy-accounts.live
buy pre-made account https://social-accounts-marketplace.live/
account trading https://accounts-marketplace.online
order Cialis online no prescription reliable online pharmacy Cialis order Cialis online no prescription
cheap Cialis online: Cialis without prescription – buy generic Cialis online
verified Modafinil vendors Modafinil for sale verified Modafinil vendors
account selling service https://accounts-marketplace-best.pro
купить аккаунт akkaunty-na-prodazhu.pro
площадка для продажи аккаунтов https://rynok-akkauntov.top/
secure checkout Viagra: safe online pharmacy – best price for Viagra
покупка аккаунтов https://kupit-akkaunt.xyz/
where to buy clomid without a prescription: Clom Health – can you buy cheap clomid prices
Amo Health Care: Amo Health Care – Amo Health Care
Amo Health Care: Amo Health Care – amoxicillin 250 mg price in india
https://prednihealth.shop/# PredniHealth
Amo Health Care Amo Health Care Amo Health Care
prednisone 20mg: purchase prednisone from india – PredniHealth
how to get prednisone without a prescription: PredniHealth – prednisone sale
PredniHealth: where to buy prednisone without prescription – PredniHealth
https://clomhealth.shop/# can you get clomid price
PredniHealth 50 mg prednisone canada pharmacy prednisone 20mg for sale
prednisone cost 10mg: PredniHealth – PredniHealth
покупка аккаунтов https://akkaunt-magazin.online
amoxicillin no prescription: Amo Health Care – Amo Health Care
купить аккаунт akkaunty-market.live
маркетплейс аккаунтов соцсетей https://kupit-akkaunty-market.xyz/
where to buy generic clomid without insurance: Clom Health – can i get generic clomid online
https://clomhealth.com/# get clomid tablets
buying clomid pills: Clom Health – cost of generic clomid pill
Amo Health Care amoxicillin order online no prescription amoxicillin 200 mg tablet
can i get clomid pill: how to get cheap clomid pills – cheap clomid pill
40 mg daily prednisone: prednisone 30 mg – buy prednisone no prescription
https://prednihealth.com/# can i order prednisone
PredniHealth: order prednisone from canada – PredniHealth
cost of cheap clomid pill cost of clomid no prescription how can i get clomid without a prescription
PredniHealth: prednisone tablets 2.5 mg – PredniHealth
https://prednihealth.shop/# buy prednisone online without a script
prednisone 10 tablet prednisone 10mg tablet cost prednisone 5093
PredniHealth: PredniHealth – PredniHealth
https://prednihealth.shop/# where to buy prednisone in canada
продажа аккаунтов https://akkaunty-optom.live/
купить аккаунт https://online-akkaunty-magazin.xyz
продать аккаунт https://akkaunty-dlya-prodazhi.pro
buy cheap clomid no prescription: Clom Health – how to buy generic clomid without rx
amoxicillin without a doctors prescription Amo Health Care buy amoxicillin online cheap
amoxicillin from canada: Amo Health Care – Amo Health Care
https://amohealthcare.store/# where can i get amoxicillin
clomid tablet: Clom Health – can you get clomid online
PredniHealth: prednisone 50 mg tablet cost – buy prednisone 20mg
prednisone nz canadian online pharmacy prednisone prednisone 5mg over the counter
маркетплейс аккаунтов https://kupit-akkaunt.online/
https://tadalaccess.com/# cialis generic online
cialis generic best price: TadalAccess – side effects cialis
cialis or levitra: TadalAccess – cialis for bph reviews
when will cialis be generic cialis professional 20 lowest price cialis ingredients
https://tadalaccess.com/# cheap cialis online tadalafil
cialis 80 mg dosage: cialis dosage reddit – how much does cialis cost with insurance
cialis genetic: cialis 10mg – cialis coupon 2019
evolution peptides tadalafil [url=https://tadalaccess.com/#]prescription for cialis[/url] is cialis covered by insurance
what does cialis do: prescription for cialis – online cialis prescription
https://tadalaccess.com/# cialis male enhancement
cialis online no prescription: Tadal Access – cialis sell
cialis dosage for bph: no prescription female cialis – no prescription tadalafil
https://tadalaccess.com/# cialis online overnight shipping
is tadalafil available in generic form TadalAccess cialis at canadian pharmacy
over the counter cialis walgreens: TadalAccess – cialis black in australia
cialis contraindications: Tadal Access – cialis for ed
https://tadalaccess.com/# cialis coupon walgreens
tadalafil without a doctor prescription TadalAccess is there a generic equivalent for cialis
cheap cialis for sale: Tadal Access – side effects of cialis tadalafil
difference between sildenafil and tadalafil: TadalAccess – cialis vs tadalafil
https://tadalaccess.com/# cheap tadalafil no prescription
when does tadalafil go generic TadalAccess cheap generic cialis canada
cialis online no prescription: TadalAccess – cialis tadalafil online paypal
cialis 20mg for sale: TadalAccess – cialis ontario no prescription
https://tadalaccess.com/# cialis dosage 20mg
generic cialis online pharmacy TadalAccess cialis free trial voucher
generic cialis tadalafil 20mg india: TadalAccess – tadalafil troche reviews
https://tadalaccess.com/# when will cialis be generic
buy accounts facebook buy facebook ad account
buy facebook profile buying facebook account
cialis 10mg price cialis paypal canada tadalafil tablets 40 mg
buy aged facebook ads account https://buy-ad-account.top/
where can i get cialis: TadalAccess – cheapest cialis online
shelf life of liquid tadalafil: cialis generic over the counter – cialis purchase canada
https://tadalaccess.com/# cialis over the counter at walmart
buying facebook account https://buy-ads-account.click
cialis online pharmacy: cialis store in philippines – cialis for daily use cost
https://tadalaccess.com/# how long does it take for cialis to start working
tadalafil review Tadal Access best reviewed tadalafil site
cialis generic canada: Tadal Access – buy cialis on line
cialis black: Tadal Access – how to get cialis prescription online
buy fb ad account https://ad-account-buy.top
buy accounts facebook https://buy-ads-account.work
buy facebook old accounts https://ad-account-for-sale.top
cialis for pulmonary hypertension how to get cialis prescription online what does cialis treat
cialis 5mg price comparison: Tadal Access – how long does cialis stay in your system
cialis online canada: TadalAccess – cialis prescription cost
https://tadalaccess.com/# how long before sex should i take cialis
cialis 5mg cost per pill: TadalAccess – buy cialis overnight shipping
buying cialis cialis generic canada canadian pharmacy tadalafil 20mg
vidalista tadalafil reviews: Tadal Access – cialis tadalafil discount
https://tadalaccess.com/# cialis manufacturer
buy facebook accounts cheap https://buy-ad-account.click
Эта публикация дает возможность задействовать различные источники информации и представить их в удобной форме. Читатели смогут быстро найти нужные данные и получить ответы на интересующие их вопросы. Мы стремимся к четкости и доступности материала для всех!
Ознакомиться с деталями – https://medalkoblog.ru/
https://tadalaccess.com/# ordering tadalafil online
buy cialis toronto generic cialis available in canada originalcialis
cialis reviews photos: cialis 80 mg dosage – cialis from mexico
cheap cialis 20mg: cialis 20 mg how long does it take to work – where to buy liquid cialis
https://tadalaccess.com/# do you need a prescription for cialis
side effects cialis Tadal Access can you purchase tadalafil in the us
india pharmacy cialis: cheap cialis pills uk – п»їwhat can i take to enhance cialis
buy facebook ads account https://ad-accounts-for-sale.work
cialis daily: cialis ingredients – vardenafil tadalafil sildenafil
buy google adwords accounts https://buy-ads-account.top
https://tadalaccess.com/# cialis 100mg review
buy google ad account https://buy-ads-accounts.click
tadalafil tablets erectafil 20 Tadal Access buy cialis online in austalia
Such a valuable read.
buy fb ads account buy facebook profiles
cialis buy australia online: TadalAccess – cialis 20 mg best price
https://tadalaccess.com/# original cialis online
cialis online usa TadalAccess how long does it take cialis to start working
google ads agency accounts buy google ads agency account
buy google adwords accounts https://ads-account-buy.work
https://tadalaccess.com/# cialis 5 mg for sale
cialis savings card TadalAccess cialis 20 mg coupon
cialis soft: cialis cheapest price – cialis tadalafil 20mg price
when will teva’s generic tadalafil be available in pharmacies: how long for cialis to take effect – cialis generic canada
https://tadalaccess.com/# cialis online without pres
cialis side effects heart TadalAccess cialis pills for sale
buy google ads account https://buy-ads-invoice-account.top
cialis at canadian pharmacy: TadalAccess – cialis effects
what are the side effect of cialis: best place to get cialis without pesricption – cialis w/dapoxetine
buy adwords account buy old google ads account
sell google ads account https://buy-ads-agency-account.top
https://tadalaccess.com/# cialis 5 mg tablet
I took away a great deal from this.
cialis canada over the counter [url=https://tadalaccess.com/#]TadalAccess[/url] buying cialis generic
cheapest cialis: TadalAccess – generic cialis from india
google ads reseller https://sell-ads-account.click/
https://tadalaccess.com/# cialis contraindications
generic tadalafil in us: Tadal Access – cialis dopoxetine
ambrisentan and tadalafil combination brands tadalafil 20mg cialis in las vegas
buy tadalafil powder: cialis pills – where to buy cialis cheap
https://tadalaccess.com/# cialis for sale
google ads agency accounts https://ads-agency-account-buy.click
levitra vs cialis: TadalAccess – canada cialis for sale
cialis on sale TadalAccess online cialis australia
us pharmacy prices for cialis: tadalafil 5 mg tablet – cialis bathtub
https://tadalaccess.com/# cialis 20 mg
how long before sex should you take cialis: TadalAccess – cialis generico
buy bm facebook buy facebook business account
buy google ads buy verified google ads account
order cialis online no prescription reviews Tadal Access tadalafil prescribing information
https://tadalaccess.com/# cialis sample request form
I’ll surely return to read more.
best price on generic cialis: TadalAccess – cialis mit paypal bezahlen
https://tadalaccess.com/# cialis canada prices
when should you take cialis cialis online paypal sublingual cialis
business manager for sale https://buy-bm-account.org/
cialis for daily use: Tadal Access – maxim peptide tadalafil citrate
facebook bm buy buy-business-manager-acc.org
buy facebook verified business account https://buy-verified-business-manager-account.org
More content pieces like this would make the web more useful.
facebook bm account https://buy-verified-business-manager.org/
https://tadalaccess.com/# cialis from canada to usa
cialis for performance anxiety: Tadal Access – who makes cialis
cialis canada online: cialis same as tadalafil – cialis over the counter usa
https://tadalaccess.com/# buying cialis in mexico
cialis 20 mg coupon Tadal Access buying cialis
cialis daily side effects: cialis coupon code – cialis prices at walmart
https://tadalaccess.com/# cialis online delivery overnight
buy cialis online reddit: TadalAccess – cialis online without prescription
buy facebook business account facebook business account for sale
facebook bm account facebook business account for sale
verified bm buy fb bm
https://tadalaccess.com/# where can i buy cialis on line
natural alternative to cialis: buy generic cialis – cialis savings card
cialis pricing TadalAccess cialis prescription online
cialis daily dosage: Tadal Access – cialis drug
facebook verified business manager for sale verified-business-manager-for-sale.org
https://tadalaccess.com/# tadalafil liquid review
buying cialis online canadian order: Tadal Access – cialis a domicilio new jersey
cialis vs.levitra: TadalAccess – cheap generic cialis canada
business manager for sale https://buy-business-manager-accounts.org/
cialis same as tadalafil what happens when you mix cialis with grapefruit? what is cialis prescribed for
tiktok ads account for sale https://buy-tiktok-ads-account.org
tiktok ads account buy buy tiktok business account
https://tadalaccess.com/# online tadalafil
cheap tadalafil no prescription: TadalAccess – cialis effect on blood pressure
cialis from canada to usa: order cialis online – buy cialis generic online 10 mg
cialis 20mg price TadalAccess us cialis online pharmacy
https://tadalaccess.com/# achats produit tadalafil pour femme en ligne
buy cialis without a prescription: cialis is for daily use – what does generic cialis look like
dapoxetine and tadalafil TadalAccess cialis discount coupons
levitra vs cialis: cialis las vegas – cialis used for
buy tiktok ads buy tiktok business account
buy tiktok ads https://tiktok-agency-account-for-sale.org
buy tiktok ads account https://buy-tiktok-ad-account.org
online pharmacy cialis Tadal Access tadalafil citrate research chemical
buy tiktok ads https://buy-tiktok-ads-accounts.org
how long does cialis last 20 mg: what is the normal dose of cialis – cialis going generic
cheap cialis dapoxitine cheap online cialis free trial canada cialis softabs online
https://tadalaccess.com/# cialis price south africa
cialis 30 mg dose: Tadal Access – does cialis make you harder
prescription for cialis cialis online without a prescription cialis from canada to usa
tiktok ad accounts https://buy-tiktok-ads.org
buy tiktok ad account https://buy-tiktok-business-account.org
were can i buy cialis: cialis package insert – buy generic cialis
https://tadalaccess.com/# where can i buy cialis online in canada
buy tiktok business account https://tiktok-ads-agency-account.org
cialis over the counter usa buy liquid tadalafil online cialis 5mg coupon
https://tadalaccess.com/# cialis payment with paypal
difference between tadalafil and sildenafil TadalAccess cialis from india
cialis side effect: cialis where to buy in las vegas nv – prescription for cialis
https://tadalaccess.com/# cialis 10mg reviews
cialis website: buying cheap cialis online – what does cialis do
tadalafil tablets 20 mg global Tadal Access cialis superactive
does cialis make you last longer in bed: buy voucher for cialis daily online – order cialis no prescription
take cialis the correct way buy generic tadalafil online cheap cialis 20 mg
https://tadalaccess.com/# cialis copay card
cialis 2.5 mg: cialis 20 mg – canadian cialis
https://tadalaccess.com/# cialis reviews
cialis dapoxetine australia TadalAccess tadalafil online canadian pharmacy
does medicare cover cialis buying cialis in canada tadalafil without a doctor prescription
This submission is excellent.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
buy antibiotics from india: best online doctor for antibiotics – buy antibiotics
Online medication store Australia: PharmAu24 – PharmAu24
https://eropharmfast.com/# ed meds by mail
buy antibiotics online: Biot Pharm – over the counter antibiotics
Online drugstore Australia Online drugstore Australia online pharmacy australia
More content pieces like this would make the internet a better place.
ed medicines online: Ero Pharm Fast – erectile dysfunction pills online
Thanks for sharing. It’s top quality.
Licensed online pharmacy AU: Pharm Au24 – Discount pharmacy Australia
Such a valuable read.
I absolutely valued the style this was laid out.
This submission is fantastic.
https://eropharmfast.com/# online ed pills
I’ll surely bookmark this page.
Pharm Au24 Discount pharmacy Australia Online medication store Australia
Ero Pharm Fast: what is the cheapest ed medication – Ero Pharm Fast
pharmacy online australia: Pharm Au24 – Pharm Au24
buy antibiotics for uti: BiotPharm – over the counter antibiotics
This is the kind of writing I truly appreciate.
You’ve evidently done your homework.
Such a valuable read.
I particularly liked the manner this was laid out.
Ero Pharm Fast: pills for erectile dysfunction online – ed online treatment
Buy medicine online Australia: Online medication store Australia – Medications online Australia
Pharm Au 24 Medications online Australia pharmacy online australia
PharmAu24 Pharm Au 24 Discount pharmacy Australia
I’ll gladly return to read more.
https://eropharmfast.com/# Ero Pharm Fast
This piece is outstanding.
More posts like this would make the blogosphere more useful.
https://pharmau24.com/# Licensed online pharmacy AU
cheap ed medicine Ero Pharm Fast Ero Pharm Fast
Ero Pharm Fast online ed meds Ero Pharm Fast
Such a practical insight.
https://biotpharm.shop/# get antibiotics quickly
I took away a great deal from this.
PharmAu24 Online drugstore Australia pharmacy online australia
https://ciasansordonnance.com/# acheter Cialis sans ordonnance
I genuinely admired the approach this was explained.
Pharmacies en ligne certifiees pharmacie internet fiable France vente de mГ©dicament en ligne
Pharmacies en ligne certifiees: trouver un mГ©dicament en pharmacie – pharmacie en ligne france livraison belgique
http://pharmsansordonnance.com/# pharmacie en ligne pas cher
Achat médicament en ligne fiable: pharmacie en ligne france – pharmacie en ligne france fiable
I’ll gladly return to read more.
Such a valuable read.
acheter kamagra site fiable: kamagra gel – livraison discrete Kamagra
acheter Kamagra sans ordonnance: kamagra oral jelly – kamagra oral jelly
I absolutely enjoyed the approach this was explained.
This is the kind of post I find helpful.
livraison discrète Kamagra: kamagra en ligne – kamagra 100mg prix
kamagra en ligne: acheter kamagra site fiable – kamagra livraison 24h
kamagra pas cher: acheter kamagra site fiable – acheter Kamagra sans ordonnance
kamagra en ligne: kamagra gel – acheter kamagra site fiable
pharmacie en ligne avec ordonnance: acheter kamagra site fiable – kamagra gel
https://ciasansordonnance.com/# cialis prix
More content pieces like this would make the web more useful.
I particularly liked the manner this was explained.
Such a useful resource.
I took away a great deal from this.
acheter mГ©dicament en ligne sans ordonnance: Cialis pas cher livraison rapide – pharmacie en ligne livraison europe
Thanks for sharing. I read many of your blog posts, cool, your blog is very good. https://accounts.binance.com/es-MX/register?ref=JHQQKNKN
pharmacie en ligne sans ordonnance: pharmacie en ligne france fiable – Achat mГ©dicament en ligne fiable
More posts like this would make the web a better place.
Cialis sans ordonnance 24h: cialis prix – traitement ED discret en ligne
cialis sans ordonnance acheter Cialis sans ordonnance Acheter Cialis
commander Cialis en ligne sans prescription: Cialis pas cher livraison rapide – acheter Cialis sans ordonnance
I took away a great deal from this.
I genuinely liked the style this was presented.
http://pharmacieexpress.com/# est-ce que je peux acheter du monuril sans ordonnance ?
donde comprar tadalafil sin receta: farmacia online polonia – comprar ivermectina sin receta
venta online farmacia: codigo descuento mi farmacia online – pildora dia de despues norlevo sin receta]
elocom crema se puede comprar sin receta farmacia veterinaria online telefono xanax farmacia online
compras farmacia online: Confia Pharma – farmacia gt online
sildenafil mylan 100 mg achat en ligne: peut on acheter du viagra en pharmacie sans ordonnance en espagne – pharmacie avec ordonnance en ligne
farmacia doctor max online: farmacia tienda inglesa online – farmacia mia online
eskim 1000 prezzo esomeprazolo 40 mg prezzo pantorc 20 prezzo
imovane ordonnance sГ©curisГ©e: ketum gel – comment obtenir une ordonnance
sterdex prix sans ordonnance: Pharmacie Express – derinox équivalent sans ordonnance
I’ll definitely bookmark this page.
comprar doxiciclina sin receta: Confia Pharma – mi farmacia online facebook
http://pharmacieexpress.com/# desloratadine sans ordonnance en pharmacie
infection urinaire ordonnance: derinox sans ordonnance en pharmacie – peut on acheter des ovules en pharmacie sans ordonnance
mГ©dicaments pour infection urinaire sans ordonnance pharmacie sans ordonnance en ligne ventoline en pharmacie sans ordonnance
pillola aglae: si può dare il bentelan al cane – dicloreum 150 mg prezzo
se puede comprar buscapina sin receta mГ©dica: Confia Pharma – farmacia online americana
comprar prozac sin receta en españa: farmacia granero online – farmacia online espanha
azitromicina comprar sin receta: farmacia hours online – comprar mascarillas farmacia online
esteclin sciroppo niklod 200 prezzo atenololo 50 mg prezzo
http://confiapharma.com/# cialis comprar sin receta
pillola naomi: effiprev prezzo – farmacia online genova
viagra sans ordonnance pharmacie: Pharmacie Express – augmentin sans ordonnance en pharmacie
peut on voir un cardiologue sans ordonnance: aerius sans ordonnance en pharmacie – finastéride prix
fucidine sans ordonnance en pharmacie: Pharmacie Express – nystatine sans ordonnance
farmacia online forum Confia Pharma puedo comprar nolotil sin receta
canal farmacia online: donde puedo comprar amoxicilina sin receta medica – se puede comprar stopcold sin receta
spirale mirena: eutirox prezzo senza ricetta – puntura decapeptyl 3 75 prezzo
farmacia linfa palermo: zindaclin gel prezzo – supracef 400 prezzo
mustela parfum immunostim sachet xenical sans ordonnance
https://farmaciasubito.com/# flantadin 30 mg prezzo
puedo comprar bactrim sin receta: farmacia compras online – mascarillas quirГєrgicas farmacia online
advantan emulsione: procoralan 5 mg prezzo – lacirex a cosa serve
puedo comprar clonazepam sin receta mejor farmacia online madrid donde comprar medicamentos sin receta
se puede comprar viagra sin receta ?: puedo comprar aspirina sin receta – comprar galotam 50 sin receta
laurea farmacia online: Confia Pharma – donde puedo comprar metformina sin receta
farmaciasdirect.com farmacia online y parafarmacia online: Confia Pharma – farmacia firenze online
comprar corticoides sin receta farmacia catena online farmacia online loreto
I’ll definitely return to read more.
http://pharmacieexpress.com/# antibiotique large spectre sans ordonnance
farmacia san vicente del raspeig online: se puede comprar viagra sin receta ? – donde puedo comprar mifepristona sin receta
vermox sciroppo: farmacia online genova – farmacia ГЁ online
descuento farmacia en casa online: se puede comprar diflucan sin receta medica – miglior farmacia online
humalog kwikpen 100: clobesol unguento prezzo – cefixoral 400 prezzo con ricetta
comprar pildora del dia despues sin receta quГ© viagra se puede comprar sin receta farmacia online albacete
This is the kind of post I value most.
brufen 600 farmacia online: stilbiotic collirio – spasmex generico prezzo
minias principio attivo: serpens 320 mg prezzo – farmacia online romania
http://farmaciasubito.com/# farmacia online lombardia
acheter saxenda sans ordonnance malarone prix eroxon en pharmacie france sans ordonnance
farmacia online scalapay: cГіdigo descuento farmacia online 24 pm – farmacia online neostrata
cortisone cane pipГ¬: omnic a cosa serve – colecalciferolo 25000
levitra sans ordonnance en pharmacie Pharmacie Express generique pilule jasmine
se puede comprar bilaxten sin receta: farmacia online singuladerm – farmacia pedido online
se puede comprar pastilla del dia despues sin receta: Confia Pharma – farmacia online spedizione estero
test rapido covid farmacia online: puedo comprar tardyferon sin receta – se puede comprar antibiГіtico sin receta en portugal
20 gocce di toradol: Farmacia Subito – sonirem principio attivo
farmacia online piu conveniente: Farmacia Subito – navizan 4 mg prezzo
canada farmacia online Confia Pharma farmacia seguro online
noremifa bustine: cilodex gocce prezzo – megavir in farmacia
riopan gel bustine: gibiter prezzo – celebrex prezzo mutuabile
mesalazina 800 Farmacia Subito crema emla prezzo
monurol 3g se puede comprar sin receta: farmacia ibaГ±ez online – farmacia andorra venta online
http://farmaciasubito.com/# farmacia online bologna
pharmacie canada sans ordonnance: cialis prix – cialis generic
zitromax sciroppo bambini prezzo: mascherine ffp2 bambini farmacia online – foster inalatore prezzo
farmacia ourense online Confia Pharma farmacia omeopatica online
bentelan 1 mg prezzo: Farmacia Subito – siti farmacia online
https://pharmacieexpress.shop/# durex elite
urixana bustine efexor 37 5 prezzo rosumibe 10/10 prezzo
deniban principio attivo: pillola del giorno dopo norlevo prezzo – dona bustine prezzo
mГ©dicament angine sans ordonnance: tamsulosine sans ordonnance pharmacie – peut on avoir une ordonnance en ligne
comprar antibiГіticos andorra sin receta: farmacia + online – donde comprar viagra sin receta en andorra
brufen effervescente: spasmex generico prezzo – metformina 850 originale
101farmacias.com farmacia online barata se puede comprar ibuprofeno 600 sin receta pantogar farmacia online
master farmacia online: farmacia san vito – cardiazol paracodina gocce senza ricetta
se puede comprar desloratadina sin receta farmacia sant’anna online terracortril se puede comprar sin receta
https://pharmacieexpress.com/# peut on aller dans une pharmacie de garde sans ordonnance
farmacia online envГo canarias: Confia Pharma – comprar benzodiacepinas sin receta
farmacia dietetica online: comprar pastilla anticonceptiva sin receta – puedo comprar meloxicam sin receta
donde puedo comprar amoxicilina sin receta: comprar nembutal online sin receta – sildenafil farmacia online
farmacia online eguilleor se puede comprar antibiГіticos sin receta comprar positon sin receta
Вїque Гіvulos se pueden comprar sin receta?: Confia Pharma – farmacia de guardia serie completa online
solupred 20 mg sans ordonnance shampooing caudalie huile caudalie visage
http://pharmacieexpress.com/# coquelusedal ordonnance
fentanil cerotto 12 mcg prezzo: Farmacia Subito – lyrica 25 mg prezzo
mascherine ffp2 bambini farmacia online: ginoden prezzo – blopress 16 mg prezzo
curso auxiliar farmacia online: Confia Pharma – cГіdigo descuento farmacia online 24 pm
farmacie sicure online: monuril prezzo – niklod 200
etoricoxib se puede comprar sin receta bravecto farmacia online codigo descuento farmacia muy barata online
minias gocce a cosa serve: shop farmacia online italia – busette principio attivo
slowmet 500 prezzo: forxiga prezzo – xanax gocce prezzo
https://confiapharma.com/# se puede comprar la pildora anticonceptiva sin receta en espaГ±a
farmacia san michele montemerlo farmacia online. sirdalud compresse prezzo
mГ©dicament contre cystite sans ordonnance: vermifuge chaton pharmacie sans ordonnance – somnifГЁre puissant sans ordonnance en pharmacie
farmacia veterinaria online canarias farmacia online mascarillas fpp3 comprar sin receta viagra
buy viagra online in india medplus pharmacy india best online pharmacy
pharmacy course india: online medicine india – medical store online
rx pharmacy coupon: xl pharmacy cialis – sams club pharmacy lipitor
http://inpharm24.com/# india pharmacy delivery to usa
п»їindia pharmacy InPharm24 online india pharmacy reviews
https://inpharm24.shop/# god of pharmacy in india
how to start a pharmacy store: Pharm Express 24 – pharmacy cialis
online medicine order: god of pharmacy in india – reliable pharmacy india
people’s pharmacy lipitor: lexapro pharmacy coupons – reputable online pharmacy
https://pharmexpress24.shop/# people’s pharmacy lisinopril
cipro online pharmacy: metoprolol online pharmacy – ambien india pharmacy
rite aid online pharmacy: Pharm Express 24 – ventolin inhaler inhouse pharmacy
http://pharmexpress24.com/# premarin online pharmacy
reliable pharmacy india: india pharmacy market outlook – pharmacy india online
mexican pharmacy near yuma where to buy wegovy in mexico acyclovir mexico pharmacy
I really admired the style this was presented.
pharmacy in india online list of pharmacies in india best online pharmacy in india
medicine online india: best online indian pharmacy – india online pharmacy international shipping
what drugs can you buy over the counter in mexico: best canadian pharmacy that ships to us – adderall from mexican pharmacy
india pharmacy delivery to usa: buy medicines online in india – online india pharmacy
http://inpharm24.com/# india pharmacy market
buy drugs from india: online medicines india – india meds
apollo pharmacy india: indian online pharmacy – buy medicines online in india
right source pharmacy: provigil online us pharmacy – metoprolol succinate online pharmacy
mexican on line pharmacy mexican online pharmacy wegovy otc drugs mexico
buy medicine online: ivermectin india pharmacy – indian pharmacy
provigil pharmacy propecia price pharmacy Brand Levitra
new zealand pharmacy domperidone: Pharm Express 24 – target pharmacy levitra
cialis us pharmacy: imiquimod pharmacy – premarin pharmacy coupon
http://pharmexpress24.com/# the peoples pharmacy
nogales mexico pharmacy: buy wegovy in mexico – hispanic pharmacy near me
drugs from india: pharmacy india – sun pharmacy india
pharmacy in india b pharmacy salary in india india online pharmacy
order medicines online: InPharm24 – get medicines from india
best canadian pharmacy that ships to us Pharm Mex safe mexican online pharmacy
online india pharmacy reviews: e pharmacy india – pharmacy website in india
pharmacy choice cetirizine: Pharm Express 24 – Cytoxan
https://inpharm24.shop/# india pharmacy delivery
india mail order pharmacy: buy viagra online in india – pharmacy india
usa online pharmacy: Pharm Mex – mounjaro mail order
mexican pharmacy online reviews: Pharm Mex – codeine mexico
cialis pharmacy cost cytotec online pharmacy senior rx care pharmacy
online overseas pharmacy: Pharm Express 24 – rx health mart pharmacy
pharmacy express: Pharm Express 24 – cymbalta mail order pharmacy
ivermectin india pharmacy: InPharm24 – buy medicine from india
https://pharmexpress24.shop/# accutane northwest pharmacy
cialis online pharmacy: Pharm Express 24 – buy hcg online pharmacy
percocet online pharmacy: kroger pharmacy store locator – viagra us pharmacy
medicine delivery in vadodara: best indian pharmacy – overseas pharmacy india
Aggrenox caps: xlpharmacy review viagra – online pharmacy overnight shipping
http://inpharm24.com/# online medicine in india
best indian pharmacy: india pharmacy international shipping – online pharmacy in india
online medicine india doctor of pharmacy india india pharmacy international shipping
meds from mexico: Pharm Mex – mexican pharmacies online
best online pharmacy in india: india pharmacy of the world – india pharmacy international shipping
mexico farmacia online mexico percocet how much is mounjaro in mexico
Such a helpful read.
Advair Diskus: rx pharmacy online – online generic pharmacy
india pharmacies: InPharm24 – india meds
http://pharmmex.com/# amoxicillin in mexico over the counter
drugs from india: InPharm24 – online medicine order
international drug store: can i order online from a mexican pharmacy – ketamine mexican pharmacy
I truly liked the style this was laid out.
http://inpharm24.com/# buy medicines online in india
med store pharmacy: 1st rx pharmacy statesville nc – online pharmacy fioricet
reputable online pharmacies mexican pharmacy hgh safe mexican pharmacy
india meds: divya pharmacy india – e pharmacy india
buy pain relievers online cialis mexican pharmacy arecov mexican pharmacy
pharmacy online india: InPharm24 – india medical
registration in pharmacy council of india: india online pharmacy – buy medicine from india
can you buy viagra over the counter in mexico pharmacy tijuana is mexican rx pharmacy legit
pharmacy name ideas in india: india rx – india pharmacy reviews
dandruff shampoo india pharmacy: pharmacy names in india – medicine online purchase
https://inpharm24.shop/# india pharmacy ship to usa
tops pharmacy adipex diet pills online pharmacy cialis online pharmacy reviews
ventolin hfa online pharmacy: Pharm Express 24 – polish pharmacy online usa
india pharmacy of the world aster pharmacy india medicines online india
female viagra no prescription VGR Sources where to buy female viagra in australia
https://vgrsources.com/# cheap viagra pills online
viagra where to buy canada: VGR Sources – where to buy female viagra
best price for viagra 100mg: buy viagra canada online – how to get a viagra prescription
generic viagra sildenafil 100mg: VGR Sources – viagra online sale in india
sildenafil purchase india: VGR Sources – sildenafil pills canada
https://vgrsources.com/# best viagra over the counter
viagra mexico online: VGR Sources – sildenafil sale in india
buy female viagra from united states VGR Sources where to buy generic viagra online
how to purchase viagra online: VGR Sources – generic viagra online prescription
sildenafil soft tablets viagra uk best price where can i get viagra in canada
us pharmacy viagra prices: VGR Sources – purchase viagra tablets
canada viagra online: viagra where to buy over the counter – viagra 50mg generic
female viagra pills: buy generic viagra online australia – sildenafil 100 mg tablet usa
sildenafil for sale: VGR Sources – viagra best buy india
viagra pills online usa: VGR Sources – buy generic viagra with paypal
https://vgrsources.com/# female viagra online buy
where to buy viagra in us VGR Sources can i buy viagra over the counter in mexico
viagra brand canada: VGR Sources – real viagra online no prescription
viagra 100mg online price in india: order viagra soft tabs – viagra soft tabs
online viagra VGR Sources buy viagra gel
Thanks for posting. It’s a solid effort.
viagra super active price: buy viagra over the counter – generic viagra pills
viagra for sale online australia: viagra over the counter australia – best price for viagra 100mg
https://vgrsources.com/# buy viagra visa
viagra 50mg online in india: generic viagra for sale in canada – viagra men
viagra without prescription in united states: viagra tablets 100mg – viagra nz buy
where to buy generic sildenafil: canadian pharmacy online viagra – sildenafil pharmacy uk
where to buy viagra online uk viagra 125 mg buy viagra australia paypal
cheap generic viagra uk: where to buy sildenafil citrate – viagra 150 mg
viagra 100 price in india: viagra prescription online canada – buy viagra online mastercard
preГ§o do viagra 50mg: female viagra tablet in india online purchase – viagra for sale mexico
viagra pills canada VGR Sources female viagra united states
buy viagra online with mastercard: VGR Sources – cheap viagra for sale canada
sildenafil women: discount generic viagra – viagra without presc uk
https://vgrsources.com/# generic viagra cheapest price
viagra tablet 25 mg: best price viagra uk – generic viagra cheapest
viagra pharmacy australia: buy sildenafil 100mg uk – how to viagra prescription
buy viagra safely online uk VGR Sources canadian pharmacy prescription viagra
viagra 100mg uk: VGR Sources – sildenafil 100mg for sale
More articles like this would make the internet a better place.
where to buy viagra uk: VGR Sources – sildenafil 200mg online
female viagra for women viagra pills price in south africa buy viagra united kingdom
https://vgrsources.com/# buy viagra australia paypal
order female viagra: viagra 50 mg pill – how to buy viagra online safely
discount viagra india: VGR Sources – order viagra pills online
buy real viagra cheap: VGR Sources – sildenafil canada buy
buy female viagra online canada: sildenafil 100mg for sale uk – buy real viagra no prescription
viagra sale: sildenafil buy paypal – generic drug for viagra
female viagra online in india sildenafil 100mg tablets buy online viagra generic in mexico
canadian pharmacy viagra paypal VGR Sources soft tabs viagra
over the counter viagra united states: VGR Sources – sildenafil online mexico
This is the kind of post I truly appreciate.
how to get viagra in mexico: VGR Sources – viagra 50mg online
sildenafil pill cost: VGR Sources – sildenafil online prescription
https://vgrsources.com/# buy generic sildenafil uk
generic viagra 150 mg online: online real viagra – generic viagra australia paypal
buy cheap sildenafil: VGR Sources – how to get viagra united states
where can i buy real viagra online VGR Sources sildenafil 5343
sildenafil 10 mg daily viagra singapore price levitra vs viagra
viagra for female for sale: VGR Sources – buy viagra with discover card
https://vgrsources.com/# online viagra canadian pharmacy online
chewable viagra soft tabs: how to get viagra uk – viagra europe online
sildenafil citrate 100mg tablets: VGR Sources – cialis generic viagra
viagra otc united states: viagra super active – online sildenafil canada
cheap sildenafil citrate tablets: VGR Sources – canadian pharmacy sildenafil
sildenafil canada over the counter: VGR Sources – where to buy real viagra cheap
how to buy generic viagra generic viagra prescription cheap viagra pills for sale
us viagra over the counter can you buy viagra in europe generic viagra singapore
where to buy viagra in canada safely: purchase cheap viagra – 100g viagra
where to get generic viagra online: viagra prescription price – sildenafil 100mg price usa
sildenafil 200mg price: VGR Sources – best canadian pharmacy generic viagra
buy cheap viagra pills online: buy viagra over the counter – generic viagra online fast shipping
viagra for sale online australia: VGR Sources – buy generic viagra no prescription
sildenafil 50mg coupon VGR Sources order sildenafil from canada
generic viagra sold in united states: VGR Sources – viagra price in india
where can i buy generic viagra online VGR Sources otc viagra pills
cheap discount viagra: VGR Sources – real viagra online no prescription
viagra europe online: buy brand viagra – canadian pharmacy generic viagra 100mg
female viagra pills price: generic viagra from canada – viagra tablet
https://vgrsources.com/# where to get viagra in australia
buy viagra online in us: VGR Sources – how to order viagra in india
buy viagra united kingdom: buy viagra from canada online – how can you get viagra
Such a useful insight.
buy sildenafil 25 mg VGR Sources sildenafil 20 mg for sale
can you buy viagra in europe: VGR Sources – viagra sales online
viagra non prescription VGR Sources average cost of viagra pill
sildenafil price comparison uk: how to get generic viagra online – buy generic viagra online in usa
viagra generic in mexico: VGR Sources – viagra 100 mg price canada
viagra mail order: otc female viagra – viagra cost australia
discount viagra online canada: best otc viagra – viagra tablets in india online purchase
viagra 50 mg tablet buy online: sildenafil uk 100mg – where can you buy viagra over the counter
viagra 150 mg online: female viagra online india – viagra 1998
https://vgrsources.com/# buy viagra free shipping
generic sildenafil 20mg cost VGR Sources generic sildenafil online
I absolutely appreciated the manner this was presented.
viagra 50 mg price in usa: VGR Sources – buy viagra in australia
where to order viagra online in canada generic viagra online pharmacy canada walgreens viagra
cheap viagra online uk: online prescriptions for viagra – canadian pharmacy viagra 200 mg
buy female viagra usa: viagra 50 mg tablet price – how much is viagra cost
https://vgrsources.com/# how to buy over the counter viagra
where to buy viagra online in australia: can i buy viagra over the counter in usa – where can i buy viagra online in india
buy viagra online with mastercard: viagra comparison prices – can you buy female viagra over the counter
sublingual viagra: generic viagra 50mg – sildenafil in singapore
brand viagra online australia: VGR Sources – viagra pharmacy over the counter
sildenafil 50 mg online VGR Sources cheap viagra uk paypal
viagra 100mg india price VGR Sources sildenafil 20 mg tablets cost
viagra gel australia,: VGR Sources – viagra 100 mg price in usa
sildenafil pills in india: brand viagra – price of viagra tablets
order viagra 100mg online: VGR Sources – sildenafil 100mg for sale uk
order viagra from canada: over the counter viagra in us – sildenafil where to buy
price of 100mg viagra pharmacy price comparison viagra buy viagra pills canada
https://vgrsources.com/# sildenafil tab 50mg cost
can i buy viagra online from canada: VGR Sources – viagra 100mg tablet online
Such a practical bit of content.
order viagra cheap online order sildenafil online uk cheap viagra australia fast delivery
generic viagra 100mg uk: viagra 100 mg price canada – canada viagra buy
generic sildenafil 40 mg: VGR Sources – generic viagra sold in united states
https://vgrsources.com/# viagra no prescription
generic viagra online mexico: where to buy viagra over the counter – price generic sildenafil
buy viagra generic: viagra brand name – purchase viagra online with paypal
sildenafil 100mg online india: VGR Sources – canadian pharmacy viagra cost
generic sildenafil prescription VGR Sources where to purchase viagra pills
sildenafil buy online usa: viagra in usa – sildenafil 100mg buy online us
viagra 4 sale best price viagra uk pfizer viagra for sale
viagra mastercard: viagra from canada no prescription – brand viagra uk
buy real viagra uk: generic viagra paypal – viagra 100mg cheap price
online viagra purchase: buy viagra generic canada – female viagra singapore
cheap viagra paypal: VGR Sources – best female viagra 2018
buy generic viagra online no prescription: VGR Sources – buy viagra australia paypal
viagra online pharmacies buy generic viagra from india otc sildenafil in us
where can i order real viagra: VGR Sources – viagra suppliers
https://vgrsources.com/# online viagra
average cost of generic prednisone prednisone 2 mg online prednisone
SemagluPharm: Semaglu Pharm – semaglutide 2.5 mg vs 5 mg
Order Rybelsus discreetly: SemagluPharm – semaglutide and depression
how to get semaglutide out of your system fast: semaglutide online prescription – Affordable Rybelsus price
Crestor Pharm: CrestorPharm – Affordable cholesterol-lowering pills
https://semaglupharm.com/# Semaglu Pharm
does lipitor lower blood pressure: Discreet shipping for Lipitor – LipiPharm
Crestor 10mg / 20mg / 40mg online CrestorPharm No doctor visit required statins
No prescription diabetes meds online: what is the starting dose of semaglutide – Semaglutide tablets without prescription
Safe atorvastatin purchase without RX 10 mg lipitor Lipi Pharm
Lipi Pharm: USA-based pharmacy Lipitor delivery – LipiPharm
Crestor mail order USA: Rosuvastatin tablets without doctor approval – CrestorPharm
prednisone 4mg tab: prednisone 20mg nz – Predni Pharm
http://prednipharm.com/# PredniPharm
rybelsus class action lawsuit: Rybelsus side effects and dosage – rybelsus uk
rosuvastatin 5mg pill identifier pink rosuvastatin 5 mg pill identifier Crestor Pharm
Semaglu Pharm: Semaglu Pharm – Semaglu Pharm
https://semaglupharm.com/# how to get semaglutide cheap
SemagluPharm rybelsus 3 mg reviews SemagluPharm
LipiPharm: LipiPharm – LipiPharm
Affordable Lipitor alternatives USA: Lipi Pharm – LipiPharm
Predni Pharm: Predni Pharm – how can i get prednisone
rybelsus dose equivalent to ozempic: п»їBuy Rybelsus online USA – Where to buy Semaglutide legally
SemagluPharm: No prescription diabetes meds online – п»їrybelsus
cheapest semaglutide: SemagluPharm – Semaglu Pharm
prednisone tablet 100 mg: prednisone 20mg online – Predni Pharm
CrestorPharm: Crestor home delivery USA – Crestor mail order USA
does atorvastatin raise blood pressure: Lipi Pharm – LipiPharm
Lipi Pharm: LipiPharm – should lipitor be taken in the morning or evening
https://semaglupharm.shop/# Semaglu Pharm
prednisone where can i buy: prednisone capsules – Predni Pharm
LipiPharm: Online statin drugs no doctor visit – atorvastatin and back pain
SemagluPharm Semaglu Pharm rybelsus side effect
PredniPharm: PredniPharm – best pharmacy prednisone
Crestor Pharm what vitamins should not be taken with crestor? Online statin therapy without RX
Predni Pharm: Predni Pharm – how to buy prednisone
Semaglu Pharm: SemagluPharm – SemagluPharm
online prednisone PredniPharm Predni Pharm
Semaglu Pharm: SemagluPharm – SemagluPharm
Lipi Pharm: LipiPharm – Lipi Pharm
https://semaglupharm.com/# Rybelsus side effects and dosage
LipiPharm: Lipi Pharm – what time of the day is it best to take lipitor
side effects semaglutide: Semaglu Pharm – SemagluPharm
http://lipipharm.com/# LipiPharm
semaglutide price: Buy Rybelsus online USA – Rybelsus for blood sugar control
Safe delivery in the US SemagluPharm semaglutide to tirzepatide conversion
Crestor Pharm: п»їBuy Crestor without prescription – Crestor Pharm
crestor recall Safe online pharmacy for Crestor Order rosuvastatin online legally
Crestor Pharm: CrestorPharm – CrestorPharm
Lipi Pharm: Lipi Pharm – what is atorvastatin good for
when should you take rosuvastatin: CrestorPharm – rosuvastatin crestor
Affordable Lipitor alternatives USA: Lipi Pharm – LipiPharm
zocor vs lipitor LipiPharm Lipi Pharm
Semaglu Pharm: semaglutide sublingual dose – Semaglu Pharm
LipiPharm: LipiPharm – USA-based pharmacy Lipitor delivery
https://prednipharm.com/# prednisone 20 mg tablet
п»їBuy Crestor without prescription: CrestorPharm – Rosuvastatin tablets without doctor approval
Predni Pharm: online order prednisone 10mg – apo prednisone
Order Rybelsus discreetly: Semaglu Pharm – SemagluPharm
https://crestorpharm.com/# CrestorPharm
generic atorvastatin 40 mg images: LipiPharm – Lipi Pharm
Semaglutide tablets without prescription: Semaglu Pharm – Semaglu Pharm
prednisone 5mg daily PredniPharm prednisone price south africa
CrestorPharm: Crestor Pharm – rosuvastatin calcium cost
25 mg prednisone prednisone 12 tablets price Predni Pharm
Affordable Lipitor alternatives USA: Safe atorvastatin purchase without RX – Lipi Pharm
Predni Pharm: PredniPharm – prednisone 10 mg canada
how to get rybelsus for weight loss: Semaglu Pharm – SemagluPharm
Generic Crestor for high cholesterol Crestor Pharm Online statin therapy without RX
image crestor Over-the-counter Crestor USA CrestorPharm
can lipitor cause anxiety: Generic Lipitor fast delivery – Online statin drugs no doctor visit
Crestor home delivery USA: Crestor home delivery USA – rosuvastatin hydrophilic
https://prednipharm.shop/# Predni Pharm
CrestorPharm: Crestor Pharm – does crestor raise blood pressure
https://semaglupharm.com/# Semaglu Pharm
Predni Pharm PredniPharm prednisone for sale without a prescription
SemagluPharm: Rybelsus 3mg 7mg 14mg – Rybelsus 3mg 7mg 14mg
can i eat bananas with atorvastatin: lipitor 10mg – is pravastatin the same as lipitor?
Safe online pharmacy for Crestor: Crestor Pharm – crestor stomach pain
Predni Pharm PredniPharm prednisone 40 mg rx
LipiPharm Lipi Pharm Lipi Pharm
CrestorPharm: crestor lawsuits – CrestorPharm
https://crestorpharm.com/# atorvastatin and rosuvastatin
CrestorPharm: crestor vs lipitor muscle pain – Crestor Pharm
Safe online pharmacy for Crestor: what is rosuvastatin for – CrestorPharm
https://semaglupharm.com/# SemagluPharm
CrestorPharm CrestorPharm Generic Crestor for high cholesterol
lipitor and blood pressure: USA-based pharmacy Lipitor delivery – atorvastatin 40 mg para que sirve
http://semaglupharm.com/# SemagluPharm
Crestor Pharm Crestor Pharm Crestor Pharm
https://semaglupharm.com/# is semaglutide going away
order prednisone from canada: PredniPharm – Predni Pharm
Rybelsus 3mg 7mg 14mg: Semaglu Pharm – rybelsus effectiveness
https://semaglupharm.com/# switching from semaglutide to tirzepatide dosage
https://semaglupharm.com/# FDA-approved Rybelsus alternative
CrestorPharm Crestor Pharm Crestor Pharm
CrestorPharm: crestor interactions with vitamins – CrestorPharm
http://lipipharm.com/# what’s the difference between lipitor and crestor
can you take berberine with crestor Crestor Pharm Crestor Pharm
difference between rosuvastatin 10 mg and atorvastatin: Lipi Pharm – lipitor nightmares
http://semaglupharm.com/# SemagluPharm
http://semaglupharm.com/# Semaglu Pharm
Semaglu Pharm: Semaglu Pharm – SemagluPharm
https://semaglupharm.shop/# semaglutide online prescription
FDA-approved generic statins online atorvastatin pill picture Affordable Lipitor alternatives USA
Predni Pharm: generic prednisone online – prednisone 20
LipiPharm Generic Lipitor fast delivery Lipi Pharm
Atorvastatin online pharmacy: plavix and atorvastatin – plavix and atorvastatin
http://semaglupharm.com/# Semaglu Pharm
https://semaglupharm.com/# what is the difference between ozempic and semaglutide
Safe online pharmacy for Crestor Generic Crestor for high cholesterol do you take crestor at night or morning
PredniPharm: otc prednisone cream – prednisone prescription for sale
https://crestorpharm.shop/# Order rosuvastatin online legally
Crestor Pharm CrestorPharm Crestor Pharm
https://semaglupharm.com/# compound rybelsus
Crestor Pharm: Crestor Pharm – rosuvastatin 5 mg price without insurance
https://prednipharm.com/# average cost of generic prednisone
SemagluPharm: SemagluPharm – Affordable Rybelsus price
https://semaglupharm.shop/# Semaglutide tablets without prescription
Lipi Pharm: LipiPharm – amlodipine/atorvastatin
SemagluPharm semaglutide weight loss near me SemagluPharm
LipiPharm LipiPharm Generic Lipitor fast delivery
https://semaglupharm.com/# Semaglu Pharm
LipiPharm: LipiPharm – Lipi Pharm
http://semaglupharm.com/# Rybelsus side effects and dosage
http://semaglupharm.com/# rybelsus 7mg tablet
http://crestorpharm.com/# Generic Crestor for high cholesterol
Crestor Pharm: rosuvastatin good rx – Safe online pharmacy for Crestor
https://semaglupharm.com/# SemagluPharm
http://crestorpharm.com/# what to avoid when taking rosuvastatin?
Predni Pharm: prednisone 10mg tablet price – Predni Pharm
https://semaglupharm.com/# SemagluPharm
contraindications of atorvastatin LipiPharm LipiPharm
Rybelsus for blood sugar control: SemagluPharm – Rybelsus online pharmacy reviews
https://semaglupharm.com/# SemagluPharm
https://semaglupharm.com/# Where to buy Semaglutide legally
prednisone 20mg capsule: Predni Pharm – Predni Pharm
semaglutide oral drops Semaglu Pharm SemagluPharm
http://semaglupharm.com/# SemagluPharm
Lipi Pharm: LipiPharm – Lipi Pharm
http://crestorpharm.com/# Generic Crestor for high cholesterol
how much is prednisone 10 mg: Predni Pharm – buy prednisone without a prescription
https://semaglupharm.com/# SemagluPharm
prednisone 5093: buying prednisone – PredniPharm
Lipi Pharm USA-based pharmacy Lipitor delivery Generic Lipitor fast delivery
https://semaglupharm.com/# Semaglu Pharm
rybelsus 14 mg para que sirve SemagluPharm difference between compounded semaglutide and ozempic
https://semaglupharm.shop/# metformin and semaglutide
Atorvastatin online pharmacy: Order cholesterol medication online – what happens if you miss a dose of atorvastatin
Atorvastatin online pharmacy: Order cholesterol medication online – lipitor alcohol
https://semaglupharm.shop/# SemagluPharm
can semaglutide cause pancreatitis: SemagluPharm – SemagluPharm
http://semaglupharm.com/# how to get on semaglutide
rosuvastatin calcium equivalent to rosuvastatin Crestor Pharm CrestorPharm
rosuvastatin and alzheimer’s: CrestorPharm – rosuvastatin pill identifier 5 mg
http://semaglupharm.com/# SemagluPharm
SemagluPharm: Rybelsus for blood sugar control – semaglutide no insurance
http://crestorpharm.com/# п»їBuy Crestor without prescription
side effects of lipitor in the elderly: lipitor vs zetia – LipiPharm
http://semaglupharm.com/# No prescription diabetes meds online
what type of drug is lipitor Atorvastatin online pharmacy atorvastatin what is it used for
http://semaglupharm.com/# Semaglu Pharm
Predni Pharm: prednisone 10 mg online – prednisone over the counter uk
https://semaglupharm.com/# Online pharmacy Rybelsus
can lipitor clean out plaque in your arteries?: Discreet shipping for Lipitor – Cheap Lipitor 10mg / 20mg / 40mg
http://semaglupharm.com/# can i take 2 7 mg rybelsus
Crestor Pharm: Rosuvastatin tablets without doctor approval – does magnesium interfere with crestor
prednisone 10mg price in india PredniPharm prednisone pill 20 mg
when is the best time of day to take crestor Crestor Pharm Crestor Pharm
SemagluPharm: does rybelsus cause dizziness – Affordable Rybelsus price
https://semaglupharm.shop/# does rybelsus cause stomach pain
http://indiapharmglobal.com/# India Pharm Global
canada pharmacy online legit: canadian drug – recommended canadian pharmacies
https://medsfrommexico.shop/# Meds From Mexico
canadian mail order pharmacy: canadian discount pharmacy – pharmacy canadian
Meds From Mexico: Meds From Mexico – purple pharmacy mexico price list
¡Hola, estrategas del azar !
Casinoextranjerosespana.es: juegos sin documentos obligatorios – https://www.casinoextranjerosespana.es/ mejores casinos online extranjeros
¡Que disfrutes de asombrosas triunfos legendarios !
best online pharmacy india: world pharmacy india – best india pharmacy
https://canadapharmglobal.com/# real canadian pharmacy
ed drugs online from canada: Canada Pharm Global – online canadian pharmacy reviews
online pharmacy india best india pharmacy India Pharm Global
https://indiapharmglobal.com/# India Pharm Global
https://medsfrommexico.shop/# Meds From Mexico
https://canadapharmglobal.com/# safe online pharmacies in canada
India Pharm Global: India Pharm Global – India Pharm Global
https://medsfrommexico.com/# Meds From Mexico
medicine in mexico pharmacies Meds From Mexico Meds From Mexico
buy prescription drugs from canada cheap canadian pharmacy victoza canadian pharmacy mall
http://indiapharmglobal.com/# India Pharm Global
¡Hola, apostadores expertos !
Casino sin licencia con promociones diarias – https://www.casinossinlicenciaespana.es/ casino sin licencia
¡Que experimentes victorias legendarias !
the canadian pharmacy: Canada Pharm Global – pharmacy canadian
https://indiapharmglobal.shop/# indian pharmacy paypal
Meds From Mexico Meds From Mexico Meds From Mexico
top 10 pharmacies in india India Pharm Global indian pharmacy online
https://indiapharmglobal.shop/# online shopping pharmacy india
http://canadapharmglobal.com/# canadian pharmacy oxycodone
https://medsfrommexico.shop/# pharmacies in mexico that ship to usa
Meds From Mexico: pharmacies in mexico that ship to usa – Meds From Mexico
canadianpharmacyworld Canada Pharm Global canadian drug stores
canadian pharmacy near me vipps canadian pharmacy 77 canadian pharmacy
¡Saludos, buscadores de tesoros!
Top casinos extranjeros para jugadores espaГ±oles – https://www.casinosextranjerosenespana.es/ casino online extranjero
¡Que vivas increíbles recompensas sorprendentes !
indianpharmacy com: India Pharm Global – pharmacy website india
Meds From Mexico: pharmacies in mexico that ship to usa – Meds From Mexico
Meds From Mexico Meds From Mexico buying prescription drugs in mexico
https://medsfrommexico.com/# Meds From Mexico
http://canadapharmglobal.com/# precription drugs from canada
Meds From Mexico: purple pharmacy mexico price list – pharmacies in mexico that ship to usa
https://canadapharmglobal.shop/# onlinecanadianpharmacy 24
mexican drugstore online Meds From Mexico buying from online mexican pharmacy
mexican online pharmacies prescription drugs medication from mexico pharmacy purple pharmacy mexico price list
canadian mail order pharmacy: canadian pharmacy 24 com – pet meds without vet prescription canada
¡Hola, exploradores del azar !
Casino online fuera de EspaГ±a con juegos instantГЎneos – https://www.casinoonlinefueradeespanol.xyz/# casino online fuera de espaГ±a
¡Que disfrutes de asombrosas premios extraordinarios !
http://indiapharmglobal.com/# online shopping pharmacy india
India Pharm Global: India Pharm Global – Online medicine home delivery
buying prescription drugs in mexico Meds From Mexico purple pharmacy mexico price list
Meds From Mexico: Meds From Mexico – mexican online pharmacies prescription drugs
canada drugs: canadian pharmacy sarasota – canada discount pharmacy
https://raskapotek.com/# apotek tannbleking
apotek fullmaktsskjema: hvordan gi fullmakt apotek – munnsГҐr apotek
https://raskapotek.shop/# apotek støttestrømper
Svenska Pharma: cough syrup apotek – Svenska Pharma
EFarmaciaIt: farmacia dr max milano – flogo fit
EFarmaciaIt EFarmaciaIt EFarmaciaIt
Rask Apotek: Rask Apotek – Rask Apotek
paracetamol 1 g Svenska Pharma nätapotek utan recept
https://papafarma.com/# Papa Farma
https://efarmaciait.com/# EFarmaciaIt
Papa Farma: Papa Farma – farmacia online italia
https://papafarma.com/# Papa Farma
¡Saludos, cazadores de riquezas !
CГіmo acceder desde EspaГ±a a casino online extranjero – https://www.casinoextranjerosenespana.es/# casino online extranjero
¡Que disfrutes de rondas vibrantes !
https://raskapotek.shop/# Rask Apotek
Papa Farma: Papa Farma – Papa Farma
amoxicillina foglietto illustrativo: EFarmaciaIt – EFarmaciaIt
eusom 2 mg a cosa serve augmentin online EFarmaciaIt
Rask Apotek: pinsett apotek – svensk apotek
https://efarmaciait.com/# lantigen b gocce
Papa Farma Papa Farma oral-b io 10 precio
Svenska Pharma: Svenska Pharma – Svenska Pharma
https://papafarma.shop/# mejor farmacia online barcelona
Svenska Pharma: Svenska Pharma – Svenska Pharma
http://raskapotek.com/# Rask Apotek
farmГЎcia perto de mim: Papa Farma – Papa Farma
https://efarmaciait.com/# farmaè lavora con noi
Svenska Pharma finns medicinen pГҐ apoteket hiv test hemma apotek
flexiban mutuabile EFarmaciaIt bijuva amazon
Papa Farma: Papa Farma – vibradores embarazo
Rask Apotek: reseptfri sovemedisin apotek – sopp i navlen apotek
https://papafarma.com/# epiduo forte espaГ±a
http://papafarma.com/# Papa Farma
Papa Farma: aquilea forte opiniones – dodot activity talla 5
https://papafarma.shop/# cialis 5 mg precio
pildora del dia despues prospecto Papa Farma misoprostol venta
Rask Apotek: er apoteket ГҐpent i dag – Rask Apotek
Papa Farma Papa Farma Papa Farma
https://efarmaciait.com/# vermox sciroppo bambini
Rask Apotek: Rask Apotek – Rask Apotek
http://papafarma.com/# sildenafilo online
https://papafarma.com/# farmacia del ahorro españa
Papa Farma: cenforce 100 amazon – productos farmasi
Svenska Pharma influensa test apotek Svenska Pharma
questran bustine prurito: drospil 28 compresse prezzo – ready for it traduzione
sildenafilo 25 mg: epiduo 1 mg/g + 25 mg/g gel – farmacia benidorm cerca de mi
https://svenskapharma.shop/# te till barn
https://efarmaciait.com/# EFarmaciaIt
hansker apotek 24 timer apotek kompresser apotek
bestГ¤ll hem covid test: grГҐ Г¶gon procent – proteinpulver rea
http://papafarma.com/# farmacias vigo 24 horas
¡Saludos, fanáticos del entretenimiento !
casino online extranjero con app intuitiva – https://www.casinosextranjero.es/ mejores casinos online extranjeros
¡Que vivas increíbles victorias épicas !
Svenska Pharma: Svenska Pharma – Svenska Pharma
https://svenskapharma.com/# Svenska Pharma
oppblГҐst mage apotek: apotek open – Rask Apotek
Svenska Pharma Svenska Pharma vilket apotek har snabbast leverans
Papa Farma: dodot 3 plus – avatar 2 online espaГ±ol
Papa Farma diprogenta 0 5 mg/g + 1 mg/g crema para que sirve Papa Farma
reseГ±as mg: Papa Farma – viagra natural farmacia
http://svenskapharma.com/# Svenska Pharma
¡Hola, aventureros de la suerte !
Casino online extranjero con opciones de depГіsito flexibles – https://www.casinoextranjero.es/# casinoextranjero.es
¡Que vivas botes deslumbrantes!
https://papafarma.com/# melatonina en gotas opiniones
¡Bienvenidos, exploradores de la fortuna !
Casino fuera de EspaГ±a sin pasos innecesarios – п»їhttps://casinoporfuera.guru/ casino online fuera de espaГ±a
¡Que disfrutes de maravillosas tiradas afortunadas !
https://papafarma.shop/# farmacia o line
Rask Apotek: lesebriller apotek – Rask Apotek
apotem: kjerringrokk apotek – Rask Apotek
https://efarmaciait.shop/# spazio pharma
EFarmaciaIt EFarmaciaIt EFarmaciaIt
apotek hemleverans: ryggsГ¤ck 30 liter rea – Svenska Pharma
apotek vulkan kvise krem apotek Rask Apotek
apotek pГҐ nett gratis frakt: kanyler apotek – Rask Apotek
https://efarmaciait.shop/# EFarmaciaIt
https://raskapotek.shop/# Rask Apotek
mariatistel apotek: Rask Apotek – Rask Apotek
Papa Farma: pomada brentan para que sirve – Papa Farma
bestill medisin pГҐ nett Rask Apotek blod i avfГёring test apotek
EFarmaciaIt: EFarmaciaIt – EFarmaciaIt
Papa Farma Papa Farma Papa Farma
bestГ¤ll recept online: Svenska Pharma – Г¤gglossningstest billigt
https://papafarma.shop/# sensitive cbd valencia
http://raskapotek.com/# gulfeber vaksine apotek
http://svenskapharma.com/# Svenska Pharma
EFarmaciaIt: EFarmaciaIt – farmacie online
https://svenskapharma.shop/# isotretinoin apotek
Rask Apotek Rask Apotek Rask Apotek
antihistaminer apotek: Rask Apotek – rosmarinolje apotek
metningsmГҐler apotek Rask Apotek Rask Apotek
https://svenskapharma.shop/# chiafrön gravid
https://raskapotek.shop/# Rask Apotek
Papa Farma: Papa Farma – sun 68 opiniones
Rask Apotek: kokosolje apotek – hansker apotek
Svenska Pharma thermometer apotek Svenska Pharma
https://efarmaciait.com/# minoxidil max
Svenska Pharma: hjГ¤rta png – bГ¤sta press-on naglar
Papa Farma Papa Farma Papa Farma
como comprar ozempic: Papa Farma – farmacia precio
https://raskapotek.shop/# Rask Apotek
http://raskapotek.com/# nГҐl til ГҐ ta hull i Гёret apotek
Гёreolje apotek: elektrolytter tilskudd apotek – Rask Apotek
https://svenskapharma.com/# top 10 svenskt godis
https://svenskapharma.shop/# Svenska Pharma
normix fiale EFarmaciaIt EFarmaciaIt
Rask Apotek Rask Apotek apotek 24/7
serum apotek: deo apotek – Svenska Pharma
https://svenskapharma.com/# flytande paracetamol
nicotinamide compresse 250 mg: EFarmaciaIt – EFarmaciaIt
Rask Apotek: godkjente nettapotek – Rask Apotek
https://svenskapharma.shop/# Svenska Pharma
Rask Apotek: Rask Apotek – antihistaminer apotek
https://pharmajetzt.com/# medik
online versandapotheke: online apotheke – apotheke online bestellen
https://pharmaconfiance.com/# Pharma Confiance
https://pharmajetzt.shop/# PharmaJetzt
mijn medicijn.nl: online apotheek recept – MedicijnPunt
apotheke venlo: online apotheke versandkostenfrei – Pharma Jetzt
https://medicijnpunt.com/# medicijne
holland apotheke MedicijnPunt MedicijnPunt
argel 7 oГ№ acheter: Pharma Confiance – dermipred 20 en pharmacie
PharmaConnectUSA ohio pharmacy law adipex PharmaConnectUSA
https://medicijnpunt.com/# medicijnen zonder recept met ideal
Pharma Confiance: Pharma Confiance – Pharma Confiance
http://pharmaconnectusa.com/# us online pharmacy viagra
gГјnstige apotheken: apotheke im internet – apotheke in deutschland
MedicijnPunt: Medicijn Punt – Medicijn Punt
ventes jdd aujourd’hui chat a donner caen gpp lille
Pharma Jetzt Pharma Jetzt medikamente kaufen
https://pharmaconnectusa.com/# no prior prescription required pharmacy
¡Saludos, descubridores de tesoros!
casino online fuera de EspaГ±a con jackpots altos – https://www.casinosonlinefueraespanol.xyz/# casinosonlinefueraespanol
¡Que disfrutes de jackpots fascinantes!
https://pharmaconnectusa.com/# pharmacy cost of cymbalta
https://pharmaconfiance.shop/# Pharma Confiance
internet apotheek: Medicijn Punt – MedicijnPunt
online apotheke auf rechnung: Pharma Jetzt – Pharma Jetzt
apotheek winkel 24 review: apteka internetowa holandia – medicijnen kopen
Pharma Connect USA estrace online pharmacy can you buy viagra at pharmacy
https://pharmaconnectusa.com/# tadalafil usa pharmacy
online apoteken PharmaJetzt die gГјnstigste online apotheke
https://medicijnpunt.com/# Medicijn Punt
¡Hola, descubridores de oportunidades !
Casinosextranjerosdeespana.es – apuesta segura – п»їhttps://casinosextranjerosdeespana.es/ casinosextranjerosdeespana.es
¡Que vivas increíbles instantes únicos !
Pharma Confiance: Pharma Confiance – Pharma Confiance
Pharma Confiance: Pharma Confiance – Pharma Confiance
https://pharmajetzt.com/# internetapotheke
https://pharmajetzt.shop/# apotrke
PharmaJetzt versandapotheke deutschland PharmaJetzt
legit non prescription pharmacies PharmaConnectUSA inducible clindamycin resistance in staphylococcus aureus isolated from nursing and pharmacy students
http://pharmaconfiance.com/# pharmacy in france
https://pharmaconnectusa.com/# internet pharmacy mexico
Pharma Jetzt: PharmaJetzt – apothe online
online medicijnen bestellen zonder recept: Medicijn Punt – apothekers
parapharmacie grenoble: Pharma Confiance – la sante.net avis
https://pharmajetzt.shop/# Pharma Jetzt
medi apotheke PharmaJetzt online apotheke deutschland
Pharma Connect USA PharmaConnectUSA online pharmacy no prescription viagra
http://pharmajetzt.com/# Pharma Jetzt
Pharma Confiance: quelle est la pharmacie de garde aujourd’hui Г brest – produit pharmaceutique
Pharma Confiance: avis sur pharmacie de la chaussГ©e – Pharma Confiance
Pharma Confiance: Pharma Confiance – Pharma Confiance
https://medicijnpunt.com/# apotheke niederlande
online pharmacy new zealand: Pharma Connect USA – drug stores near me
http://medicijnpunt.com/# MedicijnPunt
farma internet apotheek MedicijnPunt
https://medicijnpunt.shop/# MedicijnPunt
protege slip tena noir Pharma Confiance Pharma Confiance
Cefixime: nexium uk pharmacy – PharmaConnectUSA
kroger pharmacy lisinopril: Pharma Connect USA – PharmaConnectUSA
http://medicijnpunt.com/# MedicijnPunt
medicijnen kopen zonder recept: apotek online – medicatie bestellen
beste online apotheek: mijn apotheek – Medicijn Punt
https://pharmaconnectusa.shop/# online pharmacies that use paypal
internetapotheken aphotheke online Pharma Jetzt
versandapotheke bad steben apotheke PharmaJetzt
Pharma Jetzt: luitpold apotheke bad steben – Pharma Jetzt
http://pharmajetzt.com/# Pharma Jetzt
tapis chien incontinent: Pharma Confiance – protection tena femme prix
Pharma Connect USA: PharmaConnectUSA – Pharma Connect USA
https://pharmaconnectusa.com/# Pharma Connect USA
niederlande apotheke: medicijn online bestellen – MedicijnPunt
pharmacie online PharmaJetzt PharmaJetzt
https://pharmaconfiance.shop/# parapharmacie.
apotheek spanje online uw apotheek aptoheek
https://pharmaconfiance.shop/# orthopedie charcot
PharmaJetzt: welche online apotheke ist die gГјnstigste – Pharma Jetzt
https://pharmaconnectusa.shop/# PharmaConnectUSA
PharmaConnectUSA: online pharmacy free viagra samples – PharmaConnectUSA
apo apotheke online: PharmaJetzt – PharmaJetzt
dokter online medicijnen bestellen medicij Medicijn Punt
Pharma Confiance pharmacie des 4 tours 400 mg en g
Pharma Confiance: prix ozempic france – Pharma Confiance
¡Bienvenidos, seguidores de la victoria !
Casino fuera de EspaГ±a con bonos por registro – п»їhttps://casinofueraespanol.xyz/ casino por fuera
¡Que vivas increíbles giros exitosos !
http://medicijnpunt.com/# Medicijn Punt
MedicijnPunt: de apotheker – mijn apotheek medicijnen
https://pharmaconfiance.shop/# Pharma Confiance
pharmacy nl: Medicijn Punt – apotek online
PharmaConnectUSA: Grifulvin V – compounding pharmacy finasteride
gГјnstig online apotheke Pharma Jetzt medikamente deutschland
Pharma Connect USA PharmaConnectUSA 24 hour drug store near me
https://pharmaconfiance.shop/# Pharma Confiance
http://medicijnpunt.com/# online doktersrecept
snel medicijnen bestellen: Medicijn Punt – apotheke online
https://medicijnpunt.shop/# onl8ne drogist
https://pharmaconnectusa.com/# propecia online pharmacy reviews
¡Hola, aventureros del desafío !
casino online fuera de EspaГ±a sin registros complejos – https://casinosonlinefueradeespanol.xyz/# casinosonlinefueradeespanol
¡Que disfrutes de asombrosas oportunidades inigualables !
Medicijn Punt: MedicijnPunt – Medicijn Punt
PharmaConnectUSA: Pharma Connect USA – viagra online us pharmacy
Pharma Confiance avis myvariations ddp woman boutique en ligne
Medicijn Punt: pharmacy nl – mediceinen
https://pharmaconnectusa.shop/# Pharma Connect USA
inhousepharmacy biz domperidone Pharma Connect USA united pharmacy propecia
http://medicijnpunt.com/# Medicijn Punt
PharmaConnectUSA: PharmaConnectUSA – Pharma Connect USA
PharmaJetzt: Pharma Jetzt – medikamente billig
https://medicijnpunt.shop/# MedicijnPunt
medikament online PharmaJetzt PharmaJetzt
Pharma Confiance: Pharma Confiance – laboratoire cooper produits
Farmacia Asequible: Farmacia Asequible – farmacias 24 horas cerca de mi ubicaciГіn
https://rxfreemeds.com/# RxFree Meds
enclomiphene citrate: enclomiphene for men – enclomiphene citrate
cialis online american pharmacy: RxFree Meds – RxFree Meds
https://rxfreemeds.shop/# online pharmacy fluoxetine
Farmacia Asequible: Farmacia Asequible – Farmacia Asequible
RxFree Meds RxFree Meds 24 hour drug store near me
depakote pharmacy canadia online pharmacy RxFree Meds
http://farmaciaasequible.com/# viagra marca blanca
Farmacia Asequible: Farmacia Asequible – donde comprar viagra
https://enclomiphenebestprice.shop/# enclomiphene for sale
reputable online pharmacy viagra: new zealand pharmacy motilium – anti viral
enclomiphene citrate: buy enclomiphene online – enclomiphene online
http://rxfreemeds.com/# online pharmacy prozac no prescription
farmacia online sevilla: Farmacia Asequible – Farmacia Asequible
elocom crema para que sirve [url=https://farmaciaasequible.com/#]Farmacia Asequible[/url] Farmacia Asequible
http://rxfreemeds.com/# RxFree Meds
http://rxfreemeds.com/# RxFree Meds
enclomiphene buy: enclomiphene for men – enclomiphene
viagra in kuwait pharmacy: cialis american pharmacy – escrow pharmacy online
http://rxfreemeds.com/# RxFree Meds
comprar tadalafilo: Farmacia Asequible – Farmacia Asequible
viagra pharmacy checker RxFree Meds RxFree Meds
http://enclomiphenebestprice.com/# enclomiphene testosterone
enclomiphene for men enclomiphene testosterone buy enclomiphene online
enclomiphene citrate: enclomiphene testosterone – enclomiphene buy
RxFree Meds: RxFree Meds – RxFree Meds
http://enclomiphenebestprice.com/# buy enclomiphene online
https://rxfreemeds.shop/# cialis pharmacy checker
https://farmaciaasequible.com/# farmacias 24 horas murcia
enclomiphene online enclomiphene enclomiphene for men
enclomiphene for sale: enclomiphene online – enclomiphene citrate
This write-up is insightful.
RxFree Meds sam’s club pharmacy hours RxFree Meds
http://rxfreemeds.com/# RxFree Meds
RxFree Meds: boots pharmacy omeprazole – propecia generic online pharmacy
mexican pharmacies: clomid mexican pharmacy – cheap pharmacy online
https://rxfreemeds.shop/# RxFree Meds
enclomiphene for sale: enclomiphene for men – buy enclomiphene online
https://rxfreemeds.com/# Seroflo
crema licoforte 40 mg: epiduo forte farmacia – Farmacia Asequible
enclomiphene best price buy enclomiphene online enclomiphene testosterone
farmacias: Farmacia Asequible – crema emla opiniones
enclomiphene for sale enclomiphene enclomiphene
https://rxfreemeds.shop/# fry’s food store pharmacy hours
enclomiphene price: enclomiphene online – enclomiphene for sale
https://rxfreemeds.com/# RxFree Meds
https://enclomiphenebestprice.shop/# enclomiphene
enclomiphene: enclomiphene citrate – enclomiphene best price
RxFree Meds diflucan online pharmacy RxFree Meds
Farmacia Asequible: Farmacia Asequible – Farmacia Asequible
best online pharmacy reddit inhouse pharmacy general motilium RxFree Meds
https://farmaciaasequible.com/# framacia
https://rxfreemeds.shop/# pharmacy artane roundabout
RxFree Meds: RxFree Meds – pharmacy warfarin counseling
discount clomiphene pharmacy: sky pharmacy – pharmacy cialis
http://farmaciaasequible.com/# Farmacia Asequible
ativan online pharmacy: RxFree Meds – RxFree Meds
enclomiphene citrate enclomiphene for men enclomiphene
https://enclomiphenebestprice.com/# enclomiphene buy
men’s health pharmacy viagra: cymbalta pharmacy coupons – RxFree Meds
https://enclomiphenebestprice.com/# enclomiphene for men
citrafleet con receta mГ©dica: farmacias abiertas granada – Farmacia Asequible
buy growth hormone online pharmacy: RxFree Meds – best online pharmacy
https://enclomiphenebestprice.shop/# enclomiphene for men
enclomiphene for sale: enclomiphene online – enclomiphene buy
RxFree Meds RxFree Meds RxFree Meds
https://rxfreemeds.com/# Benemid
RxFree Meds: RxFree Meds – RxFree Meds
Ventolin Trimox klonopin pharmacy online
https://farmaciaasequible.com/# farmadirec
https://rxfreemeds.shop/# RxFree Meds
preferred rx pharmacy: RxFree Meds – wedgewood pharmacy flagyl